Introduction: Arduino Nano Baised IR Controlled RGB LED
in this small project i want to show you how i built Arduino based RGB LED that is controlled by IR remote and powered by USB cable.
Supplies
1. RGB LED
2. IR receiver
3. USB cable
4. Arduino nano
5. IR remote
6. some cables
7. 50-100 ohm resistors (am using 47 ohm resistors but there is not much difference)
Step 1: Choose Your Remote
So which remote you use is your decision, but your remote should have 6 keys which you want to use. 2 of them is for Red, 2 of them for Green and 2 for Blue.
Step 2: Build It on a Bread Board First (not Necessary But Recommended)
Now, build the circuit on a breadboard
connections:
RGB LED GND > Arduino nano GND
RGB LED Red > Arduino nano Digital pin 5
RGB LED Green > Arduino nano Digital pin 6
RGB LED Blue > Arduino nano Digital pin 9
USB GND > Arduino nano GND
USB 5v > Arduino nano 5v
IR receiver pin 1 > Arduino nano Digital pin 4
IR receiver pin 2 > Arduino nano GND
IR receiver pin 3 > Arduino nano 5v
(all connections are shown above)
(each color pin of RGB LED is connected series to resistors)
Step 3: The Code
code is the following:
Here is the library that i used.
#include <IRremote.h>
int IR_Recv = 4; //IR receiver pin int Rval = 0; int Gval = 0; int Bval = 0; int RvalDemo = 0; int GvalDemo = 0; int BvalDemo = 0; int R = 5; //Red pin int G = 6; //Green pin int B = 9; //Blue pin #define Rup1 1976685926 //makes red brightness go up #define Rup2 3772818013 //makes red brightness go up #define Rdown1 3843765582 //makes red brightness go down #define Rdown2 3772813933 //makes red brightness go down #define Gup1 3772797613 //makes green brightness go up #define Gup2 3774104872 //makes green brightness go up #define Gdown1 3772834333 //makes green brightness go down #define Gdown2 1784778242 //makes green brightness go down #define Bup1 3980777284 //makes blue brightness go up #define Bup2 3772781293 //makes blue brightness go up #define Bdown1 3772801693 //makes blue brightness go down #define Bdown2 3361986248 //makes blue brightness go down //NOTE: YOU SHOULD CHANGE NUMBERS TO YOUR REMOTE!!!! //I have 2 of duplicate keys for example Rup1 and Rup2. because //my remote outputs 2 numbers when you press one key. //in your case you may put the same numbers in Rup1 and Rup2, Gup1 and Gup2 and so one. //When you press a key the the number should appear in Serial monitor, //That's what you should type in "#define" section. IRrecv irrecv(IR_Recv); decode_results results; void setup(){ TCCR2A = _BV(COM2A1) | _BV(COM2B1) | _BV(WGM21) | _BV(WGM20); TCCR2B = _BV(CS22); irrecv.enableIRIn(); pinMode(R, OUTPUT); pinMode(G, OUTPUT); pinMode(B, OUTPUT); Serial.begin(9600); } void loop(){ if (irrecv.decode(&results)){ long int decCode = results.value; switch (results.value){ ///////////RED case Rup1: Rval = Rval + 10; break; case Rup2: Rval = Rval + 10; break; case Rdown1: Rval = Rval - 10; break; case Rdown2: Rval = Rval - 10; break; ////////////GREEN case Gup1: Gval = Gval + 10; break; case Gup2: Gval = Gval + 10; break; case Gdown1: Gval = Gval - 10; break; case Gdown2: Gval = Gval - 10; break; /////////////BLUE case Bup1: Bval = Bval + 10; break; case Bup2: Bval = Bval + 10; break; case Bdown1: Bval = Bval - 10; break; case Bdown2: Bval = Bval - 10; break; //////////////////////////////////////// } irrecv.resume(); } if (Rval > 255) (Rval = 255); if (Rval < 0) (Rval = 0); if (Gval > 255) (Gval = 255); if (Gval < 0) (Gval = 0); if (Bval > 255) (Bval = 255); if (Bval < 0) (Bval = 0); analogWrite(R,Rval); analogWrite(G,Gval); analogWrite(B,Bval); Serial.println(results.value); delayMicroseconds(1); }
Step 4: Finishing
I heaven't done a good job of casing. all i did is just tape it, but i tried to make it as small as possible.
If you have any questions or any mistakes be sure to tell me, i will do my best to help you of fix the mistake. thanks for reading.
Step 5: TEST
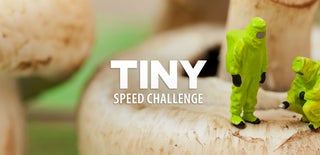
Participated in the
Tiny Speed Challenge