Introduction: Arduino 101 BLE Rover Remote Control
I bought an Arduino 101 for the Arduino compatibility combined with extra features like built-in Bluetooth Low Energy and the 6-axis accelerometer/gyroscope. I wanted to build an Arduino-based remote control rover with my Arduino 101 but struggled to find the sample code. I found numerous examples of RC rovers utilizing Bluetooth, but none for Bluetooth LE.
Breaking down the communications problem into small chunks, I needed to: 1. Figure out how to transmit a byte from my smartphone or tablet to the Arduino 101 and then 2. Determine how to create a workable remote control to send these bytes to the rover's state machine. I did solve both problems. I quickly discovered that i could modify the LED and CallbackLED samples that are included with the CurieBLE library to transmit a byte to the Arduino 101, but struggled to find a reliable, easy to use remote control. After a lot of internet searching and bit of work. I found a novel solution that is presented here.
Step 1: Sending Bytes to the Arduino 101 Over BLE
I found an answer to this basic communications challenge on the Arduino forum at: Arduino Forum> Products> Arduino 101. Here is the explanation.
In the Arduino 101 CurieBLE example called CallbackLED - an eventHandler is defined to be called when the switch characteristic is written. The code in setup() function looks like this:
// assign event handlers for characteristic
switchChar.setEventHandler(BLEWritten, switchCharacteristicWritten);
In the switchCharacteristicWritten event handler function, the code checks whether the value that was written is a zero (0x00) or something else. If non-zero, it turns the LED 'on' and if zero, it turns the LED 'off'. The code looks like this:
if (switchChar.value()) {
Serial.println("LED on");
digitalWrite(ledPin, HIGH);
else {
Serial.println("LED off");
digitalWrite(ledPin, LOW); }
to capture the byte that was written, it is only necessary to save it to a variable and then print it out on the serial monitor, the code would look like this:
if (switchChar.value()) {
Serial.println("LED on");
digitalWrite(ledPin, HIGH);
char state = switchChar.value();
Serial.print("new value written: ");
Serial.println(state); }
else {
Serial.println("LED off");
digitalWrite(ledPin, LOW); }
I verified this worked by writing the character via the nRF Connect application from Nordic Semiconductor, which is available in both the Play Store and the App Store.
Here are the detailed steps after installing the application:
1. Run the nRF Connect application
2. Run the scanner by pressing 'Scan' in the upper right corner - it usually runs automatically
3. Select your named service (in the sample it is named "LEDCB").
4. Press 'Connect' on the right.
5. Select the Unknown Service (last on the list for your service).
6. Press the up arrow on the right. This brings up a write value dialog box.
7. Press 'New value', enter a byte value in hex like 61 - which is an "a" in ASCII text.
8. Press 'Send' in the lower right and your byte is sent.
Following these steps, we are successfully writing bytes over Bluetooth LE to the Arduino 101.
Step 2: Creating the Bluetooth LE Remote Control
I like the nRF Connect application, but there was no way that I could find to make a workable remote. Entering bytes manually via the nRF Connect application was not going to allow me to control the rover efficiently. To try out other Arduino 101 samples, I had downloaded another nRF application called the nRF Toolbox. In the toolbox, the last application was titled "UART". The UART app has nine user definable buttons that can be configured to send a command on a button press. Pressing 'Edit' in the upper right corner makes all the buttons orange. Touching a button brings up a dialog box to associate an icon to the button and define the command to send when pressed.
I have an Android phone and the available icons match my remote control perfectly. On an IOS device, the icons are not quite as ideal for this usage, but pick ones that you can remember. My rover's motion control is based on a simple one byte code that uses the following configuration (I used the control sequence that was used in Deba168's Instructable for a "Smartphone Controlled Arduino Rover". Here is the list of commands and corresponding characters:
a - forward
b - left
c - stop
d - right
e - reverse
1 - 25% of motor power
2 - 50% of motor power
3 - 75% of motor power
4 - 100% of motor power
When I created the remote control the first time I entered the ASCII value of the character - this did not work. The correct command is the letter/number itself, not its ASCII value. When I entered all nine icons and commands, I pressed 'Done". My finished remote looked like the picture.
Simple, right? Well not so fast. When I tried to connect my Arduino 101 BLE service with the CallbackLED switch characteristic to the UART app, it complained that "The device does not have the required services". I did an internet search on Nordic and UART. I found that the UART application expects to see very specific service UUIDs and characteristics. Using the information at: https://www.nordicsemi.com/eng/Products/Nordic-mob.... I integrated the correct service name, UUID, and the Tx and Rx characteristics into my Arduino sketch, then I made a couple of adjustments to account for the fact that the service can transmit more than one character and therefore provides a pointer to the first element of an array and it works.
Step 3: Testing the Remote Control
The Sketch will send the transmitted byte to the Serial Monitor to verify that each button on the UART application sends the desired byte.
In order to use the remote, follow these steps:
1. Open the nRF Toolbox
2. Select the UART application
3. press the 'Connect' button and then select the name of your BLE service - "BLE_ROV".
The button should now read 'Disconnect'. You are now connected and each button press will transmit the command for that button.
NOTE: In testing this sketch with older IOS phones, I found that my advertised local name of "BLE_ROV" did not show up in the list to connect to. Instead I saw something like, 'Arduino 101-xxxx' where xxxx is the last 4 hex digits of the Mac address for the BLE chip - shown on a small sticker on the back of the Arduino 101 board. Simply select this name to connect to and everything should work.
This was the simplest solution I could find. The Arduino sketch is included for download as well as an image of the serial monitor when you press each key on the remote and then disconnect.
I hope this is useful. I will provide the full instructions for the full rover I built at a later date.
Attachments
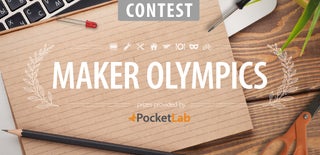
Participated in the
Maker Olympics Contest 2016