Introduction: Arduino Controlled Kitchen Timer
All of you must have experienced this that you have something being cooked on your gas stove while on the other side you get busy watching TV or get a deep conversation that you realize on detecting a smell that it was completely spoiled just because of your carelessness. This little Arduino controlled device is a simple solution to the problem! This is basically a mini battery operated portable kitchen timer which can be set to any number of hours and minutes and tells you with buzzer beeps that the provided time is over. The buzzer is loud enough to be heard even in another room thus can save your dishes from turning burn black. Wonder how great would it be if you made one yourself? Here's how to!
When talking about the inner workings, this project consists of Arduino as the brain, a Liquid crystal display to display time, a buzzer to notify you, two buttons to enter hours and minutes and two LEDs to acting as indicators. Apart from this, it is operated using a 9v battery thus making it small, light weight and portable. As you may have noticed, all of the parts are one of the most basic ones thus you won't have to hunt around. You can enter the number of hours as well as minutes which makes it an accurate device to suite your needs. You will require a maximum of two to three hours to make it.
Here is a short video of the project working:
If you like the concept and documentation of this project, please vote for it in 'Arduino All The Things' contest. On the other side, if you have any doubt, please mention it below. Do follow, like and share for more cool projects!
Subscribe to my YouTube channel here:
Step 1: How It Works?
I always add this step so as to give you the better explanation of the inner workings of this project. This will help you to learn some new things instead of just copying and making the project and will also help you to make some useful modifications. Remember that you should always be sure of the basics before going deeper into things.
So the process begins when you switch the device on and the it asks you to enter the number of hours you want set the timer. Now the body goes into a while() loop with a condition that the loop will keep on running until button 2 is pressed with this type of statement:
Check out the complete source code given in step 9.
while(digitalRead(3) == LOW) // till button 2 is not pressed
This means that pressing button two will exit the loop and the process will move further asking you to enter the minutes. But if you want to increase the number of hours, you need too press button 1 as many times as the number of hours. The following code snippet counts the number of time button 1 is pressed thus the number of hours:
while(digitalRead(3) == LOW) // till button 2 is pressed { if(digitalRead(2) == HIGH) // if button 1 is pressed { delay(500); hour++; //increase number of hours by 1 } lcd.clear(); lcd.print(hour); // print the number of hours delay(100); }
The same process is repeated when asking the number of minutes but this time, counting minutes instead of hours.
Now when all the data has been stored, the timer starts with three for() loops nested to one another each one for hours, minutes and seconds respectively. The loops keep on running until the entered time is finished and also prints the current time left. The following code snippet runs the timer with three for() loops:
<p>e2 = minute;<br> e3 = 0; for(e1 = hour; e1 >= 0; e1--) // loop 1 for hours { for(e2; e2 >=0; e2--) // loop 2 for minutes { for(e3; e3 >= 0; e3--) // loop 3 for seconds { digitalWrite(4, HIGH); // switch on the green LED lcd.clear(); lcd.print(" "); lcd.print("Time Left:"); // print the time left lcd.setCursor(0,1); lcd.print(" "); lcd.print(e1); lcd.print(":"); lcd.print(e2); lcd.print(":"); lcd.print(e3); delay(1000); } e3 = 59; } e2 = 59; }</p>
At the last, after the time is finished, the buzzer beeps along with a red LED glowing. It goes into and infinite loop which never ends until you reset your arduino. The following code snippet explains it:
<p>while(true) // the INFINITE loop<br> { digitalWrite(4, LOW); // sets the green LED off digitalWrite(5, HIGH); // sets the red LED on lcd.clear(); lcd.print("Time Finished!!"); // prints a message digitalWrite(13, HIGH); // beeps the buzzer every 500ms delay(500); digitalWrite(13, LOW); delay(500); }</p>
That's it for the explanation. Hope that this step was useful.
Step 2: Parts and Tools
The following parts and tools will be required to make this project. The total cost including everything is approx. $40 or 2500INR. All of them are basic ones which you already may have in your workshop or if not, you can find them over any hobby online store. The cost can vary with different stores you buy the parts from.
