Introduction: AllSense: an Electronic Multi-Tool
This device is still in alpha. In the future it will have an air quality sensor among other useful sensors. But this is how to make it in its present state. I'll go updating this instructable with the new upgrades I make on the device.
This is my second instructable and I hope you enjoy it. If you have any questions, please feel free to ask them in the comments.
If you like this instructable then I would really appreciate your vote in the contests I've entered, thank you!
Step 1: Aquire the Materials
Materials
• Many male to male jumper wires.
• Many male to female jumper wires.
• A 9V rechargeable battery and adapter.
• An LCD screen 16x2.
• A stripboard.
• Soldering wire.
• A diode.
• An Arduino UNO board.
• A breadboard.
• A temperature and humidity sensor, I used the DHT11.
• A air pressure sensor, the BMP180.
• A DC power connector, one that's able to charge a 9V battery, it must be a cylindrical type of connector, one with a sleeve and a tip.
• A input socket for the above connector.
• A 3.3MΩ resistor.
• About 10cm (4") of solid core wire.
• Glue sticks.
• A box (can be recycled, 3d printed, etc.) that is made of a hard material, like plastic. I used a recycled watch box that looks really good. With more or less these dimensions: 10.5cm * 10.5cm * 7.5cm (4" * 4" * 2.7").
• A tilt switch.
Tools
• A drill.
• A glue gun.
• A hacksaw.
• Soldering Iron.
Step 2: The Enclosure
Ok then. This first step is probably the simplest. We just need to cut a rectangle on the top of the box that you've decided to use to enclose the electronics. The rectangle should match the size of the LCD's actual screen, mine measured 7 by 2.4cm (or 2.7" by 0.94").
To cut out this figure, first mark the rectangle on the plastic surface with a pencil. Once marked, drill a few holes along each side. Making sure the line formed by the series of holes is long enough for your hacksaw's blade to fit in. On the two shorter sides, I just drilled the holes necessary to reach the whole line, there wasn't any point in using the saw.
Now sand down the edges with some sanding paper to get rid of the rough edges. Make sure your LCD's screen area fits through the rectangle and then you're ready to pass on to the next step.
You should also drill a hole on a side of about 7mm (0.275") in diameter, this will be for connecting the cable to charge AllSense.
Step 3: The Electronics
This step is the most complicated, so I have also made a visual representation of all the components and the way they are connected using a program called Fritzing. I say it's the most complicated because I had to put it all together from scratch on a breadboard and then take all my work off the breadboard and solder it to a stripboard, but luckily, since I've already done all that, you can directly solder it on the stripboard.
It should be quite clear what wire goes where. The only thing I think I should mention is that the grey thing to the bottom right of the LCD screen is the tilt switch, which is connected to pin 8 on the Arduino.
If you have any other issues figuring out what connects to what, then please ask me in the comments or open up the file I've uploaded to this step with fritzing, which is free to download. There you'll be able to hover the mouse over every connection and wire to know exactly where it goes.
What you can see coming out of pin A0 is the probe antenna for the EMF function, and also connected to this pin is the 3.3MΩ resistor that leads to GND.
It may be a bit difficult to keep all the wires down, so I used hot glue to glue the wires as I went soldering them to the different parts, otherwise they keep coming off.
Attachments
Step 4: The Code
We have all the electronics ready for use now, so let's upload the code. I've put a link below that will lead you to a public gist on GitHub where you can see all my code used in a perfect format, because the code kept getting changed and messed up a bit when I directly paste it as code here, but I've done it both ways. As well there should be a ".ino" file on this step that will download as a IDE file to open with the Arduino code program.
As you may have noticed, at the beginning of the code I include some libraries, so I've uploaded all the ones that I used and that don't come already with the Arduino program to this step. If you don't know how to install libraries check out this Arduino tutorial that explains it very well: Installing Libraries
The LiquidCrystal and Wire libraries are already on come withe the program.
If you want to change the time the screen stays on for then change the value that i has to reach in the while statement on line 60. Or if you want to change the time between every update of the readings then change the delay() on line 127.
And if the EMF reading is always high or never high, then you may want to fiddle about with the if statements starting on line 99, changing the values according to the problem.
Please read the comments to understand the code. The whole EMF part gets pretty complicated, if you want a more detailed explanation on this part then check out this link: http://www.aaronalai.com/emf-detector
#include <dht.h> //Here we include all the libraries that we downloaded...
#include <BMP085.h>
#include <Wire.h>
#include <LiquidCrystal.h>
#define dht_apin A2 //saying what pin the DHT module should read the info.
