Introduction: Hello Circuit Playground!
I teach a robotics class in high school, and when learning arduino some our greatest struggles are learning how to connect components on the breadboard while also learning our first coding. Both of these are valuable skills, but the Circuit Playground option allows students to focus on coding first, then once they get some of that under their belts they can start extending their projects with circuit components external to microprocessor.
So what is a circuit playground you ask?
Its an arduino compatible board with build in neopixels (three colored LEDs, see my other instructable for an introduction to coding these really cool lights!), a bunch of sensors, some buttons and a switch. Here is a complete list:
Step 1: What Is on the Board?
There are quite a few components included on this board, here is a list and description of each:
- the 8 ports around the outside that are capacitative touch inputs that are also alligator clip friendly, 4 of these pins can do analog input and PWM (pulse width modulation) output
- 10 mini NeoPixels
- a triple axis accelerometer with tap detection so it can be used to detect free fall
- a temperature sensor
- a light sensor
- a microphone to detect sound
- a mini speaker
- 2 buttons
- a side switch
- green LED to indicate the board is powered on
- a red LED that is connected to pin 13 that can used easily to indicate the status of the program
- a reset button
Step 2: Adding a Library to Your Arduino Software
Before using the Circuit Playground you need to add a library to your software. The more recent versions of Arduino make this very easy.
Launch the Arduino application.
Open the Sketch menu.
Scroll down to the "Include Library" choice and mouse over to see if the Circuit Playground library is included in the list. If it is you can select that choice to have the #include statement added automatically to the file you have open.
If it is not included in the list, go all the way to the top of the "Sketch" menu and choose "Manage Libraries." From that screen you can find the Adafruit Circuit Playground choice and install the library.
Step 3: So How Do I Program It With the Arduino Language?
There is a library written for the Circuit Playground to simplify use of its many features. Here is a glossary of sorts for the commands you can use with it. The library download includes many example programs which vary from very advanced to easy to understand. It is always a good idea to connect the board and run one of the examples first just to make sure your hardware and the connection are set up correctly before jumping in and writing your own program.
To use the library in your program, this needs to be one of the first lines of code. The only thing that can occur before it is an include statement for another library. Some punctuation cannot be typed into the editor in instructables so I took mini snapshots of how to write the properly formatted code. Libraries are added with a #include statement.
Adafruit_CircuitPlayground.h
Wire.h
SPI.h
You will also want to include a library that allows the circuit playground to use its accelerometer as a mouse pointer.
Mouse.h
During the setup subroutine, you need to let the program know that you will be using a Circuit Playground. Here is the command to indicate this:
CircuitPlayground.begin();
Now its time to do stuff with the board! The format of the commands is very intuitive for young or inexperienced people just starting to learn.
To turn on the little red LED (not one of the neopixels, just the red LED near the USB:
CircuitPlayground.redLED(HIGH); or change HIGH to LOW to turn it off.
CircuitPlayground.readCap(3); to read the value of the capacitive touch sensor, 3 represents
the third capacitive touch sensor, there are 14
CircuitPlayground.slideSwitch(); returns the value of the slide switch, to the right is true and to the
left is false
CircuitPlayground.leftButton(); reads whether or not the left button is pressed
Circuit Playground.rightButton(); reads whether or not the right button is pressed
To control the ring of neopixels around the rim of the board, you use these commands:
CircuitPlayground.clearPixels(); turns off all colors of all of the neopixels, there are no arguments inside the parentheses for this command.
CircuitPlayground.setPixelColor(1,255,200,75); determines what color the pixel will display, the first number indicates which neopixel will be this color, the next three indicate the relative brightness of the red, green, and blue pixels. The range for these values is 0 - 255.
CircuitPlayground.setBrightness(150); this line sets the brightness of the pixel from 0 which is not lit to 255 which is the brightest setting, this applies to all neopixels that are currently being lit
CircuitPlayground.colorWheel(100); this line has one argument that selects a color from the range of colors
To read from the sensors included on the board, use these commands. Each sensor returns an analog value that was read from the sensor.
