Introduction: Cubic Art
This project is for an Arduino powered 4x4x4 cube of RGB LEDs. I have seen other LED cube projects using mono-colored LEDs and Charlieplexed RGB LEDs. This one is different in that it uses APA106-F5 addressable LEDs. An APA106-5F LED is similar to the well known WS2812 LED in that it is an RGB LED that can be daisy changed and driven by a single pin on the Arduino. Where the APA106-F5 differs from the WS2812 is in the package style and the communications timing. The APA106-F5 used in this project is in a 5mm domed, frosted package. Fortunately, the Adafruit Neopixel driver can drive the APA106-F5 LEDs the same as it does the WS2812. The domed, frosted package allows the colors to mix within the dome, thus providing better colors for each individual LED. I have included a rotary encoder with a push-button switch. The rotary encoder allows the user to vary the maximum brightness of the cube and/or the speed with which some of the patterns move. Selection is made by pressing down on the control knob. When the indicator LED is green, the brightness is set by rotating the knob. When the indicator is red the speed is set by rotating the knob. When the indicator is off the the knob is disconnected, turning it has no effect. The software for this project was developed in C++ using the Arduino IDE. C++ was used to take advantage of some of the features of object oriented programming, namely inheritance and overloading. The code was written to use a minimum amount of RAM. As much as possible, initial values were pushed into the Flash memory and copied to RAM when needed. Being an older nerd, I like to think of this as the 21st century equivalent of the Lava Lamp. Make one for yourself, sit back and get mesmerized.
Make no mistake, this is a complicated project. I have tried to simplify it as much as possible. You do not need to know anything about C++ to use the provided software. In some later steps I explain how the software works and how to add your own visual effects to the cube. The most complicated part of this project is wiring up the APA106-F5 LEDs into the 4x4x4 cube. You will need good soldering skills to make a good looking, functioning cube. The build has been simplified by assembling four individual 4x4 grids of LEDs (one layer of the cube) and then wiring all the layers together.
If you are interested in building one of these and do not have access to a 3D printer, contact me. I have the 3D printed jig that I used to build my cube with. I will send it to one lucky individual.
If you need help understanding how the code works or with implementing a new Action, contact me.
Step 1: What You Will Need
- An Arduino board.
- Any 5V Arduino board will do.
- The code was written for an Arduino Uno (Atmega328).
- An Uno or Leonardo (AtMega32U4) will work.
- I used an Arduino ProMini due to its small size and low cost
- An Arduino Nano will also work, but you will need an Arduino compatible USB-to-Serial bridge to program it with.
- 65 APA106-F5 LEDs in the 5mm, domed, frosted package.
- 100 APA106-F5 LEDs can be bought from Aliexpress for under $20.00.
- 64 APA106-F5 LEDs are used for the 4x4x4 cube.
- The 65th APA106-F5 LED is used for the mode indicator.
- You could get them with an 8 mm frosted dome.
- 100 APA106-F5 LEDs can be bought from Aliexpress for under $20.00.
- One Arduino rotary encoder
- You can also find this on Aliexpress as a two-for around $1.50.
- A 5V, 4Amp power supply.
- A Power jack that mates with the connector on your power supply.
- A power switch.
- I used a small rocker switch that I bought on ebay.
- You could use any switch you like; toggle, rocker or latching push button.
- Or eliminate the power switch and just unplug it to turn it off.
- Hookup wires.
- 22 awg stranded copper wire in various colors, red, black and another color would be nice.
- Used to wire things to the Arduino board.
- Bare, tinned, 22 awg solid copper wire for wiring the APA106-F5 LEDs together.
- 4 pieces 8 1/4" long
- 4 pieces 6 1/4" long
- 4 pieces 2 3/4" long
- 8 pieces 3" long
- Two pieces of 18 awg stranded wire.
- Red and Black, used to wire power to the 4x4x4 LED cube.
- 18 awg is used to power the cube to keep the voltage drop down when all the LEDs are lit up.
- Alternatively, you could use a pair of 22 awg wires in place of the 18 awg wires.
- 22 awg stranded copper wire in various colors, red, black and another color would be nice.
- Solder
- A baseball display case, see photos above.
- I got mine from my local Micheal's store.
- Only the clear plastic top is used, the base is not used.
- It is approximately a 3.5 inch clear plastic cube.
- Optional Parts - these are optional, you can always just solder the wires to the boards
- 1 - DuPont style 8 pin shell.
- 1 - DuPont style 4 pin shell
- 1 - DuPont style 5 pin shell.
- 17 - DuPont female crimps.
- 2 - 15 pin right angle male headers.
All of the Arduino source code files, 3D printing files and wiring diagrams can be download from GitHub; Here.
Step 2: Required Tools
- Soldering iron with a small tip
- Wire strippers for 18 awg and 22 awg wires
- Wire cutters
- Small needle nose pliers
- Pliers or nut driver that fits the nut on the power jack
- Optional 3D printer
- I strongly suggest that you 3D print the the LED Soldering Jig.
- I have provided the OpenSCAN source file and an STL file for the jig.
- If you do not have a 3D printer then try to find a friend who can print it for you.
- An alternative to the 3D printed soldering jig would be to make a soldering jig from a 5x5x3/4 inch block of plywood or MDF.
- Layout a set of grid lines and drill a 5mm () hole where the grid lines intersect.
- The grid lines should be 17mm apart.
- I have included a PDF file that can be printed on a laser or inkjet printer that will print the grid for you. After printing, measure to insure that the grid spacing is close to 17mm. If the grid spacing is not close to 17mm then try scaling the image and print it again. The printed grid can be glued to a wood block and then the LED holes drilled. The printed grid also has outlines for the +5V power and Ground rails.
- Six 3D printed spacer blocks.
- These are used to hold and evenly space the LED layers while soldering them together.
- Alternatively, you can make six 0.5x0.5x3.5 inch wood spacer blocks
- These are used to hold and evenly space the LED layers while soldering them together.
- A 3D printed or wooden base to house the electronics for your LED cube.
- I have provided the OpenSCAD source and STL files for 3D printing a base for your LED cube. If you are using a different switch, baseball display case or power jack than what I used then you will have to modify the OpenSCAD source file to change the openings for theses parts.
Step 3: Building the LED Cube: 3D Print the Bending/Soldering Jig and Spacers
Do not attempt to make this project without an LED soldering jig. It is impossible to keep the rows and columns of LEDs straight and flat without one. The 3D printed jig that I have designed has the added advantage of providing supports and spacing for the power and ground rails of each layer. Using my 3D printed jig will make it a lot easier to construct each LED layer the same, which will result in a much nicer looking cube when you are finished. Same goes for the six 3D printed spacers. Trying to solder the layers together without the spaces will result in a lopsided. uneven cube.
If you do not have a 3D printer, then try to find someone with a 3D printer that will print the jig and spacers for you or send it out to a 3D printer farm and have them printed for you.
The first photo above shows the 3D printed Bending/Soldering jig.
The second photo above shows the spaces fresh off the 3D printer. The easy lift tabs have not been removed yet.
The third photo above shows the spacers after the easy lift tabs have been removed.
Included in the download from GitHub is a folder containing the files required to 3D print the Bending/Soldering jig, the spacers and the 3D printed base for your finished 4x4x4 LED cube.
- LedCubeJig.scad is the OpenSCAD source file for the Bending/Soldering jig and spacers.
- LedCubeJig.stl is an STL file for 3D printing the Bending/Soldering jig.
- Spacers.stl is an STL file for 3D printing the spacers only.
- LedCubeBase.scad is the OpenSCAD source file for the 3D printed base, top and knob for your LED cube.
- Bottom.stl is an STL file for 3D printing the LED cube base.
- Top.stl is an STL file for 3D printing the top of the LED cube base.
- Knob.stl is an STL file for 3D printing the knob for the rotary encoder.
- All.stl is an STL file for 3D printing all of the case parts at the same time.
You can edit the .scad files to 3D print only the parts you need or to modify the files to accommodate any changes you make. For instance, if you use an Arduino board with a different physical outline then you will want to edit the LedCubeBase.scad file to use a different board outline. Same for if you use a different power switch or power jack socket. Edit LedCubeTop.stl if you need to move the mounting points for your finished LED cube.
OpenSCAD is freeware that you can download here.
If you simply cannot get the 3D parts printed then you can make a jig and spacers from some scraps of wood.
- Step 31 details what you will need and provides instructions for making your own jig.
- Step 32 details what you will need and provides instructions for making your own spacers.
Once you have a bending jig you can begin. The spacers are used to hold the layers while you wire them together.
Step 4: About the APA106-F5 LEDs and How the They Are Wired
Each APA106-F5 has a Red, Green and Blue LED and a controller built into it. As such, you cannot use an Ohmmeter to test for polarity like you would with a regular LED. The only way to light one up is to provide +5V, Ground and 24 bits of asynchronous serial data with the proper timing to it. The download package from GitHub contains an Arduino sketch named LEDTest.ino that you can use to test each LED layer as you assemble it. I strongly suggest that you burn this sketch into an Arduino board and keep it handy while doing the assembly of each LED layer. It is almost impossible to replace an LED that was soldered in backwards in the middle of the 4x4x4 cube. I suggest that you solder one column of LEDs and then test them. Solder the next column and test again. Continue adding columns and testing until your layer is done. Now that you have a known good layer, put it aside and assemble another. Following this method it should be fairly easy to find and replace an LED that was soldered in backwards before it becomes inaccessible.
Note: The APA106-F5 is an addressable LED, similar to a WS2812, the entire LED cube is wired as a single daisy chain of 64 APA106-F5 LEDs. This means that the Dout of the first LED is wired to the Din of the next LED and so on for each column of one layer. The Dout of the last LED in a column is wired to the Din of the last LED in the adjacent column. The result is a serpentine wiring of the LEDS of each layer. The odd numbered layers are wired the same. The even numbered layers are also wired the same, but are wired differently than the odd numbered layers. You will be assembling two odd numbered layers and two even numbered layers. The techniques used to assemble each layer are the same. The only differences are in how the leads of the LEDs are bent and where the first LED of the layer is placed.
To keep things simple, you will assemble and test one layer at a time. Each layer has several steps. You will repeat these steps to make two identical odd layers. Then move on to make two of the even layers. When all of the layers are assembled then the layers are assembled into the cube shape.
As long as you follow the assembly directions correctly, you will end up with a working cube that is wired properly to work with the cube control software. If you reverse the order of the layers or do not assemble the layers as directed, the LED mapping will be wrong and the programmed visual effects will not look correct.
