Introduction: Arduino Box Controlled by TV Remote and CODE
This instructable show you how I have created a box controlled remote by a TV infrared transmitter. I have taken inspiration by this instructable Control Any Circuit With a TV Remote (and an Arduino). In this link you can discover library lets you decode the signal coming from your remote and instruction to download and install the library zip file. The aim of this project is recycle of old components and turn these in electronic reusable modules.
CODE (incomplete see .ino file attached)
#include .......
#include ........
#define MAX_TIME 150 #define LED_PIN_yellow 3 #define LED_PIN_green 4 #define LED_PIN_red 5 #define LED_PIN_blue 6 #define USB_RELAY 9 #define LAMP_RELAY 10
IRrecv irrecv(11); decode_results results; long lastPressTime = 0; int state = LOW; int blink_register = 0; int blink_fast_register = 0;
void setup() { pinMode(LED_PIN_red, OUTPUT); pinMode(LED_PIN_green, OUTPUT); pinMode(LED_PIN_yellow, OUTPUT); pinMode(LED_PIN_blue, OUTPUT); pinMode(USB_RELAY, OUTPUT); pinMode(LAMP_RELAY, OUTPUT); irrecv.enableIRIn(); // Start the receiver }
void loop() { if (irrecv.decode(&results)) { // -------------------------------------------------------- // yellow leds if (results.value == 0x443038C7) { if (millis() - lastPressTime > MAX_TIME) { // It's been a while since the last press, so this // must be a new press. // Toggle the state state = 1 - state; (blink_register = 0); (blink_fast_register = 0); digitalWrite(LED_PIN_yellow, state); } lastPressTime = millis(); } irrecv.resume(); // Receive the next value // -------------------------------------------------------- // green leds if (results.value == 0x4430D827) { if (millis() - lastPressTime > MAX_TIME) { // It's been a while since the last press, so this // must be a new press. // Toggle the state state = 1 - state; (blink_register = 0); (blink_fast_register = 0); digitalWrite(LED_PIN_green, state); } lastPressTime = millis(); } irrecv.resume(); // Receive the next value // -------------------------------------------------------- // red leds if (results.value == 0x443058A7) { if (millis() - lastPressTime > MAX_TIME) { // It's been a while since the last press, so this // must be a new press. // Toggle the state state = 1 - state; (blink_register = 0); (blink_fast_register = 0); digitalWrite(LED_PIN_red, state); } lastPressTime = millis(); } irrecv.resume(); // Receive the next value // -------------------------------------------------------- // blue leds if (results.value == 0x443002FD) { if (millis() - lastPressTime > MAX_TIME) { // It's been a while since the last press, so this // must be a new press. // Toggle the state state = 1 - state; (blink_register = 0); (blink_fast_register = 0); digitalWrite(LED_PIN_blue, state); } lastPressTime = millis(); } irrecv.resume(); // Receive the next value // -------------------------------------------------------- // USB_RELAY // BUTTON 4 if (results.value == 0x443020DF) { if (millis() - lastPressTime > MAX_TIME) { // It's been a while since the last press, so this // must be a new press. // Toggle the state state = 1 - state; (blink_register = 0); (blink_fast_register = 0); digitalWrite(USB_RELAY, state); } lastPressTime = millis(); } irrecv.resume(); // Receive the next value // -------------------------------------------------------- // LAMP_RELAY // BUTTON OK if (results.value == 0x44308877) { if (millis() - lastPressTime > MAX_TIME) { // It's been a while since the last press, so this // must be a new press. // Toggle the state state = 1 - state; (blink_register = 0); (blink_fast_register = 0); digitalWrite(LAMP_RELAY, state); } lastPressTime = millis(); } irrecv.resume(); // Receive the next value // -------------------------------------------------------- // all leds // button 1 if (results.value == 0x4430807F) { if (millis() - lastPressTime > MAX_TIME) { // It's been a while since the last press, so this // must be a new press. // Toggle the state state = 1 - state; (blink_register = 0); (blink_fast_register = 0); digitalWrite(LED_PIN_red, state); digitalWrite(LED_PIN_blue, state); digitalWrite(LED_PIN_yellow, state); digitalWrite(LED_PIN_green, state); } lastPressTime = millis(); } irrecv.resume(); // Receive the next value } // -------------------------------------------------------- // blink all leds // button 2 if (results.value == 0x443040BF) { (blink_register = 1); (blink_fast_register = 0); } if (blink_register == 1) { digitalWrite(LED_PIN_red, HIGH); digitalWrite(LED_PIN_yellow, HIGH); digitalWrite(LED_PIN_green, HIGH); digitalWrite(LED_PIN_blue, HIGH); delay(1000); digitalWrite(LED_PIN_red, LOW); digitalWrite(LED_PIN_yellow, LOW); digitalWrite(LED_PIN_green, LOW); digitalWrite(LED_PIN_blue, LOW); delay(1000); } // -------------------------------------------------------- // fast blink all leds // button 3 if (results.value == 0x4430C03F) { (blink_fast_register = 1); (blink_register = 0); } if (blink_fast_register == 1) { digitalWrite(LED_PIN_red, HIGH); digitalWrite(LED_PIN_yellow, HIGH); digitalWrite(LED_PIN_green, HIGH); digitalWrite(LED_PIN_blue, HIGH); delay(200); digitalWrite(LED_PIN_red, LOW); digitalWrite(LED_PIN_yellow, LOW); digitalWrite(LED_PIN_green, LOW); digitalWrite(LED_PIN_blue, LOW); delay(200); } }
Attachments
Step 1: Led Module
Led module for simple light games. In this sketch you can choose:
- on/off only blue leds
- on/off only red leds
- on/off only green leds
- on/off only yellow leds
- on/off only all leds
- slow blinking all leds
- fast blinking all leds
You can change sketch for more leds light game
Attachments
Step 2: Power Supply Unit
The power supply unit is formed by:
- one old 12v battery pack
- toggle switch module
- L7805CV module
12v battery is necessary for supply two rele modules.
The switch module is a simple toggle switch and two appropriate connectors.
The L7805CV module scheme is taken here: http://code.alessioluffarelli.it/37-alimentazione-circuito-5v-con-7805/
Step 3: IR Sensor TSOP1138
This module is ispired by data sheet of TSOP1138 sensor
Step 4: Actuators: Two Rele Modules
Two rele modules:
- one for supply female cable usb (usb device: ligth, fan, ...)
- one for 220v low power devices (ambient light)
The rele modules scheme is here: https://www.instructables.com/id/Domotic-arduino/
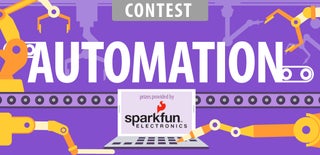
Participated in the
Automation Contest 2016