Introduction: Arduino Ultrasonic Sensor HC-sr04 Led + Distance Measure Projects.
In this intructable, we will learn about how to make an Arduino distance sensor with the help of an ultrasonic sensor ( Hc-sr04 ) and LEDs. The ultrasonic sensor is used to measure the distance of objects like scale and the LEDs are used to indicate the distance in cm. Visit my website for more tutorials.
When the first led is on that means the distance of an object is 7cm and the max distance detected of this project is 49cm that means the last LEDs will turn on. We can easily extend the range of the Hc-sr04 sensor by changing its define centimeter in code.
How ultrasonic sensor work?
The ultrasonic sensor continues to the transmitter the signal by trigpin and receives the signal by echopin. Hc-sr04 transmit the frequency of 40khz sound wave and when an object detects then reflect the signal to echopin. The time between the transmission and reception of the signal allows us to calculate the distance to an object.
Features of ultrasonic sensor:
Voltage (v): +5V DC.
Current: 20mA.
Distance Measure: 2cm to 400cm📏.
Measuring Angle: 30 degree.
Sensor Frequency: 40khz.
Usages of ultrasonic sensor:
- Arduino abstacle avoiding robot
- Distance measure
- Arduino radar sensor
- Measure water level and many more.
Step 1: Watch This Video!
In the tutorial, we show how to make a distance measurement device with Arduino Uno, Ultrasonic sensor, and some LEDs.
Step 2: Gather the Component:
Component requirements for arduino distance measurement devices.
Arduino Uno
Ultrasonic sensor Hc-sr04
7 x LEDs
7 x 220ohm resistor
Breadboard
Jumper wire
Step 3: Make the Connection:
Connect Ultrasonic sensor ( Hc-sr04 ) pins to arduino Uno pins.
1. Connnect ultrasonic sensor Trig Pin to arduino digital Pin 12.
2. Connnect ultrasonic sensor Echo Pin to arduino digital Pin 13.
3. Connnect ultrasonic sensor VCC Pin to arduino 5v Pin.
4. Connnect ultrasonic sensor GND Pin to arduino GND Pin.
Connect LEDs pins to arduino uno pins.
1. Connnect LED1(+) Pin to arduino Digital Pin 8.
2. Connnect LED2(+) Pin to arduino Digital Pin 7.
3. Connnect LED3(+) Pin to arduino Digital Pin 6.
4. Connnect LED4(+) Pin to arduino Digital Pin 5.
5. Connnect LED5(+) Pin to arduino Digital Pin 4.
6. Connnect LED6(+) Pin to arduino Digital Pin 3.
7. Connnect LED7(+) Pin to arduino Digital Pin 2.
8. Connnect all LED Negative Terminal ( - ) to arduino GND Pin.
Don't forget to add a 220ohm resistor to each led, refer the circuit diagram.
You can also add a buzzer to produce a sound, refer this link for code and circuit diagram.
If you have any problem and question comment it below.
