Introduction: GPS Car Tracker With Intel Edison
Going to the next project using the Intel Edison Transportation and Safety Kit I created a very basic application to track a car using the Grove GPS. This project took me a little more work because of the complexity of handling GPS data and the need to have the GPS connected with as many sattelites possible. I'll describe each challenge in this project in the next steps.
Step 1: Materials
• Intel Edison for Arduino
• Grove Base Shield
• Grove GPS
Step 2: Wiring
Grove GPS uses Serial communication to send data to the Intel Edison. In case of the Grove GPS I have (which uses SIM28 GPS Module), the Baud Rate can be from 9600 up to 115200, which brings fast communication so it is possible to get updated data instantly. The Grove GPS must be connected in the UART port of the Grove Base Shield.
Step 3: Challenges
• Power Consumption
Since Grove GPS needs high current power source, I realized that a USB Cable in Intel Edison as a power source is not enough, specially when you have other sensors in it (just plug Alcohol sensor and GPS and you'll see). Because of it, I used an external power source from the power plug I received to have enough power. If you want to use
• Handling GPS Data
GPS uses a specific format called NMEA(National Marine Electronics Association), which consists in some sentences which each of them describes one specific information that GPS captures (like the location and altitude data, Satellite data, etc.). One note: I found this information in the Seedstudio wiki (http://www.seeedstudio.com/wiki/Grove_-_GPS) that describes this info about the sensor. Also, this format is not used by Google Maps to show location so I needed to look for a way to convert this location data and I found an article written by Thomas Tingsted Mathiesen (http://www.tma.dk/gps/) that describes a simple way to convert (thank you so much Thomas!).
Step 4: Source
Below the simple code I wrote that does the following:
- Capture GPS info
- Look for the $GPGGA record (more information about NMEA in http://gpsinformation.org/dale/nmea.htm
- Collect Latitude and Longitude / Convert from NMEA to Dec
- Generate a Google Maps link with the GPS info
Since Google Maps API requires a key, I created one but I ommited mine in the code. Make sure to create an API key for you by following https://developers.google.com/maps/documentation/static-maps/
/*jslint node:true, vars:true, bitwise:true, unparam:true */ /*jshint unused:true */ // Leave the above lines for propper jshinting var GPSSensor = require('jsupm_ublox6'); var myGPSSensor = new GPSSensor.Ublox6(0); setTimeout(getGPSInfo(), 1000); if (!myGPSSensor.setupTty(GPSSensor.int_B9600)) { console.log("Failed to setup tty port parameters"); process.exit(0); } bufferLength = 256; var nmeaBuffer = new GPSSensor.charArray(bufferLength); function getGPSInfo() { if (myGPSSensor.dataAvailable()) { var rv = myGPSSensor.readData(nmeaBuffer, bufferLength); var GPSData, dataCharCode, isNewLine, lastNewLine; var numlines= 0; if (rv > 0) { GPSData = ""; // read only the number of characters // specified by myGPSSensor.readData for (var x = 0; x < rv; x++) { var data = nmeaBuffer.getitem(x); GPSData += data; } console.log(processData(GPSData)); } if (rv < 0) { // some sort of read error occured console.log("Port read error."); process.exit(0); } } } function processData(rawData) { var data = rawData.split(","); var index = data.indexOf("$GPGGA"); var lat = data[index+2]; var lon = data[index+4]; var latDec = convertNmeaToDec(lat); var lonDec = convertNmeaToDec(lon); if(data[index+3] == "S") latDec = latDec * -1; if(data[index+4] == "W") lonDec = lonDec * -1; var url = "https://maps.googleapis.com/maps/api/staticmap?center="; url += -latDec; url += ","; url += -lonDec; url += "&zoom=14&size=600x300&key=*** API KEY OMMITED! PLACE YOU OWN KEY HERE ***"; return url; }</p><p>// Function to convert the NMEA format from GPS to Decimal // This function is needed to show in Google Maps. // Thanks to http://www.tma.dk/gps/ function convertNmeaToDec(nmea) { var deg = (nmea / 100); var decPos = deg + ((nmea - (deg * 100)) / 60); return decPos; } // Print message when exiting process.on('SIGINT', function() { console.log("Exiting..."); process.exit(0); });
Step 5: Conclusion
The code is pretty simple but there are lots of information required to use the GPS data and how to convert to known formats (like the one that google Maps use). I hope that this instructable might help everyone use Intel Edison and Location data.
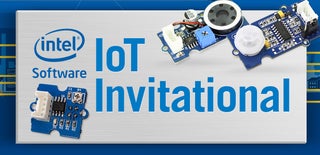
Participated in the
Intel® IoT Invitational