Introduction: Countdown Timer Prop
I was asked if I could make a “launch control console” for a schools play area. The most important part of a rocket launch is the countdown so I needed a countdown timer. This instructable shows how I made a large 10 second countdown timer for approximately than £10 with only basic tools.
First the disclaimer, this is the first electronics project I have attempted so there may be better ways to run the display and better code, but this did the job and that’s half the battle.
Step 1: Gather Your Materials
- 1 m of 5 v USB led strip 3528 ( I bought mine of ebay for ~£3) 6 LEDs per meter cuttable at every LED
- Stiff card for back of display
- Polystyrene packing ~1 cm thick (mine was free, saved from some flat-pack furniture)
- 2 sheets of velum for the diffuser (available from craft stores)
- Arduino Nano (~£2.50 from ebay)
- 1 on/off double pole push button (~1.50 ebay)
- Dupont wire for connections to nano (not strictly necessary but makes life easier)
- Wire
- Soldering iron
Step 2: Create the Light Guide
Print the LED digits on a piece of card. There are many free digital style fonts available on the web I picked the simplest I could find and printed it at 20 cm high.
(The Arduino can supply enough current to run 4 LEDs per pin which means you can have the display what ever size you like, but you will be limited to 4 LEDs which will limit the brightness. If you want more LEDs you will need to use transistors to switch the segments. There are lots of web tutorials showing you how to do this)
Next cut out the segments of the display.
You can now use this as a template for the polystyrene. Use double sided tape to stick the card to the polystyrene, then use a very sharp knife (I find the disposable DIY knives best) to cut out each segment from the polystyrene.
Step 3: Set Out the LEDs
Make a base for the display, I used a piece of cardboard mount, but any stiff material will do (MDF, of hard board, etc). Using the polystyrene light guide you just made mark the base to show where the LEDs go.
Cut the LED strip into 4 LED segments and use the self adhesive backing to stick them onto the segments.
Step 4: Wiring
Now the tricky bit, wiring.
I’m using a common anode so each –ve terminal needs to soldered in a daisy chain with a single wire for the anode.
Each LED strip needs a separate wire which will go to an individual pin on the Arduino. It’s very important that you label each wire or you’ll spend a happy hour testing each wire to find out which is which. (the diagram shows the pin on the Arduino that each segment was connected to)
Depending on exactly which Arduino you’re using will determine how you connect the wires to the pins. The nano I used required each wire to have a female Dupont connect. You can buy female/female dupont wires, cut them in half and use each end to attach to the segment wires.
Step 5:
Glue the polystyrene light guide to the base board covering the LED’s and wiring. (Double sided sticky tape works well, if you use glue check with a scrap of polystyrene to make sure it doesn’t dissolve it). Attach the diffuser. I found two layers of craft vellum was sufficient to diffuse the light enough so you can’t see the individual LED’s. If you want to tidy up the display you can top the display off with another piece of card like the one you prepared before. (it’s not necessary for the display to work, but if you’re a neat freak like me it helps tidy things up).
Step 6: Attache the Start Button
I used an emergency stop switch because it looked like the sort of thing you should have to launch a rocket. You can use any NO/NC (normally open / normally closed) switch. This type of button has two connections which are switch when the button is pressed. One side of the switch is connected to an Arduino pin. The normally connected terminal is connected to ground via a 100 ohm resistor. The normally open terminal is connected to + ve. When the button is pressed the Arduino sees a “high” signal and starts the countdown. (there’s more information on the Arduino web site)
Step 7: Programme the Arduino
The code I used is probably not the most efficient way to have a count down timer but it works well enough. The code lights each segment for the necessary number each second.
