Introduction: Coding Simple Playdoh Shapes W/ P5.js & Makey Makey
This is a physical computing project which allows you to create a shape with Playdoh, code that shape using p5.js and trigger that shape to appear on the computer screen by touching the Playdoh shape using a Makey Makey.
p5.js is a open source, web based, creative coding environment in Javascript. Learn more here: https://p5js.org/
You do not need any coding experience to do this project. This could be used as an introduction to text based coding (as opposed to block based languages like Scratch). You only need to write 4 lines of code to complete this project. There are several ways you can change and expand on this basic idea.
Supplies
Makey Makey Kit ( w/ 2 Alligator Clips)
Playdoh (Any Color)
Laptop w/ internet Connection
Step 1: Make a Playdoh Shape
Make a shape out of the Playdoh. This could be a circle, oval, square, rectangle or triangle. Be aware that you will need to code this shape later, so the simpler the shape, the easier the coding part will be. However, p5.js is able to code many different shapes, even custom ones, so you can decide on the difficulty level you want to try.
Step 2: Get Started in P5.js
If you have not used p5.js before, I recommend checking out the getting started page on the website: https://p5js.org/get-started/
I also highly recommend checking out The Coding Train youtube channel for excellent tutorials about using p5.js. Here is a link to a playlist that goes through all the basics: https://www.youtube.com/playlist?list=PLRqwX-V7Uu6...
Since p5.js is web based, you can do all your coding on the web using the p5 Web Editor. You don't need an account to do this project, but if you would like to save your work, you will need to sign up for an account.
Web Editor: https://editor.p5js.org/
The p5.js web editor has an area to write the code on the left and the canvas which will display the results of the code on the right.
Each p5.js sketch includes a setup() function and a draw() function. The setup() function will run once when the sketch first starts. In the setup() function is the createCanvas function which creates a space where your shape will be drawn. The numbers in the parentheses of the createCanvas function set the X axis (left to right) and the Y axis (top to bottom) of the canvas. The default numbers are 400, 400 which means your canvas is 400 pixels from left to right and 400 pixels from top to bottom (You can always change these to suit your needs). Be aware that the upper left hand corner of the canvas is the point 0, 0. This will be important to know when you code your shape.
The draw() function runs as a loop which means it is constantly updating, approx. 60 times per second. This can allow us to create animation in our sketches. Inside the draw() function is the background function which adds a color to our canvas. The default is 220 which is a greyscale value. 0 = black, 255 = white and number in between will be varying shades of grey. The background function can also take RGB values which allow us to add color. More on this in the next step.
Step 3: Code Your Shape in P5.js
To code your shape, you will only need to add to lines of code inside of the draw() function.
Each shape has its own function to make it appear on the canvas. Here is the reference page for all shapes in p5.js: https://p5js.org/reference/#group-Shape
To make a circle, we will use the ellipse function. This function take 3 arguments (the numbers that go inside the parentheses). The first number is the X position of the center of the circle on the canvas and the second number is the Y position on the canvas. Remember that the upper left hand corner is 0, 0 and the canvas is 400 by 400 pixels. So if I want the circle to appear in the middle of the canvas I will put it at 200 on the X axis and 200 on the Y axis. You can experiment with these numbers to get a feel for how to place things on the canvas.
The third number sets the size of the circle. For this example, it is set to 100 pixels in diameter. The ellipse function can also take a fourth argument which would change the third argument to affect the X diameter and the fourth argument would be the Y diameter. This can be used to make oval shapes instead of perfectly round circles.
To set the color of our shape, we use the fill function. This uses 3 arguments which are the RGB values(R = red, G = green, B = blue). Each value can be a number between 0 and 255. For example, to make red, we would put 255, 0, 0 which would be all red with no green or blue. Different combinations of these numbers will create different colors.
There are several websites which provide RGB values for many different colors, like this one: https://www.rapidtables.com/web/color/RGB_Color.ht...
Once you have found the RGB value to match your color of PlayDoh, write the fill function above the shape function.
You can then click the play button in the web editor and you should see your shape appear on the screen.
Step 4: Make Your Shape Appear With a Key Press
Since we want to have our p5.js sketch be interactive with the Makey Makey, we need to add some code to make something happen when we press a key on the keyboard. In this case, we want the shape to only appear if we press a key. To do this we need a conditional statement. This means that something in our code will only happen if a certain condition is met, in this case, a key is pressed.
To make a conditional this conditional statement, we start with the word if followed by parentheses. Inside of the parentheses will be the condition we want to be met. In p5.js, there is a built in variable called keyIsPressed (make sure you use the capital letters exactly the same as written here). keyIsPressed is a boolean variable. This means that it can have a value of either true or false. When the key is pressed, it's value is true and when it is not pressed, it's value is false.
Finally we add a set of curly brackets { }. Inside of the curly brackets will be the code we want to execute if our condition is met. So we are just going to put our code to make the shape in between those curly brackets.
Now when we run our sketch, we will not see the shape until we press a key on the keyboard.
IMPORTANT NOTE: When adding key presses into our code, the web editor needs to know if we are pressing a key to write code in the text editor or we are pressing the key to do the thing we coded a key press to do. When you click the play button, move the mouse over the canvas and click on the canvas. This will bring the focus of the editor to the sketch and pressing a key will trigger the key press code we want to happen.
Step 5: Set Up Makey Makey
Get out the Makey Makey board, USB cable and two alligator clips. Attach one alligator clip to the Earth and one to the Space key (since we didn't specify a key in our code, any key we press will trigger the shape to appear).
Take the alligator clip that is attached to the Space key and press the other end into the Playdoh shape.
Plug the USB cable into the laptop.
Step 6: Touch the Playdoh Shape
Hold the metal end of the alligator clip that is attached to the Earth on the Makey Makey and touch the Playdoh shape. When you touch the Playdoh shape, the coded shape should appear on the canvas of your sketch.
Here is a link to the p5.js sketch for this project: https://editor.p5js.org/mrbombmusic/sketches/9TZAc...
If the shape doesn't appear:
1. Make sure you have clicked the mouse on the canvas of the p5.js sketch before touching the Playdoh.
2. Make sure you are holding the metal clip of the Earth wire.
Step 7: Different Shapes
Yellow Triangle: https://editor.p5js.org/mrbombmusic/sketches/ASrRr...
Blue Square: https://editor.p5js.org/mrbombmusic/sketches/nS8S0...
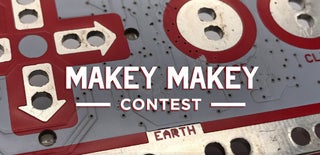
Participated in the
Makey Makey Contest