Introduction: Play / Practice Wordle on ESP32
Have you ever seen this matrix of letters, with green - yellow - grey cells? It is getting viral, with tons of tweets about it...
The game is called WORDLE, you can play it online here. It's an online word game, with a new puzzle every day. You have 6 chances to find a five-letter word, in English. After your guess, the color of each letter indicates whether it is well placed (green), it exists but in another spot (yellow) or it is not in the word (grey). You can see the rules up there.
Wordle is super popular: over 300,000 people play it daily, according to The New York Times.
This game can become quite addictive, but you only have one game each day... Everyone is playing the exact same puzzle, so you can share your score (on Twitter for example) with your friends and discuss online about it.
Wordle was created by Josh Wardle, an engineer living in Brooklyn. You can find lots of tips and tricks on how to improve your score, but the best way to win is to practice. This is why I created a version on an ESP32.
Supplies
All you need is:
- One ESP32, possibly TT-GO T-display for a more graphical version.
- The Arduino IDE
Step 1: Prepare the Game
First you need to find a dictionary, a list of 5-letter words. It is required both to choose the target word, and to verify that your guesses are correct.
I found a few of them online, in English and in French:
- English
- https://www-cs-faculty.stanford.edu/~knuth/sgb-words.txt (5757 words)
- https://www.bestwordlist.com/5letterwords.htm (12478 words)
- French:
- https://fr.wikwik.org/mots5lettres.htm (23595 words)
- https://www.listesdemots.net/mots5lettres.htm (7980 words)
The average vocabulary of native-english speakers is between 40,000 and 50,000 words. But interestingly, there exists an active and passive vocabulary. An active vocabulary consists of the words we have learned and actually use when speaking or writing. On the other hand, a passive vocabulary refers to words we’ve assimilated but do not really use. The average active vocabulary of an adult English speaker is around 20,000 words, while his passive vocabulary is around 40,000 words.
So, if you consider the first list of 5757 words, knowing that they are all 5-letter words, there is a great chance that most of the standard 5-letter words of the average active vocabulary exist in this list. But if you want a tougher game, you can use the longer list...
If you want to create similar lists in another language, you just need to find them online and create a txt file with all the words separated by a space. I uploaded 3 files below: English (long and short) and French (short).
If you want a more complicated game, with more than 5 letters (or less), first create the associated dictionary (here is a list of 4-letter words, and here for 6). Then edit the ino file and change this line:
#define NUMBER 5 // Number of letters (don't change unless you can change the dictionary)
You may also want more than 6 trials: just change this line (near the end of the ino file):
if (++trials == NUMBER + 1) {
For example, for 10 trials:
if (++trials == 10) {
To play the game, I assume you already know how to use an ESP32 with the Arduino IDE, how to compile a C++ code and upload it on the microcontroller. If not, please refer to this page, for example.
In your Arduino folder, create a new folder called 'Wordle'. In this folder, create another one called 'data'. Put the file Wordle.ino in the Wordle folder, and the txt files in the data folder.
This will look like this:
- Wordle
- Wordle.ino
- data
- Dict-5757.txt
- Dict-12478.txt
- Dict-FR-7980.txt
Of course, you can only use the dictionary files you want. But they are quite light and do not really impair the memory of the ESP32. To upload the txt files on your ESP32, you must use the ESP32 Sketch Data Uploader. Everything is explained here. Remember that you must close the serial monitor to upload the files.
You only need to upload them once.
By default, the game assumes that you use the Dict-5757 file (English words). If you want to change this, edit the ino file and change this line:
const char wordFile[] = "/Dict-5757.txt";
Each time you guess a word, the program searches it in the dictionary file. With this file, it takes up to 450ms to verify that the word is in the dictionary. If you want to use the longer file (Dict-12478.txt), you just need to change the name in the line above, but it may take up to 2 seconds to verify your entry.
If you don't want to wait, or if you want to try random words or words that don't exist, just change this line:
#define CHECK true // set false if you don't want to check your input is in the dict
Change 'true' for 'false'. The program will not check your guess in the dictionary.
Run the program. After a reminder of the rules, you can enter your first guess. All character that are not letters are ignored. If you enter more than 5 letters, only the first five are accepted. If you enter less than 5 letters, the program waits until you have entered all 5 letters.
Then the result is displayed on the monitor: below your word are a series of signs. A '+' means that the letter above is well placed. A '=' means that it is present in the word but not at this place. And a '-' means that the letter is not in the word.
If you input a '?' (without quotes), the program gives you the information you have so far: the list of well placed letters and the letters you tried but that are not in the word.
Here is an example:
Please enter a 5 letter word. ABOUT =---- BLIPS ----= THERE -+-+- Here is what you know so far... Well placed: -H-R- Not in the Wordle: BEILOPTU CHARS -+++= SHARE ++++- SHARK +++++ Congratulations, you found it in 6 trials!!
The word was SHARK. You can see that my first guess was ABOUT and only the 'A' is present, but not at the first place. Later, the word THERE shows that the H and the R are well placed (second and fourth positions).
? summarizes where H and R are placed, and shows that B E I L O P T U are not present in the word.
SHARE was a good guess, which led me to SHARK, in 6 trials.
Step 2: Graphical Version
I also wrote a graphical version of the game, which mimics the original interface on the TT-GO T-display. To use it, you need the files below. Create in your Arduino folder a new folder called 'Wordle_TTGO', and do the same as explained above (data folder, dictionary files, upload, etc) with the additional 'Free_Fonts.h' file (which goes in the 'Wordle_TTGO' folder).
You also need to install, if not already done, the TFT_eSPI library, using the library manager of the Arduino IDE. Then you have to do the following:
Select the driver for the TT-GO: in the file C:\Users\xxxxxx\Documents\Arduino\libraries\TFT_eSPI\User_Setup_Select.h :
- comment the line #include <User_Setup.h>
- uncomment the line #include <User_Setups/Setup25_TTGO_T_Display.h>
The rest is similar to the previous step.
Here is another game, that goes with the photos:
Please enter a 5 letter word. ABOUT ---=- THERE ----- LIVES -=--+ Here is what you know so far... Well placed: ----S Not in the Wordle: ABEHLORTV QUITS +++-+ AZERT This word is not in my dictionary, try again QUIPS +++++ Congratulations, you found it in 5 trials!!
Enjoy!
Attachments
Step 3: How to Win?
If you want to win, read my other instructables "Win at Wordle"
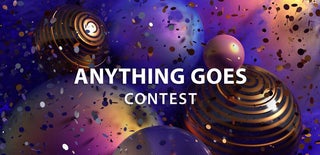
Participated in the
Anything Goes Contest 2021