const int buttonPin = 2; // the number of the pushbutton pin
const int ledPin = 13; // the number of the LED pin
// variables will change:
int buttonState = 0; // variable for reading the pushbutton status
void setup() {
// initialize the LED pin as an output:
pinMode(ledPin, OUTPUT);
// initialize the pushbutton pin as an input:
pinMode(buttonPin, INPUT);
pinMode(13, OUTPUT);
pinMode(12, OUTPUT);
pinMode(11, OUTPUT);
pinMode(10, OUTPUT);
pinMode(9, OUTPUT);
pinMode(8, OUTPUT);
pinMode(7, OUTPUT);
pinMode(5, OUTPUT);
pinMode(6, OUTPUT);
pinMode(3, OUTPUT);
}
void loop() {
digitalWrite(6, HIGH); // turn the LED on (HIGH is the voltage level)
digitalWrite(5, HIGH);
digitalWrite(7, HIGH);
digitalWrite(8, HIGH);
digitalWrite(9, HIGH); // turn the LED on (HIGH is the voltage level)
digitalWrite(10, HIGH);
digitalWrite(11, HIGH);
digitalWrite(12, HIGH);
// read the state of the pushbutton value:
buttonState = digitalRead(buttonPin);
// check if the pushbutton is pressed.
// if it is, the buttonState is HIGH:
if (buttonState == HIGH)
{
digitalWrite(6, HIGH); // turn the LED on (HIGH is the voltage level)
digitalWrite(5, HIGH);
digitalWrite(7, HIGH);
digitalWrite(8, HIGH);
digitalWrite(9, HIGH); // turn the LED on (HIGH is the voltage level)
digitalWrite(10, HIGH);
digitalWrite(11, HIGH);
digitalWrite(12, HIGH);
delay(1000);
digitalWrite(6, LOW); // turn the LED on (HIGH is the voltage level)
digitalWrite(5, LOW);
digitalWrite(7, LOW);
digitalWrite(8, LOW);
digitalWrite(9, LOW); // turn the LED on (HIGH is the voltage level)
digitalWrite(10, LOW);
digitalWrite(11, LOW);
digitalWrite(12, LOW);
// turn LED on:
digitalWrite(8, HIGH);
digitalWrite(9, HIGH); // turn the LED on (HIGH is the voltage level)
digitalWrite(10, HIGH);
digitalWrite(11, HIGH);
digitalWrite(12, HIGH);
digitalWrite(13, HIGH);
delay(1000);
digitalWrite(8, LOW);
digitalWrite(9, LOW); // turn the LED on (HIGH is the voltage level)
digitalWrite(10, LOW);
digitalWrite(11, LOW);
digitalWrite(12, LOW);
digitalWrite(13, LOW);
digitalWrite(7, HIGH);
digitalWrite(8, HIGH);
digitalWrite(9, HIGH); // turn the LED on (HIGH is the voltage level)
digitalWrite(10, HIGH);
digitalWrite(11, HIGH);
digitalWrite(12, HIGH);
digitalWrite(13, HIGH);
delay(1000);
digitalWrite(7, LOW);
digitalWrite(8, LOW);
digitalWrite(9, LOW); // turn the LED on (HIGH is the voltage level)
digitalWrite(10, LOW);
digitalWrite(11, LOW);
digitalWrite(12, LOW);
digitalWrite(13, LOW);
digitalWrite(9, HIGH); // turn the LED on (HIGH is the voltage level)
digitalWrite(10, HIGH);
digitalWrite(11, HIGH);
delay(1000);
digitalWrite(9, LOW); // turn the LED on (HIGH is the voltage level)
digitalWrite(10, LOW);
digitalWrite(11, LOW);
digitalWrite(7, HIGH);
digitalWrite(8, HIGH);
digitalWrite(9, HIGH); // turn the LED on (HIGH is the voltage level)
digitalWrite(11, HIGH);
digitalWrite(12, HIGH);
digitalWrite(13, HIGH);
delay(1000);
digitalWrite(7, LOW);
