Introduction: Arduino High Tech Safe
This is my arduino high tech safe. You have to scan your finger, scan your card, then enter a 4 digit password to unlock the drawer. This project is not recommended for beginners because it is very advanced. The code is long, but I will share it in this project. The cost is around $75 if you build the same safe as me. This project also could take 1-3 days to complete. So, lets get started!
Supplies
To build the same safe as mine you will need:
Arduino (Uno recommended because I do not have steps for arduino mega or nano. But you can still use them)
breadboard
servo
jumper wires
joystick
20*4 (you can use 16*4, but you will have to change the code)
fingerprint scanner
mfrc522 rfid card scanner
a bucket of legos
Step 1: Building a Box
First you will need a box to put your electronics in. You could use legos, a 3d printed box, or even cardboard! (Though this might be harder) Don't forget to put holes in it for a drawer, and your electronics. If you are using the rfid card scanner, you do not need to put a hole for that if your walls are thin. The cards still works, but you have to put the keychains close so the rfid card senor can read them. Also leave room for your arduino and wires inside. NOTE: When you build the drawer, leave a hole in it so your servo can turn and lock the drawer.
Step 2: Wiring Up!
This step can be complicated because you need to plug wires in the exact spot or the electronics won't work. I do not have a wiring diagram, but I will tell you will each one goes. The wiring is for arduino uno only. You will have to search for were to put the wires if you are using a arduino mega or nano. If you are not using all the electronis I have, you can just skip that wiring.
Finger print scanner: green wire: D2 white wire: D3 black wire: GND red wire: 5V
Joystick: 5V = 3.3V GND = GND X = A2 Y = A3 Switch = D4
rfid card scanner: 3.3V = 3.3V rst = D9 GND = GND miso = D12 mosi = D11 sck = D13 sda = D10
lcd screen: 5V = 5V GND = GND sda = A4 sck = A5
Servo: red wire: 5V Brown wire: GND yellow wire: D6
NOTE: DO NOT PLUG RFID CARD SCANNER INTO 5V. IF YOU DO, IT WILL BREAK!!!
You may be wondering why I said to plug the joystick power to 3.3V when it says 5V on the side. That's because the servo, screen, and fingerprint scanner need 5V. If you add the joystick to that, the electronics might not work because everything needs 5V. The joystick will still work with 3.3V. It's just the max value won't 1023, it will be ~670.
Step 3: Downloading Libraries
In this project, you will need 6 libraries to use all the electronics. 1 for the servo, 1 for the fingerprint sensor, 2 for rfid card scanner, and 2 for the screen. The joystick does not need a library. Now, what is a library? It's basically a file that holds lots of code that you can use in your project with simple commands. To get these libraries, you need to go to a place called GitHub. If you know how to download and unzip libraries, just go to the links below for the downloads. If you do not know how, you can go to my instructable which talks about how to download a library in step 3: https://www.instructables.com/id/Makey-Makeyarduin...
or search a youtube video on how to download a arduino library from GitHub
LINKS TO LIRARIES:
Servo https://github.com/arduino-libraries/Servo
fingerprint sensor: https://github.com/adafruit/Adafruit-Fingerprint-S...
spi https://github.com/PaulStoffregen/SPI
rfid card sensor https://github.com/miguelbalboa/rfid
screen library 1 https://github.com/esp8266/Arduino/blob/master/lib...
screen library 2 https://github.com/fdebrabander/Arduino-LiquidCrys...
Step 4: Setting Up Fingerprint Sensor and Rfid Card Sensor
This step talks about how to set up fingerprint sensor and rfid card sensor. Unless you have already used your fingerprint sensor, you will need to show it what your fingerprint looks like so it can save it in its memory. Even if you have used it before, you should still probably do this step. I will tell you how to do it briefly, but if it doesn't make sense go to the same instructable link in the last step. It goes over it very well. Basic just open arduino ide. Go to file > examples > adafruit fingerprint sensor > enroll. Upload code to arduino, open serial monitor, and follow the steps it tells you. When it tells you to give it a number. Type in 1#.
Now for the rfid card, this step is pretty easy. Open arduino ide. Go to file > examples > mfrc522 > read personal data. Upload to arduino and open serial monitor. Scan the card or keychain you want to use that came with your sensor. Then it will give you some information. Look for the ID of the card it will be 4 sets of 2 digits. Something like this: AB 45 2Y 45 but your code will be different. Write this down on a piece of paper. You will need it again later. That's it for this step.
Step 5: THE CODE!
This is going to be the hardest step to most of you, but it is pretty simple. First copy and paste the code into the arduino ide from below. Second, edit the 2 sections where says EDIT CODE. 1 section is for the card scanner, 1 is for the joystick. These steps cannot be skipped. Once done, upload code to arduino!
