Introduction: Actual Mac Air
The purpose of this project is to try to control features on your computer through the air by using Arduino and Python. After you set up this process you will be able to increase and decrease the volume of your computer. As well you are able to pause or play songs.
Step 1: Set Up Arduino Code
To start this first we woulld recomend you do the Arduino code first.
This code is the Arduino code:
const int trigger1 = 2; //Trigger pin of 1st Sesnor
const int echo1 = 3; //Echo pin of 1st Sesnor const int trigger2 = 4; //Trigger pin of 2nd Sesnor const int echo2 = 5;//Echo pin of 2nd Sesnor long time_taken; int dist,distL,distR; void setup() { Serial.begin(9600); pinMode(trigger1, OUTPUT); pinMode(echo1, INPUT); pinMode(trigger2, OUTPUT); pinMode(echo2, INPUT); } /*###Function to calculate distance###*/ void calculate_distance(int trigger, int echo) { digitalWrite(trigger, LOW); delayMicroseconds(2); digitalWrite(trigger, HIGH); delayMicroseconds(10); digitalWrite(trigger, LOW); time_taken = pulseIn(echo, HIGH); dist= time_taken*0.034/2; if (dist>50) dist = 50; } void loop() { //infinite loopy calculate_distance(trigger1,echo1); distL =dist; //get distance of left sensor calculate_distance(trigger2,echo2); distR =dist; //get distance of right sensor //Uncomment for debudding /*Serial.print("L="); Serial.println(distL); Serial.print("R="); Serial.println(distR); */ //Pause Modes -Hold if ((distL >40 && distR>40) && (distL <50 && distR<50)) //Detect both hands {Serial.println("Play/Pause"); delay (500);} calculate_distance(trigger1,echo1); distL =dist; calculate_distance(trigger2,echo2); distR =dist; //Control Modes //Lock Left - Control Mode if (distL>=13 && distL<=17) { delay(100); //Hand Hold Time calculate_distance(trigger1,echo1); distL =dist; if (distL>=13 && distL<=17) { Serial.println("Left Locked"); while(distL<=40) { calculate_distance(trigger1,echo1); distL =dist; if (distL<10) //Hand pushed in {Serial.println ("Vup"); delay (300);} if (distL>20) //Hand pulled out {Serial.println ("Vdown"); delay (300);} } } } //Lock Right - Control Mode if (distR>=13 && distR<=17) { delay(100); //Hand Hold Time calculate_distance(trigger2,echo2); distR =dist; if (distR>=13 && distR<=17) { Serial.println("Right Locked"); while(distR<=40) { calculate_distance(trigger2,echo2); distR =dist; if (distR<10) //Right hand pushed in {Serial.println ("Rewind"); delay (300);} if (distR>20) //Right hand pulled out {Serial.println ("Forward"); delay (300);} } } } delay(200); }
Step 2: Make Python Code
After you do the Arduino code make your Python code next.
This is the Python code we used:
Python Code:
import serial #Serial imported for Serial communicationimport time #Required to use delay functionsimport pyautogui ArduinoSerial = serial.Serial('com18',9600) #Create Serial port object called arduinoSerialDatatime.sleep(2) #wait for 2 seconds for the communication to get established while 1: incoming = str (ArduinoSerial.readline()) #read the serial data and print it as line print incoming if 'Play/Pause' in incoming: pyautogui.typewrite(['space'], 0.2) if 'Rewind' in incoming: pyautogui.hotkey('ctrl', 'left') if 'Forward' in incoming: pyautogui.hotkey('ctrl', 'right') if 'Vup' in incoming: pyautogui.hotkey('ctrl', 'down') if 'Vdown' in incoming: pyautogui.hotkey('ctrl', 'up') incoming = "";
Step 3: Start Building
After you set up both codes start building it. Follow the picture we provided to build it.
Step 4: Actions
Action 1: When both the hands are placed up before the sensor at a particular far distance then the video in VLC player should Play/Pause.
Action 2: When right hand is placed up before the sensor at a particular far distance then the video should Fast Forward one step. Action 3: When left hand is placed up before the sensor at a particular far distance then the video should Rewind one step. Action 4: When right hand is placed up before the sensor at a particular near distance and then if moved towards the sensor the video should fast forward and if moved away the video should Rewind. Action 5: When left hand is placed up before the sensor at a particular near distance and then if moved towards the sensor the volume of video should increase and if moved away the volume should Decrease.
Step 5: Conclusion
Hopefully, this works for everyone and this is a fun and interesting thing to try.
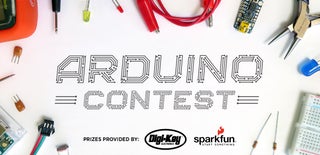
Participated in the
Arduino Contest 2017