Introduction: Basic Arduino Strobe Light
This instructable will show you how to make a basic strobe light with an arduino.
You need
- 4 leds (the code can be modified for more if you want)
- an arduino (i am using the UNO r3)
- a paperclip
- a computer with the arduino ide installed
- an A/B USB cable to plug the arduino into the computer
- possibly some pliers to help bend the paperclip
(sorry for the lack of pictures)
Step 1: Assemble the Board and Leds
put a paperclip in the digital ground pin and bend it down and across for the led leads to touch. next put the positive lead (the longer one) in pin 7 so it also touches the paperclip. Repeat for pins 9, 11, and 13.
Step 2: Install the Program
download the sketch or copy and paste it into the ide. here it is:
//leds in pins 13, 11, 9, and 7 (to make it easy to put them on i did every other pin)
//use a paperclip for ground
void setup() {
unsigned int delayvar = 100;//You can chane this but it wont do much
pinMode(13, OUTPUT); //define pins as output
pinMode(11, OUTPUT);
pinMode(9, OUTPUT);
pinMode(7, OUTPUT);
digitalWrite(13, HIGH); //blink twice
digitalWrite(11, HIGH);
digitalWrite(9, HIGH);
digitalWrite(7, HIGH);
delay(delayvar);
digitalWrite(13, LOW);
digitalWrite(11, LOW);
digitalWrite(9, LOW);
digitalWrite(7, LOW);
delay(delayvar);
digitalWrite(13, HIGH);
digitalWrite(11, HIGH);
digitalWrite(9, HIGH);
digitalWrite(7, HIGH);
delay(delayvar);
digitalWrite(13, LOW);
digitalWrite(11, LOW);
digitalWrite(9, LOW);
digitalWrite(7, LOW);
delay(delayvar);
}
void loop() {
unsigned int strobespeed = 150; //change for a different strobe speed
pinMode(13, OUTPUT);//defineing pins as output
pinMode(11, OUTPUT);
pinMode(9, OUTPUT);
pinMode(7, OUTPUT);
digitalWrite(7, HIGH);
delay(strobespeed);
digitalWrite(7, LOW);// the led on pin 7 turns off when
digitalWrite(9, HIGH);//the led on pin 9 turns on
delay(strobespeed);
digitalWrite(9, LOW);// this pattern continues
digitalWrite(11, HIGH);
delay(strobespeed);
digitalWrite(11, LOW);
digitalWrite(13, HIGH);// the strobe stops
delay(strobespeed);
digitalWrite(13, LOW); //and starts going the other way
digitalWrite(11, HIGH);
delay(strobespeed);
digitalWrite(11, LOW);
digitalWrite(9, HIGH);
delay(strobespeed);
digitalWrite(9, LOW);// repeats
}
Attachments
Step 3: Things to Remember
You can edit this code to fit your needs (please give me credit if you use it in another instructable, i worked hard to make it from scratch)
If you have any question feel free to comment or message me, i will get back to you as soon as i can.
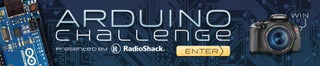
Participated in the
Arduino Challenge