Introduction: Aquabot: Arduino Gardening System
Do you struggle to remember to take care of your houseplants? Do you worry that your plants will die while you are away on vacation? Aquabot is here to help! Aquabot is an automatic watering system built around an Arduino Uno. It can be easily upgraded to care for multiple plants, use data from more sensors, and automate more functions of your garden.
Step 1: Materials, Tools and Software
Materials for the Aquabot include:
- An Arduino Uno
- This is the "brain" that runs the Aquabot
- https://store.arduino.cc/usa/arduino-uno-rev3
- If you want to make your Aquabot smarter, you could connect it to WiFi with an Arduino WiFi Rev 2
- https://store.arduino.cc/usa/arduino-uno-wifi-rev2
- This is the "brain" that runs the Aquabot
- Relay shield
- This allows the Arduino to toggle on and off circuits of high voltages and currents.
- Small water pump
- Corrosion resistant soil moisture sensor
- Soil moisture sensors allow the Arduino to measure how much water is in the soil, either through resistance or capacitance changes in the soil. Resistance based sensors tend to cause electrolysis, corroding themselves over time. I recommend using this capacitance based sensor, as it is much more durable.
- https://www.dfrobot.com/product-1385.html
- Non contact liquid level sensor
This acts as a safety switch so the we do not run the pump dry. The sensor can tell if there is liquid on the other side of a membrane.
- Jumper wires
- Spare wires, 22 gauge and solid core is best. You may also want to use an old computer chord to extend the wires on the sensors or water pump.
- Switch
- Barrel jack
- A 9v and 12v power supply
- USB type A to B cable
- A small electric beeper (piezoelectric alarm)
- A waterproof box
- Electrical tape
- Tubing to conduct the water form the pump. The pump I suggested comes with plenty
Tools you may need include:
- Soldering iron
- Hot glue gun
- Drill
- Computer
- Needle nose pliers
- Small Screwdrivers
I recommend this site for any hardware or tools you need:
Software you will need:
- Arduino IDE, download the latest version here: https://www.arduino.cc/en/main/software
Step 2: Wiring
In the next few steps we will get the hardware set up.
First, we will take a look at the wiring.
When doing the wiring there are some important things to know. The makers of the shield and modules we are using color coded and labelled everything for us, so the connections are very easy to use. The wires for the modules are color coded and the corresponding pins on the Arduino are either labelled or color coded.
- Green = Digital pins, such as D1, 2, 3, etc...
- Blue = Analog pins, such as A0, 1, 2, etc...
- Black = GND (Negative)
- Red = Power (Positive)
The connections for the Aquabot are shown in the wiring diagram and listed here:
- Realay shield stacks onto the Arduino
- Soil moisture sensor connects to 3.3v, GND. and A0 (or the Gravity pins next to A0)
- Liquid level sensor connects to GND, 5v, and D5 (you cannot use the gravity pins for this module, as they only supply 3.3v, this took me quite a while to figure out!)
- Beeper connects to GND and D5 (Digital pins can either be used as input pins, or to supply power
- The barrel jack (for the 12v power supply) connects to the switch and COM on whichever relay you are using
- The switch connects to the positive connection on the barrel jack and the positive connection on the pump
- The pump connects to the switch and to NO on whichever relay you are using (NO stands for normally open)
- Lastly, the USB cable goes from your computer to the Arduino, the 12V supply goes to the barrel jack, and the 9v power supply goes to the Arduino.
If you are using the Gravity relay shield, you need to know the digital pin number for the realy you are using, it is printed in small text next to the relay, D10 for example and can also be fond on a chart here:
https://www.dfrobot.com/wiki/index.php/Relay_Shiel...
If you are not using the shield, wire the control part of the relay to a digital pin and GND.
Here is how to use a relay:
Step 3: Code
Now its time to get our Aquabot calibrated and running.
Connect the USB cable to the Arduino, open Arduino IDE, go to tools, port, and select the port with (Arduino/Genuino UNO) listed next to it.
