Introduction: Controlling Arduino RoverBot With TV Remote
I am a poor 15 year old Arduino Hacker, who has little access to parts and materials to build a hi-tech robot. I'm sure there are many others out there who are in my situation. This little Instructable will explain and demonstrate how you can get your Arduino controlled by a TV or other Infrared remote in no time, with (hopefully) no cost at all!
Step 1: Gather Materials
Parts Needed
Arduino project that needs to be remote controlled
IR receiver (easily attained from any old device that accepts input from IR remotes)
IR remote (pretty much any remote works, some may have issues in coding though)
The chassis I used is driven by 2 Tetrix CR Servos with K'Nex wheels Jury-rigged on, and is controlled by an Arduino Uno with a WingShield and an Adafruit MotorShield.
The IR receiver that I used is from an old USB TV remote adaptor that stopped working and had no use. It took me 10 minutes to take it all apart and extract all components.
The IR remote that I used was a handy-dandy little Car MP3 remote. If you want this remote, you can buy the remote with the player here. You can also extract the IR receiver from the MP3 player if you need to.
Step 2: Setting Up the Hardware
You may set up your Arduino in any way you wish. Mine has a Wingshield so I can connect wires to the ports even when a shield is connected. As for the IR receiver, I plugged it into a breadboard with the positive pin going to the 5V terminal (duh), the negative pin to the GND terminal (another duh), and the signal pin to the 11 terminal. You can use any digital PWM terminal that is not being used, and in my case 9 and 10 are controlling the servos, and 11 was right there.
To figure out which pin is which on the IR receiver, just look up the specs of your component. A quick Google search will do the trick.
"IR component pinout" is what I searched, and many results popped up. Just pick the one that looks the closest to your receiver. OR, if you are really lazy, just do the old trial-and-error approach (be very careful, if you mix the pins up the component will heat up and fry itself and possibly your fingers!)
Step 3: Setting Up the Software
Now, for the fun part: figuring out your remote through the computer. In order to make your Arduino react to specific commands received from the remote, you need to tell one signal from another. To do this, we need to set up a IR decoder sketch.
I found a webpage which has a sketch that reads IR and runs based on inputs, and the author explains how it works and some pointers. I took that sketch and modified it to fit my needs. You can easily do the same.
In the sketch on that webpage, it's first line of code says "#include <IRremote.h>". You need to get that library in order for your Arduino to decode the IR. You can find the library here. In that library, there is an example that is called "IRrecord", and it is much easier to use to decode the remote than to make your own. Simply open that example, upload it, and start decoding through the Serial Monitor (SM), like the video demonstrates.
You will see an output in the SM when you push a button on the remote. That's what we are looking for! In my case, 2 is "FF18E7", 3 is "FF7A85", and so on. Keep track of what buttons are what codes, because we will be using them in our code later. My RoverBot is coded to drive forward/backward (2/8), turn left/right (4/6), and stop (5).
The Arduino code example that I have in the next step uses Hex code, which means we need to use the example in the webpage given earlier. My 2 in Hex is 18e7, and my 3 in Hex is 7a85, and so on. It's the same thing, just in different forms.
Step 4: Coding Time!
Once you have the Hex code for the buttons you want, we can get down to coding your Arduino to react!
Just like in the IRrecord program, you need to have the include line:
#include <IRremote.h>
Now you need to tell your Arduino that you are using pin 11 for the IR receiver:
int RECV_PIN = 11;
Initialize your variables:
String in;
String cur = "";
String cur2 = "";
Add this fancy stuff:
IRrecv irrecv(RECV_PIN);
9
10 decode_results results;
Now make your setup:
void setup()
{
Serial.begin(9600);
irrecv.enableIRIn(); // Start the receiver
}
Now, in your loop, you will need an if() block, so the Arduino knows it's looking for IR:
if (irrecv.decode(&results))
Inside this if() block, you need to tell the Arduino to make the variable "in" a string of whatever the Hex code is, then tell it to get the next IR value. My code prints "in" to the SM for debugging purposes.
{
in = String(int(results.value), HEX);
irrecv.resume(); // Receive the next value
Serial.println(in);
}
Now you need if() and else if() blocks to tell your arduino what to do. Here's what I have:
if(in == "8422" || in == "422" || in == "906f" || in == "38c7"){hold();}
else if(in == "841e" || in == "41e" || in == "18e7" || in == "a857"){straight();}
else if(in == "841f" || in == "41f" || in == "4ab5" || in == "e01f"){backup();}
else if(in == "8420" || in == "420" || in == "10ef" || in == "22dd"){left_turn();}
else if(in == "8421" || in == "421" || in == "5aa5" || in == "2fd"){right_turn();}
The first if() looks for the button 5 to be pressed (I have it set up so several remotes will work). After that, you can either have more if() blocks, which will slow the Arduino down if you have enough of them, or you can use else if() blocks. Each block contains a subroutine call, which calls up that specific subroutine to act. This keeps your code from being junked up. An example subroutine looks like this:
void straight()
{
left.write(82);
right.write(101);
}
It's telling the Arduino to turn on the left and right servos at a specific amount so they drive at equal speeds.
Now that we have the core pieces together, we can put it all together!
#include <IRremote.h>
String in;
int RECV_PIN = 11;
IRrecv irrecv(RECV_PIN);
decode_results results;
void setup()
{
Serial.begin(9600);
irrecv.enableIRIn(); // Start the receiver
}
void loop()
{
if (irrecv.decode(&results)) {
in = String(int(results.value), HEX);
irrecv.resume(); // Receive the next value
Serial.println(in);
if(in == <Hex value>){<code>}
else if(in == <Hex value> ){<code>}
else if(in == <Hex value>){<code>}
else if(in == <Hex value>){<code>}
else if(in == <Hex value>){<code>}
}
}
Now you can simply modify, upload, and start controlling your Arduino with a TV remote! Amazing!
Step 5: Est Finis!
Now it's all done! You can mess around with the code as much as you want. There are plenty of ideas out there as to what you can make with Arduino, and now you can make it Remote Controlled! Amazing!
Note: My RoverBot is only glitchy because one of the drive servos is a modified 180 degree servo. It's not a coding glitch.
Enjoy!
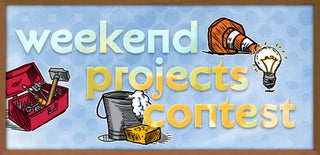
Participated in the
Weekend Projects Contest
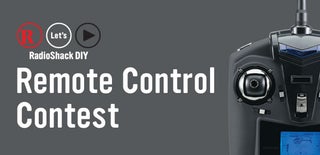
Participated in the
Remote Control Contest
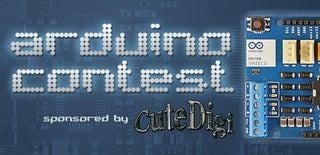
Participated in the
Arduino Contest