Introduction: Anybody Home ???
IOT is fun and commanding devices from a web-page or with Google Home is great. Even better is to have things automated. The Philips Hue lamps and a system called KlikAanKlikUit in the Netherlands have a great feature: their controller detects if you or one of your loved ones approach your home and switch on the lights automatically.
I always wondered how they did that and actually it is quit simple.
Most people like myself always carry their mobile phone around. So to test if someone is around you only have to check if their mobile phone is logged into your home network. You can do that by using PING. I am going to show you how to use the ESP8266 with a PING library to check wether a Phone is logged on and therefore that person is at home.
If you or the persons you want to involve (your loved ones for example) are at home or are approaching home a led will go ON. You can easily replace the leds by relays or altering the commands for switching leds on in commands that instruct your Home Automation system (like Domotics or Home Assitant).
And it need not be limited to approaching your home. You can start things automatically in your work-space or school or at your work this way if you have access to the information in their router.
Supplies
The setup is for explanatory purposes only and for this you will need:
- Breadboard
- Jumper wires
- 4 Leds
- 4 220 Ohm delimiting resistors
- ESP8266
Step 1: Breadboard Setup
I am going to show you how you can test if someone is at home or if your wireless devicess are attached to the internet and working as expected. For this I use a simple setup which you can change for your own needs. You can replace the leds with relays for switching lamps, ventilators, thermostat or whatever.
On the breadboard is an ESP8266 which has 4 leds attached to the following IO pins: D0, D1, D2 and D4.
Don't forget to attach current delimiting resistors of 220 ohm to each led.
Step 2: Find the IP Adresses of Mobile Phones
When the breadboard has been set up we need to know the IP adresses of the devices we want to test. I can not give you full details on this as this depends on how your router functions. I will tell you how it works in my particular case.
I have a Zyxell router. The settings of the router are accessed by a web-page. So I opened this webpage and there I can see the wired and wireless devices that are presently connected to my router. On the bottom are the wired devices and on top the wireless devices. You can see two devices: a thermometer besed on an ESP8266 and my Phone. By clicking on each device I will get more information and best of all I can give the device a fixed IP-adress.
So when my phone is out of the house (and therefore I am) the router looses contact to the phone. Then the IP adress vanishes from the list temperarely. As soon as I am in the vicinity of my home the IP adress appears again in my router.
This is what we can check with the ping command.
And that is what we are going to use to set a led on or off.
Step 3:
The code.
The last step is to write a program that will check if the IP adress is connected to your router. You will need a library for that and that is the PING library which you can find here:
https://github.com/dancol90/ESP8266Ping
There are plenty instructables that will teach you how to install a library in the Arduoino IDE.
Here is the full program:
/*
* Anybody Home ???
* This program tests IP numbers with PING to see if they are
* active. If so the Led will be on if the IP number is not
* active the Led will be off
* This way we can check activity and person presence
* Written by Luc Volders
*/
#include
#include (ESP8266Ping.h>
const char* ssid = "YOUR-ROUTER-NAME";
const char* password = "YOUR-ROUTER-PASSWORD";
const IPAddress thermometer(192, 168, 1, 65);
const IPAddress domoticz(192, 168, 1, 66);
const IPAddress android(192, 168, 1, 70);
const IPAddress elsphone(192, 168, 1, 75);
void setup()
{
pinMode(D0,OUTPUT);
digitalWrite(D0,LOW);
pinMode(D1,OUTPUT);
digitalWrite(D1,LOW);
pinMode(D2,OUTPUT);
digitalWrite(D2,LOW);
pinMode(D4,OUTPUT);
digitalWrite(D4,LOW);
delay(10);
// Start by connecting to our WiFi network
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED)
{
delay(100);
}
}
void loop()
{
if(Ping.ping(thermometer))
{
digitalWrite(D0,HIGH);
}
else
{
digitalWrite(D0,LOW);
}
if(Ping.ping(domoticz))
{
digitalWrite(D1,HIGH);
}
else
{
digitalWrite(D1,LOW);
}
if(Ping.ping(android))
{
digitalWrite(D2,HIGH);
}
else
{
digitalWrite(D2,LOW);
}
if(Ping.ping(elsphone))
{
digitalWrite(D2,HIGH);
}
else
{
digitalWrite(D4,LOW);
}
delay(1000);
}
I think the program is prerry self-explanatory however I will look into some details.
In the setup the network credentials are filled in for your router and password. Next the IP adresse for your devices are defined. You can see that I mixed wired and wireless IP adresses as I wnt to check wether someone is in and if my wired devices are working as expected. Then the pins for the leds are defined and the leds are put off.
The loop is where the action is.
The program pings each IP adress and sets the led ON if it is available and the led stays off when the IP adress is not responding.
This way we can test devices AND we can see if someones phone is present and we can safely presume that then that person is also present.
Step 4: Expanding and Real Life Use
As you can see it is quite easy to check if someone is at home or not.
If he/she is at home the led which represents his/hers IP adress is set ON.
if(Ping.ping(android))
{
digitalWrite(D2,HIGH);
}
This is the actual test. In this example android is the name attached to the IP adress of my phone. You can give it any name you want and you can replicate this part as many thimes as you like for other IP numbers.
The part where the led is switched on can be changed in anyting you like. Start a ventilator, send information to your home automation controller, have Google Home speak a welcome phrase (that is something that will be published on my weblog in the near future).
As with all my projects: use your imagination and be creative.
You can find more of my projects at my weblog:
http://lucstechblog.blogspot.com/
So have fun.
Luc
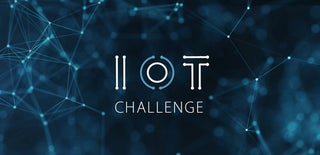
Participated in the
IoT Challenge