Introduction: Arduino Most Useless Machine Ever Project
I wanted to get into Arduino projects and introduce my sons to robotics using Arduino. So I thought about building a simple project that made use of an Arduino Uno and a servo. I saw a great post in Instructables about The Most Useless Machine Ever and was inspired to create an Arduino-powered version.
It was a most satisfying build experience with my sons and I learned a lot about programing the Arduino.
How does it work?
The Arduino "listens" to the state of the switch and actuates the servo when the switch is activated.
In the OFF state, the Arduino sends the servo to the reset position (box lid closed). When a user flips the switch to ON, the Arduino sends a signal to the servo and actuates a finger to push the switch to the OFF state.
Step 1: Parts List
Parts list.
You will need:
- wooden jewelry box (you can get these at your local craft store for less than $5)
- Arduino UNO
- Laptop (I use a Macbook Pro)
- 10k ohm resistor
- small SPDT switch
- servo motor
- wires
- 9v battery
- popsicle sticks
- wood glue
- some 0.5" x 0.5 " wooden rod
- old coax cable
- 2 pcs of small hinges (approximately the same size of the hinges that comes with the box)
Tools:
- Dremel tool
- saw
- screw driver
- pliers
- wire stripper
- soldering iron, lead solder
- voltmeter
- breadboard
- electrical tape
Schema:
Check out the schema for the component wiring. I used Fritzing app for Mac to lay out my components.
I recommend wiring up your circuit using a bread board first. Check your servo calibration and play around with the code to achieve the desired rotation or position of your servo arms. This may vary so you need to tweak the code to achieve the right motion.
Step 2: Programming the Arduino
Download the Arduino IDE for your computer and run it. I use a Mac.
Connect the Arduino UNO to the Mac using USB cable. For more info, check out: http://arduino.cc/en/Guide/MacOSX
Below is the code I used to program the Arduino. I modified the "Sweep" code by Barragan.
To make it more interesting, I randomize the speed when the finger retreats into the box.
========== CODE BELOW ========
// Sweep
// by BARRAGAN <http://barraganstudio.com>
// This example code is in the public domain.
#include <Servo.h>
const int buttonPin = 2;
int buttonState = 0;
Servo myservo; // create servo object to control a servo
// a maximum of eight servo objects can be created
int pos; // variable to store the servo position
long timeDelay;
void setup()
{
pinMode(buttonPin, INPUT);
myservo.attach(9); // attaches the servo on pin 9 to the servo object
}
void loop()
{
buttonState = digitalRead(buttonPin); // Read the button position
if (buttonState == HIGH) {
for(pos = myservo.read(); pos >=20; pos -= 1) { // goes from 90 degrees to 20 degrees in 1 step
myservo.write(pos); // tell servo to go to position in variable 'ONpos'
timeDelay = random(15, 30);
delay(15); // randomize wait time for the servo to reach the position
}
}
else {
timeDelay = random(1, 4);
for(pos = myservo.read(); pos <=90; pos += timeDelay) {// goes from 20 degrees to 90 degrees in 1 step
myservo.write(pos); // tell servo to go to position in variable 'OFFpos'
delay(15); // randomize wait time for the servo to reach the position
}
}
}
Step 3: Prepare the Box
Prepare the box.
The design we want is for the lids to open from the top of the box. To do this we would need to cut the lid in half, then re-position the hinges to the left and right side of the box.
1. Remove the metal latch from the front of the lid and the box.
2. Remove the hinges form the back.
3. Draw a line across the lid to indicate where the cut will be made. Note that the line on the side of the lid is angled so when the top opens, the lid does not jam with the other.
4. Cut the lid and smooth out the edges with a file.
5. Make notches to the sides of the box where the hinges will be re-located. Smooth it out with a file and attach the lids on each end.
6. Attach the hinges to the lid and the box. Note that you need an extra pair of hinges as the box only comes with two (you need 4 hinges total).
Step 4: Create Servo Housing and the Finger
Create the housing for the servo that will drive the "finger" that flips the switch off.
1. Measure the servo dimensions and build a housing around it using the 0.5" x 0.5" wood rod. Cut the wood and secure the pieces. I reinforced it with a nail then I glued it together.
2. Fit the servo in the housing and position it on the lid where the SPDT switch will be attached.
3. Drill a hole on the lid and secure the switch. Position the servo housing underneath and align it with the servo levers.
4. Create the "finger" using popsicle sticks. Before creating the finger, i mocked it out by drawing the finger on a piece of paper, cutting it out, then superimposing them on the servo lever. Simulate the rotation of the server lever with the finger attached to see how the finger reaches around to flip the switch off. Once you get the right size, angle, and thickness, overlay the paper mock with the popsicle stick. You can add as many layers of popsicle sticks as you can to create the right thickness so when the finger rotates, it is able to strike the switch. Create holes on the servo-end of the finger where you can attach the finger to the servo lever.
5. Attach the finger to the servo lever by using a copper wire taken from the middle of a coax cable. They can be pretty strong so you will need to use a pair of pliers to cut and bend it. Secure the copper wires by soldering the other side to "lock" the finger to the lever. Be careful not to melt the servo lever.
6. Secure the servo housing underneath the lid with the switch by applying wood glue. Rotate the finger to ensure that it goes around the lid and hits the switch lever nicely while the glue is still wet.. Once you've found the right position, use a clamp to secure the servo housing to the lid as the glue dries.
Step 5: Wiring and Attaching Power Supply
Wire the switches and servo to the Arduino. Refer to the wiring diagram on Step 1.
Note that I soldered the 10k ohm resistor directly to the wires. No need to create a PCB for this simple circuit.
Move the Arduino to the side so when the finger rotates, it does not get in the way.
Attach the 9V power supply to the Arduino. Remember to detach the battery when not in use or else it will drain your battery.
Step 6: Testing the Most Useless Machine Ever
My daughter Kayla's reaction.
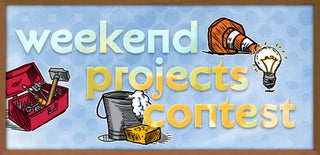
Participated in the
Weekend Projects Contest
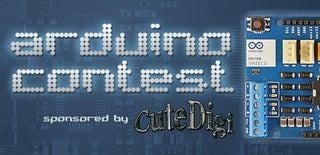
Participated in the
Arduino Contest