The rest of the assembly steps assume that you are using the 3D printed bending/soldering jig. If you are using a handmade jig then the procedures are the same. You will just not have the nice wire guides that the 3D printed jig provides.
Step 5: Bending the End of Column LEDs, Part 1
Each layer of the cube has the LEDs wired in a serpentine fashion. The end of each column connects to the end of the adjacent column. In the this step you will be bending the Dout leads of six LEDs. Four of these LEDs will be used for the even numbered layers and two will be used by the odd numbered layers. You do this now because it is easier to make these bends while the 3D printed LED Bending/Soldering jig is empty.
- Position the jig in front of you with the 3D printed word 'Front' away from you as shown in the first photo above.
- Place an LED into the second LED holder from the left in the row closest to you.
- The two long leads should be closest to you and all the leads should line up with the narrow slot in the top of the LED holder. See second photo above.
- While gently pressing down on all the leads to keep the LED in the LED holder, bend the long lead that is closest to you towards you, until the lead forms a right angle to the LED holder and is in the narrow slot. See third photo above.
- Bend the long lead again, to the right, keeping it close to the LED holder. See fourth photo above.
- Gently remove the LED from the jig and place it aside.
- This is an LED with Dout bent to the right. See fifth photo above.
- Repeat steps 2 through 6 until you have six LEDs with Dout bent to the right.
Step 6: Bending the End of Column LEDs, Part 2
- Position the jig in front of you with the 3D printed word 'Front' away from you as shown in the first photo above.
- Place an LED into the second LED holder from the left in the row closest to you.
- The two short leads should be closest to you and all the leads should line up with the narrow slot in the top of the LED holder. See second photo above.
- While gently pressing down on all the leads to keep the LED in the LED holder, bend the short lead that is closest to you towards you, until the lead forms a right angle to the LED holder and is in the narrow slot. See third photo above.
- Bend the short lead again, to the right, keeping it close to the LED holder. See fourth photo above.
- Gently remove the LED from the jig and place it aside.
- This is an LED with Din bent to the right. See fifth photo above.
- Repeat steps 2 through 7 until you have six LEDs with Din bent to the right.
Step 7: Bending the End of Column LEDs, Part 3
- Position the jig in front of you with the 3D printed word 'Front' away from you as shown in the first photo above.
- Place an LED into the third LED holder from the left in the row closest to you. The two long leads should be closest to you and all the leads should line up with the narrow slot in the top of the LED holder. See second photo above.
- While gently pressing down on all the leads to keep the LED in the LED holder, bend the long lead that is closest to you towards you, until the lead is perpendicular to the LED holder and is in the narrow slot. See third photo above.
- Bend the long lead again, to the left, keeping it close to the LED holder. See fourth photo above.
- Gently remove the LED from the jig and place it aside.
- This is an LED with Dout bent to the left. See fifth photo above.
- Repeat steps 2 through 6 until you have six LEDs with Dout bent to the left.
Step 8: Bending the End of Column LEDs, Part 4
- Position the jig in front of you with the 3D printed word 'Front' away from you as shown in the first photo above.
- Place an LED into the third LED holder from the left in the row closest to you. The two short leads should be closest to you and all the leads should line up with the narrow slot in the top of the LED holder. See second photo above.
- While gently pressing down on all the leads to keep the LED in the LED holder, bend the short lead that is closest to you towards you, until the lead is perpendicular to the LED holder and is in the narrow slot. See third photo above.
- Bend the short lead again, to the left, keeping it close to the LED holder. See fourth photo above.
- Gently remove the LED from the jig and place it aside.
- This is an LED with Din bent to the left. See fifth photo above.
- Repeat steps 2 through 6 until you have six LEDs with Din bent to the left.
You have finished bending the End of Column LEDs. You should have 24 LEDs with one lead bent to the right or left.
Step 9: Assembly of an LED Layer: Add the +5V Bus
You will need one each of the 8 1/4", 6 1/4" and 2 3/4" pieces of bare, tinned, 22 awg copper wire. The 8 1/4" piece of bare wire will be wrapped around the outside of the 3D printed Bending/Soldering jig to form the +5V Bus. The 6 1/4" piece of bare wire will be wrapped around the inside of the 3D printed jig to form the Ground Bus. The 2 3/4" piece is routed down the center of the 3D printed jig to extend the +5V Bus.
- Orient the 3D printed Bending/Soldering jig in front of you with the word 'Front' to your left.
- Straighten the 8 1/4" piece of bare wire as much as you can.
- Using the 8 1/4" piece of bare wire, insert it into the slots in the square wire guides on the side of the jig closest to you. The wire is a little longer than it needs to be. It should extend about 1/4" inch past the right most square wire guide. See first photo above.
- Using the round wire bending post on the left side, bend the wire away from you until it is perpendicular and fits into the square wire guides along the left side of the jig. See second photo above.
- Using the round wire bending post on the left side that is furthest from you, bend the wire until it forms a 'U' shape and fits into the square wire guides along the edge of the jig furthest from you. See third photo above.
- The wire should extend a little past the last square wire guide.
- Make sure that the wire is seated in the square wire guides and is a flat and straight as you can get it.
If you are using a handmade jig then the 8 1/4" wire is bent to follow the red line around three sides of the jig. Try to follow the line as closely as you can. This wire will provide +5 volts for the layer and is used for connecting layers together. The more uniform this wire is from layer to layer, the better your final cube will look.
Step 10: Assembly of an LED Layer: Add the Ground Bus
In this step you will be adding the Ground bus to the Bending/Soldering jig. You will be using the 6 1/4" piece of bare, tinned, 22 awg copper wire.
- Straighten the 6 1/4" piece of bare wire as much as you can.
- Butt the end of the wire up against the square wire guide that is closest to you on the left side.
- Lay the wire into the slots of the first empty row of square wire guides. See first photo above.
- Using the round wire bending post on the right side of the row, bend the wire away from you until it is perpendicular and fits into the single square wire guide on the right side. See second photo above.
- Using the round wire bending post on the right side of the fourth row of square wire guides, bend the wire around the post until forms a 'u' shape and fits into the slots of the fourth row of square wire guides. See third photo above.
- If the wire extends beyond the last square wire guide (the one holding the +5V bus) then trim it to be just short of the square wire guide.
- Make sure that the wire is as straight and flat as you can get it and is being held by the square wire guides.
If you are using a handmade jig then the 6 1/4" wire is bent in a 'U' shape to follow the green line on the jig. It should not contact the wire that follows the red line. Try to follow the line as closely as you can. This is the ground wire for the layer and is used for connecting layers together. The more uniform this wire is from layer to layer, the better your final cube will look.
Step 11: Assembly of an LED Layer: Finish the +5V Bus
In this step you will be finishing the +5V Bus by adding the 2 3/4" piece of wire down the center of the Bending/Soldering jig.
- Using a pair of small needle node pliers, bend a small round hook in one end of the 2 3/4" piece of bare wire. See first photo above.
- Place the hooked end of the wire around the +5V wire near the center of the left side.
- Lay the wire into the slots of the center row of square wire guides. See second photo above.
- Trim the wire such that it is just short of touching the last square wire guide in the column.
- Make sure that all of the bare wires are seated in the slots in the square wire guides and are as flat and straight as you can get them.
If you are using a handmade jig then the 2 3/4" wire should follow the red line on the jig that runs down the center of the jig. The wire should attach to the wire that follows the red line that goes around three sides of the jig. The wire should not contact the wire following the green line.
You are now ready to add LEDs and solder the layer together. Go back to the step where you came from.
Step 12: Assembly of an Odd Numbered Layer: Add the Buses and the First Column of LEDs
I strongly advise you to add one LED at a time, bending and cutting the leads as instructed. When you have filled one column, check that the LEDs are correctly oriented. It is much easier to correct the orientation of an LED before it is soldered that after it has been soldered. The first photo above shows what you need to assemble this layer.
- Go back and do Steps 9, 10 and 11 to add the +5V and Ground buses to the 3D printed Bending/Soldering jig.
- Position the 3D printed Bending/Soldering jig in front of you such that the side with the word 'Front' is closest to you. See second photo above.
- Place an LED into the the first empty LED holder in the right most column that is closest to you.The short leads should be closest to you and all the leads should be inline with the narrow slots in the top of the LED holder. See third photo above.
- While gently pressing down on all the leads to keep the LED in the LED holder, bend the short lead that is closest to you towards you until the lead is perpendicular to the LED holder and is in the narrow slot. See fourth photo above.
- While gently pressing down on all the leads to keep the LED in the LED holder, bend the long lead that is furthest away from you, away from you until it is perpendicular to the LED holder, is in the narrow slot of this LED holder and the next one in line. See fifth photo above.
- Note that the long lead just bent reaches all the way past the center of the LED holder just above it.
- While gently pressing down on the LED to keep it in the LED holder, bend the unbent short lead to the right until it is perpendicular to the LED holder, is in the wide slot and makes contact with the +5V bus wire. See sixth photo above.
- While gently pressing down on the LED to keep it in the LED holder, bend the unbent long lead to the left until it is perpendicular to the LED holder, is in the wide slot and makes contact with the Ground bus wire. See seventh photo above.
- Note that the long lead just bent reaches all the way to the center of the LED holder on the left.
- If everything looks good, then trim the long lead that is extending into the next LED holder in the column to just about even with the far side of the square wire guides on either side. See eighth photo above.
- Repeat steps 3 through 11 to add the next two LEDs to the column. See ninth photo above.
- Place one of the previously bent LEDs that has the Dout lead bent to the right when viewed from the bottom with the long leads closest to you, into the last LED holder in the column. The short leads should be closest to you and all the leads should be inline with the narrow slots in the LED holder. See tenth photo above.
- Repeat steps 5 through 10, skipping over step 6 (the long lead was bent previously). See eleventh photo above.
- Trim the short leads that are pointing at you such that they overlap the lead that points away from you on the LED below it in the same column. Trim it to line up with the near side of the two square wire guides on either side. The two leads will be soldered together, so make sure that they overlap. Do not leave a gap.Your jig should look like the twelfth photo above.
- All of the leads that are bent to the right should be the same length.
- All of the leads bent to the left should be the same length and extend into the center of the LED holder on the left. See thirteenth photo above.
- Looking at the bottom of the LEDs, the first and last lead should be inline with each other and the two center leads should be staggered, with the second lead bent to the right and the third lead bent to the left.
- If everything looks good then carefully trim the leads on the left and right such that they do not extend past the bus wire on that side. Do not trim the long lead that is bent to the left. See fourteenth photo above.
- After trimming, solder the leads to the bus wires. It may help to use something to press down on the LED lead so that it contacts the bus wire while soldering it. Be quick, try not to melt the 3D printed jig. Do not blow on the solder joints to cool them. This makes for a bad solder joint.
