Introduction: Touchscreen Door Lock
hi im still pretty new to arduino and instructables this is my first instructable so any suggestions will be appreciated.
i was a electrician in the army and since i got out i opened a online game store with my uncle and i do all the repair work to consoles. i got my first arduino a few months ago and have been playing around with simple projects when i got the idea to use one of the touchscreens from a broken nintendo ds in a project i read up on the wiring and some basic code to get started and this is what i ende up with hope yall enjyoy.
Attachments
Step 1: Parts Needed
1. soldering iron
2. 30awg wire/the hard way or / ds breakout board can be found at sparkfun for $4.00 https://www.sparkfun.com/products/9170
3.if not using the breakout board a magnifying glass will be helpful but not needed
4. a power supply to power arduino i had a 9v playstation 1 slim power supply i was able to use but anything 5v-12v should work fine with arduino
5. touchscreen/digitizer they can be found on ebay for about $4.00 if bought in the us or you could go with a china seller for around $1.50 but shipping normally takes 2 weeks or more for oversees shipments.
6. servo motor/ maglock or other door locking mechanism
Step 2: Wiring Up Your Digitizer
if you have the breakout board this part will be fairly easy if not you will have to solder the 30awg wire to each of the pins coming off the digitizer.
if soldering to the digitizer i highly suggest using a flux paste i use a tacky flux in a syringe for easy placement but any flux should work fine. if you have trouble getting the tab to sit flat while soldering use a piece of double sided sticky tape to hold it down.
if you are using the breakout board go ahead and attach the touch screen/digitizer to the breakout board and solder 4 wires to the pins.
Step 3: Hooking Up Your Arduino
your going to add your wires to the arduino as follows.
refer to picture for pinout if not using breakout board.
from touch screen y1 = arduino input A0
from touch screen x2 = arduino input A1
from touch screen y2 = arduino input A2
from touch screen x1 = arduino input A3
your servor motor will be attached to pin 9 on the arduino with power and ground being hooked up to 5v and ground on the arduino
if you want to add a led it can be added to pin 13
Step 4: Testing Your Touch Screen
this is were you hook your arduino up to your computer and start writing your sketch.
heres a example of my sketch.
int y1 = A0;
int x2 = A1;
int y2 = A2;
int x1 = A3;
void setup(){
Serial.begin(9600);
}
int readX(){
pinMode(y1, INPUT);
pinMode(x2, OUTPUT);
pinMode(y2, INPUT);
pinMode(x1, OUTPUT);
digitalWrite(x2, LOW);
digitalWrite(x1, HIGH);
delay(5);
return analogRead(y1);
}
int readY(){
pinMode(y1, OUTPUT);
pinMode(x2, INPUT);
pinMode(y2, OUTPUT);
pinMode(x1, INPUT);
digitalWrite(y1, LOW);
digitalWrite(y2, HIGH);
delay(5);
return analogRead(x2);
}
void loop()
{
int x = readX();
int y = readY();
if(x < 1000 & y < 1000){
Serial.print("x: ");
Serial.print(x-0);
Serial.print(" - y: ");
Serial.println(y- 0); }
delay(100);
}
Step 5: Finding Your X,y Coordinates
to find your x,y coordinates you may want to draw a simple sketch as shown to right down what your readings are.
now that your sketch has been uploaded open your serial monitor to serial baud 9600.
pay attention to your monitor as you touch the screen you should see your x,y coordinates showing up.
if you have constant numbers showing up it should be towards the outside range my screen showed a constant 850x and 900y coordinates this made things hard to read if you have this problem you can go back into your code and find this section.
void loop()
{ int x = readX();
int y = readY();
if(x < 1000 & y < 1000){ CHANGE THIS PART OF THE CODE TO A NUMBER LOWER THEN BEING SHOWN IN YOUR SERIAL MONITOR I WENT WITH 800 FOR MINE AND AFTER THE CHANGE IT SHOULD LOOK LIKE THIS.