Next go to tools, board and select (Arduino/Genuino UNO)
Now, copy the code form the attached file or below, and click Upload.
You will have to change this code depending on how you want Aquabot to maintain your soil
Here are a few sites to give you ideas for how to pot your plant and calibrate your Aquabot:
If you don't know how to code that is okay! I started this project with zero coding knowledge, and I've written comments to take you through the program step by step.
If you want an Arduino crash course, check out these two videos:
Lets review the code:
<p>/* <br>Aquabot Code V2.2 Last Update: 2/18/2019 Created by Grant B. Created to run AQUABOT, an open source garden care system to enable students to learn electronics. This code may be freely replicated, modified, distributed, and of course upgraded! Information on operation of this code may be found on Instrucatbles and the Arduino Forums. */ //Defining variables and telling the Arduino what pin each sensor is attached to int Voice = 4; //Beeper power pin int Relay4 = 8; //Relay4 = Pump int watersense = A0; //Watersense = moisture sensor int val = 0; //Reseting this value int waterset = 496; //water threshold (Change this to determine the soil saturation for each plant) int Watercheck = 5; //liquid level sensor int Pumptime = 3; //amount of time the pump runs, in seconds int Looptime = .1; //time interval between checks, in seconds int Loop = Looptime * 1000; //converting times into Arduino values int pump = Pumptime * 1000; //int light = 1; //pin to add a light sensor int reading; //this variable will count the amount of time the main loop runs //setup loop void setup() //setup code, telling the Arduino how to function during the program { Serial.begin(9600); // starts communicating with the serial monitor pinMode(Relay4, OUTPUT); // sets the Relay pin as an output pinMode(Watercheck, INPUT); // sets the liquid level sensor as an input pinMode(Voice, OUTPUT); // beeps to tell us that Aquabot is on/reset digitalWrite(Voice, HIGH); delay(500); digitalWrite(Voice, LOW); } //main loop void loop() //Runs as a series of checks and acts accordingly { int watersafe = digitalRead(Watercheck); val = analogRead(watersense); Serial.println("***********************************************"); //this is the start of the messages to the serial monitor, it reorde the readings from the sensors. Serial.println(" "); Serial.println("Water Level:"); Serial.println(" "); Serial.println(val); Serial.println(" "); Serial.println("Level Sensor Status:"); Serial.println(" "); Serial.println(watersafe); //run checks then respond if(val > waterset && watersafe == 1) { digitalWrite(Relay4, HIGH); // sets Relay #4 on //this is the case where watering happens, soil is dry, resevoir is full. Serial.println("Soil Status:"); Serial.println(" "); Serial.println("Dry"); Serial.println(" "); Serial.println("***********************************************"); Serial.println(" "); delay(pump); //runs the pump for a designated amount of time, change based on how much water you want per watering (note: 1000 is equal to 1 second) digitalWrite(Relay4, LOW); } if(val < waterset && watersafe == 0) { digitalWrite(Relay4, LOW); // sets Relay #4 on Serial.println("Soil Status:"); Serial.println(" "); Serial.println("Good"); Serial.println(" "); //soil is fine, reservoir is empty Serial.println("Please Refill Water Reservoir"); Serial.println("***********************************************"); Serial.println(" "); digitalWrite(Voice, LOW); delay(500); digitalWrite(Voice, LOW); } if(val < waterset && watersafe == 1) { digitalWrite(Relay4, LOW); // sets Relay #4 on Serial.println("Soil Status:"); Serial.println(" "); //reservoir is full, soil is good Serial.println("Good"); Serial.println(" "); Serial.println("***********************************************"); Serial.println(" "); } if(val > waterset && watersafe == 0) { digitalWrite(Relay4, LOW); // sets Relay #4 on Serial.println("Soil Status:"); Serial.println(" "); Serial.println("Dry"); Serial.println(" "); Serial.println("Please Refill Water Reservoir"); Serial.println(" "); Serial.println("***********************************************"); //soil is dry, reservoir is empty Serial.println(" "); digitalWrite(Voice, LOW); delay(500); digitalWrite(Voice, LOW); } delay(Loop); //waits a designated amount of time and then runs again }
Step 4: Install and Run
After getting the code setup and downloaded onto your Aquabot, gather the following materials:
- A pitcher (or other container) full of water
- Tape
- A potted plant
Push the soil moisture sensor into the soil.