#include
#include Fingerprint.h>
#include
#include
#include
#include < LiquidCrystal_I2C.h>
LiquidCrystal_I2C lcd(0x27, 2, 1, 0, 4, 5, 6, 7, 3, POSITIVE);
#define RST_PIN 9
#define SS_PIN 10
MFRC522 mfrc522(SS_PIN, RST_PIN);
SoftwareSerial mySerial(2, 3);
Adafruit_Fingerprint finger = Adafruit_Fingerprint(&mySerial);
Servo servo;
char d1;
char d2;
char d3;
char d4;
void setup(){
servo.attach(6);
servo.write(170);
lcd.begin(20,4);
Serial.begin(9600);
while (!Serial);
SPI.begin();
mfrc522.PCD_Init();
delay(4);
mfrc522.PCD_DumpVersionToSerial();
delay(100);
Serial.println("\n\nAdafruit finger detect test");
finger.begin(57600);
delay(5);
if (finger.verifyPassword()) {
Serial.println("All systems working,");
lcd.clear();
lcd.setCursor(1,0);
lcd.print("Please scan finger");
} else {
Serial.println("ERROR: Finger print sensor not found!");
lcd.setCursor(0,0);
lcd.print("ERROR: Finger print");
lcd.setCursor(1,1);
lcd.print("sensor not found!");
while (1) { delay(1); }
}
finger.getTemplateCount();
Serial.print("Sensor contains "); Serial.print(finger.templateCount); Serial.println(" templates"); Serial.println("Waiting for valid finger..."); }
void(* resetFunc) (void) = 0;
void loop() {
getFingerprintIDez();
delay(50);
}
uint8_t getFingerprintID() {
uint8_t p = finger.getImage();
switch (p) {
case FINGERPRINT_OK:
Serial.println("Image taken");
break;
case FINGERPRINT_NOFINGER:
Serial.println("No finger detected");
return p;
case FINGERPRINT_PACKETRECIEVEERR:
Serial.println("Communication error");
return p;
case FINGERPRINT_IMAGEFAIL:
Serial.println("Imaging error");
return p;
default:
Serial.println("Unknown error");
return p;
}
p = finger.image2Tz();
switch (p) {
case FINGERPRINT_OK:
Serial.println("Image converted");
break;
case FINGERPRINT_IMAGEMESS:
Serial.println("Image too messy");
return p;
case FINGERPRINT_PACKETRECIEVEERR:
Serial.println("Communication error");
return p;
case FINGERPRINT_FEATUREFAIL:
Serial.println("Could not find fingerprint features");
return p;
case FINGERPRINT_INVALIDIMAGE:
Serial.println("Could not find fingerprint features");
return p;
default:
Serial.println("Unknown error");
return p;
}
p = finger.fingerFastSearch();
if (p == FINGERPRINT_OK) {
Serial.println("Found a print match!");
} else if (p == FINGERPRINT_PACKETRECIEVEERR) {
Serial.println("Communication error");
return p;
} else if (p == FINGERPRINT_NOTFOUND) {
Serial.println("Did not find a match");
return p;
} else {
Serial.println("Unknown error");
return p;
}
Serial.print("Found ID #"); Serial.print(finger.fingerID);
Serial.print(" with confidence of "); Serial.println(finger.confidence);
return finger.fingerID;
}
int getFingerprintIDez() {
uint8_t p = finger.getImage();
if (p != FINGERPRINT_OK) return -1;
p = finger.image2Tz();
if (p != FINGERPRINT_OK) return -1;
p = finger.fingerFastSearch();
if (p != FINGERPRINT_OK) return -1;
Serial.print("Found ID #"); Serial.print(finger.fingerID);
Serial.print(" with confidence of "); Serial.println(finger.confidence);
if(finger.fingerID == 1){
lcd.clear();
lcd.setCursor(2,0);
lcd.print("Finger accepted,");
lcd.setCursor(2,0);
lcd.print("now scan card...");
lcd.setCursor(0,3);
lcd.print("===================>");
while( ! mfrc522.PICC_IsNewCardPresent());
while( ! mfrc522.PICC_ReadCardSerial());
if (mfrc522.uid.uidByte[0] == 0x92 && //========================EDIT CODE======================
mfrc522.uid.uidByte[1] == 0xAB && //Take that piece of paper with the ID on it, there were 4 sets of 2 digits
mfrc522.uid.uidByte[2] == 0x90 && //Look by the code, see where it says 0x92, 0xAB, 0x90, 0x1c? Enter each
mfrc522.uid.uidByte[3] == 0x1C) { //of the 2 digit section after the 0x. For example, the section of the ID says
