Introduction: Caesar Cipher Program in Python
The Caesar Cipher is an ancient and widely used cipher that is easy to encrypt and decrypt. It works by shifting the letters of the alphabet over to create an entirely new alphabet (ABCDEF could shift over 4 letters and would become EFGHIJ).
Caesar Ciphers are not the most secure ciphers out there but are good for small tasks such as passing secret notes or making passwords a little stronger. It is really easy to decipher the code, but it can be tedious to encrypt one if you don't have the special alphabet memorized.
To make this process easier, we can use the power of computers, more specifically the programming language Python.
This Instructable will show you how to create a program that converts messages into a cipher at your command.
Supplies
All you need is a Python interpreter: IDLE, Pycharm, and Thonny are some good, free options (I used Pycharm)
Basic knowledge of Python
Step 1: Declaring Variables and Getting Inputs
To actually store the string (text) values of the alphabet, message, shift, etc. we need to use variables. We start by declaring the variables 'alphabet', 'partialOne', 'partialTwo', and 'newAlphabet'. I've written the names of the variables in Camel Case in my code (the first word is lowercase and second uppercase) but you can write it any way you want, as long as you remember to change it throughout the rest of the code too. The alphabet variable has the value "abcdefghijklmnopqrstuvwxyz". All of the other variables are set to "", which is an empty string as we do not yet have their values.
What this is doing is setting up the Partial system, which is what we are using to actually create the shift. This will be explained in a later step.
After this, we have to get the message and shift value from the user. We use the input function to do this. This part of the code asks the user for a message and a number to shift the alphabet by.
CODE:
alphabet = "abcdefghijklmnopqrstuvwxyz"
partialOne=""
partialTwo=""
newAlphabet=""
message = input("Please enter the message you want to translate: ").lower()
key = int(input("Please enter the number you want to shift by: "))
Step 2: Creating the New Alphabet
Now to create the shifted alphabet. To do this, we will use the partial system. The partial system is where the computer splits the alphabet into two partials (a fancy way of saying parts). The first partial is however long you told the program to shift by, and the second one is the remainder. The computer switches the partials. That is exactly what the code is doing, along with the first statement, which says that if the shift is 0, the new alphabet and the old alphabet are the same since you are not switching anything.
For example:
Sequence - 123456789
Partial One - 123; Partial Two - 456789
New Sequence - 456789123
CODE:
if key == 0:
newAlphabet = alphabet
elif key > 0:
partialOne = alphabet[:key]
partialTwo = alphabet[key:]
newAlphabet = partialTwo + partialOne
else:
partialOne = alphabet[:(26 + key)]
partialTwo = alphabet[(26 + key):]
newAlphabet = partialTwo + partialOne
Step 3: Shifting the Message
Now we have our alphabet and the new alphabet. All that is left is to switch the message into the code.
First, we set a new variable and call it 'encrypted' and set it to "". Then we write a really complicated for-loop which checks each letter in the message and switches it to the new letter. It outputs the result and there you have it, a successfully converted code!
CODE:
encrypted=""
for message_index in range(0,len(message)):
if message[message_index] == " ":
encrypted+= " "
for alphabet_index in range(0,len(newAlphabet)):
if message[message_index] == alphabet[alphabet_index]:
encrypted+= newAlphabet[alphabet_index]
print(encrypted)
Step 4: Additional
Attached is the code file.
Attachments
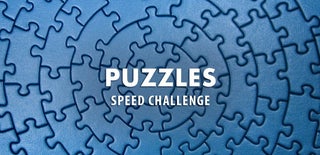
Participated in the
Puzzles Speed Challenge