Introduction: Step-By-Step LCD Wiring (4 Bit Mode) and Programming Examples for Arduino
This instructable will guide a user step-by-step in wiring and programming a Hitachi HD44780 (or a compatible) chipset LCD screen to an Arduino, using the LiquidCrystal Library. Programming examples are provided for all the Library calls.
Parts List:
1 - Arduino (I am using an UNO R3 here for the demonstration)
2 - Solderless Breadboard
3 - An Hitachi HD44780 or compatible LCD panel
4 - Current limiting resistor for the backlight (10 Ohm)
5 - 10K trim pot for the contrast
6 - 16 pin Male 0.01 Headers
Step 1: Pin Configurations May Vary
The Hitachi HD44780 chipset or compatible LCD's generally have a very standard pin set. Those without backlights may have only 14 pins, omitting the final two pins powering the light. Please refer to the datasheet for your unit to make sure they match up.
The pinout is as follows:
1. Ground
2. VCC (+3.3 to +5V)
3. Contrast adjustment (VO)
4. Register Select (RS). RS=0: Command, RS=1: Data
5. Read/Write (R/W). R/W=0: Write, R/W=1: Read
6. Clock (Enable). Falling edge triggered
7. Bit 0 (Not used in 4-bit operation)
8. Bit 1 (Not used in 4-bit operation)
9. Bit 2 (Not used in 4-bit operation)
10.Bit 3 (Not used in 4-bit operation)
11.Bit 4
12.Bit 5
13.Bit 6
14.Bit 7
15.Backlight Anode (+)
16.Backlight Cathode (-)
Step 2: Get the Backlight Working
Solder the headers onto the LCD panel and insert the LCD Panel into a solderless breadboard.
Wire the Breadboard with 5V and GND from the Arduino
Add jumpers from 5V to pin 15 on the LCD, and insert the 10 Ohm Resistor into Pin 16 on the LCD. Jumper the other end of the resistor to GND.
Power up the Arduino and the backlight should light up. Progress!
Step 3: Add Power and Display Contrast
1 - Wire GND to pin 1 and 5 (Pin 5 jumper not shown in photo)
2 - Wire 5V to Pin 2
3 - Wire one of the outer legs of the 10K trim pot to 5V and the other to GND (Both wires Yellow in Photo)
4 - Wire the middle leg of the 10K trim pot to pin 3 (Brown in photo)
Apply power to the unit and adjust the trim pot until you see rectangles appear on the screen. (See Photo)
Step 4: Control Wires and Data Wires
Add the control connections
1 - Jumper pin 4 on the LCD to Digital IO 6 on the Arduino
2 - Jumper pin 6 on the LCD to Digital IO 7 on the Arduino
Add the data connections
1 - Jumper pin 11 on the LCD to Digital IO 9 on the Arduino
2 - Jumper pin 12 on the LCD to Digital IO 10 on the Arduino
3 - Jumper pin 13 on the LCD to Digital IO 11 on the Arduino
4 - Jumper pin 14 on the LCD to Digital IO 12 on the Arduino
Before anything shows up on the LCD, you will need to load the Library and sketch provided in the next 2 steps onto the Arduino.
Step 5: Download the LiquidCrystal Library
The LiquidCrystal Library is a core library for Arduino - there should be no need to install it. If you need to install it for some reason, visit the Arduino site.
http://arduino.cc/en/Reference/Libraries
Step 6: Uploading the "Hello, World!" Sketch
Create a new sketch window enter the information below. If you display is not a 16 character by 2 line display, please modify
#define LCDColumns 16
#define LCDRows 2
to match your LCD display unit.
Upload the sketch to your Arduino to get the display to read "Hello, World!"
If your display does not function, check the wiring carefully.
#include <LiquidCrystal.h>
#define RSPin 6
#define EnablePin 7
#define DS4 9
#define DS5 10
#define DS6 11
#define DS7 12
#define LCDColumns 16
#define LCDRows 2
LiquidCrystal lcd(RSPin, EnablePin,DS4,DS5,DS6,DS7);
void setup()
{
lcd.begin(LCDColumns, LCDRows); //Configure the LCD
lcd.setCursor(0,0);
lcd.print("Hello, World!");
}
void loop()
{
}
Attachments
Step 7: Understanding "Hello, World!" Using the LiquidCrystal Library
LiquidCrystal(rs, enable, d4, d5, d6, d7) - Creates a variable of type LiquidCrystal. You are asked to define the Ardunio pins the LCD is connected to.
In the example we used define statements to set the pin values and then called LiquidCrystal using the define'd values
#define RSPin 6
#define EnablePin 7
#define DS4 9
#define DS5 10
#define DS6 11
#define DS7 12
#define LCDColumns 16
#define LCDRows 2
LiquidCrystal lcd(RSPin, EnablePin,DS4,DS5,DS6,DS7);
begin(cols, rows) - Specifies the dimensions (width in coolumns and height in rows) of the display. In this example, I am using a 16 Character by 2 line display. We used define statements to set these values and called begin() in the setup() routine.
#define LCDColumns 16
#define LCDRows 2
...
lcd.begin(LCDColumns, LCDRows);
setCursor(col, row) - Positions the cursor. setCursor(0,0) to the top left of the display
print(data) - Prints Text to the LCD, lcd.print("Hello, World!") prints"Hello, World!" on the screen, starting at the cursor position
Step 8: Advanced LiquidCrystal
Load the sketch provided below. It extends the "Hello, World!" example by including all of the LiquidCrystal command and allowing you to uncomment various sections to explore the functionality of thelibrary. Most of the commands available are there for you to play with!
Attachments
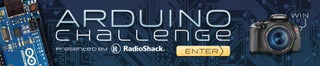
Participated in the
Arduino Challenge