Place the pump into the water, with the tube running to the plant
Tape the liquid level sensor to the outside of the pitcher, 4 inches from the bottom. It acts as a safety switch so the we do not run the pump dry.
Connect the Arduino to your computer, you will hear a beep from the Aquabot.
Open the IDE file with the Aquabot code in it.
Configure the board and ports, then open the serial monitor (top right corner). The Aquabot will begin sending you data.
Connect the power supplies to the correct ports on the Aquabot and connect the to power.
At time intervals specified in the code, the Arduino will send another set of data. If the Aquabot beeps again, the reservoir is empty.
If your soil is dry, the Aquabot should run the pump.
Step 5: Making a Finished Product
Now its time to make the Aquabot a finished product.
The first thing to do is to take that waterproof container and draw circles on it that will mark the holes or "ports" for your Aquabot be sure to think about the layout of the Arduino when you do this, so that the wiring inside will be as neat as possible. Then you can drill the holes. Next, hot glue all the components into place inside the container, threading each component through its respective port. To ensure that we can still remove the Arduino later for maintenance or upgrades, hot glue the plastic base down, but not the Arduino itself.
Congratulations, your Aquabot is complete. Now it's time to close the lid, plug in, and put your Aquabot into action!
Step 6: Suggested Upgrades
Want to water more that one plant with your Aquabot? No problem! you just need the following materials:
- Wire, anything that will fit the Arduino will work, 20 gauge is suggested.
- More soil moisture sensors
- More water tubing (you can use flexible or PVC)
- 12v solenoid valves. These could be used to water one plant at a time
- the easiest way to use these would be to remove the pump, instead, drill a hole in the bottom of your reservoir and have a tube coming out. Split the tube to go to all of your plants, and put a solenoid (up to 4)on each branch. Hang your reservoir in the air and let gravity to the pumping for you!
Here is an additional list of suggested upgrades and improvements for the Aquabot:
- Bluetooth
- WiFi
- You could configure the Aquabot to publish data from it's sensors to a site or app like ThingSpeak, Blynk, Cayenee, etc
- You could configure the Aquabot to publish data from it's sensors to a site or app like ThingSpeak, Blynk, Cayenee, etc
- Additional Sensors
- More soil moisture sensors
- Temperature and Humidity
- Ambient light
- Ph
- More Functions
- Grow lights
- Window shade raise/lower
- Auto fertilize
- You could combine Aquabot with a project like this one to make a super-garden system.
Step 7: Usage and Calibration Tutorial.
Now that Auabot is all set up, here are some tips for operating and experimenting with Aquabot.
Calibration
To get your Aquabot to keep your plant at the perfect moisture level, it is important to have the correct settings. To get the moisture level mark you want, get a soil sample that is just below the ideal level of moisture and insert the soil sensor. View the value that is put out through the serial monitor, and change the variable "waterset" to equal this value in the code.
The other aspect of watering to change is how long the pump runs. Change the variable "runtime" in the code to however long you would like to pump to run each time it waters the plant. Remember 1000 = 1 second. You should give more or less time depending how much soil your plant is in. More soil means more time to water.
Using the Serial Plotter
The latest version of Arduino IDE comes with a great tool to use with Aquabot called the serial plotter. It creates graphs from numbers sent to to the serial monitor. You can find it under "Tools" in the IDE. This could be used if you want to compare plant growth to conditions detected by the Aquabot's sensors.
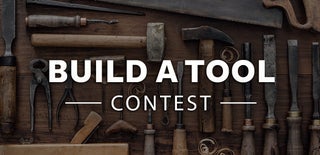
Participated in the
Build a Tool Contest