Introduction: Arduino Base Pick and Place Robot
I have made a super cheap (less than 1000 dollars) industrial robot arm to enable students to hack larger scale robotics and to enable small local productions to use robots in their processes without breaking the bank. Its Eassy to build and make the age group people of 15 to 50 years.
Step 1: Components Requirement
1. Arduino
+ Shield + Pins + Cables
2. Motorcontroller: dm860A (Ebay)
3. Steppermotor: 34hs5435c-37b2 (Ebay)
4. M8x45+60+70 bolts and M8 bolts.
5. 12mm Plywood.
6. 5mm Nylon .
7. Blind washers 8mm.
8. Wood Screws 4.5x40mm.
9. M3 Counter sunk,
10. 12v power supply
11. servo motor driver arduino
Step 2: Download Gui
http://zapmaker.org/projects/grbl-controller-3-0/
https://github.com/grbl/grbl/wiki/Using-Grbl
Step 3: Connection
Connect the wires which are given in the image is greater understanding for you.
we need to connect the motor driver to Arduino and other connectors which required as per your robot.
Step 4: Upload Firmware and Check the Code Result in Arduino Dashboard
Installing the firmware on Arduino - GRBL:
https://github.com/grbl/grbl/wiki/Compiling-Grbl
Note: You may get a conflict when compiling in Arduino. Remove all other libraries from your library folder (../documents/Arduino/libraries).
Firmware setup
Set enable to newer timeout. Use a serial connection and write:
$1=255
Set homing:
$22=1
Remember to set serial to baud: 115200
Useful G-codes
Set zero point for robot:
G10 L2 Xnnn Ynnn Znnn
Use zero point:
G54
Typical initialization to center robot:
G10 L2 X1.5 Y1.2 Z1.1
G54
Move robot to position fast:
G0 Xnnn Ynnn Znnn
Example:
G0 X10.0 Y3.1 Z4.2 (return)
Move robot to position at specific speed:
G1 Xnnn Ynnn Znnn Fnnn
G1 X11 Y3 Z4 F300 (return)
F should be between 10(slooooow) and 600(fast)
Default units for X,Y and Z
When using default step/units settings (250 step/unit) for GRBL and
stepper drive set up for 800 step/rev the following units apply for all axis:
+- 32 units = +- 180 degrees
Processing code example:
This code can communicate directly with the Arduino GRBL.
https://github.com/damellis/gctrl
Remember to set serial to baud: 115200
Code uoload in ardunio
import java.awt.event.KeyEvent;
import javax.swing.JOptionPane;
import processing.serial.*;
Serial port = null;
// select and modify the appropriate line for your operating system
// leave as null to use interactive port (press 'p' in the program)
String portname = null;
//String portname = Serial.list()[0]; // Mac OS X
//String portname = "/dev/ttyUSB0"; // Linux
//String portname = "COM6"; // Windows
boolean streaming = false;
float speed = 0.001;
String[] gcode;
int i = 0;
void openSerialPort()
{
if (portname == null) return;
if (port != null) port.stop();
port = new Serial(this, portname, 115200);
port.bufferUntil('\n');
}
void selectSerialPort()
{
String result = (String) JOptionPane.showInputDialog(this,
"Select the serial port that corresponds to your Arduino board.",
"Select serial port",
JOptionPane.PLAIN_MESSAGE,
null,
Serial.list(),
0);
if (result != null) {
portname = result;
openSerialPort();
}
}
void setup()
{
size(500, 250);
openSerialPort();
}
void draw()
{
background(0);
fill(255);
int y = 24, dy = 12;
text("INSTRUCTIONS", 12, y); y += dy;