- Once all of the bus joints are soldered, align each of the leads that run from one LED to the next such that they are overlapping and inline. Solder them when ready.
You have just finished the first column of an even layer. You can test this now to verify that you assembled it correctly. To test the column jump to Step 20, Testing and Troubleshooting a Layer.
Step 13: Assembly of an Odd Numbered Layer: Add the Second Column of LEDs
The second column of LEDs is done a little different from the first column of LEDs. This time you will be adding LEDs starting with the LED holder furthest away from you and the LEDs are inserted with the long leads closest to you.
- Find one of the previously bent LEDs that has the Din lead bent to the right when viewed from the bottom with the long leads closest to you, See first photo above.
- Insert it into the LED holder furthest from you in the second column from the right.The bent short lead should overlap the bent long lead from the LED in the end of the first column and all of the leads should be inline with the narrow slot in the top of the LED holder. See second photo above.
- While gently pushing down on the leads of the LED, bend the long lead that is closest to you, down towards you until it is perpendicular to the LED holder and in the narrow slot. The lead should extend into the narrow slot and past the center of the next LED holder in the column. See third picture above.
- While gently pushing down on the LED, bend the unbent long lead to the right, into the wide slot, until it is perpendicular to the LED holder. It should extend all the way to the center of the LED holder to the right. See fourth photo above.
- While gently pushing down on the LED, bend the unbent short lead to the left, into the wide slot, until it is perpendicular to the LED holder. It should extend to the LED holder to the left. See fifth photo above.
- If everything looks good, then trim the long lead that is extending into the next LED holder in the column to just about even with the near side of the square wire guides on either side. Trim the bent Din lead to just even with the square wire guide that is above and to the right of the LED holder. Do not trim the Dout lead from the LED at the end of the first column. The Dout and Din leads should overlap. See sixth photo above.
- Place an unbent LED into the next open LED holder such that the long leads are closest to you and all of the leads are inline with the narrow slots in the top of the LED holder. See seventh photo above.
- While gently pushing down on the LED leads, bend the short lead that is furthest from you down until it is perpendicular to the LED holder and is in the narrow slot. The lead should extend all the way into the LED holder above it.
- Trim the lead to the far side of the square wire guides on either side. The lead should overlap the lead from the LED above it.
- Repeat steps 4 through 9 until only the LED holder on the end is left empty.
- The jig should look like the eighth photo above.
- Find the LED with the long lead bent to the left when viewed from the bottom with the long leads closest to you. See ninth photo above.
- Place the LED into the last LED holder in the column.You may have to trim the +5V bus that runs down the center of the jig to keep it from shorting to the LED lead that is bent to the left. The +5V bus should not extend past the wide slots in the LED holders. See tenth photo above.
- While gently pushing down on the LED leads, bend the short lead that is furthest from you down until it is perpendicular to the LED holder and is in the narrow slot. Trim the lead to the far side of the square wire guides on either side. The lead should overlap the lead coming from the LED above it.
- Repeat steps 4 and 5 to bend the remaining leads of the LED. See eleventh photo above.
- Note that all of the leads bent to the right are the same length and extend into the center of the adjacent LED holder.
- Note that all of the leads bent to the left are the same length.
- If everything looks good then go ahead and trim the leads bent to the left and right such that they do not extend past the associated bus. Do not trim the long lead that is bent to the left that does not contact any buses. See twelfth photo above.
- After trimming, solder the leads to the bus wires. It may help to use something to press down on the LED lead so that it contacts the bus wire while soldering it. Be quick, try not to melt the 3D printed jig. Do not blow on the solder joints to cool them. This makes for a bad solder joint.
- Once all of the bus joints are soldered, align each of the leads that run from one LED to the next such that they are overlapping and inline. Solder them when ready.
- Solder the +5V bus wire the runs down the center of the jig to the +5V bus wire that runs around the outside of the jig.
You have just finished the second column of an odd layer. You can test this now to verify that you assembled it correctly. To test the layer jump to Step 20, Testing and Troubleshooting a Layer.
Step 14: Assembly of an Odd Numbered Layer: Add the Third Column of LEDs
The third column is similar to the first column. The only difference is that the first LED in the column is the LED that had its Din lead previously bent to the right.
- Find the LED with the Din (short) lead bent to the right when viewed from the bottom with the short leads closest to you. See first photo above.
- Place the LED in the LED holder closest to you in the third column of LED holders. See second photo above.
- Follow steps 5 through 10 of Step 12 to bend the leads of this LED.
- Follow steps 3 through 20 of Step 12 to add the remaining three LEDs to this column.
- When you are done, the jig should look like the third photo above.
You have just finished the third column of an odd layer. You can test this now to verify that you assembled it correctly. To test the layer jump to Step 20, Testing and Troubleshooting a Layer.
Step 15: Assembly of an Odd Numbered Layer: Add the Fourth Column of LEDs
The fourth column of LEDs is similar to the second column. The only difference is that the LED closest to you in the fourth column has its Dout lead pointing straight at you.
- Step 13 has the second column ending with an LED that has the Dout (long) lead bent to the left. The fourth column ends with an LED with the Dout lead pointing straight at you.
- Follow all the steps of Step 13, except use an LED with unbent leads in the last led holder, the one closest to you. The LED is positioned with the long leads closest to you. Bend the long lead closest to you straight down until it is in the narrow slot and is perpendicular to the LED holder.
- The LED closest to you in the fourth column should have its Dout (long) lead pointing straight at you. See photo above.
You have just finished an odd numbered layer. You can test this now to verify that you assembled it correctly. To test the layer jump to Step 20, Testing and Troubleshooting a Layer.
Once you have verified that the layer is working, gently remove it from the Bending/Soldering jig and set it aside.
You need two odd numbered layers. Repeat from Step 12 to make a second odd numbered layer.
Step 16: Assembly of an Even Numbered Layer: Add the Buses and the First Column of LEDs
I strongly advise you to add one LED at a time, bending and cutting the leads as instructed. When you have filled one column, check that the LEDs are correctly oriented. It is much easier to correct the orientation of an LED before it is soldered that after it has been soldered. The first photo above shows what you will need to assemble this layer.
- Go back and do Steps 9, 10 and 11 to add the +5V and Ground buses to the 3D printed Bending/Soldering jig.
- Position the 3D printed Bending/Soldering jig in front of you such that the side with the word 'Front' is closest to you. See second photo above.
- Place an LED into the the first empty LED holder in the left most column that is closest to you.
- The short leads should be closest to you and all the leads should be inline with the narrow slots in the top of the LED holder. See third photo above.
- While gently pressing down on all the leads to keep the LED in the LED holder, bend the short lead that is closest to you towards you until the lead is perpendicular to the LED holder and is in the narrow slot. See fourth photo above.
- While gently pressing down on all the leads to keep the LED in the LED holder, bend the long lead that is furthest away from you, away from you until it is perpendicular to the LED holder, is in the narrow slot of this LED holder and the next one in line. See fifth photo above.
- Note that the long lead just bent reaches all the way past the center of the LED holder just above it.
- While gently pressing down on the LED to keep it in the LED holder, bend the unbent short lead to the left until it is perpendicular to the LED holder, is in the wide slot and makes contact with the +5V bus wire. See sixth photo above.
- While gently pressing down on the LED to keep it in the LED holder, bend the unbent long lead to the right until it is perpendicular to the LED holder, is in the wide slot and makes contact with the Ground bus wire. See seventh photo above.
- Note that the long lead just bent reachs all the way to the center of the LED holder on the right.
- If everything looks good, then trim the long lead that is extending into the next LED holder in the column to just about even with the far side of the square wire guides on either side. See eighth photo above.
- Repeat steps 3 through 11 to add the next two LEDs to the column. See ninth photo above.
- Place one of the previously bent LEDs that has the Dout lead bent to the right when viewed from the bottom with the short leads closest to you, into the last LED holder in the column. The short leads should be closest to you and all the leads should be inline with the narrow slots in the LED holder. See tenth photo above.
- Repeat steps 5 through 11, skipping over steps 6 an 7 (the long lead was bent previously). See eleventh photo above.
- Trim the short leads that are pointing at you such that they overlap the lead that points away from you on the LED below it in the same column. Trim it to line up with the near side of the two square wire guides on either side. The two leads will be soldered together, so make sure that they overlap. Do not leave a gap.
- Your jig should look like the twelfth photo above.
- All of the leads that are bent to the left should be the same length.
- All of the leads bent to the right should be the same length and extend into the center of the LED holder on the right. See thirteenth photo above.
- Looking at the bottom of the LEDs, the first and last lead should be inline with each other and the two center leads should be staggered, with the second lead bent to the left and the third lead bent to the right.
- If everything looks good then carefully trim the leads on the left and right such that they do not extend past the bus wire on that side. Do not trim the long lead that is bent to the right. See fourteenth photo above.
- After trimming, solder the leads to the bus wires. It may help to use something to press down on the LED lead so that it contacts the bus wire while soldering it. Be quick, try not to melt the 3D printed jig. Do not blow on the solder joints to cool them. This makes for a bad solder joint.
- Once all of the bus joints are soldered, align each of the leads that run from one LED to the next such that they are overlapping and inline. Solder them when ready.
You have just finished the first column of an even layer. You can test this now to verify that you assembled it correctly. To test the column jump to Step 20, Testing and Troubleshooting a Layer.
Step 17: Assembly of an Even Numbered Layer: Add the Second Column of LEDs
The second column of LEDs is done a little different from the first column of LEDs. This time you will be adding LEDs starting with the LED holder furthest away from you and the LEDs are inserted with the long leads closest to you.
- Find one of the two LEDs with the short lead bent to the right when viewed from the bottom with the short leads closest to you. See first photo above.
- Insert it into the LED holder furthest from you in the second column.
- The bent short lead should overlap the bent long lead from the LED in the end of the first column and all of the leads should be inline with the narrow slot in the top of the LED holder. See second picture above.
- While gently pushing down on the leads of the LED, bend the long lead that is closest to you, down towards you until it is perpendicular to the LED holder and in the narrow slot. The lead should extend into the narrow slot and past the center of the next LED holder in the column. See third picture above.
- While gently pushing down on the LED, bend the unbent long lead to the left, into the wide slot, until it is perpendicular to the LED holder. It should extend all the way to the center of the LED holder to the left. See fourth photo above.
- While gently pushing down on the LED, bend the unbent short lead to the right, into the wide slot, until it is perpendicular to the LED holder. It should extend to the LED holder to the right. See fifth photo above.
