Introduction: Secret Compartment Chess Set
I play chess pretty poorly and have always wanted to get better, but I don't even own a chess set. I decided I wanted to make my own set, but with a twist. I have made a chess board with a secret drawer that is locked unless the pieces are arranged on the board in a specific pattern.
The way it works is there are magnetic switches underneath the chosen squares on the chess set and magnets glued to the bottom of every chess piece. When the chess pieces with the magnets are placed on the squares with the magnetic switches, the switches open. The arduino is programmed to move a servo with a lever attached inside of the box when the switches are open. When this lever is lifted, the spring-loaded drawer is no longer held in place and pops out.
To lock the drawer again, press the pushbutton on the side while holding the drawer in. A buzzer will go off whenever the servo lever is being opened or closed.
Step 1: What You Need
(x1) Arduino uno (RadioShack #276-128)
(x1) Standard Servo (RadioShack #273-766)
(x6) Switch-Magnetic Reed Flange (RadioShack #55050593)
(x1) Universal 1000mA AC Adapter (RadioShack #273-316)
(x1) M-type Adaptaplug (RadioShack #273-344)
(x1) Grid-Style PC board (RadioShack #276-149)
(x1) SPST Normally Open Pushbutton (RadioShack #275-644)
(x1) 75dB Piezo Electric Buzzer (RadioShack #273-793)
(x32) Round Ceramic Magnet (RadioShack #64-1883)
(x7) 10kOhm Resistor (RadioShack #271-1335)
(x3) Male header pins
(x2) 36"x24"x1/4" plywood
(x1) 1.5"x1.5"x1/8" plywood
(x1) 2"x2"x1/2" plywood
(x1) Chess Piece Set
Step 2: Cut Wood
Use a laser cutter to cut the outer box pattern on the 1/4" plywood.
Use a laser cutter to cut the drawer pattern on the other sheet of 1/4" plywood.
Step 3: Glue Outer Box
Glue together the pieces of the outer box.
Step 4: Glue Drawer
Glue together the pieces of the drawer.
Save the 1/2" squares cut from the same sheet. You will later use these to mount the servo.
Step 5: Pushbutton
Remove the pushbutton's fastening nut.
Solder a red wire to one lug of the button and a green wire wire to the other lug.
Insert the pushbutton into the hole closest to the corner of the box and fasten it in place with its mounting nut. Note: you will need to fasten the mounting nut on backwards because the wood is too thick to screw it on the correct way.
Step 6: Prep Servo
Insert the three male header pins into the three sockets on the servo.
Use the smallest round servo horn for this project. Servo horns are the white gear-like objects that come with the servo.
Detach the servo horn from your servo.
Step 7: Magnetic Switches
Discard the side of your magnetic reed switch that does not have a wire (this part is simply a magnet that can be used to activate the switch).
Decide what secret combination you would like to open your chess set. Measure how far from the edges of the board the squares of your secret combination are and draw the locations of the squares onto the back of your board using a pencil.
Peel off the layer of paper on the bottom of each magnetic switch and expose the adhesive. Stick a magnetic reed switch onto the bottom of your chess board in the middle of each of the six squares you have drawn.
Step 8: Solder
Solder the pins of the servo to the Grid-Style PC board. Solder wires to these three pins that will plug into the Arduino (red for 5V, black for ground, and yellow for pin 8).
Solder the magnetic switches to the PC board. One side should be connected to 5V (the red wire on the servo) and the other side should be connected to a 10k pull-down resistor and a blue wire that will lead to one of the Arduino's digital pins. The six switches will be connected to the Arduino's pins 2-7.
Solder the buzzer. The black wire should be soldered to ground and the red wire should be soldered to a white wire that will lead to pin 9 on the Arduino.
Solder the pushbutton to the PC board. The red wire should be soldered to 5V and the green wire should be soldered to a pull-down resistor and an additional green wire leading to pin 10 on the Arduino.
Step 9: Drill
Drill a hole into the side of the 1/2" thick piece of wood using a 3/32" drill bit.
Widen one of the existing holes in the servo horn by drilling into it using the same drill bit.
Using one of the silver screws provided with the servo, attach the wheel to the wood. This will serve as the lever for opening and closing the drawer.
Reattach the servo horn to the servo. You should place it such that when the servo is in the zero position, the lever is perpendicular to the body of the servo.
