Introduction: Color Transition on POP-X2 GLCD Using a Knob
Basically, this project shows a feature of a controller board whom I love to use. POP-X2 board, made by INEX, has a built-in colored GLCD, a knob, I/O ports and components similar to other controller boards. Please check the manual of the board for full specifications. See this link.
GLCD (Graphic Liquid Crystal Display) embedded on the controller board provides a method of displaying data, not just simply texts and numbers but also with vector graphics. In this tutorial, I will teach you how to display a simple graphics to GLCD. To make it more interesting, I have added programs for the onboard knob, as a controller for the color transition.
Remember. This tutorial mainly focused on the programming side. If you own the same board or an ATX2 board, you may do this tutorial easily. Once done, you may try exploring the other functionalities of the board. :)
Now, let's start!
Step 1: What Do We Expect?
Please watch the video above.
Step 2: Gathering the Materials
Parts & Materials:
- Laptop/Desktop Computer with installed Arduino Arduino 1.7.10 (driver signed) or higher version
- 1 POP-X2 Board (with an onboard knob)
- 1 Download Cable
- 4 pcs. AA Batteries
Step 3: Hardware and Software Setup
1. Place the 4 batteries inside the battery holder. (The board supports a maximum voltage input of 7.4V.)
Note: Please check properly the polarity of the batteries.
2. Connect the download cable to the computer and to the board. Please refer to the image above.
3. Switch on the controller board. Make sure that the blue LED indicator has been lit. Or else, you need to install the Arduino software driver.
By the way, I am using Arduino version 1.7.10(driver signed) since it has the library of POP-X2 already. Please click this link to download the software.
4. Set the Port of the board by clicking Tools>Serial Port>Select the right COM Port Number.
5. Set the board by clicking Tools>Board>POP-X2, ATMega644P @ 20MHz.
6. Try to upload the default sketch to ensure that the board is connected properly.
#include // POP-X2 Libraryvoid setup(){ OK();}void loop(){}
Step 4: Knob Test
Before doing the main program, you need to make sure that the onboard knob is working.
1. Upload the sample program for the knob. Click on File>Examples>POP-X2>popx2_KnobOKTest
Basic Operation:
- The range of analog value of the knob that is displayed to the GLCD is from 0 to 1000.
- When the knob is rotated clockwise, the analog value being displayed to GLCD increases.
- When the knob is rotated counterclockwise, the analog value being displayed to GLCD decreases.
Step 5: Programming
I have attached below the source code. So, please upload it.
Preview of the Program:
#include <popx2.h> //POP-X2 Board libraryvoid setup() { OK(); }void loop(){ int reading = map(knob(),0,1000,0,245); if ((reading >= 0) && (reading <=35)){ red(); } else if ((reading >= 36) && (reading <=70)){ yellow(); } else if ((reading >= 71) && (reading <=105)){ green(); } else if ((reading >= 106) && (reading <=140)){ cyan(); } else if ((reading >= 141) && (reading <=175)){ blue(); } else if ((reading >= 176) && (reading <=210)){ magenta(); } else if ((reading >= 211) && (reading <=245)){ white(); } glcdFillScreen(GLCD_BLACK); glcd(0,0,"%d", reading);}
void red(){ setTextBackgroundColor(GLCD_RED); glcd(3,2, " "); glcd(4,2, " "); glcd(5,2, " "); glcd(6,2, " "); setTextBackgroundColor(GLCD_WHITE); setTextColor(GLCD_VIOLET); delay(1000);}
void yellow(){ setTextBackgroundColor(GLCD_YELLOW); glcd(1,8, " "); glcd(2,8, " "); glcd(3,8, " "); glcd(4,8, " "); setTextBackgroundColor(GLCD_WHITE); setTextColor(GLCD_VIOLET); delay(1000);}
void green(){ setTextBackgroundColor(GLCD_GREEN); glcd(3,14, " "); glcd(4,14, " "); glcd(5,14, " "); glcd(6,14, " "); setTextBackgroundColor(GLCD_WHITE); setTextColor(GLCD_VIOLET); delay(1000);}
void cyan(){ setTextBackgroundColor(GLCD_CYAN); glcd(9,14, " "); glcd(10,14, " "); glcd(11,14, " "); glcd(12,14, " "); setTextBackgroundColor(GLCD_WHITE); setTextColor(GLCD_VIOLET); delay(1000);}
void blue(){ setTextBackgroundColor(GLCD_BLUE); glcd(11,8, " "); glcd(12,8, " "); glcd(13,8, " "); glcd(14,8, " "); setTextBackgroundColor(GLCD_WHITE); setTextColor(GLCD_VIOLET); delay(1000);}
void magenta(){ setTextBackgroundColor(GLCD_MAGENTA); glcd(9,2, " "); glcd(10,2, " "); glcd(11,2, " "); glcd(12,2, " "); setTextBackgroundColor(GLCD_WHITE); setTextColor(GLCD_VIOLET); delay(1000);}
void white(){ setTextBackgroundColor(GLCD_WHITE); glcd(6,8, " "); glcd(7,8, " "); glcd(8,8, " "); glcd(9,8, " "); setTextBackgroundColor(GLCD_WHITE); setTextColor(GLCD_VIOLET); delay(1000);}
Explanation:
1. The colored box (at a specified position) will be displayed to the GLCD when the value being set is true (check constraints below). To understand the coordinates of the colored box specified in the program, please refer to the image above.
2. The analog value of knob was mapped from 0 - 1000 to 0 - 245. There are 7 colors that can be displayed; therefore, each color has a range of 35 (except the first constraint).
3. Constraints:
Value Color (Box)
0 - 35 - Red
36 - 70 - Yellow
71 - 105 - Green
106 - 140 - Cyan
141 - 175 - Blue
176 - 210 - Magenta
211 - 245 - White
Note: The box display is NOT perfect since it has a gap in between lines. I used spaces in this program instead of actual coordinates, to demonstrate easily how would it look like.
Also, I created functions for each box to understand the code easily.
Attachments
Step 6: You're Done!
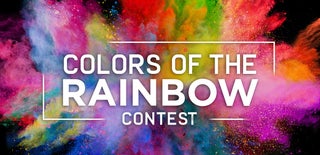
Participated in the
Colors of the Rainbow Contest