Introduction: Arduino ESP32 Color E-Paper Weather Station
This is a project I've wanted to build for over a decade now. I've finally made it and I am really excited– a battery-powered weather station project. I've built many weather station projects in the past, but this one is different. It can last on batteries for months. The reason for this is the amazing E-Paper display it uses. Let me show you how I built this project and how you can build your own using the same or a similar display.
First of all, let me explain what this project is and how it works.
As you can see, we have a large, 6-inch E-Paper display that can display 7 colors combined with an ESP32 microprocessor. Among all the e-paper boards I've used in the past six years, this is the best.
On the display, we show the temperature, humidity, barometric pressure, and weather forecast. We get the temperature, humidity, and barometric pressure from two sensors, and the weather forecast from the internet.
We also display some graphs here. A line graph that shows the temperature readings of the last 24 hours, a bar graph that shows the humidity readings of the last 24 hours, and a bar graph that shows the barometric pressure readings of the last 24 hours.
The last bar graph is the most useful of them all, in my opinion. We can take these values and, using a specific algorithm, the Zambretti algorithm, generate our own weather forecast. But that's a topic for another Instructable.
Supplies
- E-Paper Display Board: https://educ8s.tv/part/ColorEPaper
- DHT22 Sensor: https://educ8s.tv/part/DHT22
- BMP180 Sensor: https://educ8s.tv/part/BMP18
OPTIONAL
- BME280 Sensor: https://educ8s.tv/part/BME280
Step 1: Inkplate6 Color E-Paper Display
This is a 6” color E-Paper display board. I have been making things for over a decade now, and I am fascinated by e-ink technology and its uses. If you are not familiar with it, an e-ink display uses electricity only when it updates its contents, so even though this device is now off, it still displays this image. I have built many projects in this channel using an e-ink display. I always wanted an all in one board. A board that will contain everything I would ever need to build a project and that it will be easy to work with. So, let’s see what this board offers.
First of all this board uses a big Color E-Ink display. It is the first time I use a color E-Ink display and I love it. Color adds so much to any project. This display can display 7 different colors. White, Black, Red, Yellow, Blue, Green and Orange. It offers a resolution of 600x448 pixels and the refresh rate of the screen is about 11 seconds. Since the display uses the E-Ink technology, it only consumes power when it refreshes its contents, so if we don’t update the display all the time we have almost zero power consumption. This board can achieve a deep sleep current of 18μΑs. I like it, we can build projects which can last on battery for months. Amazing stuff.
The board also features an ESP32 microcontroller with integrated Wi-Fi and Bluetooth connectivity. We can also find an SD card slot where we can store files and images to display on the screen. The board can be programmed and powered by a USB-C connector, and it features a battery charger and a Lipo Battery. Also, the board comes with a RTC clock module to keep track of time and reduce power consumption. As you can see, the board also gives us many gpio pins to connect our sensors and devices. The board also comes with a 3D printed enclosure, and the files are also available online if you want to modify them. What else could a maker ask for? Everything we want from hardware is here.
Step 2: DHT22 Sensor
The DHT22 is a very popular Temperature and Humidity sensor. It is cheap, easy to use and the specification claims good precision and accuracy.
The DHT sensors are made of two parts, a capacitive humidity sensor and a thermistor. There is also a chip inside that does some analog to digital conversion and outputs a digital signal with the temperature and humidity. The digital signal is fairly easy to read using any microcontroller.
Characteristics of the DHT22
- Low cost
- 3 to 5V power and I/O
- 2.5mA max current use during conversion
- 0-100% humidity readings with 2-5% accuracy
- -40 to 125°C temperature readings ±0.5°C accuracy
- Slow
The connection with Arduino is extremelly easy. We connect the sensor pin with the + sign to the 5V or the 3.3V output of the Arduino. We connect the sensor pin with the - sign to GROUND. Lastly we connect the OUT pin to any digital pin of the Arduino.
In order to use the DHT22 sensor with Arduino we have to use the DHT library.
Attachments
Step 3: BMP180 Sensor
The BMP180 is a low-cost, low-power, digital barometric pressure sensor that communicates with Arduino via I2C. It is commonly used for measuring atmospheric pressure, temperature, and altitude. With its high precision and wide measuring range, it is a valuable tool for various applications, such as weather monitoring, altitude tracking in drones and other vehicles, and climate data collection. The BMP180's small size and ease of integration make it a popular choice for Arduino projects.
- Sensor Type: Digital barometric pressure sensor
- Communication: Protocol I2C
- Measuring Range: Atmospheric pressure: 300 to 1100 hPa (9000 meters to -500 meters above sea level)
- Temperature Measurement: Range-40°C to 85°C (-40°F to 185°F)
- Accuracy: Pressure: ±0.2 hPa (0.75 millibar); Temperature: ±1.0°C (2°F)
- Resolution: Pressure: 0.01 hPa (0.03 millibar); Temperature: 0.01°C (0.02°F)
- Power ConsumptionTypical: 0.3 µA; Maximum: 2.5 µAD
Step 4: Connections
The connection is really easy. I soldered some header pins to the board and connected the sensors to them.
