Introduction: Message in a Chip
If you had just 1024 bits of data to write a message of importance, how would you do it? That could be 128 ascii-coded characters (about a Tweets worth), or a bit map of 32 x 32 pixels.
Why would I ask such a question? I was working with a label printer and the cartridge contained a small surface mount chip on a PCB. On closer inspection, it turns out to be a fairly common 1-Kilobit EEPROM (erasable memory). The next obvious question is, "can I read and write it with an Arduino?" The answer to that is, "YES!", of course. Which leads us back to the first question. What could you do with a kilobit?
In 1974, astronomers brocast a 1.6 kilobit message from the Arecibo radio antenna. The message consisted of a 73 x 23 bitmap (prime numbers) containing coded information about humans, our basic structure, and that of our solar system. Being that the stars it was pointed at were 25,000 light years away, I doubt we'll get a reply any time soon, but it does lead to some interesting thinking about how to code information so it can be retrieved by someone with no fore knowledge of the structure or contents.
The real title of this Instructable should be "How to Read and Write to a DS2431 EEPROM with Arudino", but how boring is that? The interesting part of the DS2431 is that it uses a communication system called 1-wire which requires only a ground and a signal.
Enough pre-amble. Let's get down to bits.
Step 1: One Wire?
The PCB has three pads on the bottom, with the one in the middle marked "S", which we can assume means signal, and the ones on the edges marked "G", which by process of elimination we can assume are ground. Despite the chip having six legs, looking at the data sheet show that only two are used, one for ground and the other signal, or "IO".
The only real hitch for the Arduinoist is that the signal line requires a "strong" pull-up signal, which rules out using the internal ones. We need to wire something in the 4.7K - 8.2K ohm range. I'm using a 6.8K ohm resistor. See the diagram if you are unsure how pull-ups work. They basically ensure the signal line is at 5 volts unless a signal pulls it low.
On the PCB I designed, I decided to add the resistor to make it easy to interface to. All you need is a three pin male header. You can set the Arduino pins to be ground or supply by setting the pinmode to OUTPUT, and then setting them either HIGH (for source) or LOW (for ground).
On to some code!
Step 2: Code
I started with some code posted in the Arduino Forum by FredBlias. Even with a working code example, I still had to struggle a bit to understand what was going on under the hood. The important thing to understand is that the chip reads or writes 8 bytes at a time to a temporary "scratchpad" memory location.
You will need to download and install the OneWire library from the Arduino Playground. There is also some interesting details about the protocol mostly applying to the Dallas temperature sensors.
I've modified the code so you can either read/write 128 characters (bytes in ASCII code), or bits in a 32x32 matrix (an arbitrary layout). By default, the code attempts to talk to the chip, and if communications are established, read the memory.
ADDR= 2D 4E A1 94 B 0 0 61 <br>Reading from EEPROM as chars 0 M71 1 � 2 �;;� � 3 � � 4 � � 5 � 6 � 7 8 9 �� d< 10 M P n 11 p p � � 12 //<< 13 14 �'� 15
This looks like scrambled bits, except the "M71" matches the printer model. I assume the rest is information about expiration date and label type.
Now, lets make it our own.
Attachments
Step 3: Write
If you do not have the wires right, you will get a "No device found". That never happens to me, but try again if you see it.
Here is my attempt at 32x32 bit art. I actually started in Gimp and then wrote a Python script to parse me out some one and zeros.
No device found.<br>No device found. No device found. ADDR= 2D 4E A1 94 B 0 0 61 Writing bits to EEPROM Reading from EEPROM as bits 0 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 2 1 1 1 1 3 1 1 1 1 4 1 1 1 1 5 1 1 6 1 1 7 1 1 8 1 1 9 1 1 10 1 1 11 1 1 12 1 1 13 1 1 14 1 1 15 1 1 1 1 16 1 1 17 1 1 18 1 1 1 1 19 1 1 1 1 20 1 1 1 1 21 1 1 1 1 22 1 1 1 1 23 1 1 1 1 24 1 1 25 1 1 1 1 26 1 1 1 1 27 1 1 28 1 1 29 1 1 30 1 1 31 1 1
Or maybe you are the poetic type . . .
ADDR= 2D 4E A1 94 B 0 0 61 <br>Writing chars to EEPROM Reading from EEPROM as chars 0 Roses 1 are red, 2 violets 3 are blue 4 bits are 5 awesome, 6 and 7 bytes 8 are 9 too! 10 11 12 13 14 15
Step 4: E-Jewels?
At recent workshop I helped with for ChickTech.org, we had them create jewelry out of scrap electronic parts. It was pretty fun, and the girls were very creative. Now that we've got a chip with some meaningful data stored on it, time to make it into something wearable.
I drilled a small hole in the top and put a 6mm ring on it. I also rounded and smoothed the edges a bit. Got to love Dremels! After that, it was earrings or a necklace. None of my favorite ladies have pieced ears, so necklaces it will be.
On the PCB I designed, I created a heart silkscreen pattern for the front, and laid out a large gold pad on the back.
I have a few of these on hand if you have someone who would appreciate this type of geekiness in your life. Head over to Tindie.
Step 5: A "bit" More . . .
I sat down with my granddaughter and helped her code a message for her best friend. Realizing that it was not as easy as it should be (it never is, is it?), I wrote some scripting to help, and so here it is . . .
- Use a piece of graph paper or a 32x32 pixel image for the design. I use free, Open Source, GIMP, and if you set the scale to 200, the transparency background squares match the pixel sizes, making it easy to draw or design something.
- It needs to be coded to "1"s and "0"s, and doing 1024 by hand is a bit tedious. Free, Open Source Python to the rescue! The attached script will convert the image to text that can be cut-and-pasted into the Arduino script.
- And of course, you may not expect the recipient to have the skills to extract the message, so maybe a printout is proof enough!
Attachments
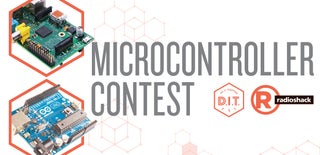
Participated in the
Microcontroller Contest
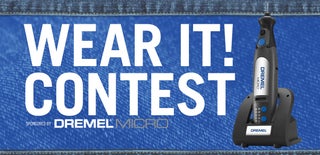
Participated in the
Wear It! Contest
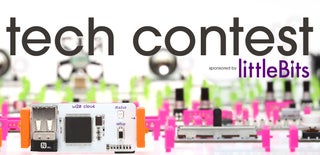
Participated in the
Tech Contest