Introduction: Arduino Indoor Doorbell
So I wanted to test out the capabilities of some basic RF transmitter/receiver modules for the Arduino and, after going through this awesome tutorial, I decided to create a small project.
In this instructable, I am going to show you how to create a simple, wireless doorbell that plays a given melody.
Why, you ask? Well, I noticed that, whenever someone (in that case parents) calls me for dinner, I can barely hear it because my door is closed or they are too far away. Since I do not check my phone so often, sending me a SMS does not do it either. I decided to change that by creating an indoor doorbell, so whenever someone needs me downstairs, pressing a button is all that person has to do. The receiver will be placed in my room and play a really loud and annoying melody.
What you will need:
- Two Arduinos (I used the Arduino Nano with the ATmega 328 chip)
- Two project boxes
- Two 220 Ohm resistors
- Two LEDs
- One RF Transmitter (e.g. with 433 MHz)
- One RF Receiver (with a matching frequency)
- One button
- One vibration sensor (Piezo) - this can be replaced by another button if you do not have one
- One Piezo loudspeaker
- One/two switches (optional)
- Two 9V batteries or other power sources
- Wires
- Two PCB boards
Step 1: Building the Transmitter
I chose to go with a 9V battery for the transmitter. This makes it very portable but requires a power switch to not drain the battery too much.
Step 2: Breadboard Assembly
Before soldering, you can put everything on a breadboard first to see if its working.
Of course, some code is required to get it working. The code is discussed in later steps.
Step 3: Prepare the Project Box
I used files to create three holes in the top part of the project box. These will later be the places for the switch, the button and the LED.
Step 4: OPTIONAL: Standoffs
It is always a good idea to not solder directly near a microcontroller. That is why I choose to solder standoffs to the PCB and insert the Arduino later.
Step 5: Solder
What? Where? How?
- Create a connection between the 5V output of the Arduino and the 220 Ohm resistor
- Solder the positive side of the LED to the resistor
- The left wire of the LED should be soldered to D3
- One wire of the push button should be soldered to GND and the other one to D2
The transmitter is powered directly by the power source (which should not be over 12V)! I used some standoffs to easily replace the transmitter, if necessary.
- The VCC of the transmitter should be connected to VIN of the Arduino, GND to GND on the Arduino
- The data pin needs to be connected to D12
- Create a connection between the center pin of the power switch and the positive pole of your power source
- One of the left pins on the switch should then be connected to VIN of the Arduino
That's it.
I am sorry for the poor image quality but you should be able to assemble everything by the steps described above.
Update:
After running into some connectivity problems, I decided to solder a 17 cm long wire to the ANT pin on the transmitter. The signal now ranges over several floors!
Step 6: Halfway There
After having soldered the 9V battery to the corresponding wires, your transmitter is ready to go!
Time to move to the receiver.
Step 7: Building the Receiver
Because the receiver has to listen for signals 24/7, I chose an 8.4V power supply instead of a 9V battery.
If you do not have a vibration sensor, you can also use a simple push button. I decided to go with the vibration sensor because that way I do not have to find a way for fixing a button to the project box
Step 8: Breadboard Assembly
Notice that a jumper wire that should go from the 5V output of the Arduino to the positive power line is missing in the first picture.
Watch out for the polarity of the Piezo loudspeaker. One pin should be marked with a + sign. Connect that one to D9.
After assembly, you can give this configuration a test run by flashing the receiver code to the Arduino and sending signals with your transmitter. You find the code some steps below.
Step 9: Solder
In picture 1, you can see the layout of the receiver. The Arduino will, as seen in picture 2, be placed on the standoffs.
Proceed as follows:
- Solder one wire of the vibration sensor to A0, the other one to the 5V output
- Connect VCC of the receiver to the 5V output
- The receiver pin next to VCC goes to D11
- Solder GND of the receiver to GND on the Arduino
- Take the Piezo loudspeaker and connect the pin with the + symbol to D9.
- The other pin of the Piezo goes to GND
- Connect the 220 Ohm resistor to D2 and the positive wire of the LED
- Connect the negative wire of the LED to GND,
Step 10: Project Box
- Drill a hole in the project box. It should be big enough for the plug of your power source.
- Wrap the vibration sensor and the power plug in electrical tape.
- Glue the sensor to the bottom of the box.
- Place the Arduino on top of it and close the box.
Step 11: Code
The hardware is ready to go, so let's focus on the software.
Both, receiver and transmitter make use of a so called library Virtual Wire. You can download it by clicking this link. The library can be installed like any ordinary Arduino library by placing the main folder into the library folder of the Arduino IDE.
