Introduction: Automatic Snow Wake-up Call
Getting out of the house in the morning can be a flurry of activity after a few inches of the white stuff settles over night. Wouldn't it be nice to be woken up a little earlier on those days to take the stress out of the morning? This project does just that!
This project uses an Arduino, a distance sensor, and IFTTT (an easy to use website) to automate a wake-up call to your phone if it's snowed overnight. Once ready, you'll place the device you built at a high point (for example on a tripod) and point it down at the ground. From there it will constantly measure the distance between itself and the ground. As it snows, the "ground" moves up towards it, so the distance it measures decreases. If there's a big enough difference between the evening and the morning, the device will alert you that it snowed!
The electronics are simple to connect and I'll provide the code, so let's jump right in!
Supplies
- A Wifi enabled Arduino-compatible microchip. For this project I'm assuming you'll use the ESP8266 NodeMCU Development Board, which I recommend for many reasons:
- It has Wifi built in.
- It exposes pretty much all the pins you might want.
- It provides an easy USB interface for programming.
- It handles the board reset when uploading code, and exposes reset buttons for debugging.
- A TF Mini Lidar range sensor.
- Note there are cheaper ultrasonic sensors like the HC-SR04, but soft snow muffles sound enough that they don't work for this.
- A mini breadboard.
- A tripod or any solution for mounting the sensor a few feet above the snow.
- A micro usb cable.
- An extension cable.
- A usb charger.
- A plastic container.
Note, items 5 and above can be bought at Dollar Tree pretty easily.
Prices vary, but I was able to do this project for around $50 (not counting the tripod) by shopping at Dollar Tree for the simple parts. The most expensive part by far is the Lidar sensor, which can definitely be re-used for other projects.
Step 1: Electronics (Hardware)
The electronics for this project should be pretty quick to assemble. Just connect the TF Mini Lidar Distance Sensor to the chip. The wires should connect as described in this excellent SparkFun guide.
Here's a quick summary:
Sensor -> ESP8266
Green -> D2 (aka GPIO 4, which we will use as our RX)
White -> D1 (aka GPIO 5, which we will use as our TX)
Red -> Vin
Black -> Gnd
Step 2: Electronics (Software)
The decision to send you a wake-up call will be made by your microchip, so we need to program it appropriately! To program your chip, we'll use a language called Arduino which you can upload to your chip using the Arduino IDE (software that runs on your computer).
1. Download the Arduino software here. This guide will be referencing the Arduino Desktop IDE menus, so go ahead and download that unless you're super comfortable with the Web IDE.
2. Set up your Arduino Desktop IDE to work with the ESP8266 microchip. The instructions for that can be found here. Going forward, this guide assumes that you got your LED blinking and know how to upload a script to the ESP8266.
3. Download the script to upload to your microchip from https://github.com/robertclaus/snowalert. No need to edit the script. Everything you need to configure will be configurable after you upload the code.
4. Open the script in Arduino and install the libraries it depends on in your system. At the top of the IDE, click: Sketch -> Include Libraries -> Manage Libraries
Then search for and install these libraries:
- WifiManager by tzapu (version 0.14.0)
- ArduinoJson by Benoit Blanchon (version 6.14.1)
- TFminiArduino by hideakitai (version 0.1.1)
- NTPClient by Fabrice Weinberg (version 3.2.0)
- ESP_DoubleResetDetector by Khoi Hoang (version 1.0.1)
5. Configure your board for this project. At the top of the IDE, click Tools and adjust these settings:
- Flash Size - 4M (1M SPIFFS) -- This reserves space for our configuration to be saved.
- Erase Flash - All Contents -- This makes sure there isn't previous data on the chip.
- Note, if you ever need to update the code, setting this to Sketch Only will preserve your configuration.
6. Make sure your ESP microchip is plugged into your computer and has an assigned port. Select the correct port in the IDE, and upload!
7. Open the Serial Monitor (Tools -> Serial Monitor) in the Arduino IDE. Then click the Reset button on your chip. Confirm that you get text showing in the Serial Monitor
Step 3: IFTTT Configuration
Now that your Arduino is running, we need to configure it to do what we want. For this tutorial, we will use a service called IFTTT that allows us to translate a simple message from our Arduino to more complicated actions.
