Introduction: Customizable Desk Lamp
This instructable will guide you through the necessary steps of creating a smart desk lamp, which can connect to the internet, respond to touch, and can adjust its color and brightness. Don't feel that you have to follow the machining steps super rigidly as the lamp can ideally fit whatever form its designer intends. Feel free to change the look and implement the electronics and software here for your own customized desk lamp!
Step 1: Machine Base
The base consists of two, 3/8" sheets of aluminum, and a back wall with tapped holes to join them. Using a mill and strap clamps, fixture your aluminum pieces down at a 7 degree angle and mill the top and bottom sides parallel. Then, once you have done this to both pieces, flip them over and draw an approximate line at 17 degrees for the cut on the other side. Cut close to this line using a band saw, and then proceed to mill down to shape. When you have completed milling this last angle, get a 1/8" slotting tool and cut a slot at a depth of 1/8" below this last angle. Do this same step to the other side, but make sure to cut on the opposite side so that both slots face each other.
For the back wall, use a 3/4" x 2" x 1' bar of aluminum and mill both the top and bottom to a 7 degree angle. This will complement your trapezoidal walls. Drill and tap holes at the 1/4, 1/2, and 3/4 lengths along the center piece. Most hole sizes will do, but 6-32 brass screws will look nice on your final assembly. Drill and countersink these same locations on your trapezoidal pieces. On the top side, drill and tap two holes at the top of your center piece, which will mate with the overhanging piece of the lamp (See next step for images).
Lastly, you will need a place to connect your power cord. Jameco offers a nice DC power jack which will fit nicely in your aluminum wall. Use a counterbore to create a depressed region for the nut, and then use a smaller sized bore to make a through hole through the rest of the piece. See the image above for how these parts fit together.
Step 2: Machine Lamp Head
For the head of the lamp, use a 17.5" x 2" x 3/4" bar of aluminum. Using a 1/2" endmill, mill a slot along the piece to a depth of 1/4". This slot will house your LED strip and a piece of 1/8" acrylic which will diffuse the light of the LEDs. Next, drill two through holes and countersink to mate with your base from Step 2. Again, many sizes of screws will work, but 6-32 is recommended. Lastly, mill a 1-2 degree taper along the top edge.
At this point, you should be able to join all 4 of your machined pieces for a completed lamp body!
Step 3: Laser Cut Acrylic Base and Light Diffuser
This step involves laser cutting two pieces of acrylic for both the base and top of the lamp. For the base, use a 1/4" or 1/8" thick piece of acrylic and laser cut it into a rectangle to fit the bottom of your lamp. Make sure create 4, correctly sized through holes for your 4 brass screws. As shown above, these screws will attach into the 4 tapped holes on your base assembly.
For the light diffuser, laser cut a 1/8" piece of acrylic to the same length as your slot for the top piece. The width should be the width of your slot, 1/2". Leave a cutout region for your wires to come through.
Step 4: Building the Capacitive Touch Sensor
Attach a 1 megohm resistor between terminals 2 and 4 on your arduino uno. Attach one lead from the receive pin to your brass plate. Using the Capacitive Sensing Library, you can upload the following demo sketch to make sure that your circuit is functional. Later on, we will incorporate all our systems into one script for the entire lamp.
#include
/* * CapitiveSense Library Demo Sketch * Paul Badger 2008 * Uses a high value resistor e.g. 10 megohm between send pin and receive pin * Resistor effects sensitivity, experiment with values, 50 kilohm - 50 megohm. Larger resistor values yield larger sensor values. * Receive pin is the sensor pin - try different amounts of foil/metal on this pin * Best results are obtained if sensor foil and wire is covered with an insulator such as paper or plastic sheet */
CapacitiveSensor cs_4_2 = CapacitiveSensor(4,2); // 10 megohm resistor between pins 4 & 2, pin 2 is sensor pin, add wire, foil CapacitiveSensor cs_4_5 = CapacitiveSensor(4,5); // 10 megohm resistor between pins 4 & 6, pin 6 is sensor pin, add wire, foil CapacitiveSensor cs_4_8 = CapacitiveSensor(4,8); // 10 megohm resistor between pins 4 & 8, pin 8 is sensor pin, add wire, foil
void setup() {
cs_4_2.set_CS_AutocaL_Millis(0xFFFFFFFF); // turn off autocalibrate on channel 1 - just as an example Serial.begin(9600);
}
void loop() { long start = millis(); long total1 = cs_4_2.capacitiveSensor(30); long total2 = cs_4_5.capacitiveSensor(30); long total3 = cs_4_8.capacitiveSensor(30);
Serial.print(millis() - start); // check on performance in milliseconds Serial.print("\t"); // tab character for debug window spacing
Serial.print(total1); // print sensor output 1 Serial.print("\t"); Serial.print(total2); // print sensor output 2 Serial.print("\t"); Serial.println(total3); // print sensor output 3
delay(10); // arbitrary delay to limit data to serial port }
Step 5: Controlling Your LED's
Following the above schematic, connect 3, N-channel MOSFETs to your Arduino to control the current to the red, green, and blue channels of your LED strip. Connect the +12V of your LED strip to your 12V DC power supply. When purchasing a power supply, make sure it supplies enough current for your whole LED strip. 1 meter of the 60-LED strip from Adafruit will draw around 1 amp of current, so plan accordingly.
