Introduction: Raspberry Pi Baby Monitor With Splunk
These steps will teach you how to assemble the components required to make a Raspberry Pi baby monitor, and collect that data into Splunk, a log reader and analytical tool, to preset that data in a meaningful way. At the end you will be able to use the Spunk dashboard to monitor sleep patterns, room temperature and humidity. We'll also pull in outside weather for a comparison.
To create the monitor you will need:
- Some Linux skills
- Raspberry Pi 2b with Raspbian installed
- The Raspberry Pi Camera module, with Infrared
- AM2302 DHT22 Temperature And Humidity
- The Raspberry Pi Wifi Dongle
- A server to host Splunk - for the Reporting dashboard, I used Linux Centos, but you can run it on Windows for Mac as well
- The Splunk Universal forwarder software for Raspberry Pi
When I started this experiment, I was sure that if we could maintain a good sleep environment for bub's, then I too would get more sleep in return, benefitting everyone. It gets pretty hot in Australia in summer, so ensuring the room was at optimal temperate for the sleep zone should really help keep everyone happy. So with some monitoring and alerting we should be able to keep it in the sweet spot for optimal baby comfort!
Step 1: Set Up the Raspberry Pi
The basis for the baby monitor is the Raspberry Pi, a cheap versatile Linux platform. You can order them from heaps of places online for about $50. I used the Model 2B, but I'm sure the newer version would also work. The Raspberry Pi 3 has integrated Wifi, so that's one less thing you need to buy.
- Order Raspberry Pi, and camera, and SD card
- Wait patiently for its delivery
- Install Raspbian linux on the SD card, follow the Raspberry Pi guide on this
- Confirm you can connect, use you favourite SSH program to log in remotely once installed
- Run an update, running the update command
sudo apt-get update
Once that is done, you've got a base operating system to build on
Step 2: Connect the Pi NoIR Camera
The Pi NoIR, is the night vision version of the camera, it has 'No Infra Red' Filter, meaning, if you have an infra red light source, at night, the camera can see them in the dark. Since our baby will do lots of sleep at night (hopefully), thats what we're using. If you want brightly lit, colour vision, don't use this camera.
Open the Raspberry Pi Case, push back the bar on the clip next to HDMI port, push it firmly, but don't bend it
Logged into the Raspberry Pi (ssh, or the console)
Type the command
sudo raspi-config
Select
Enable camera
Select enter, when you are done, It prompts for a reboot, which you should do, its wise to follow instructions
Step 3: Connect the Temperature and Humidity Sensor
At the start I advised on the sensor to use for this. The one I linked to has the embedded components, namely a resistor. I have seen another version, a blue version that doesn't have this, and may need one, to not blow up. This direct connection is applied to the red AM2302 sensor, with on board components. Others may work, but be careful.
- Connect the jumper lead to the 3 connections on the Sensor
- Orange = Ground
- Red = VCC
- Brown = DAT
Connect the other end to the Raspberry Pi. Raspberry Pi have a reference digram for connecting.
- Connect the ORANGE = Pin 6 = third from the left on the top
- Connect the RED = Pin 1 = bottom left
- Connect the Brown = Pin 22 = 8 from the boat left
Now you can close the case and connect the power up, we need to write some software to read the sensor, you know, check that it actually works.
Step 4: Install Required Libraries
To write the software, that can read the sensor, we're going to need a couple of things.
First ensure the compiler is installed already with the common
sudo apt-get install git-core
Then install the WiringPi Module with the command
git clone git://git.drogon.net/wiringPi
Then type
cd wiringPi git pull origin
Then compile and install
cd wiringPi ./build
Step 5: Read Data From the Sensor
I used this site as a guide for writing the software that will be able to read the Sensor. They advised we need to use a C program to ensure it always reads the sensor. Ive modified their original code to not loop, and format the readings better for later reading into Splunk. Splunk likes the format variable_name=value to easily identify it in the log
Open your your favourite linux text editor and make a file dat.c
Insert this code into it the dht.c file