text("p: select serial port", 12, y); y += dy;
text("1: set speed to 0.001 inches (1 mil) per jog", 12, y); y += dy;
text("2: set speed to 0.010 inches (10 mil) per jog", 12, y); y += dy;
text("3: set speed to 0.100 inches (100 mil) per jog", 12, y); y += dy;
text("arrow keys: jog in x-y plane", 12, y); y += dy;
text("page up & page down: jog in z axis", 12, y); y += dy;
text("$: display grbl settings", 12, y); y+= dy;
text("h: go home", 12, y); y += dy;
text("0: zero machine (set home to the current location)", 12, y); y += dy;
text("g: stream a g-code file", 12, y); y += dy;
text("x: stop streaming g-code (this is NOT immediate)", 12, y); y += dy;
y = height - dy;
text("current jog speed: " + speed + " inches per step", 12, y); y -= dy;
text("current serial port: " + portname, 12, y); y -= dy;
}
void keyPressed()
{
if (key == '1') speed = 0.001;
if (key == '2') speed = 0.01;
if (key == '3') speed = 0.1;
if (!streaming) {
if (keyCode == LEFT) port.write("G91\nG20\nG00 X-" + speed + " Y0.000 Z0.000\n");
if (keyCode == RIGHT) port.write("G91\nG20\nG00 X" + speed + " Y0.000 Z0.000\n");
if (keyCode == UP) port.write("G91\nG20\nG00 X0.000 Y" + speed + " Z0.000\n");
if (keyCode == DOWN) port.write("G91\nG20\nG00 X0.000 Y-" + speed + " Z0.000\n");
if (keyCode == KeyEvent.VK_PAGE_UP) port.write("G91\nG20\nG00 X0.000 Y0.000 Z" + speed + "\n");
if (keyCode == KeyEvent.VK_PAGE_DOWN) port.write("G91\nG20\nG00 X0.000 Y0.000 Z-" + speed + "\n");
//if (key == 'h') port.write("G90\nG20\nG00 X0.000 Y0.000 Z0.000\n");
if (key == 'v') port.write("$0=75\n$1=74\n$2=75\n");
//if (key == 'v') port.write("$0=100\n$1=74\n$2=75\n");
if (key == 's') port.write("$3=10\n");
if (key == 'e') port.write("$16=1\n");
if (key == 'd') port.write("$16=0\n");
if (key == '0') openSerialPort();
if (key == 'p') selectSerialPort();
if (key == '$') port.write("$$\n");
if (key == 'h') port.write("$H\n");
}
if (!streaming && key == 'g') {
gcode = null; i = 0;
File file = null;
println("Loading file...");
selectInput("Select a file to process:", "fileSelected", file);
}
if (key == 'x') streaming = false;
}
void fileSelected(File selection) {
if (selection == null) {
println("Window was closed or the user hit cancel.");
} else {
println("User selected " + selection.getAbsolutePath());
gcode = loadStrings(selection.getAbsolutePath());
if (gcode == null) return;
streaming = true;
stream();
}
}
void stream()
{
if (!streaming) return;
while (true) {
if (i == gcode.length) {
streaming = false;
return;
}
if (gcode[i].trim().length() == 0) i++;
else break;
}
println(gcode[i]);
port.write(gcode[i] + '\n');
i++;
}
void serialEvent(Serial p)
{
String s = p.readStringUntil('\n');
println(s.trim());
if (s.trim().startsWith("ok")) stream();
if (s.trim().startsWith("error")) stream(); // XXX: really?
}
Step 5: Design and Print All Part in Plywood Sheet
Download the robot part and design in AutoCAD and print the on the 12mm plywood sheet and finish and design portion. If anyone needs cad file plz leave the comment in comment section box I will `you send directly.
Step 6: Assembly
collect all the part and arrange in the sequence on image which is given and follow the image diagram.
Step 7: Setup GBRL Settings
Setting that has proven to work on our robots.