- If everything looks good, then trim the long lead that is extending into the next LED holder in the column to just about even with the near side of the square wire guides on either side. See sixth photo above.
- Place an unbent LED into the next open LED holder such that the long leads are closest to you and all of the leads are inline with the narrow slots in the top of the LED holder. See seventh photo above.
- While gently pushing down on the LED leads, bend the short lead that is furthest from you down until it is perpendicular to the LED holder and is in the narrow slot. The lead should extend all the way into the LED holder above it.
- Repeat steps 4 through 9 until only the LED holder on the end is left empty.
- The jig should look like the ninth photo above.
- Find the LED with the long lead bent to the right when viewed from the bottom. See tenth photo above.
- Place the LED into the last LED holder in the column.
- You may have to trim the +5V bus that runs down the center of the jig to keep it from shorting to the LED lead that is bent to the right. The +5V bus should not extend past the wide slots in the LED holders. See eleventh photo above.
- While gently pushing down on the LED leads, bend the short lead that is furthest from you down until it is perpendicular to the LED holder and is in the narrow slot.
- Repeat steps 5 and 6 to bend the remaining leads of the LED. See twelfth photo above.
- Note that all of the leads bent to the left are the same length and extend into the center of the adjacent LED holder.
- Note that all of the leads bent to the right are the same length.
- If everything looks good then go ahead and trim the short leads that are bent away from you. Trim them to be even with the far side of the square wire guides on either side. After trimming, they should overlap the lead coming from the LED above it in the column.
- Trim the leads bent to the left and right such that they do not extend past the associated bus. Do not trim the long lead that is bent to the right that does not contact any buses. See thirteenth photo above.
- After trimming, solder the leads to the bus wires. It may help to use something to press down on the LED lead so that it contacts the bus wire while soldering it. Be quick, try not to melt the 3D printed jig. Do not blow on the solder joints to cool them. This makes for a bad solder joint.
- Once all of the bus joints are soldered, align each of the leads that run from one LED to the next such that they are overlapping and inline. Solder them when ready.
- Align the lead of the last LED in the first column that is bent to the right with the lead of the last LED in the second column such that they overlap. Trim the short lead from the LED in the second column just to the nearby wire guide. Solder these leads when you are ready.
- Solder the +5V bus wire the runs down the center of the jig to the +5V bus wire that runs around the outside of the jig.
You have just finished the second column of an even layer. You can test this now to verify that you assembled it correctly. To test the column jump to Step 20, Testing and Troubleshooting a Layer.
Step 18: Assembly of an Even Numbered Layer: Add the Third Column of LEDs
The third column is similar to the first column. The only difference is that the first LED in the column is the LED that had its Din (short) lead previously bent to the left.
- Find the LED with the Din (short) lead bent to the left when viewed from the bottom with the short leads closest to you. See first photo above.
- Place the LED in the LED holder closest to you in the third column of LED holders. See second photo above.
- Follow steps 6 through 11 of Step 16 to bend the leads of this LED.
- When you are done, the jig should look like the third photo above.
- Follow steps 12 through 22 of Step 16 to add the remaining three LEDs to this column.
- Trim the lead that is bent to the left of the first LED in the third column so that it is even with the square wire guide near it. The lead should overlap the lead coming from the LED in the second column after it is trimmed.
- Align and solder the lead that is bent to the right from the first LED in the second column to the lead that is bent to the left of the first LED in the third column.
- When you are done, the jig should look like the fourth photo above.
You have just finished the third column of an even layer. You can test this now to verify that you assembled it correctly. To test the column jump to Step 20, Testing and Troubleshooting a Layer.
Step 19: Assembly of an Even Numbered Layer: Add the Fourth Column of LEDs
The fourth column of LEDs is similar to the second column. The only difference is that the LED closest to you in the fourth column has its Dout lead pointing straight at you.
- Step 17 has the second column ending with an LED that has the Dout (long) lead bent to the left. The fourth column ends with an LED with the Dout lead pointing straight at you.
- Follow all the steps of Step 17, except use an LED with unbent leads in the last led holder, the one closest to you. The LED is positioned with the long leads closest to you. Bend the long lead closest to you straight down until it is in the narrow slot and is perpendicular to the LED holder.
- The LED closest to you in the fourth column should have its Dout (long) lead pointing straight at you. See photo above.
- You have just finished an even numbered layer. You can test this now to verify that you assembled it correctly. To test the layer jump to Step 20, Testing and Troubleshooting a Layer.
Once you have verified that the layer is working, gently remove it from the Bending/Soldering jig and set it aside. You need two even numbered layers. Repeat from Step 16 to make a second odd numbered layer.
Step 20: Testing and Troubleshooting a Layer.
To test a layer you will need a 5V Arduino board and three jumper wires.
Visually inspect your work.
- Make sure that the +5V bus and Ground bus on the Bending/Soldering jig are not shorted together.
- Are all of the LED leads to the buses soldered ?
- Is each LED soldered to the next one in the column ?
- Are the end of column LEDs soldered together ?
Connect to an Arduino. Make the connections while the Arduino board is powered off.
- Connect a jumper from a +5V pin on your Arduino board to the +5V bus on the jig.
- Connect a jumper from a Gnd pin on your Arduino board to the Ground bus on the jig.
- Connect a jumper from Digital pin 6 on your Arduino board to the short lead (Din) of the first LED in the layer.
- Open the Arduino IDE.
- Open LEDTest.ino.
- Compile and upload it to your Arduino board.
- When power is first applied to the Arduino board, all of the LEDs in the layer may light up blue.
- You should see all of the LEDs go;
- red
- then green
- then blue
- then go off
- then light up red, in sequence, from the first LED to the last LED in the layer.
- After a short delay the pattern will repeat.
If your layer did as described then you correctly assembled the column.
If not then;
- check for shorts in the buses.
- Did you reverse the +5V and Ground bus connections ?
- Are you connected to Digital Pin 6 ?
- Are you connected to Din of the layer (short lead) ?
- Even numbered layers have Din on the left.
- Odd numbered layers have Din on the right.
- The first LED in the sequence to not light up is the one with a problem.
- Are all the leads of the LED soldered ?
- Are the middle two leads of the LED oriented the same as a working LED ?
- See photo above.
- Remove the LED and replace it with another.
- Follow the instructions for adding LEDs to the layer and try again.
- When the layer passes the test then it is time to assemble the next column of LEDs.
Step 21: Building the LED Cube: Stacking the Layers.
In this step you will stack the layers on top of each other, using the spacers to keep the distance between layers equal. The layers are stacked from bottom to top as odd, even, odd and even. The odd numbered layers have the short Din lead on the left and the even numbered layers have the short Din lead on the right. All the layers should face the same way. The LEDs are upright.
For this step you will need;
- Two odd numbered layers.
- Two even numbered layers.
- The six spacers.
- A rubber band that is a little smaller than the perimeter of one layer.
- Masking tape also works.
- Place two of the spacers on your work surface with the slots facing up.
- Align the slots.
- Place an odd numbered layer into the slots at the bottom of the spacers. Use the side of the layer that has the +5V bus and is not a side that has the center +5V wire.
- The slot in the spacer should fit on to a section of the +5V bus wire that is between two LEDs, not on a solder joint.
- There are three sections of the +5V wire. You should have the spacers on the two outer most sections.
- The Din and Dout leads of the layer should be pointing at you.
- While holding the odd numbered layer in place, add an even numbered layer into the next set of slots above the odd numbered layer. Again, the +5V bus wire should be in the next set of slots.
- Add two spacers on the opposite side, gravity will help to hold them in place.
- The +5V bus wires of the two layers should be in the slots of the spacers.
- Wrap the rubber band around the bottom of the four spaces to apply pressure to hold the spacers in place. Do not let go of the layers. Slide the rubber band up until it is halfway between the layers.
- On the side that has the +5V bus center wire soldered to it, add a spacer by slipping it under the rubber band and sliding it in until the +5V bus of both of the layers are captured by the two bottom slots of the spacer.
- Repeat step 11 only this time adding the space to the side of the cube that has the Din and Dout leads . The slots of the spacer should be capturing the bottom of the 'U' of the ground bus.
- This should give you a fairly stable, two layer cube.
- Verify that you have an odd numbered layer on the bottom and an even numbered layer on top of it.
- If all looks good then work the second odd numbered layer into place above the even numbered layer. You will need to roll the rubber band up to the even numbered layer to keep everything from popping out.
- The slots of the spacers should be on the bus wires in the areas where there are no solder joints.
- Add the last even numbered layer to the top slot of the spacers using the same technique you used to add the third layer. Roll the rubber band up to about the center of the stack.
- Your cube should look like the photos above.
- Check that you have the layers in the correct order and that all of the layers have the Din and Dout leads on the same side.
Step 22: Building the LED Cube: Wire the Layers Together.
In this step you are going to finish the assembly of the LED cube by soldering 22 awg bare wires on the sides of the cube to connect the +5V and Ground buses of each layer together. Your layers of your cube should be held in place by the spacers. The cube will be prone to racking.
- Eight pieces of straight, 22 awg, bare wires 3" long.
- Small needle nose pliers.
- Soldering Iron and solder.
- Take one of the 3" pieces of bare. 22 awg wire and using the needle nose pliers, bend one end into a hook.
- Position the cube upright in front of you with one of the bends of the outer +5V bus wires facing you.
- Place the hook in the 3" piece of wire over the +5V bus wire, as close as possible to the center of the arc of the bend.
- The wire should fall inside the cube and contact the +5V bus of each of the layers below in about the same place as the top layer. Solder the wire to the top layer.
- Position the cube to make it easier for you to solder the wire hanging down from the top layer to each layer below. Try to keep the wire as straight as possible and as vertical as possible.
- Straighten the cube, aligning the LEDs on the layers and removing any racking that has occurred. When your cube is ready, solder the wire to the remaining three layers.
- Your cube should look like the first two photos above. The rubber band will be sacrificed after the Ground buses are connected.
- Repeat steps 1 through 6 to add a second wire connecting the other bends in the +5V bus together.
- Your cube should look like the third photo above.
You now have two of the four wires that are used to interconnect the +5V buses of the layers together. Your cube should be a little more stable now. Next you are going to solder the Ground buses together.
- Take one of the 3" pieces of bare, 22 awg wire and using the needle nose pliers, bend one end into a hook.
- Position the cube upright in front of you with the bottom of the 'U' of the Ground buses facing you.
- Place the hook in the 3" piece of wire over the Ground bus wire, as close as possible to the center of the arc of the bend.
- The wire should fall inside the cube and contact the Ground bus of each of the layers below in about the same place as the top layer. Solder the wire to the top layer.