Transmitter
The transmitter code works fairly easy and can be described by two steps:
- Send a codeword to the receiver when a button is pressed.
- Flash a LED to show that messages are being sent.
After initializing the transmitter module in the setup function, you can use this function to send a message a given amount of times in the given interval.
<p>void sendMessage(char* message, int times, int interval){</p><p>digitalWrite(3, false); // Flash a light to show transmitting</p><p>for(int i = 0;i< times;i++){</p><p> vw_send((uint8_t *)message, strlen(message));</p><p> vw_wait_tx(); // Wait until the whole message is gone</p><p> delay(interval);</p><p>}</p><p>digitalWrite(ledPin, true);</p><p>}</p>
I prepared the file Transmitter.ino to automatically send the message "Food" twenty times every 100 milliseconds when the button is pressed. This increases the chance that at least one message arrives at the receiver unit.
<p>void loop() { </p><p>//Transmit the message when the button is pressed</p><p>if(!digitalRead(buttonPin)){</p><p>sendMessage(codeword, 20, 100);</p><p>}</p><p>}</p>
The loop constantly checks if the button is pressed. You could also define an interrupt. This would probably save much power.
Receiver
The receiver's code is a bit more complicated.
It is designed to play a melody over the Piezo loudspeaker as soon as the message "Food" arrives through the receiver module. As soon as the user hits the whole unit, the vibration sensor triggers a signal causing the alarm to stop.
Because the signal for the vibration sensor has to be read analogically, you can not easily define an interrupt. Instead, I defined an alternative delay function that always checks the vibration sensor's state:
<p>bool sleep(int duration){</p><p>int steps = 10; //The state of the vibration sensor is checked in this interval</p><p>for(int i=0;i<duration;i+=steps){</p><p>delay(steps);</p><p>if(checkVibrationPiezo()){</p><p>stop_it = true; //This stops the music</p><p>digitalWrite(ledPin,false);</p><p>return true;</p><p>}</p><p>}</p><p>delay(steps%duration);</p><p>return false;</p><p>}</p><p>bool checkVibrationPiezo(){</p><p>int current_state =analogRead(vibrationPin);</p><p>if(abs(vib_state-current_state)>20){ //Adjust this number by your needs. A smaller number means more sensitivity</p><p>vib_state = current_state;</p><p>return true;</p><p>}</p><p>vib_state = current_state;</p><p>return false;</p><p>}</p>
The melody makes use of code published on the Arduino website. I modified it to play the melody "Twinkle, Twinkle, Little Star". You can use own melodies by defining two arrays: One for the single notes and one for the time a single note should be played:
<p>char twinkle[] = "ccggaagffeeddc ggffeedggffeed ccggaagffeeddc "; // a space represents a rest</p><p>int twinklebeats[] = { 1, 1, 1, 1, 1, 1, 2, 1, 1, 1, 1, 1, 1, 2, 0.5, 1, 1, 1, 1, 1, 1, 2, 1, 1, 1, 1, 1, 1, 2, 0.5, 1, 1, 1, 1, 1, 1, 2, 1, 1, 1, 1, 1, 1, 2, 4 };</p>
To play such melody, you give the arrays to the playMelody function:
playMelody(twinkle,twinklebeats, sizeof(twinkle));
Because the music should stop at a vibration event, the playMelody function regularly calls the sleep function.
Check the Receiver.ino file and adjust it for your needs.
When both files are flashed to the corresponding Arduinos, you should be ready to go.
Attachments
Step 12: Sum Up
Possible improvements:
- To increase battery lifetime of the transmitter, you could remove all onboard LEDs of the Arduino and adjust the code to work with interrupts instead of the loop function.
- My latest project is working with a different RF library to enable communication between Arduinos and a Raspberry Pi. This project could be included so that the Raspberry can ring the doorbell. This is just a software problem. Check my profile, as this project will be uploaded soon.
I hope this tutorial gave you some overview about the functionality of RF modules in combination with microcontrollers.
As this was my first instructable, I am happy if you give me some feedback! Also, please leave a comment if you have some open questions!
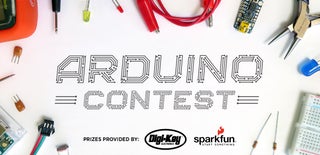
Participated in the
Arduino Contest 2017
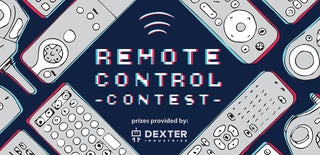
Participated in the
Remote Control Contest 2017