lcd.clear(); //3E, then enter the 3E after the 0x to make 0x3E. Do this for each section
lcd.setCursor(3,0);
lcd.print("Finally, enter");
lcd.setCursor(1,1);
lcd.print("joystick password");
while(analogRead(A2) >= 100 &&
analogRead(A2) <= 670 &&
analogRead(A3) >= 100 &&
analogRead(A3) <= 670){
}
lcd.setCursor(8,4);
lcd.print("*");
if(analogRead(A2) <= 100){
d1 = L;
}
if(analogRead(A2) >= 670){
d1 = R;
}
if(analogRead(A3) <= 100){
d1 = U;
}
if(analogRead(A3) >= 670){
d1 = D;
}
delay(500);
while(analogRead(A2) >= 100 &&
analogRead(A2) <= 670 &&
analogRead(A3) >= 100 &&
analogRead(A3) <= 670){
}
lcd.print("*");
if(analogRead(A2) <= 100){
d2 = L;
}
if(analogRead(A2) >= 670){
d2 = R;
}
if(analogRead(A3) <= 100){
d2 = U;
}
if(analogRead(A3) >= 670){
d2 = D;
}
delay(500);
while(analogRead(A2) >= 100 &&
analogRead(A2) <= 670 &&
analogRead(A3) >= 100 &&
analogRead(A3) <= 670){
}
lcd.print("*");
if(analogRead(A2) <= 100){
d3 = L;
}
if(analogRead(A2) >= 670){
d3 = R;
}
if(analogRead(A3) <= 100){
d3 = U;
}
if(analogRead(A3) >= 670){
d3 = D;
}
delay(500);
while(analogRead(A2) >= 10 &&
analogRead(A2) <= 670 &&
analogRead(A3) >= 100 &&
analogRead(A3) <= 670){
}
lcd.print("*");
if(analogRead(A2) <= 100){
d4 = L;
}
if(analogRead(A2) >= 670){
d4 = R;
}
if(analogRead(A3) <= 100){
d4 = U;
}
if(analogRead(A3) >= 670){
d4 = D;
}
delay(500);
if(d1 == L && d2 == U && d3 == L && d4 == R){ //=================EDIT CODE======================
lcd.clear(); //This section is were you can edit the password with the joystick
lcd.setCursor(2,0); //the password is set to left, up left, right. If you want to change
lcd.print("Access granted!"); //it, put a L for left, R for right, U for up, or D for down in any of the
lcd.setCursor(2,1); //4 sections with a letter after the == signs.
lcd.print("Drawer unlocked.");
lcd.setCursor(2,2);
lcd.print("When done, move");
lcd.setCursor(1,3);
lcd.print("joystick to relock");
servo.write(90);
while(analogRead(A2) >= 100 &&
analogRead(A2) <= 670 &&
analogRead(A3) >= 100 &&
analogRead(A3) <= 670);
servo.write(170);
lcd.setCursor(3,0);
lcd.print("Drawer locked");
delay(3000);
resetFunc();
}else{
lcd.clear();
lcd.setCursor(2,0);
lcd.print("ACCESS DENIED!!!");
lcd.setCursor(0,2);
lcd.print("Restarting system...");
delay(3000);
resetFunc();
}
}else{
lcd.clear();
lcd.setCursor(2,0);
lcd.print("ACCESS DENIED!!!");
lcd.setCursor(0,2);
lcd.print("Restarting system...");
delay(3000);
resetFunc();
}
}else{
lcd.clear();
lcd.setCursor(2,0);
lcd.print("ACCESS DENIED!!!");
lcd.setCursor(0,2);
lcd.print("Restarting system...");
delay(3000);
resetFunc();
}
return finger.fingerID; }
Step 6: Finish
One thing I forgot to tell you, well 2 things is that i built this before i took pictures, so I couldn't show you how I built the box. The other is that it is recommended to screw servo part onto the part that turns. If you don't, someone could pull the drawer while its locked and pull the piece off. But before you screw it on, find the right spot where to put it on because the servo turns to a certain degree. Or you could just change it in the code. If some of the electronics are acting funny, you may want to find a different way to get 5V for some of them. I noticed when my fingerprint scanner flashed, the screen would slightly flash with it, and the servo would make noise. When the servo moved, the screen would go dim. I hope you enjoyed the project! If you have any questions, leave them in comments. I am not on instructables a lot, but I will answer them as soon as I can!
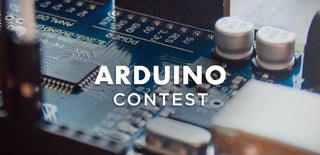
Participated in the
Arduino Contest 2020