- Position the cube to make it easier for you to solder the wire hanging down from the top layer to each layer below. Try to keep the wire as straight as possible and as vertical as possible.
- Straighten the cube, aligning the LEDs on the layers and removing any racking that has occurred. When your cube is ready, solder the wire to the remaining three layers.
- Repeat steps 1 through 6 to add a second vertical Ground wire to the other bend in the Ground buses. Solder the wire to the Ground bus of each layer.
- In the fourth photo above, notice how the rubber band has been positioned behind some of the LEDs to hold it out of the way.
- You can cut the rubber band and remove the spacers. Your cube should be very stable.
In this next series of steps you are going to add the remaining four 3" wires to the cube. The wires serve two purposes. The first is to provide multiple +5V and Ground paths to each layer. This is done to reduce the voltage drop when all of the LEDs are turned on. The second is to make the cube more stable.
- Take one of the 3" pieces of bare, 22 awg wire and using the needle nose pliers, bend one end into a hook.
- Position the cube upright in front of you with the bottom of the 'U' of the Ground buses facing you.
- Place the hook in the 3" piece of wire over the +5V bus wire end that extends out from the top layer. Position the wire to be next to the +5V lead from the LED.
- Solder the wire to the +5V bus wire. Make sure that the LED lead stays soldered to the +5V bus wire.
- Position the cube to make it easier to solder the vertical wire to each +5V bus wire in the layers below.
- Solder them when ready. Try to keep the wire perpendicular to the layers and in a straight line.
- Repeat steps 1 through 6 to connect the +5V bus ends on the other side of the cube together.
This next part is a little tricky. You are going to add wires to connect the Ground bus wires of the layers together. This time the wires are added to the interior of the cube.
- Take one of the 3" pieces of bare, 22 awg wire and using the needle nose pliers, bend one end into a hook.
- Position the cube upright in front of you with the bottom of the 'U' of the Ground buses facing away from you.
- Place the hook in the 3" piece of wire over the Ground bus wire of the top layer, halfway between the the LEDs.
- Solder the wire to the Ground bus wire of the top layer.
- Position the cube to make it easier to solder the vertical wire to each Ground bus wire in the layers below.
- Solder the wire to the Ground bus of the other three layers, halfway between the LEDs of the layer. Try to keep the wire perpendicular to the layers and in a straight line.
- Repeat steps 1 through 6 to connect the Ground bus on the other side of the cube together.
The rest of the photos above show various sides of the cube with all of the wires that connect +5V and Ground buses of the layers together.
Step 23: Building the LED Cube: Finishing the Cube.
In this step you are going to connect the Dout signal from each layer to the Din signal of the layer above it.
- Using the small needle nose pliers, grab the Dout lead from the bottom layer about 1/8" from the LED. The pliers should be between the LED and where the bend will be.
- While holding the lead with the pliers, use your fingers to bend the led straight up until it is perpendicular to the layer.
- Following the same procedure, bend the Din lead of the LED in the layer above down until it contacts the Dout lead that was bent up.
- The leads should overlap.
- Solder them together.
- Repeat steps 1 through 5 for the Dout lead of second layer and the Din lead of the third layer.
- Repeat steps 1 through 5 for the Dout lead of third layer and the Din lead of the fourth layer.
- See the photo above.
- Clip off the excess wire from all of the +5V buses. The wires should be cutoff where the wire that connects the +5V buses to the other layers is soldered.
- Your cube should look like the photo above.
Congratulations, you have completed the assembly of the LED cube. Follow the steps below to test your completed cube. Keep in mind that the USB connection that is powering your Arduino board cannot supply enough power to light up every LED in the cube.
- Connect a jumper from a +5V pin on your Arduino board to the +5V bus on the cube.
- Connect a jumper from a Gnd pin on your Arduino board to the Ground bus on the cube.
- Connect a jumper from Digital pin 6 on your Arduino board to the Din (short lead) of the bottom layer of the cube.
- Open the Arduino IDE.
- Open LEDTest.ino.
- Connect your Arduino board to your computer.
- Change the 16 in line 26 to 64
- From; #define NUMLEDS 16
- To; #define NUMLEDS 64
- Compile and upload it to your Arduino board.
- When power is first applied to the Arduino board, all of the LEDs in the cube may light up blue.
- You should see all of the LEDs go;
- red
- then green
- then blue
- then go off
- then light up red, in sequence, from the first LED to the last LED in the cube.
- The sequence is serpentine through each layer, jumping to the next layer when the end of the layer is reached.
- After a short delay the pattern will repeat.
- If your cube did as described then you correctly assembled the cube.
- If your cube does not work as described.
- Did you attach the +5V and Ground wires to the correct buses on the cube ?
- Did you attach the wire from Digital pin 6 to the Din (short lead) of the bottom layer ?
- Are all of the solder joints that connect the layers together making contact with the bus wires of the layers ?
- Are the Dout leads of each layer soldered to the Din lead of the layer above it ?
- Make sure that the +5V and Ground buses are not shorted together.
When your cube has been tested and is in good working order then proceed to the next step.
Step 24: 3D Print the LED Cube Base, Top and Knob
Included in the download from GitHub is a folder named 3D Parts. This folder containsthe OpenSCAD source file for the 3D printed base, top and knob. One file makes all of the parts or can print each part individually. The base that is printed from these files is for an Arduino ProMini. If you wish to use a different Arduino then you will need to edit the .scad file and select the type of Arduino you are using. The files are;
- LedCube.scad - this file is the source for all of the 3D printed parts.
- All.stl - this file is for printing all of the parts at the same time.
- Base.stl - this file is for printing just the base.
- Top.stl - this file is for printing the top and the four LED cube mounts.
- Knob.stl - this file is for printing just the knob.
The base holds the Arduino, the power jack and the rotary encoder. The top holds the power switch, Mode LED, the mounts for the LED cube, holes for the wires for the LED cube and for the rotary encoder shaft. The knob is for the rotary encoder. The top includes four LED cube mounts that have to be pressed into place after being 3D printed.
The file LedCube.scad is the source file for all of the parts. I have given you the source file so that you can modify it to suit your needs. For example, if you do not have a rectangular rocker switch then you can modify the source to use a round switch or no switch. The source file is organized as a collection of building blocks that make up the features added to the top and the base. The file follows the Decorator software pattern. At the top level there is the DecoratedTop, DecoratedBase and Knob. The DecoratedTop uses the Top as its starting point and then adds 'decorations' in the form of cutouts and added mounts for each component that is mounted to the top. Same for the DecoratedBase.
Arduinos come in many shapes and sizes these days. The source file has a series of values near the beginning of the file that are used to select the Arduino you wish to use. You can select an Arduino R3 compatible form or an Arduino Pro Mini or an Arduino Nano. The Arduino R3 covers the Arduino Uno and Leonardo. Along with the Arduino R3 you can select a USB type B connector or a Micro USB connector. Only one type of Arduino should be selected.
// select your Arduino by setting it to true // only one selection can be true. ArduinoR3 = false; // Uno or Leonardo ProMini = true; Nano = false; // make this true if your Arduino board uses a micro USB connector MicroUSB = true;
From the settings above, an Arduino Pro Mini is selected. The MicroUSB selection only pertains to the ArduinoR3 selection. If it is set to false then the ArduinoR3 selection will use a cutout for a USB type B connector.
Arduino boards that include a USB connector are capable of powering the Arduino board from the USB. USB 2.0 limits the available current to 500 mA. USB 3.0 limits the available current to 1 Amp. Neither of these is sufficient to fully power the LED cube. USB 2.0 can power about 20 LEDs (20 single colors). USB 3.0 can double that number. The power jack on the Arduino R3 and Pro Mini boards have a diode in series with the positive voltage input pin. The purpose of the diode is to protect the board from reversing the power connections. The Arduino R3 and Pro Mini boards also have an AS1117 5V regulator. The AS1117 regulator can handle about 1 Amp before it goes up in flames. The bottom line here is that you cannot power the LED cube from the power circuits on the Arduino R3 and Pro Mini boards. If you want to use the power jack on the Arduino R3 board then you must solder wires to the underside of the board, directly to the power jack pins. Use these wires to power the LED cube. If you do this then you do not need a power switch. You can eliminate the power switch by setting PowerSwitch to false.
// Make this false to remove the power switch. PowerSwitch = true;
If you decide to use an Arduino R3 board and do not want to use the on board power jack then you can un-comment a line of code that will move the Arduino R3 mount points back and close the opening for the on board power jack and open a hole for an stand alone power jack. To un-comment the line just delete the two slashes ('//') at the start of the line.
// uncomment this offset to hide the Arduino's power jack //ArduinoXOffset = 19 + Wall;
There are a set of values that determines which parts are printed. Setting any value to false will eliminate it from the print. You can print all three or select only what you want. You must select at least one or you will get an error message.
// Select what parts to 3D print. Set to true to print a part. // You can print all three at once or each one at a time. PrintTop = true; PrintBase = true; PrintKnob = true;
The Arduino R3 board and the rotary encoder are held in place by #2 x 1/4", pan head, wood screws. You should be able to find them at your local Loew's or Home Depot store. The Arduino Pro Mini and Nano are held in place with 3M Scotch, 1/8" thick, foam, double sticky tape.
I recently found some boards on AliExpress that are basically an Arduino Uno (AtMega328p) and an ESP8266 on the same R3 form factor board. The boards have the WeMos logo on them. If you want to make your LED cube have a wireless connection then one of these might be worth looking into. The current software does not support wireless and is beyond the scope of this project to add it.
Step 25: Assembly of the LED Cube Base.
In this step you will mount all of the parts that attach to the base and wire them together. I am using an Arduino Pro Mini so the photos will show a base printed for an Arduino Pro Mini and the wiring will be for the Pro Mini. The three PDF wiring files are also included in the GitHub download. Follow the wiring diagram for the type of Arduino that you are using.
- Wiring.pdf is for an Arduino Pro Mini or R3 where the onboard power jack is not used.
- Nano Wiring.pdf is for an Arduino Nano.
- R3 Wiring.pdf is for an Arduino R3 where the onboard power jack is used.
Click on one of the pdf files to download it to your computer. You can then print or view it. The files are in color, so use a color printer if you have one.
You will need;
- The 3D printed LED Cube base
- An Arduino Pro Mini board.
- An Arduino Rotary Encoder.
- A power jack that mates with your 5V, 4Amp power supply.
- Red and Black 18 awg, stranded wire.
- Red Black and other colors of 22-28 awg stranded hookup wire.
- 1/8" diameter heat shrink sleeving.
- Two #2 by 1/4", pan head, wood screws.
