Introduction: Analog Clock Using Math, Arduino Pro Mini, DS3231 and Nokia 5110
A digital clock is a clock which simply shows numbers to denote the time e.g. 20:56. The signal from a real time clock module (RTC)(DS3231) attached to an Arduino pro mini and a Nokia 5110 display delivers time in such a digital format.
An analog clock is a circular-faced clock with the numbers one to twelve around the outside and two hands, a shorter one pointing on hours and a longer one pointing on minutes.
This Instructable shows you how to convert a digital signal from a real time clock module into an analog format using mathematics, especially trigonometric functions like sine and cosine and provides you the code (see Download at the end of this Instructable).
The math will be done by the code loaded on an Arduino pro mini (3.3V, 8 MHz). The project is powered by a Lithium-Ion Battery 18650 salvaged from an old laptop. The module TP4056 is used as a battery management system.
As a help to understand each individual calculation an Excel file is enclosed with the explanations and the values. The file can be downloaded in Step 5: Calculation of x1 and y1.
If you have spent hours like me in math class and asked yourself if you ever will use these functions, here is a useful example. I hope you like it and vote for me in the math contest.
Step 1: Parts List
- Arduino pro mini (3.3V, 8 MHz) http://s.click.aliexpress.com/e/2PrDk8V2
- Nokia 5110 Display http://s.click.aliexpress.com/e/knP27UNE
- RTC DS3231 http://s.click.aliexpress.com/e/CFGfEpws
- LIR2032 3.6V rechargeable button cell battery http://s.click.aliexpress.com/e/sDHDzmbm
- Micro USB 5V 1A 18650 Lithium Battery Charger Module http://s.click.aliexpress.com/e/ruhZUSQk
- Rechargeable 3.7V Li Battery 18650 PF 2900mah http://s.click.aliexpress.com/e/FIMoFDL2
- 18650 Battery Case Holder http://s.click.aliexpress.com/e/ldnQ4zJa
- Cable for Breadboard http://s.click.aliexpress.com/e/qcSGRaqc
- Jumper Cable Wires Kit http://s.click.aliexpress.com/e/omM0nYaU
- Breadboard 400 Tie points http://s.click.aliexpress.com/e/pTzBAz8k
- Breadboard 170 hole http://s.click.aliexpress.com/e/eJXnomrA
- Micro switch http://s.click.aliexpress.com/e/pb8QDXzm
- Single row male pin header strip long 17mm
Step 2: General Idea
The need for a time stamp together with measured values (e.g. time: 9:25 and temperature: 18°C) is so important especially when you want to draw a graph, that I often wonder why a real time clock is not included directly in the Arduino environment. Of course there is the possibility to use the in-build millis() function but as soon as you lose power you lose the time and you have to start again. So you end up by integrating an external real time clock module (RTC) in your project. In this project the DS3231 real time clock is used together with a rechargeable battery.
The next step is how to display time from the RTC module. The cheap monochrome LCD Nokia 5110 display was used because it runs at 3.3 Volts (Li-Ion battery). It has low energy consumption and there are library’s easy available with manuals how to run the display. The library “LCD5110_Graph” was downloaded from the Rinky-Dink Electronics webpage (Graph Library). The library provides a function called “drawLine(x1, y1, x2, y2)” (see manual). This function allows you to draw lines between points. This is exactly what we need to draw the analog clock and the clock hands.
Now the question is how to translate the digital signal (e.g. 9:25) into a drawing showing the time in an analog version?
The general idea is that the LCD display with its 84x48 pixels, is our Cartesian coordinate system. A Cartesian coordinate system is a coordinate system that specifies each point uniquely in a plane (e.g. here the LCD display) by a set of numerical coordinates, which are the signed distances to the point from two fixed perpendicular oriented lines, measured in the same unit of length. Each reference line is called a coordinate axis of the system.
Each pixel on the Nokia display can be described by using the numerical coordinates within the 84x48 system. As an illustration I added a picture to visualize the x- and y-axis. Later on (see picture in step 4) the exact numbers of the x- and y-axis of the display are indicated from 0 to 47 (48 pixels) and 0 to 83 (84 pixels). This is a definition coming from the library. The function “myGLCD.drawLine (0, 0, 83, 47)” draws a line from the upper left to the lower right corner (see manual).
