Introduction: Arduino Powered Fortune Teller
I created this fun Instructable to show you how to make a completely customizable Digital Fortune Teller. When the button is pushed, you will receive a random fortune. This is all the fun of fortunes, without having to eat all the cookies! Enjoy.
Supplies:
Arduino Uno
Male/Female Wires
Breadboard
Jumper Wires
Pushbutton
10k Potentiometer
10k Resistor
LED
16x2 LCD Display with header pins
9 volt Battery Holder (optional)
Step 1: Prepare Your LCD
1.) Attach Female Wires onto each Header Pin
2.) Place 10k Potentiometer on Breadboard
Attach Wire from Left 10k Potentiometer Pin to 5V (On breadboard)
Attach Wire from Right 10k Potentiometer Pin to GND (On breadboard)
3.) Attach Wire from 5V (On Arduino) to furthest guard rail on breadboard
4.) Attach Wire from GND (On Arduino) next to previous wire
5.) Attach males wires as written below:
Header Pin1 --> GND (On Breadboard)
Header Pin2--> 5V (On Breadboard)
Header Pin3 --> Middle Pin on 10k Potentiometer
Header Pin4--> Digital Pin 12 (On Arduino)
Header Pin5--> GND (on Breadboard)
Header Pin6--> Digital Pin 11 (On Arduino)
Header Pin11-> Digital Pin 5 (On Arduino)
Header Pin 12--> Digital Pin 4 (On Arduino)
Header Pin 13--> Digital Pin 3 (On Arduino)
Header Pin 14--> Digital Pin 2 (On Arduino)
Header Pin 15--> 5V (On breadboard)
Header Pin 16--> GND (On Breadboard)
Step 2: Attach Your Button
Next, you will attach your button.
1.)Solder a wire from each prong of your pushbutton
2.)Place end of wire onto Breadboard parallel to each other
3.) Place end of 10k Resistor directly next to a wire of the push button
4.) Place the other end of the 10k Resistor into the GND (on breadboard)
5.) Place Jumper wire directly next to pushbutton and attach to 5V (on breadboard)
Step 3: Attach LED
Solder both ends of LED to two wires
1.) Place one wire from LED into Digital Pin 13
2.) Place other wire from LED into GND (on Arduino)
Step 4: Make Housing for Fortune Teller (optional)
For the housing I used an old cigar box I had lying around.
I drilled two holes, one for the Button and the other for the LED.
Next I measured the LCD (1'' x 2'') and took a jigsaw and cut it out carefully.
Carefully, hot glue the LED into place.
Screw in pushbutton into bottom hole.
Push the LCD into the hole (it's a snug fit, so it should stay in place)
Next, I used command strips to hold the Arduino, Breadboard and Battery pack to make it durable.
Step 5: Code
//Electronic "Fortune Cookie"
//Written for a class project Jul 28 2014
#include #include
prog_char s1[] PROGMEM = "PRESS ME!"; prog_char s2[] PROGMEM = "That wasnt pork."; prog_char s3[] PROGMEM = "Check your zipper"; prog_char s4[] PROGMEM = "Bout time I got out of that box"; prog_char s5[] PROGMEM = "Your favorie band sucks"; prog_char s6[] PROGMEM = "A wise man listens to a box"; prog_char s7[] PROGMEM = "Oops, wrong fortune."; prog_char s8[] PROGMEM = "Eat more Taco Bell"; prog_char s9[] PROGMEM = "ZZZ..Im sleeping"; prog_char s10[] PROGMEM = "You will recieve a fortune!"; prog_char s11[] PROGMEM = "Dont turn around"; prog_char s12[] PROGMEM = "Live long and prosper"; prog_char s13[] PROGMEM = "Fortunes M.I.A."; prog_char s14[] PROGMEM = "Run."; prog_char s15[] PROGMEM = "Help! Im trapped."; prog_char s16[] PROGMEM = "404 Fortune not found"; prog_char s17[] PROGMEM = "Do not breathe underwater"; prog_char s18[] PROGMEM = "They do make your ass look big"; prog_char s19[] PROGMEM = "Zombies are coming"; prog_char s20[] PROGMEM = "Well, this is awkward."; prog_char s21[] PROGMEM = "The best is yet to come"; prog_char s22[] PROGMEM = "You only live once"; prog_char s23[] PROGMEM = "Travel with an open heart"; prog_char s24[] PROGMEM = "I cannot help, im a box"; prog_char s25[] PROGMEM = "Follow your instincts"; prog_char s26[] PROGMEM = "Be patient."; prog_char s27[] PROGMEM = "Success is a journey"; prog_char s28[] PROGMEM = "Do or do not."; prog_char s29[] PROGMEM = "Nobody can be you"; prog_char s30[] PROGMEM = "Buy the red car"; prog_char s31[] PROGMEM = "Like what you do"; prog_char s32[] PROGMEM = "Be nice"; prog_char s33[] PROGMEM = "If you want it take it"; prog_char s34[] PROGMEM = "Never spit in wind"; prog_char s35[] PROGMEM = "Dont worry about money"; prog_char s36[] PROGMEM = "Follow your instincts"; prog_char s37[] PROGMEM = "Pick your battles"; prog_char s38[] PROGMEM = "Wow! A secret message!"; prog_char s39[] PROGMEM = "Dont panic."; prog_char s40[] PROGMEM = "Dont blink"; prog_char s41[] PROGMEM = "You are a good friend"; prog_char s42[] PROGMEM = "Stop procrastinating"; prog_char s43[] PROGMEM = "You should check FaceBook"; prog_char s44[] PROGMEM = "Just be yourself"; prog_char s45[] PROGMEM = "Someones watching"; prog_char s46[] PROGMEM = "Act rather than react";
// initialize the library with the numbers of the interface pins LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
// this table is in ordinary memory but has pointers // that reference the flash PROGMEM const char *str_tab[] = { s1, s2, s3, s4, s5, s6, s7, s8, s9, s10, s11,s12,s13,s14,s15,s16,s17,s18,s19,s20,s21,s22,s23,s24,s25,s26,s27,s28,s29,s30,s31,s32,s33,s34,s35,s36,s37,s38,s39,s40,s41,s42,s43,s44,s45,s46 }; const int Number_of_fortunes=46; #define Longest_fort 100 // buffer size for messages
unsigned long count=0; const int buttonPin = 6; // the number of the pushbutton pin const int ledPin = 13; // the number of the LED pin
void setup() { lcd.print("Mystic Fortune Teller"); //set up the LCD;s number of columns and rows #define LCD_WIDE 16 #define LCD_LINES 2 lcd.begin(LCD_WIDE,LCD_LINES); // Print a message to the LCD pinMode(ledPin, OUTPUT); digitalWrite(ledPin, LOW); // set the LED off pinMode(buttonPin, INPUT); digitalWrite(buttonPin, HIGH); // set pullup on }
void loop() { // put your main code here, to run repeatedly: if (BUTTON_PUSHED()) { digitalWrite(ledPin, HIGH); // button feedback say_it(); } while (BUTTON_PUSHED()) continue; // wait for button up delay(50); // delay to debounce digitalWrite(ledPin, LOW); // set the LED off count++; } void say_it() { int thisrow; char str[Longest_fort], str2[LCD_WIDE+1]; // temp storage for fortune lcd.clear(); strcpy_P(str, str_tab[count % Number_of_fortunes]); for(thisrow=0;// thelesser of (stringlines, LCD_LINES) thisrow<=min(((strlen(str)-1)/LCD_WIDE),LCD_LINES-1); thisrow++){ lcd.setCursor(0,thisrow); // set cursor to start of this row delay(50); // sometimes setCursor is slow // copy substring for this line, from start // to lesser of LCD line length or end of string strncpy(str2,&str[thisrow*LCD_WIDE], min(LCD_WIDE,strlen(str)+1-(thisrow*LCD_WIDE))); str2[LCD_WIDE]=0; // null terminate substring lcd.print(str2); //display it delay(10); } } int BUTTON_PUSHED() { if (digitalRead(buttonPin) == LOW) // is button pulling down? return 1; // YES, it is pushed return(0); // NOPE
}
----------------------------------------------------------------------------
This code is very easy to customize!
If you need to change the responses, or number of responses make sure you do so
here.....
// this table is in ordinary memory but has pointers // that reference the flash PROGMEM const char *str_tab[] = { s1, s2, s3, s4, s5, s6, s7, s8, s9, s10, s11,s12,s13,s14,s15,s16,s17,s18,s19,s20,s21,s22,s23,s24,s25,s26,s27,s28,s29,s30,s31,s32,s33,s34,s35,s36,s37,s38,s39,s40,s41,s42,s43,s44,s45,s46 }; const int Number_of_fortunes=46; #define Longest_fort 100 // buffer size for messages
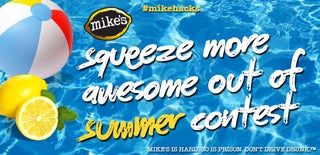
Participated in the
squeeze more awesome out of summer contest
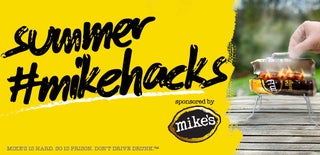
Participated in the
Summer #mikehacks Contest
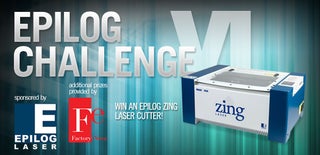
Participated in the
Epilog Challenge VI