Introduction: Custom Character Generator (Adafruit HT16k33 Matrix)
Printing Special Characters on LCDs and Led Matrices is a great fun. The procedure to print special characters or custom characters is to generate an array with binary values for each row and column. It may be hectic to find the correct code for any custom character, hence, this project will automate and generate code for an 8x8 led matrix and will also print the custom character on Adafruit HT16k33 8x8 Bicolor Matrix.
Adafruit HT16k33, a 1.2'' 8x8 Bicolor Led Matrix communicates with Arduino via an I2C communication protocol.
According to Adafruit, " This version of the LED backpack is designed for the 1.2" 8x8 matrices. They measure only 1.2"x1.2" so its a shame to use a massive array of chips to control it. This backpack solves the annoyance of using 16 pins or a bunch of chips by having an I2C constant-current matrix controller sit neatly on the back of the PCB. The controller chip takes care of everything, drawing all 64 LEDs in the background. All you have to do is write data to it using the 2-pin I2C interface. There are two address select pins so you can select one of 8 addresses to control up to 8 of these on a single 2-pin I2C bus (as well as whatever other I2C chips or sensors you like). The driver chip can 'dim' the entire display from 1/16 brightness up to full brightness in 1/16th steps. It cannot dim individual LEDs, only the entire display at once."
In this instructable, I will explain to you how to get the code for any custom character in real time and print that character on Led Matrix.
Step 1: Components
This instructable is a basic project on Adafruit HT16k33 Matrix. You need:
- Adafruit HT16k33 1.2'' x 1.2'' 8x8 Bicolor Led Matrix.
- Arduino (any variant but Uno is preferred).
- Breadboard
- Power Supply
Step 2: Schematic
Wiring Adafruit HT16k33 Led matrix is very easy as we need to connect the clock and data pin as we usually do for I2C devices. Connections will be like:
- SCL (Clock pin of Matrix) connected to A5 (Clock pin of Arduino Uno. Refer datasheet for other variants of Arduino)
- SDA (Data pin of Matrix) connected to A4. (Refer datasheet for other variants of Arduino)
- VCC connected to 5V.
- GND connected to 0V.
You can also consult the schematic shown in the figure.
Step 3: Code
Arduino Code
Firstly, we will include all the libraries required.
- Wire.h :- For I2C communication
- Adafruit_LedBackpack
- Adafruit_GFX
All these libraries are available in Arduino IDE itself. You just have to install them from Library Manager. Sketch>>Include Library >> Manage Libraries
Setup function ()
Setting an unsigned integer 8-bit array to strore8 binary values for 8 rows, of 8 bits each (8 columns). Set the address for I2C communication.
Loop function ()
As we need to print the character, we need the code for the character in real time. The most convenient method is to send the code serially and Arduino will read the serial data and print the character accordingly. Sending an array serially may be a hectic job, hence we can send a string with all 8 codes (8 bits each) separated by commas.
Reading Serial String :
if (Serial.available()>0)<br> { data=Serial.readStringUntil('\n'); Serial.println(data); }
After reading the text, we need to decode this string and get back the binary values. As we know, the format of input string will always be same. We can code it to find substrings and convert the strings to their decimal equivalent values. Then we will pass the generated decimal array (uint8_t) to print the character on matrix.
Converting String of 8 bits to decimal :
int val(String str)<br>{ int v=0; for (int i=0;i<8;i++) { if (str[i]=='1') { v=v+power(2,(7-i)); } } return v; }
For evaluating decimal equivalent using power function(pow ()), you need to deal with double type values and hence we can write our own power function as:
int power(int base,int exponent)<br>{ int c=1; for (int i=0;i { c=c*base; } return c; }
Now, at last, we will write the code to print the character using the generated array of 8 decimal values (one for each row).
void print_emoji( uint8_t emoji[],String color)<br>{ matrix.clear(); if (color=="red") { matrix.drawBitmap(0, 0,emoji, 8, 8, LED_RED); } else { matrix.drawBitmap(0, 0,emoji, 8, 8, LED_GREEN); } matrix.writeDisplay(); delay(500); }
You will easily understand this code as we are clearing the matrix first and then displaying the character using emoji array using matrix.drawBitmap() function. Do not forget to write "matrix.writeDisplay()" after all formatting as this function will only display all the formattings done so far on matrix.
Now you can send the string with all code values and Arduino will print the character on the matrix. You can download the Arduino code from below. For experimental purpose, you can write
B00111100,B01000010,B10100101,B10000001,B10100101,B10011001,B01000010,B00111100
this string in Serial Monitor and can see the character on matrix.
Now, we need to send the serial data automatically from a software when we press "Print" button. For automating this, we will make a demo 8x8 matrix and we will provide a facility to user to choose which cells should be colored and then the software will automatically generate the code and send the data serially to Arduino in string format. I chose Processing for the rest of my work. In processing, we can make a matrix using 64 buttons (rectangles with pressed function) and can assign a particular value and color at the start (Let it be the white color with value 0). Now whenever the button is pressed, we will convert the color of the button to black and set the value to 1. If user press same button again, its value will again change to 0 and color back to white. This will help the user to change the code again and again easily and can make amendments easily without erasing the whole matrix again. On the click of "Print" button, we will make a string similar to one shown above for the demo. Then the string will be sent to the particular serial port.
You can download the processing code from below. This is my first code in processing. Suggestions for the enhanced way of coding are highly appreciated.
You can see how the GUI looks like and how the character is created using the system. It will hardly take seconds to create the same character on matrix.
You can download the code and images of this project from my GitHub Repository .
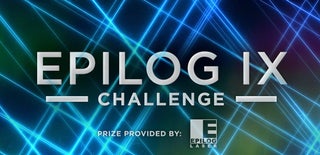
Participated in the
Epilog Challenge 9