- Four peal and stick, 10 mm rubber bumpers.
- 1 1/2" of 1/8" 3M Scotch foam double sticky tape
- Optional
- DuPont 5 pin shell
- DuPont 8 pin shell
- DuPont 4 pin shell
- DuPont female crimps
The Arduino Rotary Encoders that I bought have a place for three surface mount pullup resistors on the bottom of the board. Only two were populated. I added a 10K, 1206 smt resistor to the board, fifth photo above. The two original resistors are for the DT and CLK signals (Quadrature A & B). The missing resistor is for the push button switch (press down on the shaft of the encoder to activate it). The code has the internal weak pullup of the Arduino enabled so this is not required, but is a good thing nonetheless. With the rotary encoder wired as shown in the wiring diagrams, clockwise rotation will increase brightness and speed. Counter-clockwise rotation will decrease brightness and speed. If you reverse the DT and CLK signals then the rotations will be reversed.
The Arduino Pro Mini comes packaged with two fifteen pin straight male headers and one two-by-three pin header for the SPI port. I soldered two fifteen pin right-angle headers to the board instead of the straight headers, see third photo above. This reduces the height requirement so that my base height can be lower. I use headers because I have crimpers, crimps and shells to make the wiring harnesses that can mate with the headers. If you do not have the crimping tool, female crimps and shells then you can solder wires directly to the holes in the Pro Mini. If you are using an Arduino R3 form factor, the height of the base has been increased to accommodate the extra space needed to insert male pins into the socket strips on the Arduino R3 board. One alternative is to insert the long pins of a right-angle male header into the socket strips and solder wires to the shot pins. Another is to strip and tin the wires and then insert them into the socket strips. Your choice. The base is not tall enough to add a shield on top of the Arduino R3 board. If using an Arduino R3 board then you will need four #2 by 1/4" pan head, wood screws to hold it in place.
The rotary encoders came with a five pin, right-angle male header soldered to the board. If you have the parts you can make a wiring harness to plug onto the header or remove the header and solder wires directly to the board. I suggest that if you are soldering wires directly to the boards then you do so before mounting them to the base.
I am using a power jack that has the retaining nut on the outside. I soldered wires to the power jack before inserting it in the hole in the base and adding the nut and lock-washer. The sixth photo above shows the power jack before soldering wires to it. The seventh photo shows the power jack after the wires have been soldered and the jack mounted in the base. Notice that there a two black wires soldered to the power jack, a thick one and a thinner one. The thick wire is the ground wire for the LED cube. The thinner wire is the ground wire for the Arduino board. The red wire is the Vin wire and goes to the power switch. The solder joints are covered with shrink sleeving.
Power to the LED cube should use 18 awg wires. If you do not have 18 awg wires then run two 22 awg Vin wires and two awg ground wires to the LED cube. Doubling the 22 awg wires will reduce the voltage drop in the wires when all of the LEDs are lit up. Power to the Arduino board should use 22 awg wires.
If you are using an Arduino R3 board and you want to use the onboard power jack then you must solder wires to the bottom of the power jack to power the LED cube. See ninth photo above. The circuitry on the Arduino R3 board cannot handle the load of an LED cube with all of the LEDs lit up. This configuration does not use a power switch.
Select the wiring PDF that matches the Arduino board and wiring that you want to use.
The eighth photo above shows the base with all of the parts mounted. The power jack has wires soldered to, but the other ends are not connected yet.
- Wire the CLK pin of the rotary encode to Digital pin 4 of the your Arduino.
- Wire the DT pin of the rotary encoder to Digital pin 5 of your Arduino.
- Wire the SW pin of the rotary encoder to Digital pin 3 of your Arduino.
- Wire the plus (+) pin of the rotary encoder to the +5V pin of your Arduino.
That is it. The Base sub-assembly is complete. On to preparing the Top sub-assembly.
Step 26: Assemble the LED Cube Top.
- The 3D printed LED Cube Top.
- The four 3D printed LED cube mounts.
- An APA106-F5 LED.
- A power switch.
- I used a small, rectangular rocker switch.
- My switch is 13x8 mm and matches the cutout in the Top.
- Red and Black 18 awg, stranded wire.
- Red Black and a third color of 22-28 awg stranded hookup wire.
- Optional
- 1/4" diameter heat shrink sleeving.
- 1/8" diameter heat shrink sleeving.
- DuPont shells.
- DuPont female crimps.
- Insert the four 3D printed LED cube mounts into the Top.
- The mounts are inserted from the bottom and should fit snugly into the rectangular depressions. See second photo above.
- You may have to lightly sand or file the rectangular base of the mounts to get them to fit into the rectangular openings in the Top.
- When fully seated, the mounts will extend above the surface of the top by about 5/32". See fourth photo above.
- Most 3D printers print the first layer slightly larger than called for. The end effect is that openings need to be cleaned out to get them to be the correct size. The four holes where the LED cube mounts are inserted may have this issue. If you are having difficulty getting the mounts into the holes then run a hobby knife around the hole to open it up. The mounts are the same size as the hole, so they will be a tight fit. When you insert them, make sure that the rectangular part is all the way into the matching depression. See second photo above.
- After insertion, the bottom of the mounts should be about 1/32" (1 mm) above the the surface. See third photo above.
- If the mounts are loose after insertion then use a drop or two of Super Glue to hold them in place.
- The mounts are inserted from the bottom and should fit snugly into the rectangular depressions. See second photo above.
- Solder an 18 awg red wire and two 22 awg red wires to one of the poles of the power switch.
- The 18 awg red wire should be long enough to go through the hole in the Top that is below the +5V bus of the LED cube.
- One of the 22 awg red wires needs to be long enough to reach to the +5V pin of the Mode LED.
- The other 22 awg red wire needs to be long enough to reach the +5V pin of your Arduino.
- Leave some slack in these wires. Do not draw them tight.
- After soldering, cover the joint with a piece of 1/4" shrink sleeve if you have some.
- See fifth photo above.
- The other pole of the power switch gets the red wire from the power jack soldered to it.
- Run the red wire from the power jack through the power switch opening.
- The power switch is pressed into the opening from the outside, so the red wire from the power jack should feed through from the bottom and come out the top.
- Slip a piece of 1/4" shrink sleeving onto the wire before soldering.
- After soldering, slide the shrink sleeve into position against the switch body and shrink it.
- Press the power switch into the opening.
- The power switch should be flush with the surface of the Top.
- Bend the tabs of the power switch out so that the wires are about 1/2" from the bottom of the top.
- See photo above.
- Solder wires to three of the leads of the Mode LED (APA106-F5).
- You can bend the leads of the LED to separate them.
- The leads are too long and will need to be cutoff.
- Cutoff a lead just prior to soldering a wire to it.
- If you cut them all at once you will not be able to identify the long and short leads.
- Solder a wire to the short lead closest to the edge of the LED (Din).
- Solder one of the 22 awg red wires coming from the power switch to the next short lead (+5V).
- Slip a piece of 1/8" shrink sleeving onto the red wire prior to soldering it.
- Solder two pieces of black 22 awg wire to the next long lead (gnd).
- One of the black, 22 awg wires needs to be long enough to reach a Gnd pin on the Arduino.
- The other black, 22 awg wire should be long enough to reach the rotary encoder GND pin.
- The last long lead, nearest to the edge of the case is not used. You can cut it off.
- Slip a piece of 1/8" shrink sleeving over the each of the other two wires. Push the shrink sleeving up against the body of the LED and shrink all three pieces.
- If you do not have shrink sleeving then use some other means to keep the wires from touching. Hot glue from a hot glue gun works, as does a small amount of silicone caulking.
- When the LED is ready, push it into the hole in the Top that is between the rotary encoder and the power switch.
- The LED is pressed in from the underside of the Top and will protrude above the surface of the outside of the Top.
- The LED is a tight fit. Press on the body of the LED with a small screwdriver or other tool. Do not press on the leads.
- If the LED is loose or will not stay put then a few drops of Super Glue will hold it in place.
- The LED should be pressed in until the small lip on the base of the LED is in contact with the Top.
- Position the red 22 awg wire to face the power switch.
You have finished the Top sub-assembly.
Note: I found out later that I wired the Mode LED wrong, I wired to the Dout pin instead of the Din pin. As a result, all of the photos that show the Mode LED have the Din wire on the wrong pin !! Try to do better than me and wire yours correctly. I corrected the wiring when I realized that the Mode LED was not working. Too late to shoot new pictures.
Step 27: Final Assembly.
You are nearly done. In this step you will finish the assembly and wiring of your LED Cube.
You will need;
- The Top sub-assembly.
- The Base sub-assembly.
- The LED cube sub-assembly.
- Cut off all of the vertical bus wires even with the bottom layer of the LED cube.
- Position the LED cube sub-assembly on to the mounts on the Top.
- Position the LED cube such that the short lead (Din) that is sticking out from the lowest layer is facing the front of the Top (towards the power switch).
- The +5V bus of the lowest layer, on the left and right sides of the LED cube should go into the LED cube mounts sticking up from the surface of the Top.
- Try to center the LED cube by sliding it forward or backward. Use the cutouts for the BaseBall display case top as a reference. See first photo above.
- The LED cube mounts should end up near the center points between the LEDs of the lowest layer.
- When you have it about were it should go, bend the Din lead down, it should go into the hole in the Top near the lead.
- Solder a piece of 22 awg wire to the Din lead so that it is inline with the lead and is not visible from the top.
- The 18 awg red wire from the power switch gets fed up through the hole in the top that is nearest the edge of the Top and in the area where the LED cube mounts.
- Strip the end of the wire to about 3/16".
- Twist the strands together and tin the wire.
- You should have enough slack in the wire to work comfortably.
- Bend a small hook in the tinned section of the wire.
- Place the hook in the wire over the +5V bus wire.
- Squeeze the hook with a pair of needle nose pliers to secure it.
- Pull the wire down from below, you want as little of the insulation showing as possible.
- Carefully solder the red wire to the +5V bus of the LED cube.
- The 18 awg black wire from the power jack is fed up through the inner hole in the Top that is next to the hole with the red wire.
- Strip the end of the wire to about 3/16".
- Twist the strands together and tin the wire.
- You should have enough slack in the wire to work comfortably.
- Bend a small hook in the tinned section of the wire.
- Place the hook in the wire over the bus wire.
- Squeeze the hook with a pair of needle nose pliers to secure it.
- Pull the wire down from below, you want as little of the insulation showing as possible.
- Carefully solder the black wire to the Ground bus of the LED cube.
- The second photo above shows the red, black and Din wires from the underside of the Top.
- The third photo above shows the red and black wires soldered to their respective buses.