Step 4: The Code:
//arduino ultrasonic sensor hc-sr04 led projects code. /* Code: * by Sayed Mohsin * mrelectrouino.blogspot.com */ const int trig = 12; const int echo = 13; const int LED1 = 8; const int LED2 = 7; const int LED3 = 6; const int LED4 = 5; const int LED5 = 4; const int LED6 = 3; const int LED7 = 2; int duration = 0; int distance = 0; void setup() { pinMode(trig , OUTPUT); pinMode(echo , INPUT); pinMode(LED1 , OUTPUT); pinMode(LED2 , OUTPUT); pinMode(LED3 , OUTPUT); pinMode(LED4 , OUTPUT); pinMode(LED5 , OUTPUT); pinMode(LED6 , OUTPUT); pinMode(LED7 , OUTPUT); Serial.begin(9600); } void loop() { digitalWrite(trig , HIGH); delayMicroseconds(1000); digitalWrite(trig , LOW); duration = pulseIn(echo , HIGH); distance = (duration/2) / 28.5 ; Serial.println(distance); if ( distance <= 7 ) { digitalWrite(LED1, HIGH); } else { digitalWrite(LED1, LOW); } if ( distance <= 14 ) { digitalWrite(LED2, HIGH); } else { digitalWrite(LED2, LOW); } if ( distance <= 21 ) { digitalWrite(LED3, HIGH); } else { digitalWrite(LED3, LOW); } if ( distance <= 28 ) { digitalWrite(LED4, HIGH); } else { digitalWrite(LED4, LOW); } if ( distance <= 35 ) { digitalWrite(LED5, HIGH); } else { digitalWrite(LED5, LOW); } if ( distance <= 42 ) { digitalWrite(LED6, HIGH); } else { digitalWrite(LED6, LOW); } if ( distance <= 49 ) { digitalWrite(LED7, HIGH); } else { digitalWrite(LED7, LOW); } }
Attachments
Step 5: Code Explanation:
const int trig = 12;
const int echo = 13;
Start with defining the pin of the ultrasonic sensor to the Arduino Uno board. TrigPin is connected to Arduino Pin 12, and EchoPin is connected to Arduino Pin 13. The Trig and Echo pin are used to transmit and receive the ultrasonic sound wave.
const int LED1 = 8;
.
.
const int LED7 = 2;
Next, define the LEDs to Arduino Uno so, LED( 1, 2, 3, 4, 5, 6, 7 ) is connected to Arduino Pin ( 8, 7, 6, 5, 4, 3, 2 ) respectively. LEDs are used to indicate the distance of the object.
int duration = 0;
int distance = 0;
Now create two variables of types integer: duration and distance. The duration variable is used to hold the time between the transmission and reception of ultrasonic waves. The distance variable is used to store a distance in cm.
In void setup() , we have to set the trigPin as an output and the echoPin as an Input and LEDs as an Output.
pinMode(trig , OUTPUT);
pinMode(echo , INPUT);
pinMode(LED1 , OUTPUT);
.
.
pinMode(LED7 , OUTPUT);
Serial.begin() is used to initialize the serial communication for showing the results on the serial monitor.
In void loop(), We have to trigger the sensor by setting the trigPin HIGH for 1000 µs. For clearing the signal, We have to trigger the sensor by setting it LOW.
digitalWrite(trig , HIGH);
delayMicroseconds(1000);
digitalWrite(trig , LOW);
duration = pulseIn(echo , HIGH);
For calculation the duration with the help of pulseln() function. Pulseln() function is used to read a pulse on a pin (either HIGH or LOW). when the value is HIGH, Send the signal from LOW to HIGH then start the time, and wait for the signal is reflect an object and received by the echo pin and stops timing. Returns the length of the pulse in microseconds that is duration.
More Info! Pulseln()
After that, you can calculate the distance by using the formula.
distance = (duration/2) / 29.1 ;
You divide by two because it goes out and back so the time would be double that of a one-way travel. The speed of sound is 343 m/s which is converted into 0.0343cm/uS is equal to 1/29.1 cm/uS.
Serial.println(distance);
Print the distance in serial moniter.
if ( distance <= 7 )
digitalWrite(LED1, HIGH);
else
digitalWrite(LED1, LOW);
When the distance is equal to a given distance then LED1 is turn on. When the distance is not equal to a given distance then LED1 is turn off.
Notes that the result in cm.
Step 6: Enjoy!
After completing the circuit, connect the Arduino Uno to the computer, upload the code, and you've finished. If you guys correctly followed the instructable, put the object near to the ultrasonic sensor, when the ultrasonic sensor detects the object in a given centimeter, the LED should turn on.
Thank you for sticking to the end. I hope you all love this project and learned something new today. Let me know if you make one for yourself. Subscribe to my YouTube channel for more upcoming projects. Thank you once again!
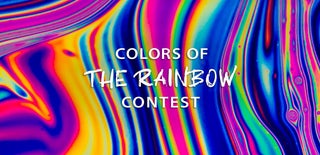
Participated in the
Colors of the Rainbow Contest