Introduction: Commute Travel Time LED Indicator
Part of a larger open source project called D.I.N.A. the at-a-glance IoT notification device. Check it out here: https://brettbuilds.myportfolio.com/dina-doineeda-...
Link to code mentioned in this instructable:
https://github.com/bhileman/yuntrafficcheck
This build will show you how to successfully use the Arduino Yun to run a python program to integrate in with the google maps matrix API and create a visual display of your commute time. The neopixel will go from green -> yellow -> red based on the range you set for good traffic to bad traffic. The function will run every 5 minutes and continue to keep you updated and let you know if you need to find a detour!
I will try to be detailed with the build involving the electrical components and getting the program running correctly, I won't be explaining too much about the enclosure. I encourage you to get creative with your own enclosure design, but just in case I will provide the STL files for my design.
You will learn:
- How to use the bridge library and the built in process functionality.
- How to do basic NEOPIXEL color changes and for loops to create a dial
- How to create a basic python script to make http get requests.
- How to parse JSON api responses
- Finally how to retrieve those responses via serial communication and display the values in a neat way!
Step 1: Hardware and Components
Electronics
- Arduino Yun
- Neopixel (ring or strip would work) in this example I use a 24 LED ring
- Minimum 1A 5V power supply
- Decent size capacitor
- SD Card (optional)
Hardware
- 3d Printed Parts
- Mainbase
- Toplid
- Laser Cut Parts
- Clearacrylic_1 and _2
- Blackacrylic_1
- Additional parts that will help with assembly
- Protoboard or small breadboard for connections
- 4mm bolts to hold toplid on
Additional
- The main version of DINA has a button because it supports multiple modes. If you would like to learn more about how to switch between modes and add a button to this build please comment.
Step 2: Wiring
If you are using the Neopixel ring you will need to solder wires onto the connections for pwr, gnd, and in.
In this example I am using pin 7 for the neopixel. Also make sure you get the polarity correct if using a polarized capacitor.
Why use a capacitor? If you are using a trusted and well made power supply you can probably get away with not using it but if you are using a cheaper supply or a large bench top supply you will want to use a capacitor to help with voltage spikes.
Step 3: Setting Up the Yun
Although probably not required for this project it is usually best to follow these instructions to expand the Yun's disk space https://www.arduino.cc/en/Tutorial/ExpandingYunDis... you will definitly want to do this if you plan on going further on the linux side trying to implement things like Nodejs.
Setting up the Arduino Yun:
First things first you need to get the Yun connected to the internet. Right out of the box if you power up the Yun it will start in what is called AP mode. In this mode the Yun is acting like it's own server allowing you to connect directly to the device via wifi.
- Turn the yun on (powered either by usb or pwr supply) find the Yun's wifi on your computer it with be "Yun-....."
- Connect to that network.
- In your browser go to http://arduino.local or if that doesn't work try 192.168.240.1
- This should pull up the yun's setup page, the default password is "arduino"
- Click configure, set a new password.
- Under the wireless parameters find your home network and enter in the network details. Finally click "Configure and Restart"
- At this point if all goes well the Arduino will automatically switch to station mode and connect to your network. To make sure it is connected wait a couple minutes then go back to your browser and enter http://arduino.local and it should bring up the same login screen. If it doesn't then it most likely didn't connect correctly. If this happens press and hold the wifi rst button on the yun for over 5 sec and it will kick back into AP mode.
- Now you are connected to the internet and ready to start coding!
Step 4: Creating the Google Maps API Integration
First you will need to get a key from Google in order to access their google maps API. In order to do this you will need to create a project, enable the API, and get a key. It is pretty straight forward once you are there and click around a bit: https://developers.google.com/maps/documentation/d...
We will specifically be using the Distance Matrix API to determine the current travel times. We are specifically going to be looking to capture the duration_in_traffic value. If you are unfamiliar with assembling the URL for the request I highly suggest downloading a software called Postman https://www.getpostman.com/ with will allow you to copy over one the examples from this page: https://developers.google.com/maps/documentation/d... run it with your key, and change parameters until you get the correct response. This software also gives you a chance to quickly see what google returns.
Here is an example of the url I am using for my request (add your key to this):
<p><a href="https://maps.googleapis.com/m" rel="nofollow">https://maps.googleapis.com/m</a>aps/api/distancematrix/json?units=imperial&origins=10+1st+St+Austin+Texas+7874&destinations=20+University+Pkwy+Round+Rock+Texas+78681&traffic_model=best_guess&key="ADD YOUR KEY HERE, DELETE THE QUOTES"&departure_time=now</p>
So now we need to put this into a python function that we will run on the Linux portion of the Yun. This part is actually very simple because this type of API call requires no authorization.
import urllib2<br>import json import sys response = urllib2.urlopen('ADD THE URL FROM ABOVE HERE WITH YOUR PARAMETERS AND KEY') data = json.load(response) ttime = data['rows'][0]['elements'][0]['duration_in_traffic']['value'] print (ttime) sys.exit()
Now you need to get this python file uploaded into the Yun. The best way to do this on windows is using WinSCP. https://winscp.net/eng/download.php Once you have WinSCP installed, connect to the Yun with SCP protocol, host-name: arduino.local, and port 22. You should be prompted to log in using root and the password you setup prior.
Once logged in navigate to /mnt/sda1/arduino/www/python and then upload your python file to this folder. This assumes that you have an SD card mounted. If not, then you can put the file in a different folder (ex. root) and change the code in the next step to reflect that different path.
Attachments
Step 5: Programming the Atmel Code Using Arduino IDE
The comments in this program do a pretty good job at explaining how this program works. To upload this sketch open the Arduino IDE and either connect to your Yun using a virtual port or USB. These options will show when you go to tools->port. If you don't see anything here check the usb connection or ensure that your yun is connected to the same wifi network.
You may need to add some of these libraries (neopixel for sure) before you can successfully execute the code. To do this go to sketch -> include library -> manage libraries. Then add the libraries list at the top of the sketch file attached.
This program will do the following:
- Initiate a bridge process with the linux processor, send a request to run the python code at startup and every 5 minutes.
- It will then monitor the serial communication and save any value that comes across into an array. This array is set to a variable and is then run through values specified for time ranges.
- It will run a fun loading screen while that python code is running, it will clear all the LEDs, and then run the full color with dial mode.
- This mode will create a color that corresponds the to the ranges specified. Usually green for low time, red for longest, and several colors in between.
- This program will also turn the neopixel into a dial and only change pixels up to a certain level based on the time returned from the check.
Enjoy not getting stuck in traffic!
Attachments
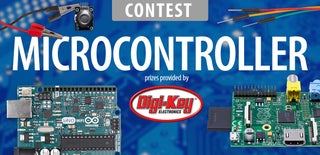
Runner Up in the
Microcontroller Contest 2017
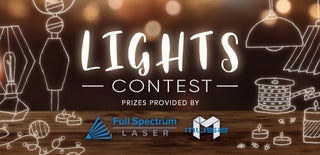
Participated in the
Lights Contest 2017