Introduction: Contactless Door Opener (vSTEM)
Wanna hear something surprising? Your average door handle can have up to 400x more bacteria than your toilet. That's right - 400!
Researchers at the University of Arizona found that "everyday door handles are often hotspots for bacteria". Being used so frequently and by so many people, it's only reasonable to assume that bacteria is present on handles - but 400x more than your toilet?! As a senior in high school, this fact surprised me and I expect it's probably not something you wanted to hear either, especially in the midst of a pandemic, but there may be some hope - in the form of this Instructable.
This contactless door unlocker automatically unlocks and opens your door once it senses your hand. Using an Arduino, a distance sensor and a servo, this project is relatively easy to set-up and doesn't require too much prior knowledge.
Ready to embrace the new, contactless normal? Then let's get started with this project!
Supplies
For this project, you will need:
- Arduino Uno
- MG 996R High Torque Servos
- HC-SR04 Distance Sensors
- Assortment of Jumper Wires
- Breadboard
- Lots of Duct Tape
- Screw Driver
- Zip Ties
- Spring (Optional)
- USB Wall Charger & USB 2.0 Cable A/B
You probably already have a lot of these tools, but I linked them all just in case.
Step 1: Put the Circuit Together
Let's begin by putting this circuit together. Look at the schematic above and try following along. Here are the main pin connections:
- Servo
- GND to GND
- VCC to 5V
- Signal to Pin 3
- Distance Sensor
- GND to GND
- VCC to 5V
- Trig to Pin 12
- Echo to pin 11
We'll be using the breadboard to apply power to both the distance sensor and the servo using the Arduino's 5V and Ground Pins.
We'll be powering the system using the USB A/B cable and a USB Wall Power adapter (the kind that usually comes with your phone). Before we get into that, however, let's upload the code.
Step 2: Write & Upload the Code
The code for this project is relatively simple. Simply copy & paste it into the Arduino IDE and upload it to your Arduino using the USB A/B Cable.
#include <Servo.h> #define trigPin 12 #define echoPin 11 int servoPin = 3; Servo Servo1; void setup() { Servo1.attach(servoPin); pinMode(trigPin, OUTPUT); pinMode(echoPin, INPUT); } void loop(){ digitalWrite(trigPin, LOW); delayMicroseconds(2); digitalWrite(trigPin, HIGH); delayMicroseconds(10); digitalWrite(trigPin, LOW); long duration, distance; duration = pulseIn(echoPin, HIGH); distance = (duration/2) / 29.1; if (distance < 10){ Servo1.write(90); } else { Servo1.write(0); } }
Explanation of the program:
Essentially, we begin by including the Servo Library. The following lines then define pin's 11 and 12 as the echo & trig pins and pin 3 as the servo's signal pin. Finally, we create a Servo object entitled Servo1.
In the setup section of the program we attach Servo1 to pin 3 or the 'servo pin'. We set the trigPin (Pin 12) as an output and the echoPin (Pin 11) as an input.
The loop section is where our program will be running. We set the trigPin (or trigger pin) to High for 10 microseconds, creating an 8-pulse wave pattern. The echoPin then waits for the pulses to be reflected back. If they are not reflected back after a period of 38 microseconds, the program loops back to the start. If they are reflected back, we can calculate the distance using both the duration and the speed of sound. We can find the duration using the pulseIn function. If we want to find the distance, we have to divide the duration by 2 (as the initial duration value is the time it took to both emit the pulse and reflect it). Then we multiply that by the speed of sound (1/29.1) - which is essentially the same as dividing by 29.1. The distance is returned in centimeters.
The final step involves an if statement which checks to see if the distance between your hand and the sensor is less than 10 centimeters. If it is, the servo will move to 90 degrees (essentially unlocking the door). If it isn't, it will move back to the locked position - at 0 degrees.
Once you've uploaded the code, test it out. If the servo rotates when you bring your hand nearby, you've done it correctly - Congratulations. Now let's attach it to our door!
Step 3: Set Up Your Circuit
Now that you have the circuit made and have the code uploaded, let's make it easier to attach your circuit onto your door.