Step 10: Mount Servo and Lever
Glue the four 1/2" squares cut out of 1/4" wood onto the 1.5" square cut from 1/8" wood. One smaller square should be glued to each corner of the larger square. Zip tie this piece to the top of the servo.
Glue this wooden mount attached to the servo by zip ties to the bottom of the top of your board. it should be placed such that when the lever is down, it holds the drawer closed and when the lever is lifted, it lies flat against the top of the box.
Step 11: Program
<pre>/* /* * Secret Compartment Chess Set * By Nicole Grimwood * * For more information, please visit: * https://www.instructables.com/id/Secret-Compartment-Chess-Set * * This code is in the public domain. */ #include <Servo.h> Servo myservo; // create servo object to control a servo int pos = 0;// variable to store the servo position // Pins for magnetic switches int switchPin1 = 2; int switchPin2 = 3; int switchPin3 = 4; int switchPin4 = 5; int switchPin5 = 6; int switchPin6 = 7; int servoPin = 8; // Pin for servo int buzzerPin = 9; // Pin for buzzer int resetPin = 10; // Pin for reset button boolean closed; // keep track of whether the drawer is currently open boolean switchOn; // keep track of whether all magnetic switches are open boolean resetOn; // keep track of whether reset button is pressed void setup(){ Serial.begin(9600); myservo.attach(8); // attaches the servo on pin 8 to the servo object myservo.write(pos); // Close drawer on servo by lowering lever to position 0 pinMode(switchPin1,INPUT); pinMode(switchPin2,INPUT); pinMode(switchPin3,INPUT); pinMode(switchPin4,INPUT); pinMode(switchPin5,INPUT); pinMode(switchPin6,INPUT); pinMode(resetPin,INPUT); pinMode(servoPin,OUTPUT); pinMode(buzzerPin,OUTPUT); closed = true; // drawer is closed initally switchOn = false; resetOn = false; } void loop(){ switchCheck(); resetCheck(); // If drawer is closed and magnetic switches are open // raise servo to open drawer if(closed&&switchOn){ digitalWrite(buzzerPin,HIGH); delay(200); digitalWrite(buzzerPin,LOW); for(pos = 0; pos < 90; pos += 1){ // moves servo lever from 0 degrees to 90 degrees myservo.write(pos); // in steps of 1 degree delay(15); } closed = false; } // If drawer is open and reset is pressed, // lower servo to zero position to close drawer if(!closed&&resetOn){ digitalWrite(buzzerPin,HIGH); delay(200); digitalWrite(buzzerPin,LOW); for(pos = 90; pos>=-1; pos-=1){ // moves servo lever from 90 degrees to 0 degrees myservo.write(pos); // in steps of 1 degree delay(15); } closed = true; switchOn = false; } delay(500); } // Check if magnetic switches are open void switchCheck(){ int switchVal1 = digitalRead(switchPin1); int switchVal2 = digitalRead(switchPin2); int switchVal3 = digitalRead(switchPin3); int switchVal4 = digitalRead(switchPin4); int switchVal5 = digitalRead(switchPin5); int switchVal6 = digitalRead(switchPin6); // If all of the magnetic switch pins are high if(switchVal1==HIGH&&switchVal2==HIGH&&switchVal3==HIGH&& switchVal4==HIGH&&switchVal5==HIGH&&switchVal6==HIGH){ switchOn = true; } // If all of the magnetic switch pins are low if(switchVal1==LOW&&switchVal2==LOW&&switchVal3==LOW&& switchVal4==LOW&&switchVal5==LOW&&switchVal6==LOW){ switchOn = false; } } // Check if reset button has been pressed void resetCheck(){ int resetVal = digitalRead(resetPin); Serial.println(resetVal); if(resetVal==HIGH){ resetOn = true; } if(resetVal==LOW){ resetOn = false; } }
Step 12: Pieces
Glue a magnet to the bottom of each of the chess pieces.
Step 13: Spring
Mount the spring behind the drawer.
Step 14: Play
Glue the top onto the box and begin storing and playing!
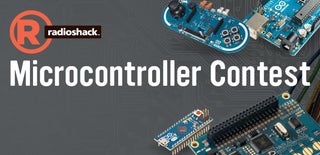
Participated in the
Microcontroller Contest