Introduction: Displaying Data on a Website - Electronic Pot [Arduino Nano + Ethernet Shield]
I always wanted a plant in my room, that would fill the space between unfinished projects and expensive, but unnecessary pieces of electronic parts. So I came up with the idea of an electronic pot – The Arduino E-Pot – that would measure the basic needs of the plant (humidity, temperature and the presence of light). I also wanted to display the results in a neat way on a website, so I could see everywhere I was if the plant is doing OK.
You can find the results on this website: janped.com/ghandipot
- The connected hardware (pic 1)
- Example results (pic 2)
- A simple schematic that shows how the whole thing works (pic 3)
I divided the whole process in three simple steps:
- Connecting the hardware
- Software for the Arduino (the .ino sketch)
- Software for the web-server (some .php files)
You can find a more detailed description of this project on janped.com
Step 1: Connect the Hardware
If you want to connect the Ethernet Shield with an Arduino Uno or Arduino Leonardo Board, you actually don’t need any wiring. The only thing you would have to connect with the set-up would be the sensors.
Parts:
- Arduino Nano Rev3 (Tested also on Leonardo and UNO)
- Ethernet Shield
- Photoresistor
- DHT11 Humidity Module
- 1x 4,7kΩ
- 1x 10kΩ
- 1x Ceramic capacitor 100nF
You can of course use other sensors with other requirements, that’s why I don’t include any specific models or data sheets. Always make sure you use components recommended by the producers of the sensors.
I based the connection between the Nano and Ethernet Shield on this post Arduino Nano with Ethernet Shield by ntewinkel.
Wiring:
yellow
- D13 on NANO – ~13 on Ethernet Shield
blue
- D12 on NANO – ~12 on Ethernet Shield
red
- D11 on NANO – ~11 on Ethernet Shield
black
- D10 on NANO – ~10 on Ethernet Shield
green
- D4 on NANO – ~4 on Ethernet Shield
cyan
- DATA on DHT11 – D2 on NANO
brown
- GND on DHT11 – GND on NANO
purple
- VCC on DHT11 – 5V on NANO
white
- 1 on Photoresistor – A0 on NANO
gray
- 2 on Photoresistor – 5V on Ethernet Shield
As you can see on the graphic above, there are also additional pull-up resistors and a capacitor. Connect the 4,7kΩ resistor between the GND and DATA of the DHT11. The 100nF capacitor between the VCC and GND of the DHT11. The last part – a 10kΩ resistor – should be placed as a pull-up resistor for the photoresistor from the data pin to GND (on Ethernet Shield in my case)
Step 2: Software for the Arduino
/* E-Pot v.1.0 ------------------------ Author: Jan Pedryc E-mail: jan.pedryc@gmail.com Date: 23.11.2015 For more information about this project check http://www.janped.com ------------------------ This is the code for the E-Pot project. The main purposes of this code are: 1. Establishing connection - ethernet shield 2. Configuration of the humidity module DHT11 3. Configuration of the photoresistor LOOP: (approximately once every hour) a) Gaining data from the sensors b) Preparing a string (data) for the _POST request c) Sending the data using the gateway to its destination */
#include <SPI.h> #include <Ethernet.h> #include <DHT.h>
// ---------------------------------------------- Web Server CONFIG // Enter a MAC address and IP address for your controller below. // The IP address will be dependent on your local network: byte mac[] = {0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED }; IPAddress ip(192,168,0,177); IPAddress gateway(192,168,0,1); IPAddress subnet(255,255,255,0);
EthernetClient client;
// ---------------------------------------------- Humidity Module CONFIG #define DHTPIN 2 // What pin we're connected to #define DHTTYPE DHT11 // DHT 11
DHT dht(DHTPIN, DHTTYPE);
// ----------------------------------------- Photoresistor CONFIG int lightPin = 0; // Variable stores the value from the photoresistor int PRvalue = 0; // Additional variable storing the value to compare int PRlight = 0; // Stands for the light presence (ON/OFF)
String data; // This string will contain the prepared data // which we will send as a POST request
void setup() { // Open serial communications and wait for port to open: Serial.begin(115200); /* For Arduino Leonardo users - uncomment this part: */ // while (!Serial) { // ; // } Serial.println("Serial connection established"); if (Ethernet.begin(mac) == 0) { Serial.println("Failed to configure Ethernet using DHCP"); } dht.begin(); Serial.println("DHT11 connection established"); data = ""; }
