Introduction: DIY Weather Station Using DHT11, BMP180, Nodemcu With Arduino IDE Over Blynk Server
Github: DIY_Weather_Station
Hackster.io: Weather Station
You would have seen Weather Application right? Like, when you open it you get to know the weather conditions like Temperature, Humidity etc. Those readings are the average value of an a big area, so if you want to know the exact parameters related to your room, you can't just rely upon the Weather Application. For this purpose lets move on to the making of Weather Station which is cost effective, and is also reliable and gives us the accurate value.
A weather station is a facility with instruments and equipment for measuring atmospheric conditions to provide information for weather forecasts and to study the weather and climate. It requires little bit of effort to plug and code. So lets get started.
About Nodemcu:
NodeMCU is an open source IoT platform.
It includes firmware which runs on the ESP8266 Wi-Fi SoC from Espressif Systems, and hardware which is based on the ESP-12 module.
The term "NodeMCU" by default refers to the firmware rather than the dev kits. The firmware uses the Lua scripting language. It is based on the eLua project, and built on the Espressif Non-OS SDK for ESP8266. It uses many open source projects, such as lua-cjson, and spiffs.
Sensors and Software requirement:
1. Nodemcu (esp8266-12e v1.0)
2. DHT11
3. BMP180
4. Arduino IDE
Step 1: Know Your Sensors
BMP180:
Description:
The BMP180 consists of a piezo-resistive sensor, an analog to digital converter and a control unit
with E2PROM and a serial I2C interface. The BMP180 delivers the uncompensated value of pressure and temperature. The E2PROM has stored 176 bit of individual calibration data. This is used to compensate offset, temperature dependence and other parameters of the sensor.
- UP = pressure data (16 to 19 bit)
- UT = temperature data (16 bit)
Technical Specs:
- Vin: 3 to 5VDC
- Logic: 3 to 5V compliant
- Pressure sensing range: 300-1100 hPa (9000m to -500m above sea level)
- Up to 0.03hPa / 0.25m resolution-40 to +85°C operational range, +-2°C temperature accuracy
- This board/chip uses I2C 7-bit address 0x77.
DHT11:
Description:
- The DHT11 is a basic, ultra low-cost digital temperature and humidity sensor.
- It uses a capacitive humidity sensor and a thermistor to measure the surrounding air, and spits out a digital signal on the data pin (no analog input pins needed). Its fairly simple to use, but requires careful timing to grab data.
- The only real downside of this sensor is you can only get new data from it once every 2 seconds, so when using our library, sensor readings can be up to 2 seconds old.
Technical Specs:
- 3 to 5V power and I/O
- Good for 0-50°C temperature readings ±2°C accuracy
- Good for 20-80% humidity readings with 5% accuracy
- 2.5 mA max current use during conversion (while requesting data)
Step 2: Connectivity
- DHT11 with Nodemcu:
Pin 1 - 3.3V
Pin 2 - D4
Pin 3 - NC
Pin 4 - Gnd
- BMP180 with Nodemcu:
Vin - 3.3V
Gnd - Gnd
SCL - D6
SDA - D7
Step 3: Setup Blynk
What is Blynk?
Blynk is a Platform with iOS and Android apps to control Arduino, Raspberry Pi and the likes over the Internet.
It's a digital dashboard where you can build a graphic interface for your project by simply dragging and dropping widgets. It's really simple to set everything up and you'll start tinkering in less than 5 mins. Blynk is not tied to some specific board or shield. Instead, it's supporting hardware of your choice. Whether your Arduino or Raspberry Pi is linked to the Internet over Wi-Fi, Ethernet or this new ESP8266 chip, Blynk will get you online and ready for the Internet Of Your Things.
For more info in setting up Blynk: Detailed Blynk Setup
Step 4: Code
//Comments for each line is given in the .ino file below #include <Adafruit_Sensor.h> #define BLYNK_PRINT Serial #include <DHT.h> #include <ESP8266WiFi.h> #include <BlynkSimpleEsp8266.h> #include <Wire.h> #include <Adafruit_BMP085.h> Adafruit_BMP085 bmp; #define I2C_SCL 12 #define I2C_SDA 13 float dst,bt,bp,ba; char dstmp[20],btmp[20],bprs[20],balt[20]; bool bmp085_present=true; char auth[]="Put your Authication key from the Blynk app here"; char ssid[] = "Your WiFi SSID"; char pass[] = "Your Password"; #define DHTPIN 2 #define DHTTYPE DHT11 DHT dht(DHTPIN, DHTTYPE); //Defining the pin and the dhttype BlynkTimer timer; void sendSensor() { if (!bmp.begin()) { Serial.println("Could not find a valid BMP085 sensor, check wiring!"); while (1) {} } float h = dht.readHumidity(); float t = dht.readTemperature(); if (isnan(h) || isnan(t)) { Serial.println("Failed to read from DHT sensor!"); return; } double gamma = log(h/100) + ((17.62*t) / (243.5+t)); double dp = 243.5*gamma / (17.62-gamma); float bp = bmp.readPressure()/100; float ba = bmp.readAltitude(); float bt = bmp.readTemperature(); float dst = bmp.readSealevelPressure()/100; Blynk.virtualWrite(V5 , h); Blynk.virtualWrite(V6 , t); Blynk.virtualWrite(V10, bp); Blynk.virtualWrite(V11, ba); Blynk.virtualWrite(V12, bt); Blynk.virtualWrite(V13, dst); Blynk.virtualWrite(V14, dp); } void setup() { Serial.begin(9600); Blynk.begin(auth, ssid, pass); dht.begin(); Wire.begin(I2C_SDA, I2C_SCL); delay(10); timer.setInterval(1000L, sendSensor); } void loop() { Blynk.run(); timer.run(); }
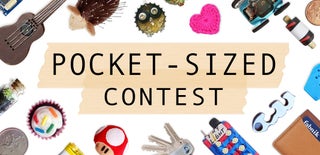
Participated in the
Pocket-Sized Contest
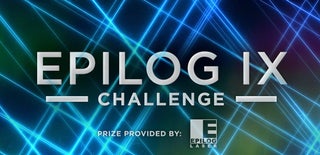
Participated in the
Epilog Challenge 9