The corner points of our clock hands therefore can be described by numbers (points) within a Cartesian coordinate system. With the help of the "drawLine" function of the library we can connect these corner points to form a clock hand.
Step 3: Clock Hands
The clock hands can be drawn in various shapes. The simplest clock hand version is by just combining two points with a line (see picture). Using three points the clock hands look like arrows. The form I like best is the form with 4 points. The next steps are explaining how to calculate these 4 points.
Step 4: Center Point
In the picture the clock hands are indicated by lines of different color to display two times (9:25 and 2:05). Four corner points are connected by four lines to form a clock hand. For the time 2:05 (black color), the hour clock hand is described by the following coordinates:
1. Point: cCx+x1 / cCy+y1
2. Point: cCx+x2 / cCy+y2
3. Point: cCx+x3 / cCy+y3
4. Point : cCx+x4 / cCy+y4
Why does these coordinates look so complicated when you actually need only two numbers to describe the points? This is because we use a reference point within our clock and that is the ClockCenterPoint (cCx = clockCenterX / cCy = clockCenterY). The reason to choose this reference point is because all information within the circular-faced clock can be derived from the center. Let’s start with the center point.
Center point:
cCx = Is half the x-axis – using a Nokia display half of 84 is 42
cCy = Is half the y-axis – using a Nokia display half of 48 is 24
… so at the moment we have the following coordinates for point 1: 42 + x1 / 24 + y1
Step 5: Calculation of X1 and Y1
The blue rhombus within the picture is the symbol for the hour hand e.g. showing two o’clock. We have defined the center point as a start (see green point in the picture). To use trigonometric functions we need to draw a right-angled triangle including the center point and the two o’clock point. This right-angled triangle is indicated with dotted lines. Given an acute angle alpha (yellow color in the picture) of the right-angled triangle the hypotenuse (hhe = hour hypotenuse end) is the side that connects the two acute angles. The side adjacent to alpha is the side of the triangle that connects y1 to the right angle. The third side (x1) is said opposite to y1.
The trigonometric functions that can be applied here are (a = alpha .... seems that this homepage is not supporting Greek letters ...sorry):
sin a = opposite/hypotenuse
If you substitute opposite by x1 and hypotenuse by hhe (hour hand end) you can solve the equation to receive: sin a = x1/hhe -> x1 = sin a x hhe
The variable “hhe” stands for the length of an hour hand. When using the Nokia 5110 display, half of 48 pixels are 24 pixels. During experimenting, I found empirically that 25 works as well as a maximum length for a hand. Since the hour hand should be a little shorter than the minute hand 20 was found to look nice. So replacing hhe in the equation with 20 means:
x1 = sin a x 20
Now the only unknown variable left is the angle. The angle is dependent from the digital time coming from the RTC module in the following way: 360° degrees (clock face) divided by 12 hours = 30° degrees per hour.
Since the hour hand is also moving between one hour, we include:
30° degrees per hour divided by 60 Minutes = 0.5° degrees per minute. The angle therefore can be calculated in the following way:
a = (Hour x 30°) + (Minute x 0.5°)
In our example with 2:05 (see picture in step 4) we obtain the following angle: (2 x 30) + (5 x 0.5) = 62.5°
x1 = sin 62.5 x 20
When solving trigonometric expressions like sine or cosine, it is very important to realize that Arduino and Excel uses radians, not degrees to perform these calculations! If the angle is in degrees you must first convert it to radians. To convert degrees to radians, take the number of degrees to be converted and multiply it by π/180. When checking the code for Arduino (see step 10) you will find the expression π/180 replaced by 0.0175.
General: x1 = sin (a/180 x π) x hhe
Example with 2:05: x1 = sin (62.5/180 x π) x 20 -> x1 = 18 for the hour hand (Excel File for calculation can be downloaded in step 5)
It is the same for y1 just using cosine function and a negative value (due to the orientation within the system).
