Introduction: Relay Control Using Alcohol Sensor
Thanks to instructables, I received an Intel kit for Transportation and Safety. I'm enjoying a lot and the kit is awesome. I'll try to create a series of instructables with the evolution of my project and I'm starting with the system I'm planning to install in my car: a device to read alcohol consumption and only allow the driver to turn on the car if the alcohol consumption is not high.
Step 1: Materials
- Intel Edison for Arduino
- Grove Base Shield
- Grove Alcohol Sensor
- Grove Touch Sensor
- Grove Smart Relay
- Grove LCD RGB Backlight
Step 2: Wiring Everything
The Grove Alcohol Sensor uses Analog pins in Grove Base Shield, I'm using pin A0. Touch Sensor and Smart Relay use digital pins and I plugged them in ping D3 and D2 respectively. At last, the LCD RGB Backlight is plugged in the I2C pin. The image above shows in details the pins I used.
Step 3: Coding
Intel has developed a very good set of hardware, software and tools to code IoT projects. The Intel XDK IoT Edition is an IDE created to make IoT projects with Intel Edison easily, and using Node.js. Also, Intel published two libraries for their boards called MRAA and UPM. MRAA is intended to be the low-level API for Hardware communication with its sensors. UPM is the high-level API and it is based on most Grove sensors.
The code is very simple and it does the following:
- Wait 2 minutes to heat the Alcohol sensor (The Seeed Studio Wiki page says the best preheat time for the sensor is 48 hours!)
- Run runAlcoholSensor() function every second where:
- Listens for Touch sensor to be on
- If someone is touching the sensor, it starts collecting 100 samples of the Alcohol sensor
- Then the code takes the average value of the samples
- If the value is between 200 and 500 (I'm still working to understand why did I do that), then it turns on the Relay.
The code is listed below:
<p>/*jslint node:true, vars:true, bitwise:true, unparam:true */<br>/*jshint unused:true */ // Leave the above lines for propper jshinting</p><p>// Load sensor modules var mq303a = require('jsupm_mq303a'); var lcd = require('jsupm_i2clcd'); var sensorModule = require('jsupm_ttp223'); var groveSensor = require('jsupm_grove');</p><p>// Instantiate an mq303a sensor on analog pin A0 // This device uses a heater powered from an analog I/O pin. // If using A0 as the data pin, then you need to use A1, as the heater // pin (if using a grove mq303a). For A1, we can use the D15 gpio, // setup as an output, and drive it low to power the heater. var myAlcoholObj = new mq303a.MQ303A(0, 15); var display = new lcd.Jhd1313m1(0, 0x3E, 0x62); var touch = new sensorModule.TTP223(3); var relay = new groveSensor.GroveRelay(2);</p><p>console.log("Enabling heater and waiting 2 minutes for warmup.");</p><p>// give time updates every 30 seconds until 2 minutes have passed // for the alcohol sensor to warm up statusMessage(1); statusMessage(2); statusMessage(3);</p><p>function statusMessage(amt) { setTimeout(function() { console.log((amt * 30) + " seconds have passed"); }, 30000 * amt); }</p><p>// run the alcohol sensor in 2 minutes setTimeout(runAlcoholSensor, 120000);</p><p>function runAlcoholSensor() { var notice = "This sensor may need to warm until the value drops below about 450." console.log(notice);</p><p> // Print the detected alcohol value every second setInterval(function() { if(touch.isPressed()) { var val = 0; for(i=0;i<100;i++) { val += myAlcoholObj.value(); } val = val / 100; var sensor_volt = val / 1024 * 5.0; var RS_air = sensor_volt / (5.0 - sensor_volt); var msg = "Alcohol: "; console.log(msg + val) + "sensor_volt = " + sensor_volt; display.setCursor(0,0); display.write(msg + val); if(val > 200 && val < 500) { relay.on(); } } else { relay.off(); } }, 1000); }</p><p>// Print message when exiting process.on('SIGINT', function() { console.log("Exiting..."); process.exit(0); });</p>
Step 4: Conclusion
Intel did a good thing with Edison, Grove and XDK IoT Edition making it as easy as programming Arduino boards. The good thing is that you can use other languages like C/C++, Python, Java and others to code Edison. Also, Edison is more than a microcontroller, is a complete mini PC with lots of good features in it, like embedded WiFi, Bluetooth, external storage(the OS is in the internal storage) and others.
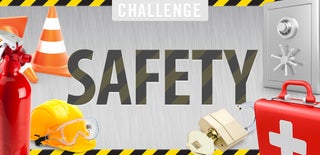
Participated in the
Safety Challenge