Parts:
- 1x Arduino UNO or Nano or any other compatible
- 1x Liquid Crystal Display (16x2)
- 2x Tactile push buttons
- 2x LEDs (any color)
- 2x 10K resistors
- 2x 330ohm resistors
- 1x 9v battery
- 1x Battery clip
- 1x Toggle switch
- 1x Buzzer
- Jumper wires
- Rainbow wire
- A proper enclosure
Tools:
- Soldering iron
- Solder wire
- Hot glue gun
- Rotatory tool
- Wire cutter/stripper
- Pliers
Step 3: Prep Your Enclosure
The very first step is to prepare the enclosure to as to accommodate all the parts. You should always choose your enclosure wisely and must take care that it fits is your budget and can be worked upon easily. For example you shouldn't use a metal enclosure (such as an ice cube box) for electronic projects as being conductive in nature, it can cause short circuits and you have to insulate it entirely. On the other hand, it can be expensive too. The simplest and cheapest option which I use for my most of the projects is a plastic kitchen ware box. If you want durability, you can use a wooden one as well.
Now about appropriately cutting the enclosure, you want to fix a total of 6 components above the enclosure- An LCD, a switch, 2 buttons and 2 LEDs. Using a drill machine or a multipurpose rotatory tool to cut and drill everything after measuring and marking all the parts. Please wear all necessary safety equipments while working with power tools.
Step 4: Connect the LCD to Arduino
A liquid crystal display is one of the major parts of this project as this is the place where everything from the time left to setting the timer will be displayed. So without a display, this project would simply be useless. Now there are several types of displays- particularly LCDs and LEDs, I would like to mention that this project ONLY deals with the standard 16x2 LCD display with 16 pins and compatible with arduino. So even if you're using a grove LCD (the one with four pins) on this project, it wouldn't work with the same connections and the code. You will need to completely modify the code for it work.
For your LCD, connect it as per the following. Please note that according to these connections, the backlight will be always on. You can modify as per your choice it if you don't want the backlight always on.
- Pin 1 (Vss) on LCD to Arduino Gnd
- Pin 2 (Vcc) on LCD to Arduino 5v
- Pin 3 (Vee) on LCD to 10K Preset or Potentiometer Pin 2 (Middle)
- Pin 4 (Rss) on LCD to Arduino Digital 7
- Pin 5 (R/W) on LCD to Arduino Gnd
- Pin 6 (E) on LCD to Arduino Digital 8
- Pin 11 (DB4) on LCD to Arduino Digital 9
- Pin 12 (DB5) on LCD to Arduino Digital 10
- Pin 13 (DB6) on LCD to Arduino Digital 11
- Pin 14 (DB7) on LCD to Arduino Digital 12
- Pin 15 (LED+) on LCD to Arduino 5v with 100ohm resistor
- Pin 16 (LED-) on LCD to Arduino Gnd
- Pin 1 (Left) on Preset or Potentiometer to Arduino Gnd
- Pin 2 (Right) on Preset or Potentiometer to Arduino 5v
At last, glue the LCD on the enclosure in such a way that the screen is visible from outside.
Step 5: Connect the Push Buttons to Arduino
Two tactile push button added here just to set the timer initially when you switch on the device. In an attempt to add as few control buttons as possible, I ended up with two which would be easier to control. It would also have been possible with just one of them but that would make it a bit complicated. When setting the hours and minutes, the count of hours or minutes is 0. When you press button one, the count increases by one while on pressing button 2, it saves the count and moves ahead.
Connect both the buttons to arduino as per the following. You will need a 10K resistor for each of the buttons.
Button 1:
- Pin 1 to Arduino Digital 2
- Pin 1 through 10K resistor to Arduino Gnd
- Pin 2 to Arduino 5v
Button 2:
- Pin 1 to Arduino Digital 3
- Pin 1 through 10K resistor to Arduino Gnd
- Pin 2 to Arduino 5v
At the end, glue both the buttons at the top of the enclosure at an appropriate position.
Step 6: Add Indicator LEDs
These have no proper use but are just added to make the project a little better. The first LED which is the green one glows when the timer is going on while the second or the red one glows when the time is over. This helps the project to give visual signals along with audio.
Connect both the LEDs as per the following. The recommended colors are red and green but it is not mandatory to use them. You can even skip adding the LEDs. You will need a 330ohm resistor for each LED else they may burn out.
LED 1 (Green):
- Positive to Arduino Digital 4
- Negative through 330ohm resistor to Arduino Gnd
LED 2 (Red):
- Positive to Arduino Digital 5
- Negative through 330ohm resistor to Arduino Gnd
Lastly, glue both the LEDs on the enclosure at their appropriate positions.
Step 7: Connect the Buzzer
A necessary part, this one beeps every 500ms when the set time is finished to give you an audio signal. For the sound to reach you, you must only use a big buzzer rather than the micro one.
