Introduction: Make a Simple Operating System
Ever wanted to make an Operating System? Ever waned to know how command line operating systems work? Everything's here!
So we'll make a basic Operating System in C#, don't worry, if you even know some basics of c++ or java, you'll understand the code :D
By end of this tutorial, you'll be able to make your cool operating system!
So lets begin with some ind
Step 1: The Concept
So, how actually a OS works? I'll explain from the level of a electric circuit.
Most of you know logic gates in electric circuits. when many logic gates are connected, i.e. billions of transistors are connected together inside a chip, they can store 0s and 1s. Each gate could store either 0 or 1.
Then the connected transistor forms a Micro-Controller or a Micro-Processor. Here we are going to program on the micro-processor which is in your computer.
Did you know that your computer can also run with a operating system!? Wonder how! Every chip in your computer can run individually without a microprocessor but then without OS, it can't do multi tasking or run multiple threads together like in simple words, play a video in which one time music can play and one time graphics can play. But both can't run together.
Because of an operating system, today you can listen to music while browsing the internet.
The OS works based on its kernel. The kernel stores all the library files and whenever we run a program, the program's code calls header files from the kernel. In windows, kernel is 'Win32'
Step 2: Materials and Prerequisite Knowledge
What will you need-
1) Microsoft Visual Studio 2012/2013/2010 (Any Edition)
Link: https://www.visualstudio.com/
2) COSMOS User Development Kit Milestone 4 (C# Open Source Managed Operating System)
Link: https://cosmos.codeplex.com/downloads/get/90082
3) Any Virtulization Software to run the OS. I'm using VMware Player.
Perquisite Knowledge-
Basics of C++, Java, .net would work
I just request to just browse some 5-10 basic online tutorials of c# so you can get a better understanding
Step 3: Introduction to COSMOS
Cosmos (C# Open Source Managed Operating System) is an operating system development kit which uses Visual Studio as its development environment. Despite C# in the name, any .NET based language can be used including VB.NET, Fortran, Delphi Prism, IronPython, F# and more. Cosmos itself and the kernel routines are primarily written in C#, and thus the Cosmos name. Besides that, NOSMOS (.NET Open Source Managed Operating System) sounds stupid.
Cosmos is not an operating system in the traditional sense, but instead it is an "Operating System Kit", or as I like to say "Operating System Legos". Cosmos lets you create operating systems just as Visual Studio and C# normally let you create applications. Most users can write and boot their own operating system in just a few minutes, all using Visual Studio. Milestone 5 includes new features such as an integrated project type in Visual Studio, and an integrated debugger. You can debug your operating system directly from Visual Studio using breakpoints. Cosmos is available in two distributions, the developer kit (dev kit), and the user kit. The dev kit is designed for users who want to work on Cosmos itself. The user kit is designed for those who are interested in building their own operating system and doing some Cosmos work. The dev kit might be thought of as the Cosmos SDK. Most users should start off with the user kit as it is not so overwhelming like the dev kit. This article focuses on the user kit.
Step 4: Writing Your First Operating System
So, first lets write a OS which can simply print some text on screen!
So first of all, install all of the software required that is mentioned in the previous step.
1) Open visual studio and navigate to File>New Project
2) In the new project dialog box, select COSMOS and then COSMOS Project in the templates box. Name the project and click OK!
3) Now you'll see a file "program.cs" in the solution explorer. Click and load it.
4) You'll see a whole lot of code in it, don't be scared, we just need to edit code under "public static void Init()" block only :D
5) Now click on start button you see on visual studio toolbar or from Debug menu, click "start debugging"
6) You'll see a COSMOS Builder window appear, without any modification, just boot click build
7) WOW! So that QEMU window appear? QEMU is actually a operating system emulator so you are seeing the code being executed. Now lets make some modifications and personalize the OS.
Step 5: Personalizing the OS
Now just make some simple changes in code,
under public static void Init(),
Change Console.WriteLine("Welcome! You just booted C# code. Please edit Program.cs to fit your needs"); to Console.WriteLine("Hello World! This is my first operating system");
So, you've changed Welcome! You just booted C# code. Please edit Program.cs to fit your needs to Hello World! This is my first operating system.
We've just edited the text. Now lets compile the code and see the output. Perform the steps in previous step to compile the code.
Saw that! Text has changed! you can even put your name here. Congrats! you've won half the battle by understanding the basics. But how about a operating system that takes input from user and gives user a output? Just like command line operating systems or Linux terminal.
Step 6: Making a Command Line OS
So here we'll make a command line operating system. As we saw the previous code, the code just writes some text on screen via Console.WriteLine() function. Now we will write a OS where the user gives the input and the computer processes it.
So, lets make a code which prints Hello User! Thanks for using this operating system! when we give hello as input to the computer.
In programming, there are different kinds of loops which most of you know like If loop which does the action if the conditions are met. So here will will use if loop and if the input of a user is hello, then it will print the line we mentioned above.
