Introduction: DIY Glowing Bracelet
This bracelet puts magic at your fingertips! This circuit board bracelet is a sweet addition to any halloween costume, blacklight getup or everyday outfit!
Using a lithium ion battery for power, an ATtiny85 controls four high power LEDs using input from a button. Also, special functions have been implemented to help the ATtiny85 consume less power, so this project should theoretically be able to run for a very long time.
Step 1: Materials
Part | Source |
Attiny85 | (Sparkfun, free samples) |
8 pin IC socket | (Sparkfun, Radioshack) |
Random broken circuit boards | RAM, old motherboard, etc. |
4 High power LEDs (red works best, but white works too) | (Radioshack) |
Resistors (5x 10Ω and 2x 330Ω) | (Sparkfun, Radioshack) |
Female JST | (Sparkfun) |
Small(ish) rechargeable battery | (Sparkfun, Radioshack) |
Small pushbutton | (Sparkfun) |
8x Male headers (right angle) | (Sparkfun) |
8x Female wire connector | (Mouser) |
Sparkfun wish list with all of the parts here
Tools:
Step 2: The Concept
This bracelet is inspired by the fact that red light passes through your skin, while other wavelengths of light are stopped. This is why a red LED is ideal, for all other wavelengths will be absorbed into your skin. Unsurprisingly, light also passes through the skin in your cheeks, which makes for an interesting-looking picture!
I try to upcycle as much as possible, so using broken computer parts was a given. The RAM chips I used were about to be thrown out, so I took them for use in this project! Also, let's be honest, circuit board bracelets look amazing.
Step 3: Preparing the Circuit Boards
To create the body of the bracelet, I used old RAM chips and a small section of an old motherboard. Also, you should cut the protoboard to the correct size and drill the holes now (it makes the creation of the board much easier). These circuit boards needed to be cut, sanded, and drilled, as described below:
- Cut the circuit board into sections approximately ½"x1" using the Dremel's cutting wheel
- Sand the edges of these boards to be rounded so they aren't sharp
- Drill a 4 holes (~5/64") in the circuit boards about the same distance from the edge (see the pictures)
- Clean the debris and rinse with water
Note: This should be done outside or in a well-ventilated area. Anything inhaled during this process may be harmful.
Step 4: Making the Circuit
This simple circuit will allow an ATtiny85 to control 4 LEDs at high power and read a momentary push button. The trick when making this circuit board is to solder everything after the perfboard is cut and drilled. I didn't do that and it would have saved me a lot of time.
To make the ground headers, simply stack two right angle headers on top of each other and solder.
A few notes:
- Since it's such a small board, you may need to run some resistors under the IC holder
- The bottom of the board gets very uncomfortable when touching skin, so I covered the bottom with a layer of Sugru
- If you have any questions about the circuit, ask me in the comments below!
Step 5: Threading the Wire
To hold everything together I used red and black 22 gauge threaded-core wire from RadioShack. The threaded-core (or stranded-core) wire is flexible and less susceptible to breaking from movement, making it idea for this project. First, cut out a length of wire around 1.5 times longer than the actual bracelet. This will give you space to add a little something on each end.
Then, thread the wire through the holes and adjust it so it fits comfortably. You may need to switch around the order of different sized circuit boards to get the most comfort (e.g. putting the smallest ones on the curviest part of your wrist).
At each end, I chose to solder the wires together and cover them in heat shrink. The curve that was created from joining the wires allowed me to easily use a wire tie to close the bracelet.
Step 6: The LEDs
These high-power LEDs connect to the circuit through headers and threaded-core wire that's threaded around itself (otherwise know as a threadception XD).
The length of each wire varies greatly from person to person, so be sure to make careful measurements and a few tests before you solder it all together. Also, make sure it's comfortable when you make a fist because the wires don't stretch around your hand.
Step 7: Programming the ATtiny85
This program uses 5 DIO pins to switch on LEDs and read a button state.
A really cool feature of this code is the fact that it actually puts the ATtiny85 into power-saving mode when not in use, extending the standby battery life A LOT. Also, the program switches off the ADC at the beginning of every program to save even more power.
Every time the button is pressed, the animation switches between these modes (in order):
- All on
- Back/forth (see pictures)
- Light painting mode (just pointer finger LED on)
- All off
Attachments
Step 8: Light Paintings!
Light paintings are created when a camera's exposure time is great enough that light will continuously excite the sensors in the camera, drawing a picture. To do this, you'll need to set the camera's shutter speed to a big number (bigger delay). Also, if your camera has a "bulb" setting, this will allow you to keep your shutter open indefinitely, which is good for larger and more intricate paintings. What this setting does is keep the shutter open until you tell it to close.
The device that really helped out in this process was an external remote. I hooked up two wires coming from a small connector in the camera to a pushbutton. This allowed me to take pictures easily and precisely.
After a lot of use, you may need to recharge the battery, so this will be helpful!
Please vote for me if you enjoyed this Instructable!
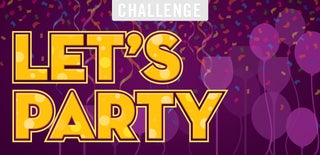
First Prize in the
Let's Party! Challenge
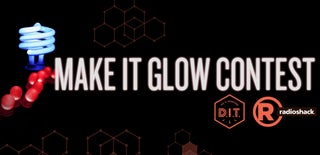
Participated in the
Make it Glow!