Introduction: Automated Chicken Coop Door ( Arduino )
Hi Guys !
I ( or at least my dad ) wanted an automated chicken coop door so that you don't really have to worry if it rains, if you are sleeping too much or if you go to dinner with friends.
So I finally decided to do one, and you can see it on the picture.
At first I wanted to do a lateral opening door, using drawer glides but I was too difficult not to have problems with chickens making them dirty and thus unusable.
So I finally decided to do a basic vertical opening door, and you will discover how exactly in the next steps !
Enjoy
I have to say I used an instructable to build my door, but at the end,the result is quite different so I decided to write one, but have a look at Julio's :
https://www.instructables.com/id/Chicken-Door-arduino/
Step 1: Do Your Math and Get Your Material
Here is what I bought, you can buy the exact same, or try to inovate, it will probably work the very same way.
There is just a question about the solar powerbank. With a very simple calcul, I thought I would be fully autonomous but I'm not, it might be due to the high tempratures in the plastic box when sun shines, I don't really know.
I'm just going to tell you how I did my math, if some see something wrong just tell me, and if not well you will have an idea of how to do that.
With a nano in sleep mode, I use about 5mA and my motor, when not opening the door, consumes around 100mA ( maybe an other problem, it might consume much more when opening the door.
My solar panel is 1,5W, and I need 5mA, 1,5W/5V = 300mA, so in theroy, when the sun shines, I'm largely able to store energy for the night and all, but apparently no.
Moreover, the powerbank was sold for 10 000mA but when I opend it it was written 8 000 on it, so well.
But admitting the solar panel doesn't work, my consumption would be ( approximatly ) : 5mA *24h + NumberOfWakeUp*durationOfWakeUp*60mA ( close to 0 ) + 3m*MotorEnergy .< 150mAh.
But It works just for about a week, while 8000/150 = 53days...
Here there is something I don't get well, so do your math carefully and ty to uderstand what you really need ( for me one week is ok, works for the weekends, etc... ) but don't hesitate to buy something better.
What is tricky is that those two steps are really closely linked and work together.
My material :
- ldr resistance
- arduino nano
- stepper motor
- bread board
- solar powerbank ( or not solar depending on what you need )
- other stuff you can find probably anywhere.
Step 2: Use Arduino
Okay so now that you have everything you need, we can start the arduino code. You can see here what how I did the set up.
Basically what you have to understand is that my LDR tells me how much light there is outside, and I process it. I also have 2 buttons, one to make the door go up, and an other to make it go down, just so that I can place it exactly as I want, and if there is to be a problem ( like a hen playing with my door ), I can easily put it back in initial position.
The code is here and I explain it along so that you can understand it step by step.
You will need to download two libraries here ( thanks to Julio ) :
https://mega.nz/folder/MCYmSQyB#if8FQkk6RSbSdkQdjYASKQ
#include <LowPower.h>
#include <CheapStepper.h> CheapStepper stepper (8,9,10,11);
boolean moveClockwise=true; const int ldrPin = A2; const int OuvPin = 5; const int FermPin = 6; int x = 1; const int pinReveil=2; const int pinReveil2=3; const int pinReveil3=4; const int pinReveil4=12;
So here I basically define all my constants and variables, I'm French so their names may sound romantic to you. First I define which pins are to be the ones for the stepper motor. Then I define the pins that will recieve information ( from the LDR and from the buttons ). X is my door's state ( 1 = open, 0 = closed ).