void loop() { delay(1000); // ------------------ Humidity Module ACTION Serial.println("Humidity Module ACTION"); // Wait a few seconds between measurements. int t = 0; // temperature int h = 0; // humidity // Reading temperature or humidity takes about 250 milliseconds! // Sensor readings may also be up to 2 seconds 'old' // (its a very slow sensor) h = dht.readHumidity(); delay(500); // Read temperature as Celsius (the default) t = dht.readTemperature(); delay(500);
// Check if any reads failed and exit early (to try again). if (isnan(h) || isnan(t)) { Serial.println("Failed to read from DHT sensor!"); return; } // ------------------ Photo Resistor ACTION PRvalue = analogRead(lightPin); // 500 is optional in this case. You should test the // photoresistor in your environment and decide how // sensitive the sensor should be if (PRvalue>500) { PRlight = 1; // 1 means in my case that there is enough light } else { PRlight = 0; // 0 means there is unefficient light } // The Serial.print & Serial.println commands are optional // (I used them for testing purposes) Serial.print("Temp: "); Serial.println(t); Serial.print("Hum: "); Serial.println(h); Serial.print("Light: "); Serial.println(PRlight); // Preparing the data from the sensors to send via the // ethernet shield data = "ahum1=" + String(h) + "&temp1=" + String(t) + "&light=" + String(PRlight); Serial.println(data); // For testing purposes // ------------------ Ethernet Shield ACTION if (client.connect("www.janped.com", 80)) { // Your domain Serial.println("Client connected."); client.println("POST /add.php HTTP/1.1"); client.println("Host: janped.com"); // Your domain client.println("Content-Type: application/x-www-form-urlencoded"); client.println("Connection: close"); client.println("User-Agent: Arduino/1.0"); client.print("Content-Length: "); client.println(data.length()); client.println(); client.println(data); } if (client.connected()) { client.stop(); } // Now wait approximately one hour // 1000ms * 60 = 60 000ms = 1 min // 60 000ms * 60 = 3 600 000ms = 1 h // The delay() function should know it's a long int, // that's why there is a 'L' included at the end delay(3600000L); }
I tried to comment everything where could occur any problems. If something isn't clear or the code doesn't work as it should, please contact me in any way.
You can find a more detailed description of the code on my website: janped.com
Attachments
Step 3: Software on Your Web Server
At this point you should log into your database and create the needed table. This step depends on the type of your database, in my case (MySQL) I just went to the ‘SQL’ tab in the control panel and created the table with this query:
CREATE TABLE `tempLog` (
`timeStamp` TIMESTAMP PRIMARY KEY DEFAULT CURRENT_TIMESTAMP,
`ahum1` INT(11) NOT NULL,
`temp1` INT(11) NOT NULL,
`light` INT(11) NOT NULL
)
Now the main part: the first two files are needed to handle the communication between:
- Arduino Ethernet Shield - Web server (add.php)
- Web server - Database (connect.php)
First, let's create the file for the database connection (connect.php):
<?php function Connection(){ $server="db_server"; // for example: my_sql.database.com $user="username"; $pass="password"; $db="db_name"; // for example: my_dbase
// The above information you should get from your hosting company $connection = mysql_connect($server, $user, $pass);
if (!$connection) { die('MySQL ERROR: ' . mysql_error()); } mysql_select_db($db) or die( 'MySQL ERROR: '. mysql_error() );
return $connection; } ?>
Second, the file "waiting" for the POST request (add.php):
<?php include("connect.php"); $link=Connection();
$ahum1=$_POST["ahum1"]; $temp1=$_POST["temp1"]; $light=$_POST["light"];
$query = "INSERT INTO `tempLog` (`ahum1`, `temp1`, `light`) VALUES ('".$ahum1."','".$temp1."','".$light."')"; mysql_query($query,$link); mysql_close($link);
header("Location: index.php"); ?>
After this steps, you should see data in your database in the table 'tempLog'.
The next steps are related only with web development. We create the main file which always open when we have a visitor on our server (index.php) and a second file that contains the code responsible for the menu at the top - header.php. The third file is optional - I just wanted to show some photos of my project that's why I included a gallery.php file.
These three files are included above (e-pot.rar). You need to make couple of changes (substitute the domain names with your domains), but it shouldn't cause any problems.
However if you get stuck at any point, just let me know and I will be happy to help you.
Good luck with your E-Pot :)
?>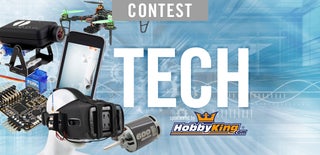
Participated in the
Tech Contest