<p>/*<br> * dht.c: * read temperature and humidity from DHT11 or DHT22 sensor */ #include #include #include #include #define MAX_TIMINGS 85 #define DHT_PIN 3 /* GPIO-22 */ int data[5] = { 0, 0, 0, 0, 0 }; void read_dht_data() { uint8_t laststate = HIGH; uint8_t counter = 0; uint8_t j = 0, i; data[0] = data[1] = data[2] = data[3] = data[4] = 0; /* pull pin down for 18 milliseconds */ pinMode( DHT_PIN, OUTPUT ); digitalWrite( DHT_PIN, LOW ); delay( 18 ); /* prepare to read the pin */ pinMode( DHT_PIN, INPUT ); /* detect change and read data */ for ( i = 0; i < MAX_TIMINGS; i++ ) { counter = 0; while ( digitalRead( DHT_PIN ) == laststate ) { counter++; delayMicroseconds( 1 ); if ( counter == 255 ) { break; } } laststate = digitalRead( DHT_PIN ); if ( counter == 255 ) break; /* ignore first 3 transitions */ if ( (i >= 4) && (i % 2 == 0) ) { /* shove each bit into the storage bytes */ data[j / 8] <<= 1; if ( counter > 16 ) data[j / 8] |= 1; j++; } } /* * check we read 40 bits (8bit x 5 ) + verify checksum in the last byte * print it out if data is good */ if ( (j >= 40) && (data[4] == ( (data[0] + data[1] + data[2] + data[3]) & 0xFF) ) ) { float h = (float)((data[0] << 8) + data[1]) / 10; if ( h > 100 ) { h = data[0]; // for DHT11 } float c = (float)(((data[2] & 0x7F) << 8) + data[3]) / 10; if ( c > 125 ) { c = data[2]; // for DHT11 } if ( data[2] & 0x80 ) { c = -c; } float f = c * 1.8f + 32; printf( "Humidity=%.1f Temperature=%.1f\n", h, c ); }else { printf( "Data not good, skip\n" ); } } int main( void ) { if ( wiringPiSetup() == -1 ) exit( 1 ); read_dht_data(); return(0); }</p>
Step 6: Compile Your Reader Software
The code you put into the dot.c file now needs to be compiled
Type the command
cc -Wall dht.c -o dht -lwiringPi
You can then run the program with the command
sudo ./dat
You should see some output, this will only work if sensor is connected correctly, and code is working. It will show
Humidity=66.9 Temperature=18.3
Obviously exchange the reading for your local temperature - I've removed the Fahrenheit reading, since we don't use that down under.
Step 7: Write the Data to a Log File
When we get to using Splunk, it reads log files. So the data we are collecting from the sensors we are going to write to a log file, to then tell Splunk to monitor for changes.
First we are going to create a directory in the pi home folder, called logs, to store the files in
mkdir /home/pi/logs
Now, still in the home directory create a bash script file
touch /home/pi/temperature_script.sh
Edit the file with your favourite text editor, vim or something, and insert this code
#! /bin/bash log="/home/pi/logs/" #run the client "/home/pi/dht" > temperature.txt OUTPUT=`cat temperature.txt` # Write values to the screen TEMPERATURE=`echo "$OUTPUT"` # Output data to a log file echo "$(date +"%Y-%m-%d %T" ): ""$TEMPERATURE" >>"$log"temperature.log
Step 8: Schedule Your Scripts
We are going to use Cron on the Raspberry Pi to run the job. It gives us the flexibility of when to run each of our scripts
The temperature sensor required sudo (root) access to run, so we have to sudo the crontab, type the command
sudo crontab -e
Add the line to the file
*/5 * * * * /home/pi/temperature_script.sh
This will run the temperature program every five minutes. You can check you log file is being written to with the command
cat /home/pi/logs/temperature.log
Your log should start looking like the one attached.
Step 9: Set Up the Webcam
Now we are going to set up the webcam. We enabled it earlier but now we're going to install some software to actually use it (crazy right!).
The software is called motion, and it can run a web server, for viewing, detect motion, and record frames, and pictures. In this setup, I turn that off, I don't have a lot of space for video files, so I just run the live feed for monitoring, and use the log for tracking sleep movements.
The default install of motion doesn't have support for the Pi Camera. So we are using a special build made to work with it. The full guide is here. Ill give the short version.
Type the command
sudo apt-get install motion
Now download the special build
wget href="https://www.dropbox.com/s/0gzxtkxhvwgfocs/motion-mmal.tar.gz
Now unzip
tar zxvf motion-mmal.tar.gz
Open the config file motion-mmalcam.conf with your favourite text editor, and update a few of the settings, daemon on so it runs in the background, increase video height and video for quality, set the log file to our other location, and increase log level for events.
daemon on width=1280 height=720 logfile /home/pi/logs/motion.log log_level 7
Turn off stream local host, it mean's you can connect to it remotely.
stream_localhost off
Text_Left is the text that comes up on the feed
text_left Baby's Room %t
There is a setting for username and password, set that up if you ahem this internet facing. Otherwise leave it as is.
Save the file, and start motion with the command
sudo ./motion -c motion-mmalcam.conf
Step 10: Connect to the Web Stream
You should now be able to connect to the web stream from the camera
Point your web browser, preferably Chrome or Firefox at
And you should get a live feed. You can also check the motion log and see if events are getting recorded.
cat /home/pi/logs/motion.log
These motion events are what we are going to count to detect sleep movements.
If you want to turn the red LED off, make the camera more discreet, edit the boot config
sudo vim /boot/config.txt
Add these lines, and after reboot it won't came on.