Here is where the zip ties will come in handy. I only had to use 2, but depending on the size of your breadboard you may have to use more. We'll use these zip ties to attach the Arduino, Distance Sensor and Breadboard to each other, creating a singular unit of sorts. The servo will remain separate as this will make it easier to position it. I took one zip tie and twisted it around the Arduino and the breadboard. Once locked in, I took another and twisted it around the Distance Sensor and the breadboard. Ensure that there are spaces between and around the assembly. This creates a compact and lightweight assembly that (as you'll see in the coming steps) was fairly easy to attach on the door.
We'll also need a way to power the circuit up. I used the USB A/B cable (the same one you uploaded the program with) in combination with a USB Wall Charger. Don't plug it into the arduino just yet, but keep it ready for now.
The final step (if you haven't done this already) is to add the 2-pronged servo horn atop the Servo. If you used the Servo's I linked, the horn should have come with it. If you are using your own Servo, you may have to purchase a similar one. A 1-pronged horn is also usable. We use a 2-pronged servo as opposed to a 3 or 4-pronged one because it allows for just 2 states - locked and unlocked - controlled easily by moving the servo from 0 to 90 degrees or from 90 to 0. Keep the 3 Servo Wires unplugged for now. We'll be plugging them back in once we've secured the circuit onto the door.
You're almost complete! In the next step, we'll be setting up our door and making it easier to attach the circuit.
Step 4: Set Up Your Door
We want to set up our circuit as the singular mechanism that allows you to open your door, which means we will have to remove all handles and locks that are already on our door (these are generally pretty easy to remove and put back). If you don't feel comfortable disassembling your door, don't worry - you can use a little duct tape and achieve a similar result.
Let's begin by unscrewing the door handles. This is pretty easy to do (and if you decide you don't want to keep this project on your door, pretty easy to screw back in as well). There are generally four screws you have to remove. I suggest removing the two connecting the latch first. Once that has been removed, take out the two screws connecting both knobs together. Once out, you should see a hole in your door where the handle once was. You can screw the two knobs back inside to plug up that hole, but be sure to leave the latch out. That being said, I actually didn't bother to plug the hole back up.
Like I mentioned before, if you feel uncomfortable disassembling your door, you can use duct tape instead. This method will not be as smooth and it will probably come undone easy. That being said, if you are just doing this for fun/temporarily, this will probably suffice. Simply tape the latch down with a few layers of duct tape. Make sure you use enough to keep the latch from popping up, but also ensure there is enough space between the door and the door-frame - so that the door can still freely open.
This final part is optional, and I actually wasn't able to implement it perfectly. If you did find a better method, let me know in the comments below! I was attempting to use a spring to have the door pop open the second I unlocked it, thus making it completely contactless. Several springs either fell off the door frame or broke, and I ultimately decided to simply kick the door open once it was unlocked.
Now that we have the door prepared, let's attach our circuit.
Step 5: Put Everything Together
This step is going to involve Duct Tape - a lot of it. I was worried that I may have to screw the circuit in, or worse - glue it onto my door, but Duct Tape seemed to do the job extremely well. In fact, after having built this project a week ago, both the servo and the circuit assembly are still firmly secured.
Using the spaces you created previously, tape down the circuit towards the center of your door. Ensure you have placed it such that the Servo can still easily connect to it. Also be sure that you can easily place your hand in front of the distance sensor. Once you have the circuit assembly taped down, tape the servo near the edge of the door. The servo horn should be placed in such a way that the door doesn't open when it is in its locked position. Now that you have both of these taped onto the door, plug the Servo back into the Arduino (Signal --> Pin 3, VCC to 5V, GND to GND)
Once you have everything taped down in a neat way, bring your power source (the USB A/B Cable) and plug it in to your wall adapter. Then, plug in the 'B' side into the Arduino. It should light up immediately. Try putting your hand in front of the distance sensor. If the servo rotates and the door unlocks, you're done! Congratulations - you're well on your way toward avoiding 'door handle bacteria'!
Step 6: You're Done!
Nice job! You now have a contactless door opener on your very own door! Check out the video above if you're confused as to how the project should work or want to make sure you did it right.
Looking for ways to make life in the pandemic easier? Check out some of my other COVID-19-related projects like the 'Alexa song lyrics finder'. I'm working on uploading new projects every few weeks, so be sure to keep a lookout for them!
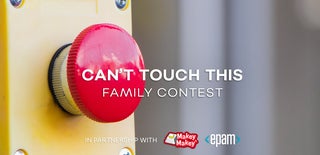
Participated in the
"Can't Touch This" Family Contest