Introduction: Automatically Track Views on Your Instructable With Python!
So after publishing my first instructable (for a while) yesterday I got excited about the number of people that were already reading it!
You can read it here if you are into ESP8266.
So I decided to re-purpose a script that I had written to estimate delivery times from a certain courier in order to track the number of people who have currently viewed your site.
The previous script used Yodel's "Delivery before yours" website feature to give a (fairly accurate) time of your delivery that updated in real time, and maybe I can 'ible that one day. Leave a comment if you think that'd help you out.
In this instructable I will show you how I built this program but you can just as easily skip to the end and download the final python script.
Step 1: Download and Install Python
In this instructable I am using python 2.7. Don't know why, just I always use python 2.7
Download it from https://www.python.org/downloads/
Download for your system and install as you normally would a program on your computing device.
If you are using Android, I wrote a tutorial for getting python up and running on Android on my no longer updated old and bare electronics blogger blog (some description eh?) that you can view by clicking this link right here.
Now that the rest of us have python installed, we can move on to the next step.
Step 2: Request Raw HTML Data From 'ibles.com
Next you will need to open IDLE, which is the developing platform, or IDE for Python.
Click "File" -> "New File"
Then copy the following code into your window:
import urllib2
from datetime import datetime import time myInstructable = ' COPY AND PASTE THE LINK TO YOUR INSTRUCTABLE HERE ' response = urllib2.urlopen(myInstructable) html = response.read() print html
And then be sure to copy and paste the link to your instructable where it says COPY AND PASTE THE LINK TO YOUR INSTRUCTABLE HERE, being sure to keep the
' '
either side.
Next click "Run" -> "Run Module"
Step 3: Inspect What 'ibles Gives You
Your screen should now look like the image above, just a lot of HTML code.
We are going to look for a specific line in the HTML that is telling us how many views we have had on our instructable.
You could scroll through in the IDLE window to find it, or you can do it the easy way.
The easy way involves saving the HTML code, and then opening it with a browser so you can inspect what you are looking for.
For this I like to edit it to remove the bits and pieces from IDLE using notepad++, a great code editor that works with many languages.
Download from https://notepad-plus-plus.org/ and install it on your machine.
Next, go back to the IDLE window with all of the HTML code splurted everywhere and press Ctrl+A, to select all, and Ctrl+C to copy it.
Then, Open Notepad++ and paste the data into a new document.
Step 4: Edit What 'ible Gives You
It will then look like the first picture, which is grey and black and not very easy to read.
If you click "File" -> "Save" and then save it as: YOURHTML.html to somewhere on your computer, then it will become much easier to read.
Just be sure to add .html after the name and notepad++ will know that it is supposed to be looking at an HTML file and correct accordingly.
Now it should look like the second picture.
Now what I recommend to do is delete the code that isn't HTML, from IDLE.
So scroll all the way to the top and find where it says:
Python 2.7.13 (v2.7.13:a06454b1afa1, Dec 17 2016, 20:42:59) [MSC v.1500 32 bit (Intel)] on win32
Type "copyright", "credits" or "license()" for more information. >>> ======= RESTART: C:/Users/YOU/Desktop/Instructables html request.py =======
and delete that, you don't need it. If you miss it you can get it back from IDLE.
Then scroll all the way to the bottom and delete the:
>>>
If you miss those, press "Shift" + "." three times.
Save again somewhere easily accessible.
Step 5: Open With Google Chrome
Next, find where you have saved the HTML file to, and open it with Google Chrome
If it appears like the image above then just double click it, if not right click it and select "Open With" -> "Google Chrome".
It should appear like the second image, a sort of stripped bare version of your instructable.
What you want to do next is, in Google Chrome, scroll down to where it says "About This Instructable", which should be right above your profile picture and below your informative post.
Then right-click on where it says your number of views and select "Inspect"
This will then show you exactly the code we are looking to pull out of the 'ible server in order to count our views.
So right-click on the line that was automatically highlighted and click "copy element".
Step 6: Update Our Python Script
Next, paste the element back into our IDLE file (Not the shell).
We want to charm our python into giving us just the number in between
<span class="count view-count">
and
</span>
In order to do this, we will parse the information.
So to the end of the file, add the lines:
html = html.partition("<span class=\"count view-count\">")[2]
html = html.partition("</span>") [0]
just before where it prints the html, so that it chops off everything before and after the number we are after.
The code now should read:
import urllib2
from datetime import datetime import timemyInstructable = ' THE LINK TO YOUR INSTRUCTABLE '
response = urllib2.urlopen(myInstructable) html = response.read()
html = html.partition("<span class=\"count view-count\">")[2] html = html.partition("</span>") [0] print html
Then when you click "Run" -> "Run Module" again, instead of getting loads of html back you will just get back the amount of people who have viewed your post. Cool innit?
Step 7: Get the Code to Repeat and React According to Views
OK, in this step I have, comparatively, added a lot of code to the example, but I will walk you through it from the top:
count = -1
while(count!=1):
This section makes all that is indented below it run forever. You are basically saying until "count" magically turns from -1 into +1 then keep running the code. As long as you don't change the value of "count" to 1, you will be fine.
html = html.replace(",", "")
htmlint = int(html)
What I have only just learned since gaining over 1,000 views is that after 1,000 instructables adds a comma to the number.
Since Python is not very open minded, we have to use the replace line to get rid of the comma and replace it with nothing.
Then we need to tell python that actually the HTML we are looking at is actually a number so we use the int() function, which tells python that anything inside the () is an integer.
print datetime.now().time()
print htmlint
This section prints the current time at which the python program executed, giving you an accurate record.
It then prints the number of views you have had.
if htmlint % 10 == 0:
print "Another TEN people have read your 'ible"
This line uses an IF statement to find out if the number of views is directly divisible by 10, and if it is it means 10 more people have viewed your instructable. It then tells you that.
time.sleep(60)
This line then tells the whole program to wait for 60 seconds until it checks again.
Step 8: That Is It!
Once you leave that running for a while, you should get a print out of the time and how many views you had at that time.
I have attached the final file to this step.
If a print out is not enough for you you can use Twilio to integrate a text feature to text you at every landmark (a congratulatory text when I hit 1,000 would have been great).
You can also use a predictive algorithm to see how many views you are likely to get maybe?
If you have any ideas or want me to make a tutorial on the text feature or the Yodel predictor that I talked about in step one then please leave a comment.
Thanks for reading!
Tom
P.S.
If you are advanced, I am sure you would have no problem running this on a web server somewhere if you have a hosting account, then it can just run all day long with no problems and let you know when you hit your self set landmarks. OR EVEN set it up on your own raspberry pi. Let me know what you get up to.
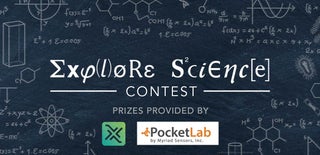
Participated in the
Explore Science Contest 2017
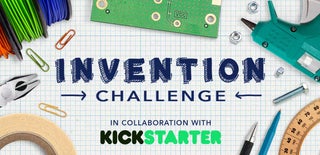
Participated in the
Invention Challenge 2017