Introduction: Arduino Solar Cell Tester
When I'm building Solar Shrubs and other solar-powered creations, I often scavenge cells from various off-the-shelf devices such as solar garden or security lights. But these cells are rarely labeled as to their voltage, current, and power output.
So it's off to my bread-boarding station. First, I put each cell under a lamp and connect it to my multimeter to get the volts. Then I build an I-R curve by measuring the voltage across a series of different sized resistors. This takes a lot of time and effort and I still don't know what the values are in sunlight. I could drag the multimeter, breadboard, and all associated components outside, but that would be a hassle and it's still very time consuming if I have a lot of cells to test.
My solution was to build an Arduino-based, handheld Solar Cell Tester. This tester can read up to 15 Volts at 1 Amp. Now I can carry one device outside, attach a single solar cell or a group of cells in series or parallel, and read voltage, current, and power quickly and accurately!
Here's how you can build one too!
NOTE: At first glance this device looks very similar to Ladyada's impressive Portable Solar Charging Tracker, But mine is actually more limited; only testing the cells, not lithium batteries and charging circuits. I used Adafruit's enclosure and protoshield for this project, but the calculations, circuits, and program are all mine (except for the excellent help, as always, from the Arduino on-line community!)
Step 1: Materials & Tools Needed
Materials Used:
(1) Arduino Uno
(1) Arduino Proto Shield (Adafruit #51)
(1) White Enclosure for Arduino (Adafruit #271)
(1) Parallax Serial LCD Panel (Parallax #27977)
(2) 10 ohm 10W Power Resistors (for current sensing circuit) (Radio Shack #271-132)
(1) 2K ohm resistor (for voltage sensing circuit)
(1) 1K ohm resistor (for voltage sensing circuit)
(1) DPDT Sub-Mini Toggle Switch (Radio Shack #275-614)
(1) SPDT Slide Switch (Radio Shack #275-409)
(1) 1/8" Phone Jack (Radio Shack #274-251)
(1) 1/8" Phone Plug (Radio Shack #274-284)
(2) 14" Insulated Test Leads (Radio Shack #278-1156)
(2) AAA Battery Holders (2 cell holder) (Radio Shack #270-413)
Tools Needed:
Soldering Iron
Solder
Helping Hands
Wire strippers
Side cutters
Dremel
Step 2: The Circuits
A DPDT toggle switch (SW1) serves as the on-off control which disconnects the 4-AAA batteries from the Arduino as well as the "Cell Under Test" from the sensing circuits. The cell under test is connected via red and black test leads attached to a phone plug and plugged into a 1/8" phone jack on top of the tester.
The tester includes two sensing circuits; one for measuring voltage and one for measuring current. A SPDT toggle switch (SW2) connects the cell under test to these circuits individually.
The Voltage Circuit: The Arduino analogRead command reads voltage up to +5V and returns an integer between 0 and 1023. In order for the tester to read up to 15V, you'll create a voltage divider that consists of a 2K (R3) and 1K (R4) resistor. The voltage across R4 is one-third the source voltage so it can read 0-15V. (NOTE: You can use any two resistors with a 2 to 1 ratio).
The Current Circuit: Since the analogRead command returns a value of 0-1023 (for a max of 5V), each unit is 5 divided by 1024 or 4.9mV. And from Ohms law, we know that the voltage drop across a resistor is equal to the current times the resistance. So the voltage drop across a 4.9 ohm resistor with 1mA of current is 4.9mV. This means we can effectively read the current across a 4.9 ohm resistor using the actual value returned by the analogRead command. There are at least two problems with this strategy.
(1) Because of it's low resistance, this circuit can produce a high current and consequently too much power for standard 1/4 or 1/2 Watt resistors. They would become dangerously hot very fast. So I recommend using Power resistors with at least a 5W rating.
(2) I looked high and low and couldn't find a 4.9 ohm power resistor.
The Solution? Connect two 10 ohm resistors in parallel, which will give you approximately 5 ohms (close enough for this project). The power resistors I used are rated at 10W so they'll stay nice and cool in this circuit.
The voltage, current, and power readings will be displayed on a 2x16 character serial LCD panel. You can use any LCD panel, but the wiring and program may need to be modified to accommodate it.
Step 3: Preparing the Enclosure
Adafruit's Arduino enclosure is perfect for projects like this. It comes with all the knockouts and screws you need to make a nice, neat, professional looking device.
First, you'll have to pull the knockouts apart and trim all of the access plastic using a razor knife or dremel tool. Then, drill holes in the top knockout panel for the two switches and the phone jack.
The bottom knockout panel includes openings for the Arduino power and USB ports. This is great for testing and updating your Arduino sketch.
Step 4: Wiring It Up
1. I used an Arduino Proto Shield from AdaFruit to wire up the circuits and connect them to the Arduino, but any prototyping board will do. All you'll use are the board and bottom pins, so there's no need to attach the headers, LEDs, resistors, or switch that come with the shield.