Finally, I define pins on which are some resistances, and "réveil" in french means wake up, because I'm going to stimulate my powerbank every few seconds ( you will see that just after ) so that it doesn't turn off. Every power bank is different so you will have to try with a very basic program what is the minimum intensity and for how long, for me it was at least 50mA for at least 100ms every 50s. So just solder resistances depending on what you found.
void setup() { pinMode(pinReveil,OUTPUT); pinMode(pinReveil2,OUTPUT); pinMode(pinReveil3,OUTPUT); pinMode(pinReveil4,OUTPUT); pinMode(ldrPin, INPUT); pinMode(OuvPin, INPUT); pinMode(FermPin, INPUT); stepper.setRpm(1); Serial.begin(9600); }
Here I just say you will receive current and who will give some, and I chose the speed of my stepper motor, it has to be between 1 and 8, 8 being the slowest.
void loop() digitalWrite(pinReveil,HIGH); // The pins is now +5V digitalWrite(pinReveil2,HIGH); digitalWrite(pinReveil3,HIGH); digitalWrite(pinReveil4,HIGH); delay(100); digitalWrite(pinReveil,LOW); // The pin is now 0 digitalWrite(pinReveil2,LOW); digitalWrite(pinReveil3,LOW); digitalWrite(pinReveil4,LOW); // Here that is what I was talking about : stimulating my powerbank so that I don't have to // open it and solder things, or power my arduino with 3.6V. if(digitalRead(OuvPin) == 1 ){ while ( digitalRead(OuvPin) == 1 ){ moveClockwise = true; stepper.moveDegrees(moveClockwise, 1 ); } digitalWrite(8,LOW); digitalWrite(9,LOW); digitalWrite(10,LOW); digitalWrite(11,LOW); x=1; // We consider that we will use this button only to put the door in full "open" position } // I just checked whether my 'open' button was used or not, and acted accordingly. else if(digitalRead(FermPin) == 1 ){ while ( digitalRead(FermPin) == 1 ){ moveClockwise = false; stepper.moveDegrees(moveClockwise, 1 ); } digitalWrite(8,LOW); digitalWrite(9,LOW); digitalWrite(10,LOW); digitalWrite(11,LOW); x=0; // We consider that we will use this button only to put the door in full "closed" position } // Same here with closing button. int ldrStatus = analogRead(ldrPin); delay(500); // I get the value of the ldr resistance, and let a delay to process the information. if(ldrStatus > 150 && x==0){ // If my door is closed and light is enough moveClockwise = true; stepper.moveDegrees(moveClockwise,2500); stepper.moveDegrees(moveClockwise,2500); stepper.moveDegrees(moveClockwise,2500); stepper.moveDegrees(moveClockwise,2500); stepper.moveDegrees(moveClockwise,2000); x=1; digitalWrite(8,LOW); digitalWrite(9,LOW); digitalWrite(10,LOW); digitalWrite(11,LOW); } // I open my door if it's closed and if the threshold is reached ( you have to observe your chickens // so that you know when they get in and out and adapt values accordingly. else if (ldrStatus<6 && x==1) { moveClockwise=false; stepper.moveDegrees(moveClockwise,2500); stepper.moveDegrees(moveClockwise,2500); stepper.moveDegrees(moveClockwise,2500); stepper.moveDegrees(moveClockwise,2500); stepper.moveDegrees(moveClockwise,2000); x=0; digitalWrite(8,LOW); digitalWrite(9,LOW); digitalWrite(10,LOW); digitalWrite(11,LOW); } // same with closing else { for (int i=0; i<6;i++) LowPower.powerDown(SLEEP_8S, ADC_OFF, BOD_OFF) } // If I'm not in those situations ( full day or night ), I just put my arduino in sleep mode // to try to save energy. }
Here you have all the code, and don't hesitate to ask questions.
Step 3: Build Your Door.
I'm a lucky boy because we already had a door in rails, so I just had to adapt it for it to work. But it was really too heavy so I had to use the hoists to reduce the force needed.
Then I used a some wheels I had in an old game and I pasted them on my motor.
The rest is just balancing.
The door opens slowly, due to the pulleys, but it works well ( about 1 minute and a few seconds to open ).
I took an old plastic box for my powerbank to be protected but doesn't work as expected due to the high temprature inside... I'm going to try new things out in the next few weeks and I will publish an update if I find something better.
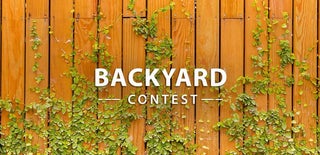
Participated in the
Backyard Contest