CODE:
string input;
input = Console.ReadLine();
if (input == "hello")
{ Console.WriteLine("Hello User! Thanks for using this operating system!");
}
Here in this code, we made a string named input in which we read some data. The If loop checks that if the input matches the word hello, then it should print Hello User! Thanks for using this operating system!
Now lets go and execute this code!
You can now type into the operating system now! type hello
Excited? Saw the output? :D
You made your own command line OS. but how about adding many more features in this? Like if you type about, it tells you the info about the OS and if you type help it shows the list of commands? Lets do it in the next step.
Step 7: Adding More Features to Our OS
So, lets add more features like about which displays COSMOS OS 1.0 and help which displays
hello - Simple OS command
about - Know about OS
Similarly like the previous step, make 2 more if loops.
CODE:
string input;
input = Console.ReadLine();
if (input == "hello")
{ Console.WriteLine("Hello User! Thanks for using this operating system!"); }
if (input == "about")
{ Console.WriteLine("COSMOS OS 1.0 ");
}
if (input == "help")
{
Console.WriteLine("hello - Simple OS command");
Console.WriteLine("about - Know about OS ");
}
In this code as you see we've made 2 more if loops. Now execute the code and type about. Saw that, it displays COSMOS OS 1.0 and then close the window and re-execute the code and then type help. Saw that? This means everything is going good.
Now every OS has a option of shut down or power off. So lets add features like shutdown and restart.
Step 8: Adding Shutdown and Restart Features
Adding shutdown and restart feature is also extremely easy. In cosmos the code to shutdown is Cosmos.Sys.Deboot.ShutDown(); and to restart is Cosmos.Sys.Deboot.Reboot();
Now make IF loops which have input as shutdown and restart.
CODE:
string input;
input = Console.ReadLine();
if (input == "hello") { Console.WriteLine("Hello User! Thanks for using this operating system!"); }
if (input == "about") { Console.WriteLine("COSMOS OS 1.0 ");
}
if (input == "help") { Console.WriteLine("hello - Simple OS command"); Console.WriteLine("about - Know about OS "); }
if (input == "shutdown") { Cosmos.Sys.Deboot.ShutDown(); }
if (input == "restart") { Cosmos.Sys.Deboot.Reboot(); }
Now execute the code and type restart. Saw it xD! It restarted! Now type shutdown. Saw it, the window closed which means the OS shut down.
This may be the most exciting part when you made a OS which can shutdown and restart itself!
Now lets improve this OS by giving it final touches.
Step 9: Final Touches
In the final touches, we will use the switch case because it is much more simpler and better then IF statement. We will also make a command that when the user types a keyword which is not in list of our commands, it should show No such command
Just see the code once.
CODE:
while (true)
{
string command = Console.ReadLine();
switch (command) {
case "shutdown": { Cosmos.Sys.Deboot.ShutDown();
break;
}
case "help":
{
Console.WriteLine("hello - Simple OS command");
Console.WriteLine("about - Know about OS ");
break;
}
case "about":
{
Console.WriteLine("COSMOS OS 1.0 ");
break;
}
case "reboot": {
Cosmos.Sys.Deboot.Reboot();
break;
}
case "hello": {
Console.WriteLine("Hello User! Thanks for using this operating system!");
break;
}
default: { Console.WriteLine("No such command");
command = null; break; } }
}
Ok, just copy the code into visual studio and execute the code. Note that we added the break;. This is used in switch case. Now have the fun with your OS!
Now try to type a wrong command like help me. this command was not defined so it shows No command found. This means now our OS won't accept wrong commands. And try all commands. You may have noted that now you can type another command after previous as we are taking input in a constant loop.
Step 10: Running & Testing the OS
So i'm now going to test the OS we made. I'll be using a Virtualization software to run the OS. I'll be using VMware player which is free to download!
Ok, so create a virtual machine and open C:\Users\ {Your Username} \AppData\Roaming\Cosmos User Kit
Here you will find cosmos.iso file after you select build ISO in visual studio. Just boot this file in VMware and enjoy your operating system! (make it full screen)
Step 11: Thanks & About Me
I'm a 16 year old embedded developer, working with MIT Media Labs India Initiative. I work in various technologies like ARM, AVR, Arduino and Raspberry Pi.
You can find about me here: http://www.sarthaksethi.net/
Facebook: https://www.facebook.com/pages/Sarthak-Sethi/25272...
Youtube: https://www.youtube.com/channel/UCv7OszKtRFkJ_e0Af...
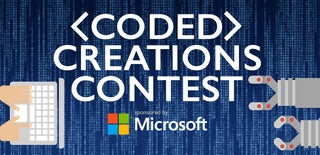
Participated in the
Coded Creations
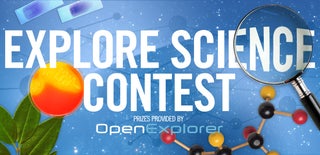
Participated in the
Explore Science Contest