Introduction: Arduino Retro Game Controller (Atari)
The Atari 2600 was a a home video game console made by Atari, Inc. It was released on September 11, 1977. You could play games like Frogger, Pac-Man, and Space Invaders. Since it is such an old console there's not a whole lot you can do with it, so I decided to repurpose its controllers and make something new with them.
Step 1: Cut the Cord and Clean It Out
I tried just sticking some jumper wires directly into the pins but they were too small and didn't fit, so we'll just cut off the end of the cord. Next, you should clean the controller since its probably nasty from not being used in 30 years. Here you go:
- Cut the cord a little bit from the end
- Undo all four screws in the back
- Pull out the electronic board (with the cord attached)
- Take apart all of the plastic pieces
- Carefully pry off the little plastic circle
- Wash all of the plastic stuff with soap and water
- Rinse it off
- Let it dry
- Put it back together (the same as taking it apart, but in reverse)
Step 2: Solder Pins
Like I said before, the built-in pins were too big for jumper cables. We'll have to make our own with some headers from SparkFun. If you're new to soldering, here's a helpful link.
- Strip the cord you just cut (you should see six new colored wires)
- Strip each of these wires as close to the end as you can
- Break off a six piece segment of headers
- Carefully solder the six wires onto the headers. It doesn't matter what order, but I did:
- Black
- Orange
- Brown
- White
- Green
- Blue
Step 3: Connect to Arduino
Follow the above diagram to connect this circuit to the Arduino board.
- Black (ground) to GND - this isn't shown in the diagram, so just pretend it is.
- Orange (button) to D3
- Brown (right) to D5
- White (up) to D6
- Green (left) to D9
- Blue (down) to D10
You'll notice we only used the PWM~ pins. This is because if we compensate for it in the code, we can avoid having to use resistors in the circuit.
Step 4: Serial Code
This code will allow you to view all of the incoming data in the serial monitor:
void setup() { pinMode(3, OUTPUT); pinMode(5, OUTPUT); pinMode(6, OUTPUT); pinMode(9, OUTPUT); pinMode(10, OUTPUT); Serial.begin(9600); } void loop() { digitalWrite(3, HIGH); digitalWrite(5, HIGH); digitalWrite(6, HIGH); digitalWrite(9, HIGH); digitalWrite(3, HIGH); digitalWrite(10, HIGH); Serial.print("Button: "); Serial.print(digitalRead(3)); Serial.print("\tLeft: "); Serial.print(digitalRead(9)); Serial.print("\tRight: "); Serial.print(digitalRead(5)); Serial.print("\tUp: "); Serial.print(digitalRead(6)); Serial.print("\tDown: "); Serial.println(digitalRead(10)); }
Step 5: Alternate Circuit
This one is a bit more complicated, but it will allow the control of two servos using the Atari controller. I added a battery pack to the diagram because using multiple servos can draw a lot of power.
Step 6: Test It in Real Life
As an example to show you how this can be applied, I made a turret with two servo motors that can move in four directions (up, down, left, and right). Use this code:
#include <Servo.h> Servo base; Servo tilt; int xangle = 90; int yangle = 90; void setup() { pinMode(3, OUTPUT); pinMode(5, OUTPUT); pinMode(6, OUTPUT); pinMode(9, OUTPUT); pinMode(10, OUTPUT); base.attach(7); tilt.attach(8); base.write(xangle); tilt.write(yangle); delay(100); } void loop() { digitalWrite(3, HIGH); digitalWrite(5, HIGH); digitalWrite(6, HIGH); digitalWrite(9, HIGH); digitalWrite(3, HIGH); digitalWrite(10, HIGH); int left = digitalRead(9); int right = digitalRead(5); int up = digitalRead(6); int down = digitalRead(10); int button = digitalRead(3); if (right == 0) { if (xangle < 180) { ++xangle; } } else if (left == 0) { if (xangle > 0) { --xangle; } } if (up == 0) { if (yangle < 180) { ++yangle; } } else if (down == 0) { if (yangle > 0) { --yangle; } } tilt.write(yangle); base.write(map(xangle, 0, 180, 180, 0)); delay(10); }
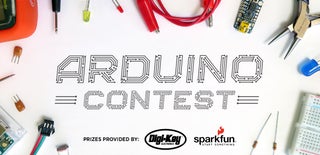
Participated in the
Arduino Contest 2017