For example, if our Arduino says "It Snowed!" then IFTTT should call our cell phone with a wake-up call.
1. You'll need a free IFTTT account, which you can create at https://ifttt.com/join
2. To create the new Applet that uses this logic, navigate to Create, or just follow this link: https://ifttt.com/create
3. Click on This -> Search and select Webhooks -> If it asks you to, click Connect -> Enter snow_alert in the box.
4. Click on That -> Search and select Phone Call (US Only) -> If it asks you to, click Connect -> If you get a popup, follow the prompts -> Enter a message like It snowed last night! that you would like the phone call to read out for you.
5. Click Finish to activate your applet.
6. Test your Webhook by navigating to your Webhooks service settings in IFTTT, and finding the test URL listed there. Navigate to that URL and replace {event} with snow_alert. Then click Test It. If everything is working, you should receive a phone call!
7. On the test page, save the url towards the bottom of the page. You'll need it in a later step. It should look something like this:
https://maker.ifttt.com/trigger/snow_alert/with/key/d-Y8rXge5kibp0dkdrCgxu
For debugging issues, users may also want to log the snow height over time. They can configure a separate IFTTT applet that accepts the snow_measurement webhook and logs to Google Sheets. To do this, simply repeat the steps above, but replace snow_alert with snow_measurement in the Webhooks step above and replace the Phone Call step with the Google Sheets service -> Add row to spreadsheet.
Step 4: SnowAlert Configuration
At this point the last software step is configuring the code on your ESP to send the messages to your new IFTTT applet.
For this configuration, I'm going to recommend you follow the SnowAlert instructions on Github because the instructions here might be outdated if SnowAlert gets new features.
At the time of writing these instructions, you would do the following.
Very importantly, all the times you configure need to be in the UTC timezone and in 24 hour time format (not AM/PM).
- Decide what time you want to receive the call in the morning. This is your End Time.
- Decide what time to start measuring the previous evening. This is your Start Time.
- Plug in your ESP and open the Serial Monitor in the Arduino IDE like we did previously.
- Connect to the SnowMeasure wifi network on your computer. You should see some activity in the Serial Monitor as you connect.
- You should be directed to a setup page in your browser automatically after a few seconds.
- Click Configure Wifi
- Enter the following values:
- SSID - The wifi network the ESP should connect to for internet.
- Password - The password to connect to that wifi network.
- Start Hour - The hour you want it to measure the snow height in the evening.
- Start Minutes - The minute component to the time you want it to measure in the evening.
- End Hour - The hour you want it to measure the snow height in the morning (and potentially call you)
- End Minutes - The minute component to the time you want it to measure in the morning.
- The Alert Webhook URL - This should be the url you saved in the previous step that looks something like this: https://maker.ifttt.com/trigger/snow_alert/with/k...
- The Measurement Webhook URL - This should be the same url as above, but replace snow_alert with snow_measurement
Step 5: Mount the Sensor
At this point everything should be ready to go. You'll want to cut holes in the plastic container, and mount it somewhere a few feet off the ground. How exactly you mount it will depend on your parts and goal, but here are a few recommendations to get it right.
- Make sure your sensor is facing down at a slight angle. You don't want it looking straight down since your box will cast a shadow where the snow doesn't hit the ground.
- You'll want the sensor up off the snow about 2-3 feet.
- Snow melts into water, so make sure your setup is reasonably waterproof.
- Make sure you have power! Either a long usb cable, or an extension cord should get you away from a roof to get to a useful spot. Either way, make sure that it's safe outside.
Step 6: Get the Call!
If everything goes according to plan, you should get the call in the morning if it snows. If something isn't working, check your distance logs in Google Sheets to see what was actually measured.
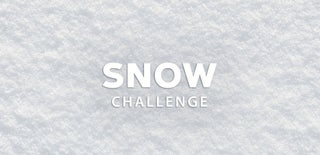
Runner Up in the
Snow Challenge