Introduction: Back to School LED Strip Automation
This project is for the intermediate DIY-er who has a slight understanding of what raspberry pi and arduino are. Even if you are a beginner, there are many resources out there to get you started with this cool and fun project. I will answer any comments you have as well.
This project can easily be expanded from controlling LEDs to controlling alarm clocks, coffee makers, door locks, etc! I'm just focusing on LED strips here.
This is my first instructable, so let me know in the comments if there is anything I should elaborate on.
I am also entering this 'ible into the automation contest. If you enjoyed this please vote for me!
Step 1: Overview
This project uses:
- Smartphone or computer to control lights
- Raspberry pi to host (1) an MQTT broker and (2) a flask webserver.
- RGB LED strips
- 3 MOSFETs to control RGB PWM
- Adafruit feather M0 Wifi or other Wifi-enabled micro controller to control the colors of the LED strip.
- Make sure that your micro controller has at least 3 PWM pins on it!! The ESP8266 does not have hardware built in for PWM, although it is possible to do it through software...
- Soldering Iron and assorted wires
Control Flow:
- Smartphone/computer clicks on a button on the Flask webserver running on the pi, which changes the URL to include the color the user wants
- The webserver parses the URL to find the color the user wants
- The webserver publishes an MQTT message containing the color to the Adafruit feather M0
- The Adafruit feather M0 handles the MQTT message and triggers the appropriate PWM response to create the desired color on the LED strips
Step 2: Raspberry Pi Configuration
If you are unfamiliar with the pi, there are many tutorials on instructables and youtube that will guide you in the right direction. Personally, I find the easiest way to use a raspberry pi is to control it using ssh from my laptop.
Use this guide to get started with ssh if you don't know how: https://www.raspberrypi.org/documentation/remote-a...
Once you are situated with your raspberry pi and ssh, install the following packages:
sudo apt-get install python-pip
sudo apt-get install mosquitto mosquitto-clients python-mosquitto
sudo pip install paho-mqtt
sudo pip install flask
sudo pip install flask-mqtt
sudo pip install flask-socketio
Now you are ready to edit the mosquitto config file. Go to:
cd /etc/mosquitto
sudo nano mosquitto.conf
Edit your config file to say:
allow_anonymous true
So that you don't need a username and password. Since we're only controlling LED strips, there is no security issue here.
Now you can start the mosquitto broker in the background:
sudo systemctl restart mosquitto
Step 3: MQTT and Flask Logic on Pi
To get acquainted with Flask on the pi, check out the official user-friendly documentation from raspberrypi.org:
https://www.raspberrypi.org/learning/python-web-se...
The documentation for using Flask and MQTT together can be found here:
http://flask-mqtt.readthedocs.io/en/latest/usage.h...
MQTT was a little confusing for me, so here are some helpful tips:
MQTT is made up of publishing and subscribing to different topics. Open up two new ssh windows and do the following:
- Window 1: type mosquitto_sub -t "test"
- This will subscribe that window to the topic "test"
- Window 2: type mosquitto_pub -t "test" -m "Hello from window 2"
- This will publish the message "Hello from window 2" to all windows subscribed to the topic "test"
This should hopefully give you enough info to start messing around and get a feel for MQTT.
____________________________________________
To set up the webserver on the pi, go to home:
cd ~
Then create a new folder to house your project; let's call it RoomServer
sudo mkdir RoomServer
now enter that folder
cd RoomServer/
We need to create three things inside of RoomServer,
- The "Brains" of our webserver which will be a python script
- A folder to hold our html
- A folder to hold our bootstrap, javascript, and other css
To get an idea of what this hierarchy looks like, go to my github and browse these files:
https://github.com/guitarman513/RoomServer
starting with the Brains, create a file called webserver.py:
sudo nano webserver.py
now copy the following code into the file. Look at the attached screenshot of the code in case your browser displays the indents improperly:
##################################################################################
from flask import Flask, render_template
from flask_mqtt import Mqtt
from flask_socketio import SocketIO
app = Flask(__name__)
app.config['MQTT_BROKER_URL'] = 'localhost' # use the free broker from HIVEMQ
app.config['MQTT_BROKER_PORT'] = 1883 # default port for non-tls connection
#app.config['MQTT_USERNAME'] = '' # set the username here if you need authentication for the broker
#app.config['MQTT_PASSWORD'] = '' # set the password here if the broker demands authentication
app.config['MQTT_KEEPALIVE'] = 5 # set the time interval for sending a ping to the broker to 5 seconds
app.config['MQTT_TLS_ENABLED'] = False # set TLS to disabled for testing purposes
mqtt = Mqtt(app)
socketio = SocketIO(app)
@app.route('/')
def main():
return render_template('html/main.html')
############### 404 page
@app.errorhandler(404) def page_not_found(e):
return render_template('html/notFound.html'), 404
###################################### routing the colors
@app.route("/LED/color/ ")
def action(color):
message = color
mqtt.publish("LED/color",message)
return render_template('html/main.html')
##################################### running the server
if __name__ == '__main__':
socketio.run(app, debug=True, host='0.0.0.0')
##################################################################################
Now exit by pressing control + x and save your work!
You can run your server by typing
python webserver.py
You can see this server run by going to your computer on the same wifi and typing the address of your pi into the address bar. The server runs on port 5000. For me, since my pi's address is 10.0.0.63, I type this into the address bar:
10.0.0.63:5000
Since you don't have any of the html yet, you will most likely get an error!
As you can see from @app.route("/LED/color/ ") above in the code, as the URL changes from, for example,
LED/color/red to
LED/color/blue
The webserver will publish the color using the MQTT protocol!
To finish the folder structure for the flask webserver, visit my github and download the necessary files. Change them around as you like to get a feel for everything!
Step 4: Arduino Coding Time
Now lets get to the LEDs. Using the Arduino IDE, we will upload the code to the FeatherM0. First, you should go to my github (again) and install the custom, simple RGB LED PWM Arduino library I made. This will make the code we upload to the board much cleaner.
https://github.com/guitarman513/RGB_LED_PWM_Arduin...
Now upload the attached .ino file to the Adafruit Feather M0. You will have to change your pi's local IP address and your local wifi name and password of course!
If you are having problems uploading, check the adafruit website for how to update your board and install the proper drivers on the Arduino IDE.
Attachments
Step 5: Wiring the Circuit and Hanging LEDs
Make sure your MOSFETs can handle the specs of this project:
Gate voltage should be around 5V
Able to withstand ~7A tops depending on length of LED strip
The Feather M0 pins trigger the gate pins of the MOSFETs, allowing current to flow between the Source and Drain pins, powering the LED strips. Make sure that the pins you use correspond to the pins you chose in the arduino sketch!
This may seem intimidating but it's actually a pretty simple circuit. Once this is done you're ready to hang up the strips. Most strips come with an adhesive backing, which is usually good enough. I used thumbtacks to pinch the end of the strip against the ceiling at the corners where the adhesive wasn't good enough.
If you are daisy-chaining multiple LED strips, make sure that your power supply can handle the increased current.
Step 6: Test It Out!
Now you are ready to enjoy the fruits of your labor! I encourage you to change the html and color combinations as you see fit.
This is my first instructable and I am entering it in the automation contest. Please vote for me if you enjoyed this! Leave a comment if you are stuck on something; I'm happy to help.
Best,
Joe
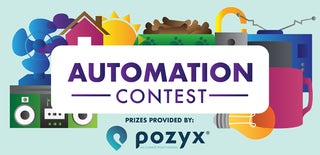
Runner Up in the
Automation Contest 2017