Introduction: Remote Controlled Microbit Robot
I use Microbits in tech clubs and wanted a simple remote controlled robot that was easy enough for children to build and code. This project uses two Microbits, one as the controller and one to drive the motors. The robot is controlled by tilting the Microbit controller, it broadcasts the value of the x and y orientation using the accelerometer. They communicate over the onboard Bluetooth ('Radio' in the PXT editor) To keep things simple it uses the Kitronik motor driver board and their own block to drive the motors. It also uses two micro metal gear motors, 3d printed wheels and an old CD as a chassis.
Both hex files needed are included in the discussion about the code. Alternatively you can see both scripts at the following links;
Controller - https://pxt.microbit.org/45274-63007-00432-61019
Robot - https://pxt.microbit.org/50000-70822-21817-78361
(Robot requires Kitronik block - See https://www.kitronik.co.uk/blog/using-pwm-kitronik...)
Parts needed
- 2 x Microbits
- Kitronik Microbit motor driver board
- 2 x micro metal gear motors with connector shim
- Battery box 4 x AA and connector
- Battery box 2 x AAA with JST connector
- An old CD (ask your parents!)
- 4 x Nylon Nuts and Bolts M3x20
- 4 x Metal M2x30 nuts and bolts
- 2 x 3d printed wheels and mounts**
- 2 x o rings / elastic bands for tyres
- 4 x M-F jumper leads to connect the motors
- Some sticky pads
** These links are to designs by bellbm on Thingiverse. If you haven't got access to a 3d printer round plastic lids work as wheels, use a bit of cork glued to the lid to mount them to the motor shafts.
Tools needed
- A drill to make holes in the CD
- Small screwdriver for the terminal blocks
- A 3d printer, or a friendly makerspace that has one.
Step 1: Build the Robot
To build the robot you need to drill some holes into your CD chassis so that you can fix the board and motor mounts in place.
Put the pieces on the CD as shown above and mark where you need to drill the holes. There are two for each motor mount and four for the motor controller board. It's important the motors are opposite each other, draw a line right across the CD going through the middle to help with this.
Now carefully drill the holes in the CD.
Use the four nylon bolts to fix the motor controller board in place.
Fix the wheels to the motor shafts and add the tyres, then use the four metal bolts to fix the motors to the CD using the motor mounts. (NB I didn't have the correct bolts when I took the photos so I used threaded bar)
Step 2: Wire Everything Up
Use the jumper wires to connect the motors to the terminal blocks on the motor controller. Use the 'Motor 1' and 'Motor 2' blocks.
Then connect the other ends to the motors. At this stage it doesn't matter which wire connects to the + and - pins. If your robot doesn't behave as it should swap them round.
Connect the 4AA battery box connector to the power terminal block. Make sure the + and - are the right way round!
Use the sticky foam pads to fix the 4AA battery box to the other side of the CD to the motor controller. Leave the battery unconnected for now. Your robot is built, now to write the code.
Step 3: Program the Controller
The code for the controller is fairly straightforward.
Start loop
In the 'On start' loop you need to set the radio channel with a 'Radio set group' block. I'm using '1' in the attached code but as long as you use the same for both Microbits it will work. I also use a 'Show icon' block to display a heart image on the LEDs, this is just an extra clue to show the code is successfully deployed, it's not needed for the robot to work. (But who doesn't like a bit of extra love?)
Forever loop
The only other code needed is in the 'Forever' loop. We are going to broadcast the values of the X and Y readings from the accelerometer over and over. X is the value that tells us if the Microbit is tilted left or right, sometimes called the roll. Y tells us if it's angled forward or backwards, know as the pitch.
So that the receiving Microbit knows if the controller is sending an X or a Y value we use 'Radio send value' blocks. This lets us transmit the value from the accelerometer and a name that tells us which direction of tilt the number relates to. So 'x' for the X value and 'y' for the Y value.
Before we send the value of the acceleromoter we divide it by ten. This is because the accelerometer gives us values between -1024 and +1024, but the motor block wants values between 0 and 100. So if we divide these numbers by ten we save ourselves some work later.
