Introduction: Distance Sensitive Alerting Machine / Price List
This instructable will teach you how to create a distance sensing machine that alerts you at different stages in terms of your distance from the sensor. These alerting tools include an RGB LED that illuminates the three traffic light colors as you get closer and closer, a seven segment display that displays your distance from the sensor, and a motor that is always running to let you know that the machine is on. This build also includes a normal red LED that is always on above the seven segment display to let you know that the power is on.
Links To Parts
Seven Segment Display: https://www.amazon.ca/Segment-Display-4pcs-0-5-0-7...
Red LED: https://www.amazon.ca/Gikfun-Assorted-Arduino-100p...
DC Motor: https://www.amazon.ca/RioRand%C2%AE-Mini-Torque-El...
Distance Sensor: https://www.amazon.ca/Aukru-Ultrasonic-Distance-Me...
General Wiring: https://www.amazon.ca/Elegoo-120pcs-Multicolored-B...
H-Bridge: https://www.amazon.ca/Adafruit-H-Bridge-Motor-Driv...
Decoder: https://www.amazon.ca/INTEGRATED-CIRCIUT-SEGMENT-D...
RGB LED: https://www.amazon.ca/Diode-SODIAL-Common-Anode-Gr...
Resistors: https://www.amazon.ca/uxcell%C2%AE-0-25W-Flameproo...
Arduino Board: https://www.amazon.ca/Elegoo-Board-ATmega2560-ATME...
Step 1: Adding the H-Bridge
The first step is to wire in your H-Bridge that will control your motor. I used the instructable down below to help me build this part. The picture above shows you what the finished result looks like. The pins that connect the H-Bridge to the arduino are the ones that are used in the reference instructable. The coding behind this H-Bridge came from the second link down below.
PS: Due to faulty equipment, my H-Bridge was unable to control my motor so I was led to control the motor with the use of just simple wiring to the power and ground rail.
Reference To H-Bridge Build: https://www.instructables.com/id/Introduction-90/
Reference To H-Bridge Code: https://www.instructables.com/id/Writing-the-Code-...
Step 2: Making the Seven Segment Display With Decoder
This step is the most difficult step because it requires you to wire the pins from the seven segment display to the decoder and from the decoder to the arduino board. I made this seven segment display with decoder from reference to the links below. This step requires you to follow the guides below and wire it properly. Once the wiring is done like in the pictures below, you are required to follow the truth table from the decoder link and use that table to determine what numbers will be displayed on the seven segment display.
Link To Decoder Reference: http://tlc.iith.ac.in/img/arduino/Team2/driving-se...
Link To Seven Segment Display Reference: https://docs.google.com/document/d/1m09N4D7WFq2k_M...
Step 3: Adding in the Distance Sensor
This distance sensor will determine the distance of any object from itself. I used the link below as reference when I built it and wrote the code. The VCC & GND pins are to be put with power and ground respectively, while the trig pin sends out the signal and the echo pin receives it which determines the distance that an object is from it. The trig and echo pin can be placed in any of the arduino digital pins. The reference that I used also contained the basic code to set up the distance sensor.
Link To The Distance Sensor Reference: https://www.tautvidas.com/blog/2012/08/distance-s...
Step 4: Adding the RGB Led
The RGB Led can be seen in the top left portion of this picture. This LED basically can illuminate a multitude of colors when you set each Red, Green, or Blue to different values. For my project's case, it will project the traffic light colors which include red, yellow, and green. I used the link below to help me build it and write the basic code which can be found on the website.
Link To RGB Led Reference: https://docs.google.com/document/d/10i2CXRDnHs8-nu...
Step 5: Update: Arduino Board
This picture is what the arduino board will look like once you utilize all your components.
Step 6: Creating the Code
For my machine, I compiled all the base codes of the different parts and modified the void loops so that the machine would serve my wishes. To start, I added the int's which define all the pins. This part of the code includes,
const int controlPin1 = 2;
const int controlPin2 = 7;
const int enablePin = 13;
const int trigPin = 8;
const int echoPin = 12;
const int A = 3;
const int B = 4;
const int C = 5;
const int D = 6;
const int redPin = 9;
const int greenPin = 10;
const int bluePin = 11;
After these int's, I added one quick line of code that corresponds to the distance sensor sketch which just tells the arduino the distance equation which includes,
long microsecondsToInches(long microseconds) {
return microseconds / 74 / 2;
}
Moving on, I wrote the setup using a combination of all the basic setups from the individual component's sketches. This part will include,
void setup() {
pinMode(A, OUTPUT);
pinMode(B, OUTPUT);
pinMode(C, OUTPUT);
pinMode(D, OUTPUT);
pinMode(controlPin1, OUTPUT);
pinMode(controlPin2, OUTPUT);
pinMode(enablePin, OUTPUT);
digitalWrite(enablePin, LOW);
pinMode(echoPin, INPUT);
pinMode(trigPin, OUTPUT);
pinMode(redPin, OUTPUT);
pinMode(greenPin, OUTPUT);
pinMode(bluePin, OUTPUT);
}
These setups declare each pin as output or input and if they are low or high in terms of receiving power.