digitalWrite(8, LOW);
digitalWrite(9, LOW); // turn the LED on (HIGH is the voltage level)
digitalWrite(11, LOW);
digitalWrite(12, LOW);
digitalWrite(13, LOW);
digitalWrite(8, HIGH);
digitalWrite(9, HIGH); // turn the LED on (HIGH is the voltage level)
digitalWrite(11, HIGH);
digitalWrite(12, HIGH);
digitalWrite(13, HIGH);
delay(1000);
digitalWrite(8, LOW);
digitalWrite(9, LOW); // turn the LED on (HIGH is the voltage level)
digitalWrite(11, LOW);
digitalWrite(12, LOW);
digitalWrite(13, LOW);
digitalWrite(9, HIGH); // turn the LED on (HIGH is the voltage level)
digitalWrite(10, HIGH);
digitalWrite(12, HIGH);
digitalWrite(13, HIGH);
delay(1000);
digitalWrite(9, LOW); // turn the LED on (HIGH is the voltage level)
digitalWrite(10, LOW);
digitalWrite(12, LOW);
digitalWrite(13, LOW);
digitalWrite(8, HIGH);
digitalWrite(9, HIGH); // turn the LED on (HIGH is the voltage level)
digitalWrite(10, HIGH);
digitalWrite(11, HIGH);
digitalWrite(13, HIGH);
delay(1000);
digitalWrite(8, LOW);
digitalWrite(9, LOW); // turn the LED on (HIGH is the voltage level)
digitalWrite(10, LOW);
digitalWrite(11, LOW);
digitalWrite(13, LOW);
digitalWrite(7, HIGH);
digitalWrite(8, HIGH);
digitalWrite(10, HIGH);
digitalWrite(11, HIGH);
digitalWrite(13, HIGH);
delay(1000);
digitalWrite(7, LOW); // turn the LED on (HIGH is the voltage level)
digitalWrite(8, LOW);
digitalWrite(10, LOW);
digitalWrite(11, LOW);
digitalWrite(13, LOW);
digitalWrite(9, HIGH); // turn the LED on (HIGH is the voltage level)
digitalWrite(10, HIGH);
delay(1000); // wait for a second
digitalWrite(9, LOW); // turn the LED on (HIGH is the voltage level)
digitalWrite(10, LOW);
digitalWrite(7, HIGH);
digitalWrite(8, HIGH);
digitalWrite(9, HIGH); // turn the LED on (HIGH is the voltage level)
digitalWrite(10, HIGH);
digitalWrite(11, HIGH);
digitalWrite(12, HIGH);
delay(1000);
digitalWrite(3, HIGH);
delay(200);
digitalWrite(3, LOW);
delay(200);
digitalWrite(3, HIGH);
delay(300);
digitalWrite(3, LOW);
delay(300);
digitalWrite(3, HIGH);
delay(200);
digitalWrite(3, LOW);
delay(200);
digitalWrite(3, HIGH);
delay(2000);
digitalWrite(3, LOW);
digitalWrite(3, HIGH);
delay(200);
digitalWrite(3, LOW);
delay(200);
digitalWrite(3, HIGH);
delay(300);
digitalWrite(3, LOW);
delay(300);
digitalWrite(3, HIGH);
delay(200);
digitalWrite(3, LOW);
delay(200);
digitalWrite(3, HIGH);
delay(200);
digitalWrite(3, LOW);
delay(200);
}
}
The 7 segment display can be used to make any device needing a large display like a clock. The Arduino only has enough pins to run 10 segments, to run more you will need to use multiplexing, there are plenty of tutorials on the web to show you how to do this.
Step 8: The Final Result
Once the countdown timer was ready I incorporated it into a "Launch console" with a few extra switches and lights for the kids to play with.
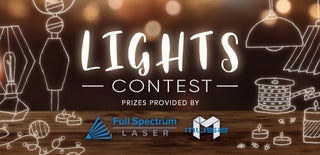
Participated in the
Lights Contest 2017