Introduction: Control Stuff From the Internet, Create a WIFI Switch.
Learn about relay, WIFI and how to create and internet switch! Use the Wemos D1 Mini (ESP8266) and the relay shield.
Step 1: What Is a Relay
- A relay is an electrically operated switch.
- Most Relays use and Electromagnet to mechanically operate the switch.
- Since relays are switches, the terminology applied to switches is also applied to relays; a relay switches one or more poles, each of whose contacts can be thrown by energizing the coil.
- It is mainly used to control higher voltage circuits with lower voltage. The 'control' and 'controlled' circuits are electrically isolated from each other.
- Normally-Open(NO) : The circuit is disconnected i.e. open when the relay is inactive.
- Normally-Closed(NC) : The circuit is connected i.e. closed when the relay is inactive.
- There are other relays most notably a solid state relay. Relay are extremely common electronics and really easy to use.
Relay come in many different shapes and sizes. In the images above you can see the few examples I have on my desk.
Step 2: Soldering
For me I soldered this relay shield with the females headers with long legs. This give enough room to put a shield on top and connect it to the Wemos board below.
in the video you can see how I draw the solder from one side of the pad to the other. It is a really useful trick that i have picked up. This link will bring you right to it: https://youtu.be/i6sZ_leNkJo?t=1m27s
Step 3: Connecting Your Board
The Board should fit on top of the Wemos board no problem. It may take a little finesse to get it on there.
Step 4: Testing Your Board With Example Code
The images will walk you though how to get this code.
Download the: https://github.com/wemos/D1_mini_Examples
Open the Arduino IDE, go to Sketch > Included Library > Add zip Library. This will prompt you to navigate to the zip file you just downloaded. Once you click opened you will see that it has installed.
Next you want to navigate to File> Example> Wemos D1 Mini Example> Shields > Relay Shields > BlinkWithoutDelay.
Open this example and load it onto you board. see the first part video/instructable for more details on this processes. https://www.youtube.com/watch?v=q2k3CzT5qE0
Step 5: Connecting Your Board, Correctly*
So... I had the shield install incorrectly.
This is a perfect example of why you should start with code that you know works. I know something was wrong with my board or my soldering and it only took me a few seconds to realize I may have it installed backwards.
The first picture is wrong.
The second is correct.
Step 6: Custom Code, Creating the Internet Button
I go over details of the code in the video. You can read through the code and see my comments. Let me now if you have any questions.
//This example will use a static IP to control the switching of a relay. Over LAN using a web browser. //A lot of this code have been resued from the example on the ESP8266 Learning Webpage below. //http://www.esp8266learning.com/wemos-webserver-example.php //CODE START //1 #include <ESP8266WiFi.h> // Below you will need to use your own WIFI informaiton. //2 const char* ssid = "THE WIFI Guest"; //WIFI Name, WeMo will only connect to a 2.4GHz network. const char* password = "52269738"; //WIFI Password //defining the pin and setting up the "server" //3 int relayPin = D1; // The Shield uses pin 1 for the relay WiFiServer server(80); IPAddress ip(10, 0, 0, 99); // where xx is the desired IP Address IPAddress gateway(10, 0, 0, 1); // set gateway to match your network IPAddress subnet(255, 255, 255, 0); // set subnet mask to match your network // void setup is where we initialize variables, pin modes, start using libraries, etc. //The setup function will only run once, after each powerup or reset of the wemos board. //4 void setup() { Serial.begin(115200); delay(10); pinMode(relayPin, OUTPUT); digitalWrite(relayPin, LOW); Serial.print(F("Setting static ip to : ")); Serial.println(ip); // Connect to WiFi network //5 Serial.println(); Serial.println(); Serial.print("Connecting to "); Serial.println(ssid); WiFi.config(ip, gateway, subnet); WiFi.begin(ssid, password); //Trying to connect it will display dots while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } Serial.println(""); Serial.println("WiFi connected"); // Start the server server.begin(); Serial.println("Server started"); // Print the IP address Serial.print("Use this URL : "); Serial.print("http://"); Serial.print(WiFi.localIP()); Serial.println("/"); } //void loop is where you put all your code. it is a funtion that returns nothing and will repeat over and over again //6 void loop() { // Check if a client has connected WiFiClient client = server.available(); if (!client) { return; } // Wait until the client sends some data Serial.println("new client"); while(!client.available()){ delay(1); } // Read the first line of the request String request = client.readStringUntil('\r'); Serial.println(request); client.flush(); //Match the request, checking to see what the currect state is int value = LOW; if (request.indexOf("/relay=ON") != -1) { digitalWrite(relayPin, HIGH); value = HIGH; } if (request.indexOf("/relay=OFF") != -1){ digitalWrite(relayPin, LOW); value = LOW; } // Return the response, build the html page //7 client.println("HTTP/1.1 200 OK"); client.println("Content-Type: text/html"); client.println(""); // do not forget this one client.println("<!DOCTYPE HTML>"); client.println("<html>"); client.print("Relay is now: "); if(value == HIGH) { client.print("Engaged (ON)"); } else { client.print("Disengaged (OFF)"); } client.println("<br><br><br>"); client.println("<a href=\"/relay=ON\">Click here to engage (Turn ON) the relay.</a> <br><br><br>"); client.println("<a href=\"/relay=OFF\">Click here to disengage (Turn OFF) the relay.</a><br>"); client.println("</html>"); delay(1); Serial.println("Client disconnected"); Serial.println(""); }//END
Step 7: Changing the Code for Your Network
The only thing you will need to change are the lines below.
These line hold the information for YOUR LOCAL network. In the video I describe how to find this information.
SSID is the name of your network
password is the password for you network.
You can get it by going to network > Network and sharing center > change adapter settings > Right CLICK on the device you use > Properties > CLICK Internet Protocol Version 4 > CLICK Properties.
const char* ssid = "THE WIFI Guest"; //WIFI Name, WeMo will only connect to a 2.4GHz network. const char* password = "52269738"; //WIFI Password IPAddress ip(10, 0, 0, 99); // where xx is the desired IP Address IPAddress gateway(10, 0, 0, 1); // set gateway to match your network IPAddress subnet(255, 255, 255, 0); // set subnet mask to match your network
You can see that in the second image the serial window will display us some valuable information. This is how we will know if the board was able to connect to our WIFI.
Step 8: Picking Something to Control
You can control just about electronic device with this. It however is mainly used for high voltage like 120vac. In my case I am controlling a fan. The relay on this board is rated for 10A. Some relay may be high or lower be sure to check before connecting anything.
This shield has terminal block with 3 outputs NO (normally open) COM (common) and NC (normally closed).
We will be using NO this will allow the device to be off when everything is powered up. When we engage the relay but supply the coil with 5vdc the connection will be made the the fan will power up.
The NO screw terminal is indicated on the board by a NO. The center terminal in the COM. (not indicated on the board)
You could do this with a light, garage door, or anything.
Step 9: Finishing Up and Testing
Now you can navigate to the IP address you assigned to the board and start turning things on an off.
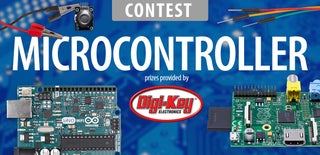
Participated in the
Microcontroller Contest 2017