To test your setup, upload the following sample code to your arduino (from this Adafruit tutorial).
// color swirl! connect an RGB LED to the PWM pins as indicated
// in the #defines // public domain, enjoy! #define REDPIN 5 #define GREENPIN 6 #define BLUEPIN 3 #define FADESPEED 5 // make this higher to slow down void setup() { pinMode(REDPIN, OUTPUT); pinMode(GREENPIN, OUTPUT); pinMode(BLUEPIN, OUTPUT); } void loop() { int r, g, b; // fade from blue to violet for (r = 0; r < 256; r++) { analogWrite(REDPIN, r); delay(FADESPEED); } // fade from violet to red for (b = 255; b > 0; b--) { analogWrite(BLUEPIN, b); delay(FADESPEED); } // fade from red to yellow for (g = 0; g < 256; g++) { analogWrite(GREENPIN, g); delay(FADESPEED); } // fade from yellow to green for (r = 255; r > 0; r--) { analogWrite(REDPIN, r); delay(FADESPEED); } // fade from green to teal for (b = 0; b < 256; b++) { analogWrite(BLUEPIN, b); delay(FADESPEED); } // fade from teal to blue for (g = 255; g > 0; g--) { analogWrite(GREENPIN, g); delay(FADESPEED); } }
Step 6: Coding on Arduino or Electric Imp
Download the LampController.ino file attached at the bottom of this page. Adjust the variable definitions at the top of the file to the pins used on your arduino for each channel of your RGB LED strip. Additionally, make sure that the following line contains the correct pins for your capacitive sensor:
CapacitiveSensor cs_4_2 = CapacitiveSensor(2,4);
If you are finding that your light's brightness is permanently changing, you should increase the value of the variable named "threshold." If you are finding that your touch has no effect, make sure that the demo code from the "Building the Capacitive Touch Sensor" section is working. If that works, consider lowering your threshold value. This will take some work to get right, so be patient! If you find that your plate has too much noise, consider putting Kapton tape over the edges of your brass plate. This will insulate the plate electrically, and should give you good data for threshold calibration.
Attachments
Step 7: Optional: Connect to WIFI
If you are interested in connecting this circuit to WIFI, consider using an Electric Imp. Instead of attaching the color control leads to 3 PWM Arduino pins, you will connect them to 3 PWM pins on the Electric Imp. This way, you can control the colors independent of brightness. Using the Wunderground API for Electric Imp, you can get data such as sunsets and sunrises and sync your lamps colors to those events. For mine, I have chosen to change from a white light to a warmer color upon sunset. At sunrise, the colors will change back to their old values. This step makes your lamp incredibly customizable and I highly recommend taking the time to do the implementation! Happy lamp building!
Attachments
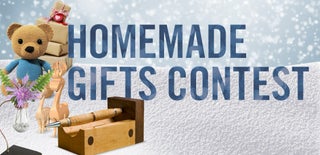
Participated in the
Homemade Gifts Contest
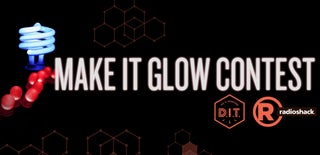
Participated in the
Make it Glow!
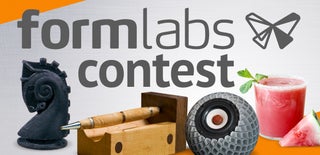
Participated in the
Formlabs Contest