Connect the positive terminal of buzzer to Arduino Digital pin 13 and negative terminal to Arduino Gnd. Finally, glue it inside the enclosure. Make a hole near the place you've glued it so that the sound can reach outside.
Step 8: Add Power to the Project
For this project, you have a variety of options when considering the power supply but to follow the aim, the best option can be to use a battery which makes it portable and handy. When it comes on the type of battery to be used, 9v may not be the best option but a cheaper one. Ofcourse, it wouldn't last much long so if you're not satisfied with it, you can use lithium batteries or possibly a power bank. If none of them works, then move to an adapter or a wall wart.
Now for connecting your supply of power, it becomes necessary yet optional to add a switch to turn the device on or off easily without the need to remove the battery every time. Since Arduino UNO and Nano already have a voltage regulator, you can use any power supply between 5-12v without the need of an external voltage regulator (You may notice a regulator in the image above. I added it because the regulator on my arduino was burnt so please ignore it).
Connect the Positive terminal of your battery to the first pin on your switch, the Negative terminal of battery to Arduino Gnd while the second pin of switch to Arduino Vcc (NOT 5v). Finally, glue the battery inside the enclosure and fix the switch in place.
Step 9: Upload the Code
The last step to get your project into action is to upload the code to arduino. Download the attached ino file below and open it through your IDE. You must have the Liquid Crystal library for the code to compile and run else it would show an error. I have also attached the library which you can find below. After extracting the file, copy all the contents under Documents>Arduino>Libraries.
Before uploading the code, make sure the board under Tools>Board and COM port under Tools>Serial Port are correct. After everything is done, finally upload the code.
<p>#include "LiquidCrystal.h"<br>LiquidCrystal lcd(7, 8, 9, 10, 11, 12);</p><p>int e1 = 0; int e2 = 0; int e3 = 0; int hour = 0; int minute = 0;</p><p>void setup() { pinMode(2, INPUT); pinMode(3, INPUT); pinMode(4, OUTPUT); pinMode(5, OUTPUT); pinMode(13, OUTPUT); lcd.begin(16, 2); lcd.clear(); lcd.print("Hey There!"); delay(3000); }</p><p>void loop() { lcd.clear(); lcd.print("Enter Hours"); delay(2000);</p><p>while(digitalRead(3) == LOW) { if(digitalRead(2) == HIGH) { delay(500); hour++; } lcd.clear(); lcd.print(hour); delay(100); } lcd.clear(); lcd.print("Enter Minutes"); delay(2000); while(digitalRead(3) == LOW) { if(digitalRead(2) == HIGH) { delay(500); minute++; } lcd.clear(); lcd.print(minute); delay(100); } delay(1000); e2 = minute; e3 = 0; for(e1 = hour; e1 >= 0; e1--) { for(e2; e2 >=0; e2--) { for(e3; e3 >= 0; e3--) { digitalWrite(4, HIGH); lcd.clear(); lcd.print(" "); lcd.print("Time Left:"); lcd.setCursor(0,1); lcd.print(" "); lcd.print(e1); lcd.print(":"); lcd.print(e2); lcd.print(":"); lcd.print(e3); delay(1000); } e3 = 59; } e2 = 59; } while(true) { digitalWrite(4, LOW); digitalWrite(5, HIGH); lcd.clear(); lcd.print("Time Finished!!"); digitalWrite(13, HIGH); delay(500); digitalWrite(13, LOW); delay(500); } }</p>
Step 10: You're Done!
And, you're finally done making your own Arduino Controlled Kitchen Timer! Now you just have to place it near your oven or your gas stove and alas! This thing will prevent all your dishes and pizzas from burning out. For using the device, first switch it on. The device will ask you to enter the number of hours. Now when you press button 1, the no. of hours will increase by one. When you have selected the right number of hours, press button 2 and it will ask you to enter number of minutes following the same process. After this, the timer begins and the green LED glows. As the time is finished, the buzzer beeps and the red LED starts glowing.
This instructable also comes to an end with this. Hope that you liked this one. Now all I have to say is that do follow, share and vote for this project if you loved it.
Thanks for watching :)
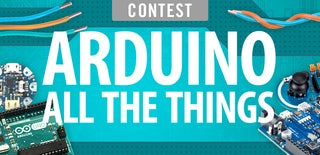
Participated in the
Arduino All The Things! Contest