if(x < 800 & y < 800)
now your serial monitor should be empty until the touch screen/ digitizer is touched this should make mapping your coordinates a lot easier.
now that you can find your coordinates you can create two simple buttons on the screen to lock and unlock your door
Step 6: Adding Buttons to Touch Screen
im a big halo fan so i printed out a cool halo picture to fit the size of the touch screen you can leave this blank or print whatever you like. in the future i plan on adding more buttons and a code so i will probably use a different printout soon.
because im still learning how to code arduino i kept it pretty simple i wanted it to lock when i pressed the 4 on the picture and to unlock when i touched mastercheifs helmet. to do this i had to map out the coordinates for where those spots were. check picture to get idea for coordinates.
to get your coordinates for buttons you need to find your min and max x,y coordinates for that section you want to be a button then write a greater then less than statement to include those coordinates wich should look like this.
button 1
if(y>0 && x>520 && x<1000 && y<300){
button2
if(y>500 && x>20 && x<300 && y<1000){
to do a simple test i added my led to the project here and had one button turn a led on and the other turn it off and touched around the rest of the screen to make sure my buttons were where i wanted them to be.
Step 7: Putting It All Together
pretty much everything i used i had laying around it wouldnt be hard to make this look a lot nicer or even add a maglock or something similar.
i just used some pvc a metal pipe and some scrap metal lined everything up then attached the servo motor i used a cheap $5 project box from radioshack that i had laying round to house it all in.
now for the final code feel free to use mine though you will probably have to tweak your servo motor to get it aligned with your locking mechanism.
#include
Servo myservo; // create servo object to control a servo
int pos = 0;
int y1 = A0;
int x2 = A1;
int y2 = A2;
int x1 = A3;
int ledPin = 13;
void setup() {
Serial.begin(9600);
}
int readX(){
pinMode(y1, INPUT);
pinMode(x2, OUTPUT);
pinMode(y2, INPUT);
pinMode(x1, OUTPUT);
pinMode (ledPin, OUTPUT);
myservo.attach(9);
digitalWrite(x2, LOW);
digitalWrite(x1, HIGH);
delay(5);
return analogRead(y1);
}
int readY(){
pinMode(y1, OUTPUT);
pinMode(x2, INPUT);
pinMode(y2, OUTPUT);
pinMode(x1, INPUT);
digitalWrite(y1, LOW);
digitalWrite(y2, HIGH);
return analogRead(x2);
}
void loop()
{
int x = readX();
int y = readY();
if(y>0 && x>520 && x<1000 && y<300){
Serial.print("x: ");
Serial.print(x-0);
Serial.print(" - y: ");
Serial.println(y- 0);
digitalWrite(ledPin, LOW);
myservo.write(154);
}else
if(y>500 && x>20 && x<300 && y<1000){
Serial.print("x: ");
Serial.print(x-0);
Serial.print(" - y: ");
Serial.println(y- 0);
digitalWrite(ledPin, HIGH);
myservo.write(60);
}
}
Attachments
Step 8: Final Thoughts
thank you for checking out my instructable this is my first instructable i hope everyone enjoyed all constructive criticism is welcome.
this is a fun little project to get playing with touch screens and mapping buttons while adding outputs if you dont have the need to build a door lock please give it a try and have some fun you can easily set the buttons for diffrent outputs just for fun controlling a servo motor or adding leds. ill give a update once i map more buttons and create a lock code if anyone is interested in more then a simple two button lock unlock system.
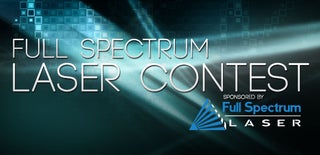
Participated in the
Full Spectrum Laser Contest
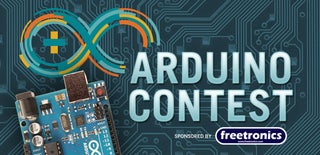
Participated in the
Arduino Contest