CircuitPlayground.lightSensor(); returns the amount of light detected by the light sensor
CircuitPlayground.soundSensor(); returns the sound level detected by the microphone
CircuitPlayground.playTone(523,2000,TRUE); there are three arguments for this command, the first is the frequency of the tone that will be played, the second is the duration of the tone, and the third has two choices true or false. If true is used then the code will pause for the amount of time you specified for the tone before moving to the next command. If you choose false, the code will move along immediately to the next line of code while playing the tone. The default option for this command is to pause while the tone is played.
CircuitPlayground.motionX(); these three commands return the value of the acceleration in each direction in m/s^2
CircuitPlayground.motionY();
CircuitPlayground.motionZ();
CircuitPlayground.setAccelRange(); this commands sets a range for the values of acceleration
CircuitPlayground.setAccelTap(2,3); this sets the threshold for what will be detected as a tap, the first argument is 0 for off, 1 for a single tap, and 2 for a double tap, the second argument is for the threshold of what is considered a tap
CircuitPlayground.getAccelTap(); returns a value of 0 -2 for no tap, single tap, or double tap
CircuitPlayground.temperature(); returns the temperature in Celcius
CircuitPlayground.temperatureF(); returns the temperature in Fahrenheit
CircuitPlayground.senseColor(red,green,blue); if you include the three parameters inside the parentheses this command will use the light sensor and the closest neopixel to return a value of red, green, and blue amounts of light hitting the light sensor.
Step 4: So What Else Can I Do With This Thing?
I like a nice project box before I begin working, and several people have created easy to print boxes on a 3D printer. Here are a few links to some examples...
- There is the wearable watchband, I printed mine in purple ninja flex filament. The clasp works well and it fits nice and snug. http://www.thingiverse.com/thing:1682293
- Or if you prefer wearing it as a pin, there is the Star Trek combadge style case. http://www.thingiverse.com/thing:1733517 I found this one while finishing this instructable, I'll be printing this one soon!
- The yo-yo case is unique. http://www.thingiverse.com/thing:1766385
- I like the basic shell case. I put the shell on the back of the processor so that I can access all of the capacitative touch sensors, buttons, etc. on the front. Both styles are an easy print. I used teal PLA filament. http://www.thingiverse.com/thing:1676423
- There is another case with a battery compartment behind the circuit playground. http://www.thingiverse.com/thing:1700966
Well, there are a wealth of options for things to do with your Circuit Playground! Here are just a few...
- make it into a game controller using the accelerometer to read the tilt, a 3d printed case or sewn case with a mix of regular fabric and conductive fabric would add some panache
- Design a watch band and use the ring of neopixels as a visual clock with lights advancing in different colors for the hour hand, minute hand, and second hand. You could even add a tone for announcing the passing of an hour or for alarms that you preset.
- Create a wrist band that you can use to monitor your pulse using the light sensor and the neopixel adjacent to it.
- Make a wall clock that uses advancing neopixels in three different colors to show the change in hours, minutes, and seconds.
- Make a visual accelerometer to help students learn about motion variables in physics class.
- Make a wireless accelerometer so it can be mounted onto an experiment so students can study the acceleration throughout a specific motion like dropping a ball onto a soft pillow.
- Make a visual display of sound waves by changing the onboard neopixels or adding lines of neopixels that show the magnitude of the amplitude or frequency like a bar graph.
- Explore what happens when you mix the primary colors of light.
If you have other ideas share them in the comments! Happy making!
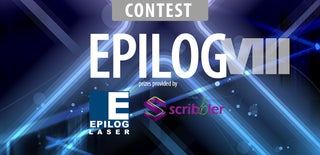
Participated in the
Epilog Contest 8
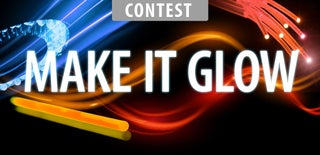
Participated in the
Make it Glow Contest 2016
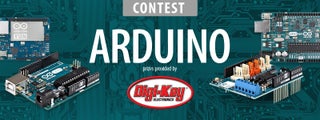
Participated in the
Arduino Contest 2016