General: y1 = -cos (a/180 x π) x hhe
Example with 2:05: y1 = -cos (62.5/180 x π) x 20 -> y1 = -9 for the hour hand (Excel File for calculation can be downloaded in step 5)
1. Point: cCx+x1 / cCy+y1: 42 + 18 / 24 - 9 -> 60 / 15 (the first coordinates of the hour hand).
Check out the register "Drawing" in the Excel File. There you can verify if the coordinates are matching.
Attachments
Step 6: Calculation of X2 and Y2
Calculation of the coordinates for point 2 of the hour hand is only slightly different to the calculation shown in step 5. The difference is that the hypotenuse has a different length. Instead of using the variable hhe (20) the variable hhs (hour hypotenuse start) is used with a value of 3.
General: x2 = sin (a/180 x π) x hhs
Example with 2:05: x2 = sin (62.5/180 x π) x 3 -> x2 = 3 for the hour hand
For y2: = -cos (a/180 x π) x hhs
Example with 2:05: y2 = -cos (62.5/180 x π) x 3 -> y2 = -1 for the hour hand
2. Point: cCx+x2 / cCy+y2: 42 + 3 / 24 - 1 -> 45 / 23 (the second pair of coordinates of the hour hand).
Step 7: Calculation of X3 and Y3
Calculation of the coordinates for point 3 of the hour hand: Here the length of the hypotenuse is somewhere between 20 and 3. Just from the "looks" 8 has been found to look nice (variable hhm = hour hand middle). In addition the angle A (14° degrees) for the rhombus has to be added to alpha.
General: x3 = sin ((a+A)/180 x π) x hhm
Example with 2:05: x3 = sin (76.5/180 x π) x 8 -> x3 = 8 for the hour hand
For y3: = -cos (a+A/180 x π) x hhm
Example with 2:05: y3 = -cos (76.5/180 x π) x 8 -> y3 = -2 for the hour hand
3. Point: cCx+x3 / cCy+y3: 42 + 8 / 24 - 2 -> 50 / 22 (the third pair of coordinates of the hour hand).
Step 8: Calculation of X4 and Y4
Calculation of the coordinates for point 4 of the hour hand: In contrast to point 3 the angle A (14° degrees) has to be subtracted from alpha.
General: x4 = sin ((a-A)/180 x π) x hhm
Example with 2:05: x4 = sin (76.5/180 x π) x 8 -> x4 = 6 for the hour hand
For y4: = -cos (a-A/180 x π) x hhm
Example with 2:05: y4 = -cos (76.5/180 x π) x 8 -> y4 = -5 for the hour hand
4. Point: cCx+x4 / cCy+y4: 42 + 6/ 24 - 5 -> 48 / 19 (the last coordinates of the hour hand).
The calculation for the 4 points of an hour hand is now completed.
The calculation for the minute hand is similar just the parameters are different (mhe=25, mhm=10, mhs=3). The angle again is dependent from the actual time in the following way: 360° degrees (clock face) divided by 60 minutes = 6° degrees per minute. Because the minute hand is thinner, the angle A used is 8° degrees instead of 14° degrees compared to the hour hand. The rest is calculated as for the hour hand.
Step 9: Assemble Arduino
The hardware required for this project can be found in the chapter “parts list”. Assemble everything as given in the R&I scheme (see picture in step 9). The video may help you where to place each component in order to build a very compact programming platform. It is so small that you can take it with you on a holiday trip. As you can see I used it with a convertible notebook at the beach.
Step 10: Upload Code
The code for the Arduino is given in the file below. When scrolling through the program in the IDE you will find the corresponding trigonometric functions in the code like explained in the previous steps. After having assembled the hardware you can upload the code and enjoy your analog clock.
........ finally something useful done using trigonometric functions!
Hope you enjoy this Instructable and please vote for me in the math contest.
Attachments
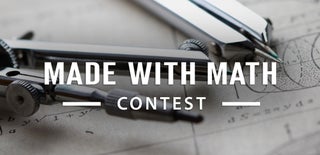
Participated in the
Made with Math Contest