Now, I made voids for each number that the seven segment will display. These voids will be used in the "if" statements later on in the code. This code includes,
void zero() {
digitalWrite(A, LOW);
digitalWrite(B, LOW);
digitalWrite(C, LOW);
digitalWrite(D, LOW);
}
void one() {
digitalWrite(A, HIGH);
digitalWrite(B, LOW);
digitalWrite(C, LOW);
digitalWrite(D, LOW);
}
void two() {
digitalWrite(A, LOW);
digitalWrite(B, HIGH);
digitalWrite(C, LOW);
digitalWrite(D, LOW);
}
void three() {
digitalWrite(A, HIGH);
digitalWrite(B, HIGH);
digitalWrite(C, LOW);
digitalWrite(D, LOW);
}
void four() {
digitalWrite(A, LOW);
digitalWrite(B, LOW);
digitalWrite(C, HIGH);
digitalWrite(D, LOW);
}
void five() {
digitalWrite(A, HIGH);
digitalWrite(B, LOW);
digitalWrite(C, HIGH);
digitalWrite(D, LOW);
}
void six() {
digitalWrite(A, LOW);
digitalWrite(B, HIGH);
digitalWrite(C, HIGH);
digitalWrite(D, LOW);
}
void seven() {
digitalWrite(A, HIGH);
digitalWrite(B, HIGH);
digitalWrite(C, HIGH);
digitalWrite(D, LOW);
}
void eight() {
digitalWrite(A, LOW);
digitalWrite(B, LOW);
digitalWrite(C, LOW);
digitalWrite(D, HIGH);
}
void nine() {
digitalWrite(A, HIGH);
digitalWrite(B, LOW);
digitalWrite(C, LOW);
digitalWrite(D, HIGH);
}
Now that we have these voids, we will now be able to put them really simply into our "if" lines of code so that the decoder will be able to light up the display easily.
Moving on, I added the base code for the RGB Led which includes,
void setColor(int red, int green, int blue) {
analogWrite(redPin, red);
analogWrite(greenPin, green);
analogWrite(bluePin, blue);
red = 255 - red;
green = 255 - green;
blue = 255 - blue;
The void setColor states that the pins can be called simply by their color names instead of saying Pin after all of them. The "255 - red" states that when a pin is LOW, it will have full power, so that means if we want a certain color to be illuminated, it must be set to 0 and the others to be set at 255, which means that this is a Common Anode Led.
Now, we will move onto the void loop which is where the main body of code is written. To start, I wrote the basic void loop for my distance sensor sketch. This includes,
void loop() {
long duration, inches;
digitalWrite(trigPin,LOW);
delayMicroseconds(2);
digitalWrite(trigPin,HIGH);
delayMicroseconds(10);
digitalWrite(trigPin,LOW);
duration = pulseIn(echoPin,HIGH); {
inches = microsecondsToInches(duration);
Serial.print(inches);
Serial.print("in, ");
Serial.println();
delay(100);
}
This code tells the trig pin to send out a signal and for the echo pin to receive it and determine the distance.
Now for the "if" statements, they will determine what each component will do at different distances that this machine reads.
if(inches < 1) {
zero();
setColor(100, 0, 0);
delay(1000);
}
else if(inches < 2) {
one();
setColor(0, 255, 255);
delay(1000);
}
else if(inches < 3) {
two();
setColor(0, 255, 255);
delay(1000);
}
else if(inches < 4) {
three();
setColor(0, 0, 255);
delay(1000);
}
else if(inches < 5) {
four();
setColor(0, 0, 255);
delay(1000);
}
else if(inches < 6) {
five();
setColor(0, 0, 255);
delay(1000);
}
else if(inches < 7) {
six();
setColor(0, 0, 255);
delay(1000);
}
else if(inches < 8) {
seven();
setColor(255, 0, 255);
delay(1000);
}
else if(inches < 9) {
eight();
setColor(255, 0, 255);
delay(1000);
}
else if(inches < 10) {
nine();
setColor(255, 0, 255);
delay(1000);
}
}
Basically, with all these "if" statements, they tell the seven segment display what number to display, and what color to display when a certain distance is reached.
This concludes my entire sketch for the Distance Sensing Alerting Machine!
Step 7: Conclusion
Thank you for reading my instructable and I hope that you have learned how to successfully make this machine.
If I were to improve this machine in any way, it would be to have a bigger arduino so that I would be able to add a button that would control the motor or even a better H-Bridge / Motor that would have worked with the code that I used to control it.
Thank you!
Made By: Tirth Patel
For: Mrs. Edwards
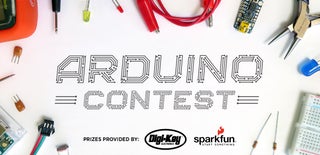
Participated in the
Arduino Contest 2017