# Turn off camera Red LED disable_camera_led=1
Step 11: Install Splunk and the Universal Forwarder
I'm not going to get into much details on the Splunk server. You can install on whatever OS you want. I've done it on Mac OSX and Linux, and both worked fine. So just follow the Splunk guide.
Once you have Splunk installed, ensure its got at least one receiving connection. The default port is 9997. Once this is done, and assuming no firewalls will block it, you can configure a Universal Forwarder to send data in.
Go to Splunk and get the universal forwarder for Linux. You need to get the version for ARM
Follow the instructions at Splunk.
Run the Splunk installer when you have the file
tar xvzf splunkforwarder-<…>-Linux-arm.tgz -C /opt
Configure the forwarder to point to your Splunk server.
Edit the file at
sudo vim opt/splunkforwarder/etc/system/local/outputs.conf
My server is 192.168.0.10
[tcpout:default-autolb-group] server = 192.168.0.10:9997
Save and close, you can now start splunk forwarder
/splunkforwarder/bin/splunk start
No add a motor to our log file location
sudo /opt/splunkforwarder/bin/splunk add monitor /home/pi/logs
Our Splunk forwarder should now start collecting logs in that directory. You can check the Splunk forwarder log if its not going though to check for issues
sudo cat /opt/splunkforwarder/var/log/splunk/splunkd.log
Step 12: Create a Dashboard
Now log into your Splunk server and confirm if data is coming in.
Perform a search for events
index=main source="/home/pi/logs/temperature.log"
If you switch to Verbose mode in Splunk, it should identify the Temperature and Humidity variables. You can now graph them using a Splunk search, like;
index=main source="/home/pi/logs/temperature.log"| timechart max(Temperature)
You can create a dial for Temperature, run the below search and save to a new Dashboard, give it a name "Baby Monitor"
index=main Temperature source="/home/pi/logs/temperature.log" | stats first(Temperature)
And again for Humidity, select visualisation, Radial gauge. Save as a Dashboard panel, to the existing Panel, "Baby Monitor"
index=main Humidity source="/home/pi/logs/temperature.log" | stats first(Humidity)
Step 13: Add More to the Dashboard
If the motion log is successfully being logged we should have events for it in Splunk now. If we search for events detected, we should get results back
index=main detected
If we have results we can graph them. We are going to graph the volume of events. This will show us how much movement was detected over time.
The query looks like this
index=main detected | timechart count span=5min
By combining these searches and adding to a dashboard we can build up a lot of useful metrics on inside and outside temperatures, and baby movements.
The query for inside and outside temperature together looks like this:
index=main source="/home/pi/logs/outside_weather.log" OR source="/home/pi/logs/temperature.log" | timechart max(Temperature) AS "Baby's Room" max(Outside_Weather) AS "Outside" span=30min
Step 14: Connect With the App and Mounting
Splunk App
Splunk make an App for IOS, its free to install, and yo can enter in the password and web address and search your dashboards on your Tablet.
If you want to connect remotely, you can use a port forward on your home router to open up the address. It required ports 8000 and 8089 for Splunk, to the SPlunk server, and 8081 for the webcam, straight to the Raspberry Pi as that's where its hosted.
Mounting
I mounted using sticky tape over the back of the crib, the Pi is pretty light. Whilst this is OK for now, as soon as your baby is able to sit up, this becomes very dangerous, you need to then mount it out of reach!
They will tear it off and try and eat it!
Step 15: Bonus Script - Outside Weather
I used a script to get the Weather from the Australian weather site. Its downloads a file from the Bureau of Meteorology and outputs the location closest to me, and writes that to a log file for Splunk to ingest.
#! /bin/bash<br>log="/home/pi/logs/" #run the client<br>rm IDW60XXX.94610.axf wget href="http://www.bom.gov.au/fwo/IDW60901/IDW60XXX.94610.axf" OUTPUT=`cat IDW60XXX.94610.axf | grep -m 1 0,94610,` TEMPERATURE=`echo "$OUTPUT"` echo "$(date +"%Y-%m-%d %T") , ""$TEMPERATURE" >>"$log"outside_weather.log
Add that to a cron job
*/30 * * * * /home/pi/outside_weather.sh
Then you can start adding more data, cross referencing and building bigger dashboards, the possibilities are endless!
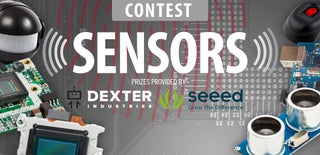
Participated in the
Sensors Contest 2016
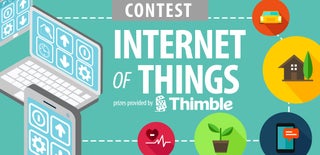
Participated in the
Internet of Things Contest 2016
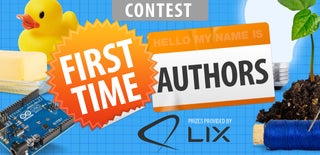
Participated in the
First Time Author Contest 2016