Introduction: CNC Part Picking Machine
If you're a serious maker like I am, then you'll most likely have countless resistors, capacitors, and various other electronic components lying around. But there is a major problem: How does one keep track of what or how many of something they have? For this issue I created a CNC machine that gets information from a MySQL database that then goes and retrieves the item that was requested. In addition to the database back-end, I made a front-end webpage that allows for users to login and then create categories of parts, add new parts, and change the quantities of parts. This way every single item can be accounted for, just like a stock management system.
Components:
Arduino UNO & Genuino UNO
Machine Screws: 8mm,3mm,4mm
MOSFET N-channel
Rectifier Diode 1N4001
Stepper Motor NEMA 17 x2
Driver DRV8825 for Stepper Motors x2
Capacitor 100 µF x2
Step 1: Theory
The basis of this system is to keep track of inventory. For example, if someone buys 20 Arduino Uno boards they could easily add that amount to a database table. The category would be "Arduino", name of "Uno", and a quantity of 20. For multiple people, the owner of that part would be the username of the person who added it. The part would also include data about the it's location on a grid. Whenever the part amount changes the CNC machine would then select that part and give it to the user.
Step 2: Database
I needed a ubiquitous database that could be accessed by both Python and PHP. It also had to be easy to use with plenty of support, making MySQL the perfect database server. I began by downloading the mysql installer from https://dev.mysql.com/downloads/windows/installer/ and then ran it. I chose to install the server (of course), and also the workbench, shell, and utilities. When you choose a username and password make sure to remember it, as those same credentials are needed in all of the PHP files and the Python script. After starting the server enable it to run as a background process so it will always be active. From here on in everything must be spelled and in the exact same order as I have it. Next, create a new database (schema) called "components". Then add the following tables: "categories", "parts", and "users". In the categories table add the following columns in this exact order: "id" -int(11), PK, AI; "name" -varchar(45); "owner" - varchar(45).
In the parts table add the following columns in this exact order: "id" -int(11), AI, PK; "category" -varchar(45); "name" -varchar(45); "quantity" -int(11); "owner" -varchar(45); "locationX" -int(11); "locationY" -int(11);
In the users table add the following columns in this exact order: "id" -int(11), AI, PK; "username" -varchar(45); "password" -varchar(128);
Step 3: Setting Up Apache
The webpages I have created utilize HTML, CSS, Javascript, and PHP. Start by downloading the latest apache version from http://www.apachelounge.com/download/ and unzip it, moving the folder to the C:\ directory. Next, download PHP from http://windows.php.net/download#php-7.2 and make sure it is the Thread Safe version. Unzip it, rename it to "PHP", and move it to the C:\ directory. Then go into C:\Apache24\conf\httpd.conf and edit it. Add the following lines right below the section:
LoadModule php7_module C:/PHP/php7apache2_4.dll
<IfModule php7_module>
DirectoryIndex index.html index.php
AddHandler application/x-httpd-php .php
PHPIniDir "C:/PHP"
</IfModule>
Then test your server by running httpd.exe located in the bin folder. Head to "localhost/" in your browser and see if the hello world page comes up. If it does, hurray, you now have a local webserver.
Step 4: Setting Up PHP
In order to set up MySQL for PHP several things must be done. First, rename "php.ini-recommended" to "php.ini" and then open it in notepad. Head to the extensions section and add or uncomment "extension=php_mysqli.dll" which will let PHP communicate with the MySQL server. Now restart httpd.exe and create a new file called "phptest.php" and put into the file. Now go to localhost/phptest.php and see if your browser information comes up.
Step 5: Designing the Machine
I began by creating some basic parts in Fusion 360: a 6mm rod, linear bearing, and a stepper motor. Then I spanned two rods across to form the y axis, and also put a timing belt around the stepper motor and bearing. I also added an x axis, as well. I then started to 3D print various parts and also CNC routed two side panels.
Step 6: Making the Machine
I ended up going through multiple iterations of each part, so if any are different that is why. I started by sanding each part and then drilling out each hole in the 3D printed parts. Then I put linear bearings into the holes and ran the 6mm rods through them. I also mounted the stepper motors into their respective locations after attaching the pulleys to their shafts. The timing belt got looped around each of the two sides for both axes. Eventually I realized that the gripper would be too cumbersome, so I opted for an electromagnet instead. I also had some help while building it, in the form of a cat.
Step 7: Arduino Code
My basis for this machine was GRBL. The start of the code lists various parameters, such as distance per rotation, offsets, and extents. I used the BasicStepperDriver library to control the DRV8825 stepper motor drivers. The stepper drivers are set to use 1/32 micro-stepping, increasing the resolution. Whenever the machine "boots up" it goes through a homing sequence where each axis steps until it hits a limit switch. Then it moves based off of the offset to a set location and sets the location to 0, 0. Now whenever it receives a move command via serial it moves to that grid location.
Step 8: Python Program
I chose to use Flask as a webserver that would receive GET requests from the main website. The requests consist of the name and category of the part. After Flask handles it the data gets parsed, then the MySQL server gets queried to find out the location of the part. Then the python script sends a command to the Arduino, specifying where part is.
Attachments
Step 9: Using the Part Picker
I have provided the website files in my github repository: https://github.com/having11/cnc_part_picker_webpages
Replace the missing parameters in the PHP files for your specific MySQL server. Put the files into the htdocs folder in the Apache folder. Simply run the python script and then whenever the part amount gets changed the machine will go to that location and get it. Find the 3D printing files here and the webpage files here.
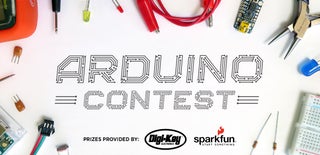
Participated in the
Arduino Contest 2017