#define NUMREADINGS 15 // here we are defining NUMREADINGS as 15 data values; raise this number to increase data smoothing. This is for the EMF.
int senseLimit = 15;
int probePin = A0; //setting probePin as analog 0.
int val = 0; // reading from probePin
dht DHT;
BMP085 bmp;
LiquidCrystal lcd(12, 11, 5, 4, 3, 2); //starting the lcd with the pins we assigned.
const int inUse = 8; //this will be the pin where the tilt switch is connected to.
int i = 0;
const int screen = 6;
int readings[NUMREADINGS]; // the readings from the analog input
int index = 0; // the index of the current reading
int total = 0; // the running total
int average = 0;
void setup() {
// set up the LCD's number of columns and rows:
lcd.begin(16, 2);
Serial.begin(9600);
bmp.begin();
// Print a message to the LCD.
pinMode(inUse, INPUT);
pinMode(screen, OUTPUT);
for (int i = 0; i < NUMREADINGS; i++)
readings[i] = 0;
}
void loop() {
i = 0;
if (digitalRead(inUse) == HIGH) {
lcd.clear();
digitalWrite(screen, LOW);
}
else {
while (i < 30) {
digitalWrite(screen, HIGH);
DHT.read11(dht_apin);
// set the cursor to column 0, line 1
// (note: line 1 is the second row, since counting begins with 0):
//Print a message to second line of LCD
lcd.clear();
lcd.setCursor(8, 0);
lcd.print((bmp.readPressure() + 2000) / 100);
Serial.println(bmp.readPressure());
lcd.print("hPa");
lcd.setCursor(1, 1);
lcd.print(DHT.humidity);
Serial.println(DHT.humidity);
lcd.print("%");
lcd.print(" ");
lcd.print(DHT.temperature);
lcd.print("C");
val = analogRead(probePin); // take a reading from the probe
lcd.setCursor(0, 0);
lcd.print("IE");
if (val >= 1) { // if the reading isn't zero, proceed
val = constrain(val, 1, senseLimit); // turn any reading higher than the senseLimit value into the senseLimit value
val = map(val, 1, senseLimit, 1, 1023); // remap the constrained value within a 1 to 1023 range
total -= readings[index]; // subtract the last reading
readings[index] = val; // read from the sensor
total += readings[index]; // add the reading to the total
index = (index + 1); // advance to the next index
if (index >= NUMREADINGS) { // if we're at the end of the array...
index = 0; // ...wrap around to the beginning
}
average = total / NUMREADINGS;
Serial.println(average);
if (average > 150) { //checking the value of the average and printing a ">" if it's significant.
lcd.print(">");
}
if (average > 350) { //checking again, but this time for a higher value.
lcd.print(">");
}
if (average > 550) { // and so on...
lcd.print(">");
}
if (average > 750) {
lcd.print(">");
}
if (average > 950) {
lcd.print(">");
}
}
i += 1;
delay(1000);
}
}
}
Step 5: Securing the Electronics to the Box
Ok, nearly finished, now we need to take the electronics that you soldered in the last step and secure them to different parts of the enclosure.
The first thing to secure is the LCD screen, which we'll be securing to the rectangular hole we made for it on the top. If you cut the hole a bit too big, like me, then put some hot glue in the gap between the LCD's borders and the hole. Once you've done this, stick the Arduino and battery or battery connector to the inner base of the box with some double-sided tape or blu-tack.
Then come the two sensors and the probe for the EMF function. Use some double-sided tape to stick the BMP180 and the DHT11 to the inner sides of the box. Try to keep them as far away from anything that you think could generate heat; then just glue with hot glue the solid-core wire that is used as the probe to a inner side as well, but make sure it isn't too close to any electronic components that you think could generate a significant amount of an electromagnetic field.
The last things to secure to the base are the tilt switch, which has to be positioned at an inclined angle to make sure that it is easily deactivated (thereby activating the screen), and the jack for the cylindrical cable connector that charges the 9V battery, which has to be well aligned with the hole we drilled on the side of the box. Secure these two pieces with hot glue.
Step 6: Finished
And there you have it! A multi-use, electronical, arduino based, tricorder-like box called AllSense.
It is very easy to use, once you've connected the battery and charged it if it needed charging, every time you move the box a small bit, the LCD will light up, displaying the humidity, temperature, air pressure and any nearby EMF. The readings will update every second for 30 seconds.
In the future I'll maybe add a strap so you can take out and about the place to take readings, and I'll probably make small "ventilation" holes so the readings will be more accurate.
I hope you enjoyed this instructable, and even though making things can get very stressful sometimes, the important thing is to push through these moments until you reach the end, then you won't regret it. If you find any errors or you have any questions feel free to tell me in the comments.
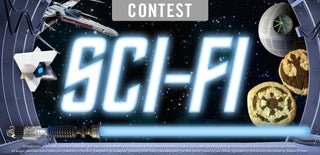
Participated in the
Sci-Fi Contest
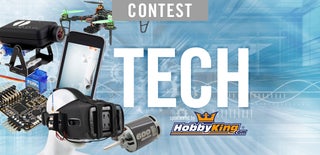
Participated in the
Tech Contest