Then all we need to do is send the numbers. This code sends the x value, pauses for 100ms and then sends the y value. After another quick pause it returns to the top of the loop and starts again. The pause is there to give the receiving Microbit time to receive and store the numbers. Try experimenting with different pause times, or miss them out altogether and see what happens.
That's it. Download the code and send it to one of the Microbits.
Attachments
Step 4: Program the Robot Microbit - Part One
This code is a bit more tricky, so it gets two steps! But don't worry we'll go slowly. (and you can download and deploy the hex file and then upload it to the PXT editor to play with if you like.)
Start loop
The 'Start loop' code is very similar to the controller. We need to set the radio group so that the Microbit knows what it's listening for. I've also added a 'Show icon' block with a different image so that it's easy to work out which Microbit is which. In this code I've also set up two variables called xValue and yValue to store the numbers we receive from the controller. They are both set to 0 to start with.
On radio received loop
We also need to write the code that catches the numbers broadcast by the controller Microbit. The on radio received loop is triggered every time a message is sent from the other controller. Each time it receives the name and value we sent from the controller. So that we can store them in the correct variable we use an 'If' block and some logic to check the name. Then we can store the value in either xValue and yValue depending on which name, x or y, we received. We will use these values in the next step to control the motors.
Attachments
Step 5: Program the Robot Microbit - Part Two
Now the code that makes the wheels turn. I'm using the Kitronik motor driver and they have provided some helpful code that goes with it that makes it easier to control motors. To get their code click 'Add package' in the PXT editor and then paste this address into the box. (Clicking the link here will take you to the code page on Github if you want to see what they have done.)
https://github.com/KitronikLtd/pxt-kitronik-motor-...
More details of this code can be found on the Kitronik blog post about the motor controller board.
Forever loop
There are two parts to the forever loop, doing the maths to work out the speed and direction of the motors and then sending this information to the motor driver board. This is the hardest bit of the project so you might need to read it a few times!
Deciding on speed and direction for each wheel
To make the robot move we are going to use the Y value, tilting the board forward and backwards, to move the robot forwards and backwards. To make this happen we need to turn both wheels at the same speed in the same direction. If the yValue is a positive number we'll go forward, if it's negative we'll go backwards.
For turning we'll use the xValue number as the speed, but turn the wheels in opposite directions. This will make the robot spin. A positive X value will mean the wheel turns forwards, and a negative value backwards. So for the left wheel we will use +xValue, for the right wheel we will use -xValue.
Putting this together we can add the xValue and yValue together to come up with a value for each wheel called 'leftWheel' and 'rightWheel'
leftWheel = yValue + xValue
rightWheel = yValue - xValue
Actually making the wheels move
Now we have our leftWheel and rightWheel values we can send them to the motors. There is an 'If' block for each motor, this is to check if the leftWheel (or rightWheel) value is positive or negative. To do this we check if the number is greater than 0 (leftWheel > 0, rightWheel > 0). If it's a positive number we use the 'forward' option in the motor block, if it's negative we use 'reverse'. Notice in the reverse blocks there is also an 'Absolute of' block before the wheel variable. This is because the motor block needs a positive number for the speed, 'absolute of' tells the Microbit to ignore the minus sign if a number is negative and use it as a positive number.
That's all we need! Download this code to the other Microbit and you are ready to go.
Step 6: Using Your Robot
Plug the controller Microbit into the motor driver board and connect the 4AA battery box. The motor board powers the Microbit and the motors, so you should see your icon on the LEDs.
Plug in the two AAA battery box into the remote Microbit.
Now if you tilt the remote board your robot should move!
If you want to extend this project you can;
- Think about how we calculate the leftWheel and rightWheel variables. As the code stands these variables can be greater than the 100 maximum the motor block expects. This isn't a problem, it works, but can you think of a way to change the calculations so that the variables are always less than 100?
- Add lights, a buzzer or a micro servo to the breakout pins on the motor driver board so your robot can do more. You can use the buttons on the Microbit to control these extras.
- Design a new chassis to replace the CD
- Show the direction the controller board is tilting with an arrow on the robot Microbit LEDs
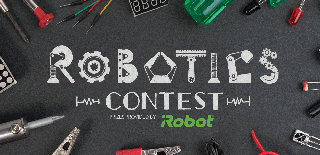
Participated in the
Robotics Contest 2017