$0=10 (step pulse, usec) $1=255 (step idle delay, msec) $2=7 (step port invert mask:00000111) $3=7 (dir port invert mask:00000111) $4=0 (step enable invert, bool) $5=0 (limit pins invert, bool) $6=1 (probe pin invert, bool) $10=3 (status report mask:00000011) $11=0.020 (junction deviation, mm) $12=0.002 (arc tolerance, mm) $13=0 (report inches, bool) $20=0 (soft limits, bool) $21=0 (hard limits, bool) $22=1 (homing cycle, bool) $23=0 (homing dir invert mask:00000000) $24=100.000 (homing feed, mm/min) $25=500.000 (homing seek, mm/min) $26=250 (homing debounce, msec) $27=1.000 (homing pull-off, mm) $100=250.000 (x, step/mm) $101=250.000 (y, step/mm) $102=250.000 (z, step/mm) $110=500.000 (x max rate, mm/min) $111=500.000 (y max rate, mm/min) $112=500.000 (z max rate, mm/min) $120=10.000 (x accel, mm/sec^2) $121=10.000 (y accel, mm/sec^2) $122=10.000 (z accel, mm/sec^2) $130=200.000 (x max travel, mm) $131=200.000 (y max travel, mm) $132=200.000 (z max travel, mm)
Step 8: Upload the Final Code and Check the Virtual Result in Arduino Uno Software Dashboard
// Units: CM
float b_height = 0;
float a1 = 92;
float a2 = 86;
float snude_len = 20;
boolean doZ = false;
float base_angle;// = 0;
float arm1_angle; // = 0;
float arm2_angle; //= 0;
float bx = 60;// = 25;
float by = 60;// = 0;
float bz = 60;// = 25;
float x = 60;
float y = 60;
float z = 60;
float q;
float c;
float V1;
float V2;
float V3;
float V4;
float V5;
void setup() {
size(700, 700, P3D);
cam = new PeasyCam(this, 300);
cam.setMinimumDistance(50);
cam.setMaximumDistance(500);
}
void draw() {
//ligninger:
y = (mouseX - width/2)*(-1);
x = (mouseY - height/2)*(-1);
bz = z;
by = y;
bx = x;
float y3 = sqrt(bx*bx+by*by);
c = sqrt(y3*y3 + bz*bz);
V1 = acos((a2*a2+a1*a1-c*c)/(2*a2*a1));
V2 = acos((c*c+a1*a1-a2*a2)/(2*c*a1));
V3 = acos((y3*y3+c*c-bz*bz)/(2*y3*c));
q = V2 + V3;
arm1_angle = q;
V4 = radians(90.0) - q;
V5 = radians(180) - V4 - radians(90);
arm2_angle = radians(180.0) - (V5 + V1);
base_angle = degrees(atan2(bx,by));
arm1_angle=degrees(arm1_angle);
arm2_angle=degrees(arm2_angle);
//println(by, bz);
//arm1_angle = 90;
//arm2_angle = 45;
/*
arm2_angle = 23;
arm1_angle= 23;
arm2_angle=23;
*/
// interactive:
// if (doZ)
//
// {
// base_angle = base_angle+ mouseX-pmouseX;
// } else
// {
// arm1_angle =arm1_angle+ pmouseX-mouseX;
// }
//
// arm2_angle = arm2_angle+ mouseY-pmouseY;
draw_robot(base_angle, -(arm1_angle-90), arm2_angle+90 - ( -(arm1_angle-90)));
// println(base_angle + ", " + arm1_angle + ", " + arm2_angle);
}
void draw_robot(float base_angle, float arm1_angle, float arm2_angle)
{
rotateX(1.2);
rotateZ(-1.2);
background(0);
lights();
pushMatrix();
// BASE
fill(150, 150, 150);
box_corner(50, 50, b_height, 0);
rotate(radians(base_angle), 0, 0, 1);
// ARM 1
fill(150, 0, 150);
box_corner(10, 10, a1, arm1_angle);
// ARM 2
fill(255, 0, 0);
box_corner(10, 10, a2, arm2_angle);
// SNUDE
fill(255, 150, 0);
box_corner(10, 10, snude_len, -arm1_angle-arm2_angle+90);
popMatrix();
pushMatrix();
float action_box_size=100;
translate(0, -action_box_size/2, action_box_size/2+b_height);
pushMatrix();
translate(x,action_box_size- y-action_box_size/2, z-action_box_size/2);
fill(255,255,0);
box(20);
popMatrix();
fill(255, 255, 255, 50);
box(action_box_size, action_box_size, action_box_size);
popMatrix();
}
void box_corner(float w, float h, float d, float rotate)
{
rotate(radians(rotate), 1, 0, 0);
translate(0, 0, d/2);
box(w, h, d);
translate(0, 0, d/2);
}
void keyPressed()
{
if (key == 'z')
{
doZ = !doZ;
}
if (key == 'h')
{
// set all to zero
arm2_angle = 0;
arm1_angle = 90;
base_angle = 0;
}
if (key == 'g')
{
println(degrees(V1));
println(degrees(V5));
}
if (keyCode == UP)
{
z ++;
}
if (keyCode == DOWN)
{
z --;
}
if (key == 'o')
{
y = 50;
z = 50;
println(q);
println(c, "c");
println(V1, "V1");
println(V2);
println(V3);
println(arm1_angle);
println(V4);
println(V5);
println(arm2_angle);
}
}
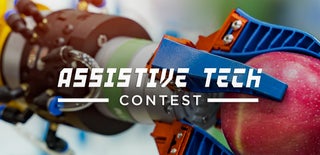
Participated in the
Assistive Tech Contest