The BMP180 sensor uses the I2C interface so we have to connect power and two more wires to SCL and SDA pins of the board.
The DHT22 sensor needs power and a single wire which I have connected to pin IO13.
Step 5: Code & Libraries
In order for the project to compile we need 4 libraries, the Inkplate library for the display, a library for the BMP180 sensor, a library for the DHT22 sensor and the ArduinoJson library to parse the data we get from the internet.
Libraries
- Inkplate6 Library
- DHT22 Library
- BMP180 Library
- Arduino JSON
You can download all the libraries from the Arduino IDE directly so you don't need to visit any websites.
The software of this project consists of many files. I decided to use Object Oriented Programming to make this project easier to work with. Each functionality of the project has its own Class. So we have a Class that retrieves the weather forecast. Then we have a class that reads the sensors. Then we have a Class that is responsible for reading and writing data to the EEPROM memory. Another class we need is a class that holds all the historical sensor data and finally a class that is responsible for drawing on the display. This way, if you want to use a different display you only need to write your own Display class. If you want to use different sensors, let say the BMP280 you only need to modify the sensors class. All the other code will work just fine.
You can find a link to the code here: https://educ8s.tv/arduino-e-paper-weather-station/
Or in this GitHub repository: https://github.com/educ8s/Arduino-Color-E-Paper-Weather-Station
It is completely open source, and you can expand it with other functionality if you wish. I would love to see what you are going to build with this code as a starting point .
Step 6: Running the Code for the First Time
Before running the project for the first time it is a good idea to run the clean_eeprom program once. You can find the code inside the .zip file I shared in the previous step, or in the github repository. This small program will initialise the values of the EEPROM memory we are going to use to zero and it will erase any data that was stored there previously. This is needed because we read the EEPROM memory every time we wake the chip up, and if the EEPROM is not reset, we will encounter issues.
Now, in the project code we need to enter 4 values. First of all we need to enter the SSID and the password of our WiFi network so the board can connect to the internet to retrieve the weather forecast. Now to retrieve the weather forecast we will use the Openweathermap free api. To use it, we have to create an account. When we create an account we will get access to a personal API key. We need to enter the key in the APIKEY variable. The last thing we have to enter is the ID of the city we want to get the weather forecast. Just search for your city, and note down the ID that appears at the end of the URL. You need to enter this value in this variable. That’s it. Our code is ready to be uploaded to the board.
The device now displays all the data gathered from the sensors and the weather forecast. However, the graphs are not yet visible as they lack historical data. It will take 24 hours for the graphs to be properly drawn.
Step 7: How the Code Works
The project operates by taking temperature, humidity, and pressure readings every six minutes. It then enters deep sleep mode, where the device is nearly completely disabled, consuming only 15µA of current. The display, even though it is inactive, is still displaying the previously recorded readings, which is the main reason I chose it.
Since deep sleep mode disables all device memory to conserve power, any data stored in RAM is lost. Therefore, before entering sleep mode, I save all readings to the device's EEPROM memory, a permanent storage solution. Upon waking, the device retrieves all readings from the EEPROM and updates them.
Every 10 wake-up cycles, or 60 minutes, the device obtains temperature, humidity, and pressure readings and updates the graph accordingly. Every 60 wake-up cycles, or 360 minutes (6 hours), the device connects to the internet to retrieve the weather forecast. This process is limited to four times a day to conserve power, as WiFi connectivity consumes a significant amount of energy.
By implementing these power-saving strategies, the battery life can extend for months. I'm currently using a 1200mAh battery, and I'll keep you updated on its longevity. The project has been running reliably for the past 3 days, I couldn’t wait 6 months to share this project with you.
Step 8: Enclosing the Project
To enhance the appearance of the device, it's time to enclose it. I'm using the original enclosure that comes with the board, but you can design and 3D print your own if you prefer. I simply made a hole for the sensors to protrude from the enclosure, enabling them to collect data effectively. It looks so cool on my desk, I can’t stop staring at it.
Step 9: Conclusion
I hope you like this project. It is one of my all time favourites. I always wanted an e-paper weather station that would look like this. I can’t buy it because nobody makes such a device commercially. So, I built my own. We are lucky that we live in an age where we can make anything we want by ourselves in just a few days. We are also lucky because with the help of the internet we can find all the knowledge required and get in touch with like minded people. So, if you like what I do on this channel and want to follow me on my journey follow me on Instructables and subscribe to my YouTube channel and you will be notified when I create a new project.
Thanks for reading this Instructable.
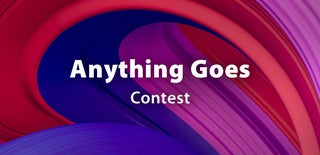
Runner Up in the
Anything Goes Contest