- If you have not done so yet, clip off the unconnected long lead of the top layer's last LED.
- The lead should be pointing straight out at the top of the LED cube sub-assembly.
- This lead is not used.
- re-center the LED cube.
- If the LED cube is not staying in place then put a drop or two of Super Glue on each of the mounts where the +5V bus wire passes through them.
- Wait for the glue to dry before proceeding.
- Connect the Din wire from the LED cube to Digital pin 6 of the Arduino.
- Connect the Din wire of the Mode LED to Digital pin 7 of the Arduino.
- Connect the one of the 22 awg black wires soldered to the Mode LED to the GND pin of the Rotary Encoder
- Connect the other 22 awg black wire soldered to the Mode LED to a GND pin on the Arduino.
- Connect the 22 awg black wire from the power jack to another GND pin on the Arduino.
- Connect the + pin of the Rotary Encoder to the 5V pin of the Arduino.
- Connect the SW pin of the Rotary Encoder to Digital pin 3 of the Arduino.
- Connect the CLK pin of the Rotary Encoder to Digital pin 4 of the Arduino.
- Connect the DT pin of the Rotary Encoder to Digital pin 5 of the Arduino.
- Connect the 22 awg red wire coming from the Power Switch to the Vin pin of the Arduino.
- The Arduino R3 and the ProMini have the Vin, GND and 5V pins on the same side. All of the Digital pins used are on the other side.
- The Top is a press fit into the Base. You can press them together now or wait until you load the software and try running your LED Cube.
- The clear, BaseBall Display case cover is also a press fit.
- Insert the tabs on the two sides of the clear cover into the two slots in the Top.
- After pressing the Top into the Base, you can press the Knob into the shaft of the rotary encoder.
- The hole in the Knob matches the 'D' shape of the rotary encoder shaft. The first time it will be tight.
- Press it all the way down to where the 'D' shape ends.
Congratulations, you are finished with the assembly of your LED Cube. All that is left is to upload the software into the Arduino.
Step 28: Program the Arduino in Your LED Cube.
This is the last step. Time to upload the software into your Arduino. If you chose to use an Arduino Nano then you will need a USB-to-Serial bridge in order to program your Nano. A USB-to-Serial bridge is a board with an FTDI or CH340 or some other USB-to-Serial chip on it. The board is wired with jumpers to the Tx, Rx, RST, Vcc and GND pins of your Nano. If you are using an Arduino R3 or Pro Mini then connect a USB cable to your Arduino.
The software for the LED Cube was developed using the Arduino IDE, Version 1.8.5. You can download the Arduino IDE here. There are plenty of places on the web that can teach you how to use the Arduino IDE to develop programs to run on Arduinos and other micro-controllers. Teaching you how to program and use the Arduino IDE is beyond the scope of this Instructable. For this project you will need a copy of the Adafruit NeoPixel driver library and the Encoder library. Both of these libraries are available in the Arduino IDE's Library Manager and can be installed from there.
To install the libraries;
- Open the Arduino IDE.
- On the top menu bar, click Sketch.
- Slide down the pop-up menu to Include Library.
- Slide over and click on Manage Libraries.
- In the Library Manager dialog box, click on Filter your search.
- Enter; Adafruit NeoPixel.
- Go down to Adafruit NeoPixel and click on Install.
- if Install is not visible then click on More Info.
- Repeat step 5, except search for; Encoder.
- All of the required libraries should now be installed.
You can download the code for the LED Cube from GitHub here. After unzipping the downloaded archive, the folder CubeObject has all of the source files. Open CubeObject.ino with the Arduino IDE, compile and upload the code to your Arduino board. The folder LEDTest has the source code for testing the LED layers as they are being assembled.
- Make sure that the power switch of the LED Cube is turned on and that the 5V, 4A power supply is plugged into a wall outlet and into the LED Cube's power jack.
- All of the LEDs may turn on blue. This is normal.
- Attach a USB cable from your computer to your Arduino.
- Open the Arduino IDE.
- Click on File, Open and browse to where you saved the CubeObject folder.
- Click the Open button.
- You should have 18 file tabs open in the Arduino IDE.
- Click on Tools on the top menu bar.
- Slide the pointer down to Board:
- Select the Appropriate Arduino board.
- You should be using a 5V Arduino with a 16MHz crystal.
- Repeat step 7.
- Slide down to Port:
- Select the port that your Arduino is plugged into.
- Click on the Circle with the right pointing arrow in it to compile and start the upload.
- When the upload finishes.
- All of the LEDs will go out.
- The first Action will start running.
- A group of LEDs will start moving through the cube, from the first LED to the Last LED.
- A second group of LEDs will be moving in the opposite direction, starting from the last LED and moving towards the first LED.
- The LEDs will change color when they wrap around from last to first or first to last.
Sit back and marvel at your handiwork.
There are seven different Actions that the cube will do. Several of the Actions have multiple parts.
Step 29: How the Software Works
The software for the LED Cube was written in C++. C++ was used so that I could take advantage of inheritance and overloading. These are characteristics of object-oriented design. Basically, I realized that each of the actions (light patterns used by the cube) had a lot of code in common. To take advantage of this and keep the code relatively simple, I created a base class named Action.. The Action class has the methods that are common to all actions implemented and some methods that are declared, but have no code. To make a new action, I simply had to derive a new class from the base class and then fill in the methods that were not implemented in the base class. Deriving a class from another class is known as inheritance. The new class gets every method and variable of the parent class. You can add new methods or write a new implementation of an inherited method by simply defining a method in the new class that has the same signature as one in the parent class, but does something different. This is know as overloading.
The LED Cube uses a concept borrowed from video games, the sprite. A sprite is typically an on screen object that maintains its own position and color information. Each Action object in the LED Cube manipulates one or more sprites in unique ways. Therefore, each Action object has its own methods for moving sprites and adding sprites to the Cube.
The LEDs used in the cube are all wired in a daisy chain. The output of one LED is wired to the input of the next LED. The daisy chain wiring is serpentine across each layer and jumps up to the next layer when it gets to the end of a layer. The daisy chain consists of 64 LEDs. There is a simple Action class that moves a series of LEDs of the same color (a sprite), sequentially through the chain of LEDs. When it gets to the last LED it moves down the side of the cube and goes around again. Some other Actions move four LEDs as a group across a layer or up a face of the cube. These Actions do not follow the sequence of the daisy chain. Instead, they use a map. A map is an array of 64 bytes. The array is indexed by the current position of the the sprite and returns what the next position of the sprite should be. Using maps, it becomes quite simple to make complicated movements through the cube. Here is how the LEDs in the cube are numbered. Notice how the sequence goes up one column and down the next.
// The number represents the LED position in the strip, The layers are; //---- Layer 0 ---- + ---- Layer 1 ---- + ---- Layer 2 ---- + ---- Layer 3 ---- // 3, 4, 11, 12 | 28, 27, 20, 19 | 35, 36, 43, 44 | 60, 59, 52, 51 // 2, 5, 10, 13 | 29, 26, 21, 18 | 34, 37, 42, 45 | 61, 59, 52, 51 // 1, 6, 9, 14 | 30, 25, 22, 17 | 33, 38, 41, 46 | 62, 57, 54, 49 // 0, 7, 8, 15 | 31, 24, 23, 16 | 32, 39, 40, 47 | 63, 56, 55, 48
To make a light move around the circumference of the first layer, you would turn on and off the LED in each of these positions in this sequence; 0 -> 1 -> 2 -> 3 -> 4 -> 11 -> 12 -> 13 -> 14 -> 15 -> 8 -> 7 -> 0. LED[0] is on, then LED[0] is off and LED[1] is on. Then LED[1] is off and LED[2] is on... and so on.
The AtMega328p commonly used by Arduino boards has only 2K bytes of RAM memory. This is not a lot of RAM. Due to this, I wrote the code to keep all of the maps and sprite initializers in Flash memory and copy them to RAM when needed. Due to the the maps being in Flash memory, special instructions are required to access them. The keyword PROGMEM tells the compiler to place the data in Flash memory. The memcpy_P(pdst, psrc, len) function copies the data from Flash to RAM where it can be worked with. I also do not destroy the Action objects. Instead an array of object pointers keeps all of the Action objects alive. A variable is used to index the current Action. When the end of the array is reached the index is set back to the start of the array. An array of sprites is maintained in RAM. Each Action will initialize the sprites in the array that it will use. The size of the array is determined by the Action that uses the most sprites. All of this allows me to minimize the RAM footprint of the cube code. The result is that the LED Cube program uses about 36% of the Flash memory and 34% of the RAM memory. So there is plenty of Flash and RAM left to implement more Actions.
Step 30: How to Add Your Own Actions.
First you need to decide what your new Action will do, then figure out how to make it happen.
To add your own Actions do the following;
- Create two new files in the CubeObject folder.
- The files have the same name.
- My convention was to name the files ActionXXXX, where XXXX describes the action.
- One file gets the .cpp extension
- The .cpp file must have a #include "ActionXXXX.h" in it.
- The .cpp file has the code for the methods that are declared in the .h file.
- The other file gets a .h extension.
- The .h file has the class definition and any required #include statements.
- The files have the same name.
The class definition (.h file) should something look like this;
// ActionBrighten base class definition #ifndef ACTIONBRIGHTEN_H #define ACTIONBRIGHTEN_H #include "Action.h" // Action base class definitions class ActionBrighten : public Action { // class variables protected: // class methods public: void start(Sprite_t *ps); // start the action void addSprites(void); // add all sprites to the cube void moveSprite(void); // move the sprite within the cube }; // ActionBrighten class
Breaking it down;
This statement indicates that the parent class definition file is included in this file.
#include "Action.h" // Action base class definitions
This statement starts the class definition for ActionBrighten and indicates that it is derived from the Action class.
class ActionBrighten : public Action
These statements declare the signatures of the new or overloaded methods of the new class. In this case, start is a new method. The other two methods are overloads of methods in the parent (Action) class.