2. The 10W power resistors are really big and take up most of the circuit board. Solder one end of each resistor to a GND pad and the other to a row of individual holes that can also accommodate the wires connected to SW2 and A2 pin of the Aruduino.
3. Next, solder in the 1K and 2K resistors (along with the SW1 lead and ground connection) for the voltage divider circuit.
4. The Serial LCD panel includes a 3-pin connector with 5V, GND, and Rx. Cut off one end of the 3 jumper wires and solder to 5V, GND, and digital pin D3 on the proto board.
5. Use ordinary hookup wire for the switches, battery holders, and phone jack. Make sure you wire the two battery holders in series (negative to positive) so you'll get the 6 Volts needed to power the Arduino. BTW, I used two 2xAAA holders specifially because that's what will fit into the enclosure.
6. Mount the switches and phone jack to the top knockout.
7. Mount the Arduino and LCD panel. They should fit neatly inside the enclosure. The screw holes line up perfectly!
NOTE: If you use the same serial LCD panel I did, you will find that the jumper connectors stick up a little too high for the enclosure. So you'll have to bend the pins to accommodate.
8. Once everything is wired up and screwed in, it's time to close the case. It will be really crowded in there, so be careful to bend your wires around components and screw holes and get everything lined up before putting in the final case screws.
9. Label the switches "ON - OFF" and "V - A". (My label making skills are weak as you can see.)
10. And finally, solder the ends of red and black jumper wires to the phone plug making a nice little plug-in jumper cable.
Step 5: The Program
The sketch is very simple. I'm basically reading analog values on A1 and A2 and updating the LCD via D3.
/*
Solar Cell Tester 15V-1A
by Mike Soniat
September 8, 2012
*/
const int RxPin = 3;
#include <SoftwareSerial.h>
SoftwareSerial lcdPanel = SoftwareSerial(255, RxPin);
int voltPin = 1;
int ampPin = 2;
int readVolts = 0;
int readAmps = 0;
int maxVolts = 15; //Must match voltage circuit resistors
float voltageFactor = 0;
float voltage = 0;
float current = 0;
float power = 0;
const int clearIt = 12;
const int cr = 13;
const int backLightOn = 17;
const int backLightOff = 18;
void setup() {
pinMode(RxPin, OUTPUT);
digitalWrite(RxPin, HIGH);
lcdPanel.begin(9600);
startUp();
}
void loop()
{
//measure voltage
readVolts = analogRead(voltPin);
if (readVolts > 0)
{
while (readVolts > 0)
{
readVolts = analogRead(voltPin);
if (readVolts > 0)
{
voltageFactor = 1024 / maxVolts;
voltage = readVolts / voltageFactor;
clearLCD();
lcdPanel.print("Reading ");
lcdPanel.print(voltage);
lcdPanel.print("V");
lcdPanel.write(cr);
lcdPanel.print("Move Switch to A");
}
delay(1000);
}
}
else
{
startUp();
}
//switch to current circuit
readAmps = analogRead(ampPin);
while (readAmps > 0)
{
if (voltage > 0)
{
readAmps = analogRead(ampPin);
current = readAmps;
power = voltage * current;
clearLCD();
lcdPanel.print(voltage);
lcdPanel.print("V ");
//lcdPanel.print("I = ");
lcdPanel.print(current);
lcdPanel.print("mA");
lcdPanel.write(cr);
lcdPanel.print(power);
lcdPanel.print("mW");
delay(1000);
}
else{
readAmps = analogRead(ampPin);
}
}
delay(1000);
}
void clearLCD()
{
lcdPanel.write(clearIt); // Clear
delay(5);
}
void startUp()
{
clearLCD();
lcdPanel.write(backLightOn);
lcdPanel.print("15V - 1A Solar");
lcdPanel.write(cr);
lcdPanel.print("Cell Tester");
delay(3000);
clearLCD();
lcdPanel.print("Move Switch to V");
lcdPanel.print("and Connect Cell");
delay(2000);
}
Step 6: Trying It Out
Here's how it's supposed to work:
When you turn the power on, the LCD will toggle between: "15V - 1A Solar Cell Tester" and "Move Switch to V and Connect Cell".
As soon as the tester senses voltage, the LCD changes to: "Reading " and the voltage in Volts, followed by, "Move Switch to A"'
When you move the slide switch to "A", the LCD will display voltage in Volts, current in mA, and power in mW.
NOTE: If the reading is less than 1mA, the tester will assume no cell is connected and display the initial "15V - 1A Solar Cell Tester" message.
Field Testing!
Before I took it outside, I had to add the finishing touch; an Instructables Robot Sticker!
Then, I grabbed a variety of solar cells from my workbench and went outside to test. You'll see the results in the photos!
Success!!!
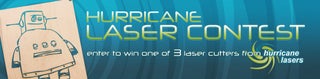
Participated in the
Hurricane Lasers Contest