// class methods public: void start(Sprite_t *ps); // start the action void addSprites(void); // add all sprites to the cube void moveSprite(void); // move the sprite within the cube
The class implementation (.cpp file) looks something like this;
//****************************************************************************** // The ActionBrighten class fills the cube with a single color and then // brightens the color until MAXBRIGHTNESS is achieved. Then the cube dims // until all LEDs are blanked. Color is changed and the process continues. //****************************************************************************** // Action class implementation #include "ActionBrighten.h" // ActionBrighten class definition #define RANDOMDELAY 1000 #define NUMREVOLUTIONS 1 #define STEP 2 #define MAXBRIGHTNESS 64 // limit the brightness to 25% // ***** Sprite initializers ***** const Sprite_t Sprite[] PROGMEM = {{{.rgb = {0, 0, 0}}, 1, NUMLEDS, 0, FWD, 50}}; byte mode = 0; //****************************************************************************** // Start the action. //****************************************************************************** void ActionBrighten::start(Sprite_t *ps) { pSprites = ps; memcpy_P(pSprites, Sprite, sizeof(Sprite)); numSprites = 1; count = NUMREVOLUTIONS; // seed the random number generator randomSeed(analogRead(0)); } // start //****************************************************************************** // Fill the cube with one color //****************************************************************************** void ActionBrighten::addSprites(void) { for (int i = 0; i < NUMLEDS; i++) { xorSprite(pSprites, i); } // for } // addSprites //****************************************************************************** // Nothing moves. This will be invoked every RANDOMDELAY milliseconds. //****************************************************************************** void ActionBrighten::moveSprite(void) { int i; switch (mode) { case 0: // red brighten if (pSprites->led.rgb.red) { i = pSprites->led.rgb.red; i += STEP; if (i < MAXBRIGHTNESS) { pSprites->led.rgb.red = i; } else { pSprites->led.rgb.red = MAXBRIGHTNESS; mode++; } } else pSprites->led.rgb.red = STEP - 1; break; case 1: // red dim if (pSprites->led.rgb.red) { i = pSprites->led.rgb.red; i -= STEP; if (i > 0) { pSprites->led.rgb.red = i; } else { pSprites->led.rgb.red = 0; mode++; } } else pSprites->led.rgb.red = STEP - 1; break; case 2: // green brighten if (pSprites->led.rgb.green) { i = pSprites->led.rgb.green; i += STEP; if (i < MAXBRIGHTNESS) { pSprites->led.rgb.green = i; } else { pSprites->led.rgb.green = MAXBRIGHTNESS; mode++; } } else pSprites->led.rgb.green = STEP - 1; break; case 3: // green dim if (pSprites->led.rgb.green) { i = pSprites->led.rgb.green; i -= STEP; if (i > 0) { pSprites->led.rgb.green = i; } else { pSprites->led.rgb.green = 0; mode++; } } else pSprites->led.rgb.green = STEP - 1; break; case 4: // blue brighten if (pSprites->led.rgb.blue) { i = pSprites->led.rgb.blue; i += STEP; if (i < MAXBRIGHTNESS) { pSprites->led.rgb.blue = i; } else { pSprites->led.rgb.blue = MAXBRIGHTNESS; mode++; } } else pSprites->led.rgb.blue = STEP - 1; break; case 5: // blue dim if (pSprites->led.rgb.blue) { i = pSprites->led.rgb.blue; i -= STEP; if (i > 0) { pSprites->led.rgb.blue = i; } else { pSprites->led.rgb.blue = 0; mode = 0; count--; } } else pSprites->led.rgb.blue = STEP - 1; break; } // switch mode } // moveSprite
This statement includes the class definition file
#include "ActionBrighten.h" // ActionBrighten class definition
In this statement, ActionBrighten:: indicates that the method start is a member of the ActionBrighten class.
void ActionBrighten::start(Sprite_t *ps)
The start method initializes the sprites used by copying values from Flash memory into the array of sprites.
The addSprite method adds all of the sprites used by the class to the LED Cube's buffer. The sprites are xor added. Using xor will combine the colors of two or more sprites such that the common LEDs of the cube will show a color that is the combination of the colors of all the sprites involved. As the sprites separate their true colors will be restored. It is a very cool effect to have a blue and a red sprite collide, turn purple and then separate back into blue and red.
The moveSprite method would normally change the position of the sprite in the LED chain. However, for this class it changes the brightness of the LED by increasing or decreasing the value of each color used by the sprite.
Finally the new class is added to the array of ActionObjects. In the CubeObject.cpp file, add a #include for your new Action class. It should be added to this list of #include statements.
#include "ActionContinuous.h" // ActionCotinuous class definitions #include "ActionYaw.h" // ActionYaw class definitions #include "ActionPitch.h" // ActionPitch class definitions #include "ActionRoll.h" // ActionRoll class definitions #include "ActionSlide.h" // ActionSlide class definitions #include "ActionRandom.h" // ActionRandom class definitions #include "ActionBrighten.h" // ActionBrighten class definitions #include "ActionXXXX.h" // your new Action class definition file
Increase the value of NUMACTIONS from 7 to 8.
#define NUMACTIONS 8
Modify the setup() function to add the creation of your new class object to the array of Action objects.
void setup() { pinMode(DBGLED, OUTPUT); digitalWrite(DBGLED, LOW); pinMode(SWPIN, INPUT_PULLUP); Serial.begin(115200); randomSeed(analogRead(0)); Leds.begin(); ModeLed.begin(); // turn off all LEDs ModeLed.setPixelColor(0, 0, 0, 0); fillCube(0); showCube(); // allocate an array of pointers to each instantiated Action pActions[0] = new ActionContinuous(); pActions[1] = new ActionYaw(); pActions[2] = new ActionPitch(); pActions[3] = new ActionRoll(); pActions[4] = new ActionSlide(); pActions[5] = new ActionRandom(); pActions[6] = new ActionBrighten(); pActions[7] = new ActionXXXX(); // setup first action selectAction(); delay(100); } // Setup
That is it. Compile and upload the code to your Cube. Your new action will run after all the others. You can make your new Action first in the array by re-ordering the array.
// allocate an array of pointers to each instantiated Action pActions[0] = new ActionXXXX(); pActions[1] = new ActionContinuous(); pActions[2] = new ActionYaw(); pActions[3] = new ActionPitch(); pActions[4] = new ActionRoll(); pActions[5] = new ActionSlide(); pActions[6] = new ActionRandom(); pActions[7] = new ActionBrighten();
Change the value of NUMACTIONS to 1 and only your new Action will run.
#define NUMACTIONS 1
Step 31: Hand Made Soldering Jig.
Click on the SpacingJig.pdf file above to download it to your computer. The file is in color, so print it on a color printer if you have one. The file is also included in the GitHub download in the Diagrams folder.
To make your own soldering jig will need;
- 7/32 " drill bit
- A brad-point drill bit is preferred
- Drill press or Power Drill
- If you are going to use a Power Drill and you have a drill guide for it then I strongly recommend that you use it. It will help you to make holes that are more vertical than you can by drilling without one.
- Optional drill bit stop collar
- If you are using a Power Drill then use a stop collar to set the hole depth.
- Prick Punch or nail
- 4x4x3/4 inch block of wood, plywood or MDF
- This block is used to make the LED soldering jig
- At least one corner of the block should have a perfect 90 degree angle.
- Ideally, the sides of the block are parallel and all corners are 90 degrees.
- This will make it easier to make a proper grid with perpendicular lines.
- 2x3.5x3/4 inch block of wood, plywood or MDF.
- This block is used to make the spacers used for the final assembly of the LED cube.
- Optional color or monochrome printer
Cut the printed grid out, center it and glue it to your 4x4x3/4 inch block of plywood. Use a prick punch or a nail to make a small indent in the center of the blue circles, where the grid lines intersect. The indents will help to keep the drill bit from wandering. The closer you come to centering the holes in the blue circles, the better your finished cube will look.
If your holes are not lined up then your LEDs will not be inline.
If your holes are drilled at an angle, then your LEDs will be soldered at an angle.
If you are using a Drill Press;
- Chuck the 7/32" drill bit into your Drill Press
- Set the depth stop for a 7/16" deep hole.
- If you are using a metal cutting drill bit (not a brad point) set the depth using the point of the drill bit.
- If you are using a brad point drill bit then set the depth using the outside shoulders, not the brad point, of the drill bit.
- The objective is to have a hole that the APA106-F5 LED drops into. The bottom of the LED case should be about 0.2" below the surface of the block. Also, the depth of all the holes should be uniform. If the depth is not uniform then the LEDs in the layers will not be uniform.
If you are using a Power Drill;
- Chuck the 7/32" drill bit into your Power Drill
- Set the drill bit stop collar to drill a hole with a depth of 7/16"
- If you are using a metal cutting drill bit (not a brad point) set the depth using the point of the drill bit.
- If you are using a brad point drill bit then set the depth using the outside shoulders, not the brad point, of the drill bit.
- The objective is to have a hole that the APA106-F5 LED drops into. The bottom of the LED case should be about 0.2" below the surface of the block. Also, the depth of all the holes should be uniform. If the depth is not uniform then the LEDs in the layers will not be uniform.
- If you do not have a drill bit stop collar then wrap a piece of masking tape around the drill bit at 7/16".
- The tape will act as your depth stop, not the best way, but it is workable.
After drilling the LED holes, you can add brads or small nails to where the small black circles are located. Cut the heads off the nails. Leave about 1/4" sticking out of the jig. These will make it a little easier to bend the 22 awg bare wires to the required shapes. They will also help to keep the wires in place while you solder the LEDs to them. The heads are cutoff so that you can get a completed layer off of the jig. You can file the cutoff nails to remove any sharp edges.
Attachments
Step 32: Hand Made Spacers.
The 22 awg wire used to make the LED Cube is about 1/32" thick. The spacers will work best if the slots that are cut into them are about 1/32". So you are looking for a saw with the thinnest kerf. A hacksaw, coping saw or bandsaw would be best.
- Start with a block of wood that is about 3.5"x3"x1/2".
- The actual thickness does not matter, 3/4" or 1/4" will also work.
- The 3" width is the minimum, a little wider would not hurt.
- Make sure that the block has at least on square corner.
- Using a combination square, draw four lines spaced about 13/16" apart across the width of the block.
- Using the saw with the thinnest kerf, cut a slot on one of the lines that is at least 1/6" deep.
- Repeat step 4 for each of the other four lines
- Using whatever saw you have available, rip the block into six pieces that are all about 3/8" wide.
- These cuts should be made perpendicular to the slots that you cut.
- When you are done you should have a minimum of four spacers. Six is ideal.
By starting with a single block and cutting all of the slots across the width of the block and then ripping the block to make the spacers should result in a set of spacers with the slots in the same places. This will help you to make a more uniform, better looking LED cube.
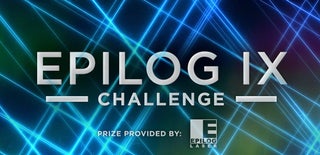
Participated in the
Epilog Challenge 9
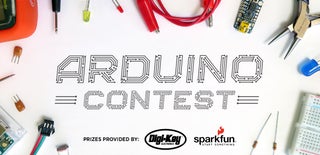
Participated in the
Arduino Contest 2017